Python使用if语句来检查文件的存在、类型或内容,关键步骤包括使用os模块进行文件操作、通过if语句进行条件判断、以及处理可能出现的异常。 例如,可以使用os.path.exists()来检查文件是否存在,os.path.isfile()来判断是否为文件。详细描述一下如何使用os.path.exists()来检查文件是否存在。
如果要检查一个文件是否存在,可以使用os.path.exists()函数。这个函数会返回True或False,分别表示文件存在与否。下面是一个示例代码:
import os
file_path = 'example.txt'
if os.path.exists(file_path):
print(f'The file {file_path} exists.')
else:
print(f'The file {file_path} does not exist.')
在这个示例中,我们导入了os模块,然后定义了一个文件路径变量file_path。接着使用os.path.exists()函数检查文件是否存在,并根据检查结果输出相应的消息。
一、文件存在性检查
文件存在性检查是文件操作中的基础步骤,在Python中可以通过os模块和if语句组合来实现。os模块提供了多种方法,可以帮助我们检测文件或目录的存在性。
1、os.path.exists()
os.path.exists()函数用于检查文件或目录是否存在。它会返回一个布尔值:如果路径存在,则返回True;否则返回False。
import os
file_path = 'example.txt'
if os.path.exists(file_path):
print(f'The file {file_path} exists.')
else:
print(f'The file {file_path} does not exist.')
在这个示例中,我们使用os.path.exists()函数检查了文件example.txt是否存在,并根据检查结果输出相应的消息。
2、os.path.isfile()和os.path.isdir()
os.path.isfile()和os.path.isdir()函数分别用于检查路径是否为文件和是否为目录。这些函数在文件操作中也非常有用。
import os
file_path = 'example.txt'
dir_path = 'example_directory'
if os.path.isfile(file_path):
print(f'The file {file_path} is a file.')
else:
print(f'The file {file_path} is not a file.')
if os.path.isdir(dir_path):
print(f'The directory {dir_path} is a directory.')
else:
print(f'The directory {dir_path} is not a directory.')
在这个示例中,我们使用os.path.isfile()和os.path.isdir()函数分别检查了路径是否为文件和是否为目录,并根据检查结果输出相应的消息。
二、文件读写操作
文件读写操作是文件操作的核心部分,在Python中可以使用内置的open()函数来实现。通过open()函数,可以以不同的模式打开文件,例如读模式('r')、写模式('w')和追加模式('a')。
1、读取文件
读取文件可以使用open()函数以读模式('r')打开文件,然后使用read()、readline()或readlines()方法读取文件内容。
file_path = 'example.txt'
if os.path.exists(file_path):
with open(file_path, 'r') as file:
content = file.read()
print(content)
else:
print(f'The file {file_path} does not exist.')
在这个示例中,我们首先检查文件是否存在,然后以读模式打开文件,并使用read()方法读取文件内容,最后输出内容。
2、写入文件
写入文件可以使用open()函数以写模式('w')或追加模式('a')打开文件,然后使用write()或writelines()方法写入内容。
file_path = 'example.txt'
content = 'Hello, world!'
with open(file_path, 'w') as file:
file.write(content)
print(f'Content written to {file_path}.')
在这个示例中,我们以写模式打开文件,并使用write()方法将内容写入文件。写模式会覆盖文件的原有内容,如果文件不存在,则会创建一个新文件。
三、异常处理
在文件操作过程中,可能会遇到各种异常情况,例如文件不存在、权限不足等。为了使程序更加健壮,可以使用try-except语句来捕获和处理这些异常。
1、捕获文件不存在异常
当尝试打开一个不存在的文件时,会引发FileNotFoundError异常。可以使用try-except语句来捕获并处理这个异常。
file_path = 'nonexistent.txt'
try:
with open(file_path, 'r') as file:
content = file.read()
print(content)
except FileNotFoundError:
print(f'The file {file_path} does not exist.')
在这个示例中,我们使用try-except语句捕获了FileNotFoundError异常,并输出相应的消息。
2、捕获权限不足异常
当尝试打开一个权限不足的文件时,会引发PermissionError异常。可以使用try-except语句来捕获并处理这个异常。
file_path = 'protected.txt'
try:
with open(file_path, 'r') as file:
content = file.read()
print(content)
except PermissionError:
print(f'Permission denied to open the file {file_path}.')
在这个示例中,我们使用try-except语句捕获了PermissionError异常,并输出相应的消息。
四、文件路径操作
文件路径操作在文件管理中非常重要,Python的os.path模块提供了一系列函数,可以帮助我们进行路径操作,例如获取文件名、目录名、文件扩展名等。
1、获取文件名和目录名
os.path.basename()和os.path.dirname()函数分别用于获取路径的文件名和目录名。
file_path = '/path/to/example.txt'
file_name = os.path.basename(file_path)
dir_name = os.path.dirname(file_path)
print(f'File name: {file_name}')
print(f'Directory name: {dir_name}')
在这个示例中,我们使用os.path.basename()和os.path.dirname()函数分别获取了路径的文件名和目录名,并输出结果。
2、获取文件扩展名
os.path.splitext()函数用于获取文件的扩展名。该函数会返回一个包含路径和扩展名的元组。
file_path = 'example.txt'
path, extension = os.path.splitext(file_path)
print(f'Path: {path}')
print(f'Extension: {extension}')
在这个示例中,我们使用os.path.splitext()函数获取了文件的扩展名,并输出结果。
五、文件复制和移动
文件复制和移动是文件管理中的常见操作,Python的shutil模块提供了简单的方法来实现这些操作。
1、文件复制
shutil.copy()函数用于复制文件。该函数会复制文件的内容和权限。
import shutil
src_path = 'example.txt'
dst_path = 'example_copy.txt'
shutil.copy(src_path, dst_path)
print(f'File copied from {src_path} to {dst_path}.')
在这个示例中,我们使用shutil.copy()函数将文件example.txt复制到了example_copy.txt,并输出结果。
2、文件移动
shutil.move()函数用于移动文件或目录。该函数会将文件或目录移动到指定位置。
import shutil
src_path = 'example.txt'
dst_path = 'moved_example.txt'
shutil.move(src_path, dst_path)
print(f'File moved from {src_path} to {dst_path}.')
在这个示例中,我们使用shutil.move()函数将文件example.txt移动到了moved_example.txt,并输出结果。
六、删除文件和目录
删除文件和目录是文件管理中的最后一步操作,Python的os模块和shutil模块提供了相关方法来实现这些操作。
1、删除文件
os.remove()函数用于删除文件。该函数会删除指定路径的文件。
import os
file_path = 'example.txt'
if os.path.exists(file_path):
os.remove(file_path)
print(f'The file {file_path} has been deleted.')
else:
print(f'The file {file_path} does not exist.')
在这个示例中,我们使用os.remove()函数删除了文件example.txt,并输出结果。
2、删除目录
os.rmdir()和shutil.rmtree()函数分别用于删除空目录和非空目录。
import os
import shutil
删除空目录
empty_dir_path = 'empty_directory'
if os.path.exists(empty_dir_path):
os.rmdir(empty_dir_path)
print(f'The directory {empty_dir_path} has been deleted.')
else:
print(f'The directory {empty_dir_path} does not exist.')
删除非空目录
non_empty_dir_path = 'non_empty_directory'
if os.path.exists(non_empty_dir_path):
shutil.rmtree(non_empty_dir_path)
print(f'The directory {non_empty_dir_path} has been deleted.')
else:
print(f'The directory {non_empty_dir_path} does not exist.')
在这个示例中,我们使用os.rmdir()函数删除了空目录empty_directory,并使用shutil.rmtree()函数删除了非空目录non_empty_directory,最终输出结果。
七、文件属性操作
文件属性操作是文件管理中的一个重要方面,Python的os模块提供了获取和修改文件属性的方法,例如文件大小、修改时间等。
1、获取文件大小
os.path.getsize()函数用于获取文件的大小(以字节为单位)。
file_path = 'example.txt'
if os.path.exists(file_path):
file_size = os.path.getsize(file_path)
print(f'The size of the file {file_path} is {file_size} bytes.')
else:
print(f'The file {file_path} does not exist.')
在这个示例中,我们使用os.path.getsize()函数获取了文件example.txt的大小,并输出结果。
2、获取文件修改时间
os.path.getmtime()函数用于获取文件的最后修改时间(以时间戳形式返回)。
import os
import time
file_path = 'example.txt'
if os.path.exists(file_path):
modification_time = os.path.getmtime(file_path)
readable_time = time.ctime(modification_time)
print(f'The last modification time of the file {file_path} is {readable_time}.')
else:
print(f'The file {file_path} does not exist.')
在这个示例中,我们使用os.path.getmtime()函数获取了文件example.txt的最后修改时间,并将其转换为可读格式后输出结果。
八、文件权限操作
文件权限操作涉及到对文件的访问权限进行管理,Python的os模块提供了修改文件权限的方法,例如改变文件的读写权限。
1、修改文件权限
os.chmod()函数用于修改文件的权限。可以使用八进制数来表示权限,例如0o644表示所有者可读写,组和其他用户只读。
import os
file_path = 'example.txt'
if os.path.exists(file_path):
os.chmod(file_path, 0o644)
print(f'The permissions of the file {file_path} have been changed.')
else:
print(f'The file {file_path} does not exist.')
在这个示例中,我们使用os.chmod()函数将文件example.txt的权限修改为0o644,并输出结果。
2、获取文件权限
os.stat()函数用于获取文件的状态信息,其中包含文件的权限信息。可以使用st_mode属性来获取文件的权限。
import os
import stat
file_path = 'example.txt'
if os.path.exists(file_path):
file_stat = os.stat(file_path)
file_mode = stat.filemode(file_stat.st_mode)
print(f'The permissions of the file {file_path} are {file_mode}.')
else:
print(f'The file {file_path} does not exist.')
在这个示例中,我们使用os.stat()函数获取了文件example.txt的状态信息,并提取文件的权限信息后输出结果。
九、总结
通过上述各个方面的介绍,可以看出Python在文件管理方面提供了丰富的功能。使用os模块和shutil模块,可以轻松实现文件的存在性检查、读写操作、异常处理、路径操作、复制移动、删除、属性操作和权限操作等任务。 这些功能的结合使用,可以帮助我们更高效地进行文件管理,提高程序的健壮性和可维护性。
十、实战应用
为了更好地理解和掌握这些文件操作方法,我们可以通过一个实战应用来综合运用这些知识。例如,我们可以编写一个文件管理工具,实现文件的备份、恢复和日志记录等功能。
1、文件备份
文件备份是文件管理中的一个重要任务,可以通过复制文件的方式实现。
import os
import shutil
import time
def backup_file(file_path, backup_dir):
if os.path.exists(file_path):
backup_file_name = f"{os.path.basename(file_path)}_{time.strftime('%Y%m%d%H%M%S')}.bak"
backup_file_path = os.path.join(backup_dir, backup_file_name)
shutil.copy(file_path, backup_file_path)
print(f'File {file_path} has been backed up to {backup_file_path}.')
else:
print(f'The file {file_path} does not exist.')
file_path = 'example.txt'
backup_dir = 'backup'
if not os.path.exists(backup_dir):
os.makedirs(backup_dir)
backup_file(file_path, backup_dir)
在这个示例中,我们定义了一个backup_file()函数,用于将文件备份到指定目录。备份文件名包含时间戳,以避免文件名冲突。
2、文件恢复
文件恢复是文件管理中的另一个重要任务,可以通过复制备份文件的方式实现。
def restore_file(backup_file_path, restore_path):
if os.path.exists(backup_file_path):
shutil.copy(backup_file_path, restore_path)
print(f'File {backup_file_path} has been restored to {restore_path}.')
else:
print(f'The backup file {backup_file_path} does not exist.')
backup_file_path = 'backup/example.txt_20231010120000.bak'
restore_path = 'example_restored.txt'
restore_file(backup_file_path, restore_path)
在这个示例中,我们定义了一个restore_file()函数,用于将备份文件恢复到指定路径。
3、日志记录
日志记录是文件管理中的一个辅助功能,可以通过将操作记录写入日志文件的方式实现。
def log_operation(log_file, operation):
with open(log_file, 'a') as file:
file.write(f'{time.strftime("%Y-%m-%d %H:%M:%S")} - {operation}\n')
log_file = 'operations.log'
log_operation(log_file, 'Backup example.txt')
log_operation(log_file, 'Restore example.txt')
在这个示例中,我们定义了一个log_operation()函数,用于将操作记录写入日志文件。每条日志记录包含时间戳和操作描述。
通过这些实战应用示例,可以更好地理解和掌握Python文件操作的各种方法和技巧。希望通过本文的介绍,能够帮助读者在实际项目中更高效地进行文件管理。
相关问答FAQs:
如何在Python中检查文件是否存在?
在Python中,可以使用os
模块中的path.exists()
函数来检查一个文件是否存在。示例如下:
import os
file_path = 'your_file.txt'
if os.path.exists(file_path):
print("文件存在")
else:
print("文件不存在")
这个方法非常有效,适用于任何文件路径的检查。
在Python中如何读取文件内容?
要读取文件内容,可以使用open()
函数搭配read()
或readlines()
方法。例如:
with open('your_file.txt', 'r') as file:
content = file.read()
print(content)
使用with
语句可以确保文件在读取后自动关闭,避免资源泄露。
如何在Python中创建并写入文件?
可以使用open()
函数的写入模式来创建或打开文件并写入内容。示例代码如下:
with open('new_file.txt', 'w') as file:
file.write("Hello, World!")
如果new_file.txt
不存在,Python会自动创建它;如果存在,内容将被覆盖。
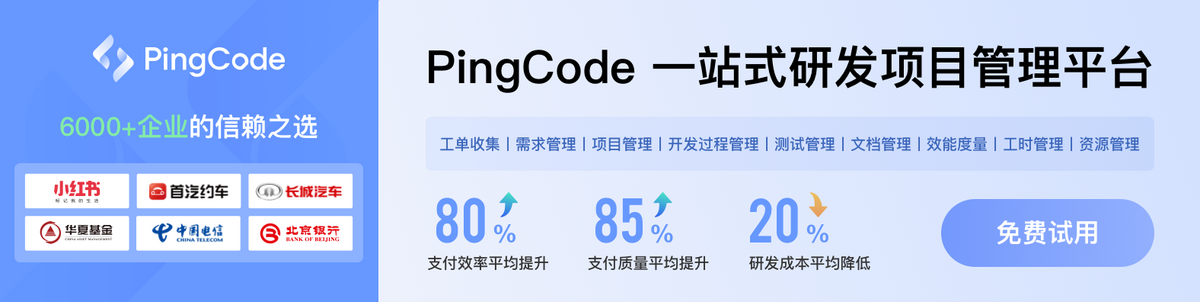