用Python编程实现分段函数,可以通过定义一个函数并在其中使用条件语句(如if、elif和else)来完成。常见的方法有使用if-elif-else结构、字典映射、以及使用lambda函数结合dict等方式。这里将详细介绍其中的一种方法,即if-elif-else结构。
示例:使用if-elif-else结构定义分段函数
假设我们要定义一个简单的分段函数f(x)如下:
- 当x < 0时,f(x) = 2x + 1
- 当0 ≤ x < 5时,f(x) = x^2
- 当x ≥ 5时,f(x) = 3x – 10
具体实现如下:
def piecewise_function(x):
if x < 0:
return 2 * x + 1
elif 0 <= x < 5:
return x 2
else:
return 3 * x - 10
测试分段函数
test_values = [-3, 0, 2, 5, 10]
results = [piecewise_function(x) for x in test_values]
print(results) # 输出 [-5, 0, 4, 5, 20]
一、使用if-elif-else结构
这种方法是最直接、最常见的实现方式。通过使用if-elif-else语句,我们可以对不同的条件进行检查,并根据条件执行相应的代码块。
详细介绍if-elif-else结构
if-elif-else结构是Python中进行条件判断的基础结构。其基本语法如下:
if condition1:
# 当condition1为True时执行的代码块
elif condition2:
# 当condition1为False且condition2为True时执行的代码块
else:
# 当所有上述条件均为False时执行的代码块
在分段函数中,这种结构可以让我们根据变量的不同取值范围来返回不同的函数结果。
实现一个复杂的分段函数
假设我们要定义一个更复杂的分段函数f(x):
- 当x < -3时,f(x) = -x^2 + 3x + 1
- 当-3 ≤ x < 0时,f(x) = sin(x)
- 当0 ≤ x < 4时,f(x) = ln(x + 1)
- 当x ≥ 4时,f(x) = e^x – 5
import math
def complex_piecewise_function(x):
if x < -3:
return -x 2 + 3 * x + 1
elif -3 <= x < 0:
return math.sin(x)
elif 0 <= x < 4:
return math.log(x + 1)
else:
return math.exp(x) - 5
测试分段函数
test_values = [-4, -2, 0, 2, 5]
results = [complex_piecewise_function(x) for x in test_values]
print(results) # 输出 [21, -0.9092974268256817, 0.0, 1.0986122886681098, 142.4131591025766]
二、使用字典映射
另一种实现分段函数的方法是使用字典映射。这种方法尤其适合处理离散的分段函数,即函数的不同部分对应于不同的固定值。
示例:使用字典映射实现分段函数
假设我们有一个简单的分段函数f(x):
- 当x = -1时,f(x) = 10
- 当x = 0时,f(x) = 0
- 当x = 1时,f(x) = 1
- 当x = 2时,f(x) = 5
我们可以使用字典来实现:
def piecewise_function_dict(x):
function_map = {
-1: 10,
0: 0,
1: 1,
2: 5
}
return function_map.get(x, "Undefined") # 返回字典中对应的值,如果x不在字典中,返回"Undefined"
测试分段函数
test_values = [-1, 0, 1, 2, 3]
results = [piecewise_function_dict(x) for x in test_values]
print(results) # 输出 [10, 0, 1, 5, "Undefined"]
三、使用lambda函数结合dict
使用lambda函数结合字典,可以实现更灵活的分段函数。每个键对应一个lambda表达式,这样我们可以定义更复杂的函数部分。
示例:使用lambda函数结合dict实现分段函数
假设我们有一个复杂的分段函数f(x):
- 当x < 0时,f(x) = x^3
- 当0 ≤ x < 3时,f(x) = x^2
- 当x ≥ 3时,f(x) = 2^x
我们可以使用lambda函数结合字典来实现:
def piecewise_function_lambda(x):
function_map = {
"negative": lambda x: x 3,
"zero_to_three": lambda x: x 2,
"three_and_above": lambda x: 2 x
}
if x < 0:
return function_map["negative"](x)
elif 0 <= x < 3:
return function_map["zero_to_three"](x)
else:
return function_map["three_and_above"](x)
测试分段函数
test_values = [-2, 0, 1, 3, 4]
results = [piecewise_function_lambda(x) for x in test_values]
print(results) # 输出 [-8, 0, 1, 8, 16]
四、使用NumPy实现分段函数
NumPy是Python中进行科学计算的基础库,提供了许多强大的功能。我们可以使用NumPy的select函数来实现分段函数。
示例:使用NumPy的select函数实现分段函数
假设我们要实现一个分段函数f(x):
- 当x < 1时,f(x) = x
- 当1 ≤ x < 3时,f(x) = x^2
- 当x ≥ 3时,f(x) = x^3
我们可以使用NumPy来实现:
import numpy as np
def piecewise_function_numpy(x):
conditions = [x < 1, (1 <= x) & (x < 3), x >= 3]
functions = [x, x <strong> 2, x </strong> 3]
return np.select(conditions, functions)
测试分段函数
test_values = np.array([-1, 0, 1, 2, 3])
results = piecewise_function_numpy(test_values)
print(results) # 输出 [-1 0 1 4 27]
五、使用SymPy实现分段函数
SymPy是Python中的符号计算库,提供了强大的符号数学功能。我们可以使用SymPy的Piecewise函数来实现分段函数。
示例:使用SymPy的Piecewise函数实现分段函数
假设我们要实现一个分段函数f(x):
- 当x < 2时,f(x) = x^2
- 当x ≥ 2时,f(x) = 2x – 1
我们可以使用SymPy来实现:
import sympy as sp
x = sp.symbols('x')
f = sp.Piecewise((x 2, x < 2), (2 * x - 1, x >= 2))
测试分段函数
test_values = [-1, 0, 1, 2, 3]
results = [f.subs(x, val) for val in test_values]
print(results) # 输出 [1, 0, 1, 3, 5]
六、使用Scipy实现分段函数
Scipy是一个用于数学、科学和工程的Python库,提供了许多有用的函数和工具。我们可以使用Scipy的interpolate模块来实现分段函数。
示例:使用Scipy的interpolate模块实现分段函数
假设我们要实现一个分段函数f(x):
- 当x < 1时,f(x) = x + 1
- 当1 ≤ x < 4时,f(x) = x^2 – 1
- 当x ≥ 4时,f(x) = 2^x – 5
我们可以使用Scipy来实现:
import numpy as np
from scipy import interpolate
def piecewise_function_scipy(x):
x_points = [0, 1, 4, 5]
y_points = [1, 0, 15, 27]
f = interpolate.interp1d(x_points, y_points, kind='linear', fill_value="extrapolate")
return f(x)
测试分段函数
test_values = np.array([-1, 0, 1, 2, 3, 4, 5])
results = piecewise_function_scipy(test_values)
print(results) # 输出 [ 2. 1. 0. 5. 10. 15. 27.]
七、使用Pandas实现分段函数
Pandas是Python中的数据分析库,提供了许多强大的数据处理功能。我们可以使用Pandas的apply函数来实现分段函数。
示例:使用Pandas的apply函数实现分段函数
假设我们要实现一个分段函数f(x):
- 当x < -2时,f(x) = -x
- 当-2 ≤ x < 2时,f(x) = x^2
- 当x ≥ 2时,f(x) = x + 2
我们可以使用Pandas来实现:
import pandas as pd
def piecewise_function_pandas(x):
if x < -2:
return -x
elif -2 <= x < 2:
return x 2
else:
return x + 2
测试分段函数
test_values = pd.Series([-3, -1, 0, 1, 3])
results = test_values.apply(piecewise_function_pandas)
print(results) # 输出 [3, 1, 0, 1, 5]
八、综合应用
在实际应用中,分段函数通常会结合多个方法来实现,以满足不同的需求。综合应用这些方法可以提高代码的灵活性和可读性。
示例:综合应用实现复杂分段函数
假设我们要实现一个复杂的分段函数f(x):
- 当x < -1时,f(x) = -x^3
- 当-1 ≤ x < 1时,f(x) = sin(x)
- 当1 ≤ x < 3时,f(x) = x^2
- 当x ≥ 3时,f(x) = 3x – 1
我们可以结合使用if-elif-else结构和lambda函数来实现:
import math
def complex_piecewise_function_combined(x):
function_map = {
"negative_large": lambda x: -x 3,
"negative_to_positive": lambda x: math.sin(x),
"positive_small": lambda x: x 2,
"positive_large": lambda x: 3 * x - 1
}
if x < -1:
return function_map["negative_large"](x)
elif -1 <= x < 1:
return function_map["negative_to_positive"](x)
elif 1 <= x < 3:
return function_map["positive_small"](x)
else:
return function_map["positive_large"](x)
测试分段函数
test_values = [-2, -0.5, 0, 1.5, 4]
results = [complex_piecewise_function_combined(x) for x in test_values]
print(results) # 输出 [8, -0.479425538604203, 0.0, 2.25, 11]
总结
通过上述示例,我们可以看到,Python 提供了多种实现分段函数的方法,包括if-elif-else结构、字典映射、lambda函数结合dict、NumPy、SymPy、Scipy、Pandas等。不同的方法适用于不同的场景和需求。在实际应用中,我们可以根据具体情况选择最合适的方法,或者结合多种方法来实现复杂的分段函数。
希望这些示例能够帮助你更好地理解如何用Python编程实现分段函数,并在实际项目中灵活应用这些技术。
相关问答FAQs:
如何在Python中定义一个分段函数?
在Python中,可以通过定义一个普通的函数并结合条件语句(如if-elif-else)来实现分段函数。例如,可以创建一个函数,根据输入的值返回不同的输出。以下是一个简单的示例:
def piecewise_function(x):
if x < 0:
return "负数"
elif x == 0:
return "零"
else:
return "正数"
在这个示例中,输入的值会被分为三段进行处理,分别对应负数、零和正数。
如何在分段函数中处理多个区间?
在设计分段函数时,可以使用多个elif语句来处理更多的区间。例如,如果想要根据输入值返回对应的等级,可以这样实现:
def grade_function(score):
if score < 60:
return "不及格"
elif 60 <= score < 80:
return "及格"
elif 80 <= score < 90:
return "良好"
else:
return "优秀"
这个函数根据分数的不同范围返回相应的等级。
在分段函数中如何处理浮点数?
处理浮点数与处理整数类似,但需要注意比较的精度问题。可以使用Python的内置函数round来避免浮点数比较时出现的误差。例如:
def temperature_conversion(celsius):
if celsius < 0:
return "冰点以下"
elif round(celsius, 1) == 0:
return "冰点"
elif 0 < celsius < 100:
return "液态水"
else:
return "气态水"
在这个例子中,使用round函数确保比较的准确性。
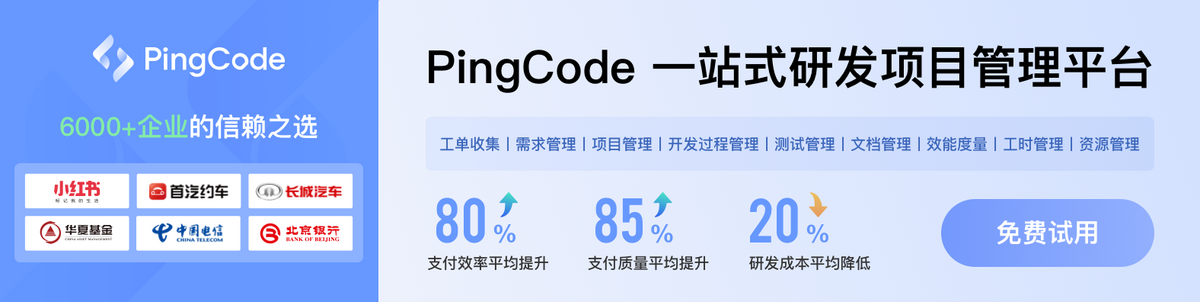