实现Python可视化的方法有很多,主要包括使用Matplotlib、Seaborn、Plotly、Bokeh等库。 在这些工具中,Matplotlib是最基础和广泛使用的,它为其他高级库提供了很多基础功能。Seaborn基于Matplotlib,提供了更高级和美观的统计图表。Plotly和Bokeh则用于创建交互式和动态的图表。
Matplotlib是Python中最基础的绘图库之一,功能强大,适用于各种静态图表。以下是如何使用Matplotlib实现可视化的详细描述。
一、MATPLOTLIB
1、安装和导入Matplotlib
要使用Matplotlib,首先需要安装它。可以使用以下命令:
pip install matplotlib
安装完成后,可以在Python脚本或Jupyter Notebook中导入:
import matplotlib.pyplot as plt
2、绘制基本图形
Matplotlib可以绘制各种基本图形,如折线图、柱状图、散点图等。下面是一些基本的示例。
折线图:
import matplotlib.pyplot as plt
数据
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
绘制折线图
plt.plot(x, y)
添加标题和标签
plt.title('Basic Line Plot')
plt.xlabel('X Axis')
plt.ylabel('Y Axis')
显示图形
plt.show()
柱状图:
import matplotlib.pyplot as plt
数据
categories = ['A', 'B', 'C', 'D']
values = [4, 7, 1, 8]
绘制柱状图
plt.bar(categories, values)
添加标题和标签
plt.title('Basic Bar Plot')
plt.xlabel('Category')
plt.ylabel('Value')
显示图形
plt.show()
散点图:
import matplotlib.pyplot as plt
数据
x = [5, 7, 8, 7, 2, 17, 2, 9, 4, 11]
y = [99, 86, 87, 88, 100, 86, 103, 87, 94, 78]
绘制散点图
plt.scatter(x, y)
添加标题和标签
plt.title('Basic Scatter Plot')
plt.xlabel('X Axis')
plt.ylabel('Y Axis')
显示图形
plt.show()
3、定制图形
Matplotlib提供了丰富的定制功能,可以调整图形的样式、颜色、网格、注释等。
调整颜色和样式:
import matplotlib.pyplot as plt
数据
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
绘制折线图,设置颜色和样式
plt.plot(x, y, color='green', linestyle='--', marker='o')
添加标题和标签
plt.title('Customized Line Plot')
plt.xlabel('X Axis')
plt.ylabel('Y Axis')
显示图形
plt.show()
添加网格和注释:
import matplotlib.pyplot as plt
数据
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
绘制折线图
plt.plot(x, y, marker='o')
添加网格
plt.grid(True)
添加注释
plt.annotate('Highest Point', xy=(5, 11), xytext=(4, 10),
arrowprops=dict(facecolor='black', shrink=0.05))
添加标题和标签
plt.title('Line Plot with Grid and Annotation')
plt.xlabel('X Axis')
plt.ylabel('Y Axis')
显示图形
plt.show()
二、SEABORN
Seaborn是基于Matplotlib的高级库,提供了更美观和复杂的统计图表。
1、安装和导入Seaborn
同样地,首先需要安装Seaborn:
pip install seaborn
安装完成后,导入Seaborn:
import seaborn as sns
import matplotlib.pyplot as plt
2、基本用法
Seaborn的基本用法与Matplotlib类似,但提供了更多的默认样式和颜色配置。
绘制箱线图:
import seaborn as sns
import matplotlib.pyplot as plt
加载示例数据集
tips = sns.load_dataset('tips')
绘制箱线图
sns.boxplot(x='day', y='total_bill', data=tips)
添加标题
plt.title('Box Plot of Total Bill by Day')
显示图形
plt.show()
绘制热图:
import seaborn as sns
import matplotlib.pyplot as plt
加载示例数据集
flights = sns.load_dataset('flights')
flights_pivot = flights.pivot('month', 'year', 'passengers')
绘制热图
sns.heatmap(flights_pivot, annot=True, fmt='d', cmap='YlGnBu')
添加标题
plt.title('Heatmap of Flight Passengers')
显示图形
plt.show()
三、PLOTLY
Plotly是一个用于创建交互式图表的库,适用于Web应用和数据仪表盘。
1、安装和导入Plotly
安装Plotly:
pip install plotly
导入Plotly:
import plotly.express as px
2、基本用法
Plotly的基本用法与Matplotlib和Seaborn有所不同,主要通过函数接口生成图表,并支持交互功能。
绘制交互式折线图:
import plotly.express as px
数据
df = px.data.gapminder().query("country == 'Canada'")
绘制交互式折线图
fig = px.line(df, x='year', y='gdpPercap', title='GDP per Capita in Canada')
显示图形
fig.show()
绘制交互式散点图:
import plotly.express as px
数据
df = px.data.iris()
绘制交互式散点图
fig = px.scatter(df, x='sepal_width', y='sepal_length', color='species',
title='Scatter Plot of Iris Dataset')
显示图形
fig.show()
四、BOKEH
Bokeh是另一个用于创建交互式图表的库,特别适用于大规模数据集和实时数据流。
1、安装和导入Bokeh
安装Bokeh:
pip install bokeh
导入Bokeh:
from bokeh.plotting import figure, show, output_file
2、基本用法
Bokeh的基本用法与其他库类似,通过创建图表对象并添加数据来绘制图形。
绘制交互式折线图:
from bokeh.plotting import figure, show, output_file
输出文件
output_file("line.html")
创建图表对象
p = figure(title="Simple Line Plot", x_axis_label='x', y_axis_label='y')
添加数据
x = [1, 2, 3, 4, 5]
y = [6, 7, 2, 4, 5]
p.line(x, y, legend_label="Temp.", line_width=2)
显示图形
show(p)
绘制交互式散点图:
from bokeh.plotting import figure, show, output_file
输出文件
output_file("scatter.html")
创建图表对象
p = figure(title="Simple Scatter Plot", x_axis_label='x', y_axis_label='y')
添加数据
x = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
y = [6, 7, 2, 4, 5, 6, 7, 8, 9, 10]
p.circle(x, y, size=10, color="navy", alpha=0.5)
显示图形
show(p)
五、总结
Python提供了丰富的可视化工具和库,Matplotlib适合静态图表,功能全面且灵活;Seaborn基于Matplotlib,适合统计图表;Plotly和Bokeh则适合交互式和动态图表。根据不同的需求和数据特点,选择合适的可视化库,可以更好地呈现和分析数据。
相关问答FAQs:
如何选择适合的Python可视化库?
在Python中,有许多可视化库可供选择,包括Matplotlib、Seaborn、Plotly和Bokeh等。选择合适的库通常取决于您的需求。例如,Matplotlib适合基本的图形绘制,而Seaborn则提供更美观的统计图表。如果需要交互性,Plotly和Bokeh是更好的选择。了解每个库的特点,有助于您做出明智的选择。
如何在Python中处理大型数据集以实现可视化?
处理大型数据集时,建议使用Pandas库进行数据清理和处理。通过将数据加载到DataFrame中,您可以轻松地进行过滤、分组和聚合操作。在准备好数据后,可以使用上述提到的可视化库进行图表绘制。此外,考虑使用数据抽样或聚合技术,以提高可视化的性能和速度。
如何将Python可视化嵌入到网页中?
要将Python可视化嵌入到网页中,可以使用Dash和Flask等框架。Dash是一个专门用于构建Web应用的框架,支持交互式图表的创建,非常适合数据可视化。通过Flask,您可以将生成的图表作为图像或HTML文件嵌入到网页中。确保您熟悉HTML和JavaScript,以增强您的可视化效果和用户体验。
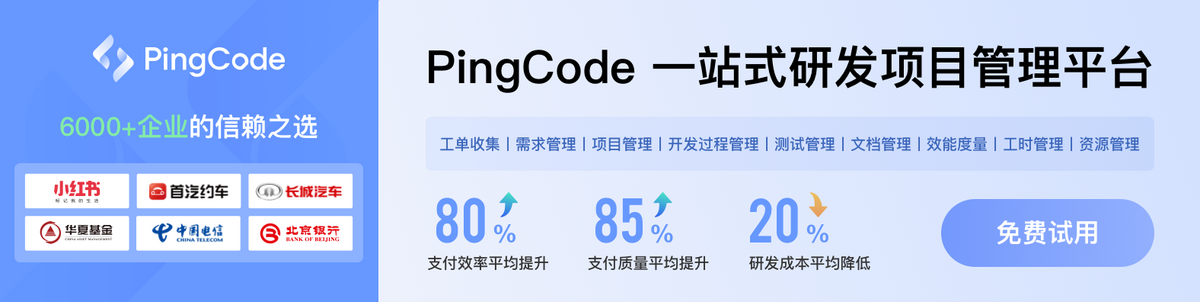