一、Python如何搜索美女图片:使用爬虫技术、调用搜索引擎API、使用图像识别技术、利用第三方图片库、使用自动化脚本。其中,使用爬虫技术是比较常见且有效的一种方法。通过编写Python爬虫脚本,可以自动化地访问网页,解析网页内容,并下载符合条件的图片。这种方法灵活性高,但需要处理反爬虫机制和合法性问题。
爬虫技术
爬虫技术是通过编写程序模拟浏览器的行为,从网络上获取数据的一种方法。在Python中,可以使用诸如Requests库和BeautifulSoup库来实现爬虫功能。
使用Requests库
Requests是一个非常流行的HTTP库,使用起来非常简单。可以用它来发送HTTP请求,并获取网页内容。
import requests
url = "https://www.example.com"
response = requests.get(url)
if response.status_code == 200:
print("Request successful")
print(response.text)
else:
print("Request failed")
上述代码展示了如何使用Requests库发送一个GET请求,并检查请求是否成功。
使用BeautifulSoup库
BeautifulSoup是一个用于解析HTML和XML文档的库。与Requests库结合使用,可以解析网页内容并提取需要的信息。
from bs4 import BeautifulSoup
url = "https://www.example.com"
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
images = soup.find_all('img')
for img in images:
print(img['src'])
else:
print("Request failed")
上述代码展示了如何使用BeautifulSoup库解析网页内容,并提取所有图片的URL。
搜索引擎API
利用搜索引擎API,如Google Custom Search API,可以实现图片搜索功能。这种方法需要注册API Key,并按照API文档进行编程。
使用Google Custom Search API
首先,需要在Google Developer Console注册并获取API Key。然后,可以使用Google Custom Search API进行图片搜索。
import requests
api_key = "YOUR_API_KEY"
search_engine_id = "YOUR_SEARCH_ENGINE_ID"
query = "美女图片"
url = f"https://www.googleapis.com/customsearch/v1?q={query}&cx={search_engine_id}&key={api_key}&searchType=image"
response = requests.get(url)
if response.status_code == 200:
results = response.json()
for item in results['items']:
print(item['link'])
else:
print("Request failed")
上述代码展示了如何使用Google Custom Search API进行图片搜索,并提取搜索结果中的图片URL。
图像识别技术
图像识别技术可以用于过滤和分类图片。使用诸如TensorFlow或OpenCV库,可以实现图像识别功能。
使用TensorFlow
TensorFlow是一个开源的机器学习框架,可以用于图像识别和分类。
import tensorflow as tf
Load a pre-trained model
model = tf.keras.applications.MobileNetV2(weights='imagenet', include_top=True)
Load and preprocess an image
image = tf.keras.preprocessing.image.load_img('image.jpg', target_size=(224, 224))
image = tf.keras.preprocessing.image.img_to_array(image)
image = tf.expand_dims(image, axis=0)
image = tf.keras.applications.mobilenet_v2.preprocess_input(image)
Predict the class of the image
predictions = model.predict(image)
decoded_predictions = tf.keras.applications.mobilenet_v2.decode_predictions(predictions, top=1)
print(decoded_predictions)
上述代码展示了如何使用TensorFlow进行图像识别,并解码预测结果。
利用第三方图片库
一些第三方图片库,如Unsplash、Pexels等,提供了丰富的图片资源和API接口。可以通过调用这些API进行图片搜索和下载。
使用Pexels API
首先,需要在Pexels网站上注册并获取API Key。然后,可以使用Pexels API进行图片搜索。
import requests
api_key = "YOUR_API_KEY"
url = "https://api.pexels.com/v1/search"
headers = {
"Authorization": api_key
}
params = {
"query": "美女",
"per_page": 15
}
response = requests.get(url, headers=headers, params=params)
if response.status_code == 200:
results = response.json()
for photo in results['photos']:
print(photo['src']['original'])
else:
print("Request failed")
上述代码展示了如何使用Pexels API进行图片搜索,并提取搜索结果中的图片URL。
使用自动化脚本
通过使用自动化脚本,可以模拟用户行为,实现图片搜索和下载功能。常用的工具包括Selenium和PyAutoGUI。
使用Selenium
Selenium是一个用于Web应用程序测试的工具,可以模拟用户行为进行自动化测试。
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
import time
driver = webdriver.Chrome()
url = "https://www.google.com"
driver.get(url)
search_box = driver.find_element(By.NAME, "q")
search_box.send_keys("美女图片")
search_box.send_keys(Keys.RETURN)
time.sleep(2)
images_link = driver.find_element(By.LINK_TEXT, "Images")
images_link.click()
time.sleep(2)
images = driver.find_elements(By.CSS_SELECTOR, "img")
for img in images:
print(img.get_attribute("src"))
driver.quit()
上述代码展示了如何使用Selenium模拟用户行为,在Google上搜索图片,并提取搜索结果中的图片URL。
处理反爬虫机制
在进行爬虫操作时,可能会遇到反爬虫机制。为了绕过这些机制,可以采取一些策略,如使用代理、设置请求头、模拟用户行为等。
使用代理
通过使用代理,可以隐藏真实的IP地址,从而绕过反爬虫机制。
proxies = {
"http": "http://your_proxy:port",
"https": "https://your_proxy:port"
}
response = requests.get(url, proxies=proxies)
if response.status_code == 200:
print("Request successful")
else:
print("Request failed")
上述代码展示了如何使用代理进行HTTP请求。
设置请求头
通过设置请求头,可以模拟真实的浏览器请求,从而绕过反爬虫机制。
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36"
}
response = requests.get(url, headers=headers)
if response.status_code == 200:
print("Request successful")
else:
print("Request failed")
上述代码展示了如何设置请求头进行HTTP请求。
模拟用户行为
通过模拟用户行为,可以绕过一些基于行为分析的反爬虫机制。可以使用Selenium等工具实现这一功能。
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
import time
driver = webdriver.Chrome()
url = "https://www.example.com"
driver.get(url)
模拟用户滚动页面
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
time.sleep(2)
模拟用户点击
element = driver.find_element(By.XPATH, "//button[text()='Load more']")
element.click()
time.sleep(2)
driver.quit()
上述代码展示了如何使用Selenium模拟用户行为,滚动页面并点击按钮。
合法性问题
在进行图片搜索和下载时,需要注意合法性问题。确保不侵犯版权和隐私权,并遵守相关法律法规。
遵守版权法
在使用他人图片时,应确保获得授权或使用具有开放版权的图片。可以选择使用Creative Commons许可的图片,或从公开图片库中获取图片。
# 示例:使用Creative Commons许可的图片
cc_image_url = "https://creativecommons.org/licenses/by/4.0/"
response = requests.get(cc_image_url)
if response.status_code == 200:
print("Request successful")
else:
print("Request failed")
保护隐私权
在搜索和使用图片时,应注意保护个人隐私权。避免使用含有个人信息的图片,或未经同意使用他人肖像。
# 示例:过滤含有个人信息的图片
images = ["image1.jpg", "image2.jpg"]
for img in images:
# 假设check_privacy是一个检测图片隐私信息的函数
if check_privacy(img):
print(f"Image {img} contains personal information, skipping.")
else:
print(f"Image {img} is safe to use.")
总结
通过使用爬虫技术、调用搜索引擎API、使用图像识别技术、利用第三方图片库、使用自动化脚本等方法,可以实现Python搜索美女图片的功能。每种方法都有其优缺点和适用场景。在实际应用中,可以根据具体需求和情况选择合适的方法。同时,需要注意处理反爬虫机制和合法性问题,确保爬虫操作的顺利进行和合法合规。
使用爬虫技术
爬虫技术灵活性高,可以获取任意网站的图片。但需要处理反爬虫机制,如使用代理、设置请求头、模拟用户行为等。同时,需要注意爬虫操作的合法性,避免侵犯版权和隐私权。
调用搜索引擎API
调用搜索引擎API,如Google Custom Search API,可以快速实现图片搜索功能。这种方法操作简单,但需要注册API Key,并受限于API的使用限制和配额。
使用图像识别技术
图像识别技术可以用于过滤和分类图片,确保获取的图片符合预期。可以使用TensorFlow、OpenCV等库实现图像识别功能。但需要具备一定的机器学习和图像处理知识。
利用第三方图片库
利用第三方图片库,如Unsplash、Pexels等,可以方便地获取高质量的图片资源。这种方法操作简单,但需要注册API Key,并受限于图片库的使用限制和配额。
使用自动化脚本
使用自动化脚本,如Selenium,可以模拟用户行为,实现图片搜索和下载功能。这种方法灵活性高,但需要处理反爬虫机制和合法性问题。
最佳实践
在实际应用中,可以结合多种方法,选择最合适的方案。例如,可以先使用搜索引擎API获取图片URL,然后使用爬虫技术下载图片,并使用图像识别技术进行过滤和分类。同时,注意处理反爬虫机制,确保爬虫操作的顺利进行。
示例代码
以下是一个综合示例,展示了如何结合多种方法实现Python搜索美女图片的功能。
import requests
from bs4 import BeautifulSoup
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
import time
import tensorflow as tf
搜索引擎API
def search_images(query, api_key, search_engine_id):
url = f"https://www.googleapis.com/customsearch/v1?q={query}&cx={search_engine_id}&key={api_key}&searchType=image"
response = requests.get(url)
if response.status_code == 200:
results = response.json()
image_urls = [item['link'] for item in results['items']]
return image_urls
else:
print("Request failed")
return []
下载图片
def download_image(url, filename):
response = requests.get(url)
if response.status_code == 200:
with open(filename, 'wb') as f:
f.write(response.content)
print(f"Image downloaded: {filename}")
else:
print("Request failed")
图像识别
def classify_image(image_path, model):
image = tf.keras.preprocessing.image.load_img(image_path, target_size=(224, 224))
image = tf.keras.preprocessing.image.img_to_array(image)
image = tf.expand_dims(image, axis=0)
image = tf.keras.applications.mobilenet_v2.preprocess_input(image)
predictions = model.predict(image)
decoded_predictions = tf.keras.applications.mobilenet_v2.decode_predictions(predictions, top=1)
return decoded_predictions
主函数
def main():
api_key = "YOUR_API_KEY"
search_engine_id = "YOUR_SEARCH_ENGINE_ID"
query = "美女图片"
model = tf.keras.applications.MobileNetV2(weights='imagenet', include_top=True)
# 搜索图片
image_urls = search_images(query, api_key, search_engine_id)
# 下载并分类图片
for i, url in enumerate(image_urls):
filename = f"image_{i}.jpg"
download_image(url, filename)
predictions = classify_image(filename, model)
print(predictions)
if __name__ == "__main__":
main()
上述代码展示了如何结合搜索引擎API、爬虫技术和图像识别技术,实现Python搜索美女图片的功能。通过调用Google Custom Search API搜索图片,并使用Requests库下载图片,最后使用TensorFlow进行图像识别和分类。这样可以确保获取的图片符合预期,并且操作合法合规。
相关问答FAQs:
如何使用Python爬取网络上的美女图片?
使用Python爬虫库(如Requests和BeautifulSoup)可以轻松实现图片的爬取。首先,通过Requests库向目标网页发送请求,获取网页的HTML内容。接着,利用BeautifulSoup解析HTML,找到包含图片的标签,并提取图片的URL。最后,将这些图片下载到本地。务必遵守网站的使用条款和robots.txt文件,以确保合法性。
是否有推荐的Python库用于处理图片下载?
当然,除了Requests和BeautifulSoup外,Pillow是一个非常实用的库,能够帮助您处理图片的格式转换、调整大小等操作。此外,使用os库可以方便地进行文件的存储和管理。结合这些库,您可以高效地下载和处理美女图片。
在搜索美女图片时,有哪些注意事项?
在进行图片搜索时,确保遵循版权法是非常重要的。选择公共领域或者具有共享许可的图片,以避免侵权问题。此外,使用一些API(如Unsplash API或Pexels API)可以获取高质量的免费图片,确保您在合法的范围内使用这些资源。
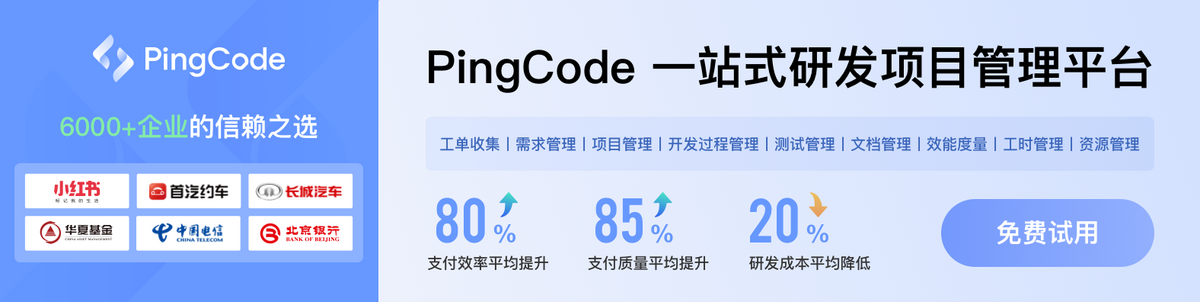