使用Python进行词频统计的方法主要有:读取文本内容、分词、统计词频、输出结果。其中,分词是一个关键步骤,它将文本按单词进行分割;而统计词频则是通过字典或集合等数据结构来实现的。下面,我们将详细介绍这些步骤并提供相关代码示例。
一、读取文本内容
首先,我们需要读取文本内容。可以从文件、网页等多种来源读取文本数据。在这里,我们假设文本数据存储在一个本地文件中,并使用Python内置的open
函数来读取文本内容。
def read_text(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
text = file.read()
return text
二、分词
分词是将文本分割成一个个单词的过程。在英文文本中,分词相对简单,可以直接使用空格和标点符号进行分割。而对于中文等语言,分词则复杂得多,通常需要借助专业的分词工具,如jieba
。
英文文本分词示例:
import re
def tokenize(text):
# 使用正则表达式去除标点符号,只保留单词和数字
words = re.findall(r'\b\w+\b', text.lower())
return words
中文文本分词示例:
import jieba
def tokenize(text):
words = jieba.lcut(text)
return words
三、统计词频
统计词频可以使用Python的字典或collections.Counter
类来实现。collections.Counter
类是专门用来计数的容器,使用起来更加简便。
from collections import Counter
def count_words(words):
word_counts = Counter(words)
return word_counts
四、输出结果
最后,我们可以将词频统计结果输出,可以打印在控制台,也可以写入文件或可视化展示。
将结果打印在控制台:
def print_word_counts(word_counts, top_n=10):
# 打印前top_n个高频词
for word, count in word_counts.most_common(top_n):
print(f'{word}: {count}')
将结果写入文件:
def write_word_counts_to_file(word_counts, file_path):
with open(file_path, 'w', encoding='utf-8') as file:
for word, count in word_counts.items():
file.write(f'{word}: {count}\n')
五、完整代码示例
以下是完整的代码示例,展示了如何读取文本、分词、统计词频并输出结果:
import re
from collections import Counter
读取文本内容
def read_text(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
text = file.read()
return text
分词
def tokenize(text):
words = re.findall(r'\b\w+\b', text.lower())
return words
统计词频
def count_words(words):
word_counts = Counter(words)
return word_counts
打印词频统计结果
def print_word_counts(word_counts, top_n=10):
for word, count in word_counts.most_common(top_n):
print(f'{word}: {count}')
将词频统计结果写入文件
def write_word_counts_to_file(word_counts, file_path):
with open(file_path, 'w', encoding='utf-8') as file:
for word, count in word_counts.items():
file.write(f'{word}: {count}\n')
主函数
def main(file_path, output_file_path):
text = read_text(file_path)
words = tokenize(text)
word_counts = count_words(words)
print_word_counts(word_counts)
write_word_counts_to_file(word_counts, output_file_path)
示例调用
if __name__ == "__main__":
input_file = 'example.txt'
output_file = 'word_counts.txt'
main(input_file, output_file)
六、进一步优化
在实际应用中,我们可能需要对词频统计进行进一步优化和扩展,例如:
- 去除停用词:停用词是一些在文本中频繁出现但无实际意义的词,如“the”、“and”等。可以通过自定义停用词列表来过滤掉这些词。
- 处理大小写:在英文文本中,大小写不同的单词实际上是同一个词,可以统一转换为小写或大写。
- 词形还原:对于英文文本,可以使用词形还原(lemmatization)技术将不同形式的单词还原为其原形,如将“running”还原为“run”。
去除停用词示例:
def remove_stopwords(words, stopwords):
filtered_words = [word for word in words if word not in stopwords]
return filtered_words
示例停用词列表
stopwords = {'the', 'and', 'is', 'in', 'to', 'of'}
词形还原示例:
import nltk
from nltk.stem import WordNetLemmatizer
nltk.download('wordnet')
def lemmatize_words(words):
lemmatizer = WordNetLemmatizer()
lemmatized_words = [lemmatizer.lemmatize(word) for word in words]
return lemmatized_words
七、可视化词频统计结果
将词频统计结果可视化可以帮助我们更直观地理解数据。常用的可视化工具包括matplotlib
和wordcloud
。
使用matplotlib
绘制词频柱状图:
import matplotlib.pyplot as plt
def plot_word_counts(word_counts, top_n=10):
common_words = word_counts.most_common(top_n)
words, counts = zip(*common_words)
plt.bar(words, counts)
plt.xlabel('Words')
plt.ylabel('Counts')
plt.title('Top Words by Frequency')
plt.show()
使用wordcloud
绘制词云图:
from wordcloud import WordCloud
def generate_wordcloud(word_counts):
wordcloud = WordCloud(width=800, height=400, background_color='white').generate_from_frequencies(word_counts)
plt.figure(figsize=(10, 5))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
八、总结
通过本文,我们介绍了使用Python进行词频统计的完整流程,包括读取文本内容、分词、统计词频和输出结果。我们还讨论了进一步优化和扩展的方法,如去除停用词、词形还原以及可视化词频统计结果。希望本文能帮助读者更好地理解和掌握词频统计技术,并应用于实际项目中。
相关问答FAQs:
如何使用Python进行文本词频统计?
在Python中,词频统计的基本步骤包括读取文本数据、分词、统计每个词的出现次数。可以使用内置的collections.Counter
类来简化统计过程。首先,读取文本文件内容,然后使用split()
方法分割文本,接着通过Counter
类统计每个词的频率,最后输出结果。
有哪些Python库可以帮助进行词频统计?
Python中有多种库可以帮助进行词频统计。nltk
(自然语言工具包)提供了丰富的文本处理功能,包括分词和去除停用词;pandas
可以用来处理数据表格,方便统计和可视化;sklearn
中的CountVectorizer
也可以有效地进行词频统计。
如何处理文本中的停用词和标点符号?
在进行词频统计时,处理停用词(如“的”、“是”等)和标点符号是非常重要的。可以使用nltk.corpus.stopwords
来获取常见的停用词列表,并通过正则表达式或string
库中的punctuation
来清洗文本,确保统计结果的准确性。通过这些方式,可以提高词频统计的有效性和可靠性。
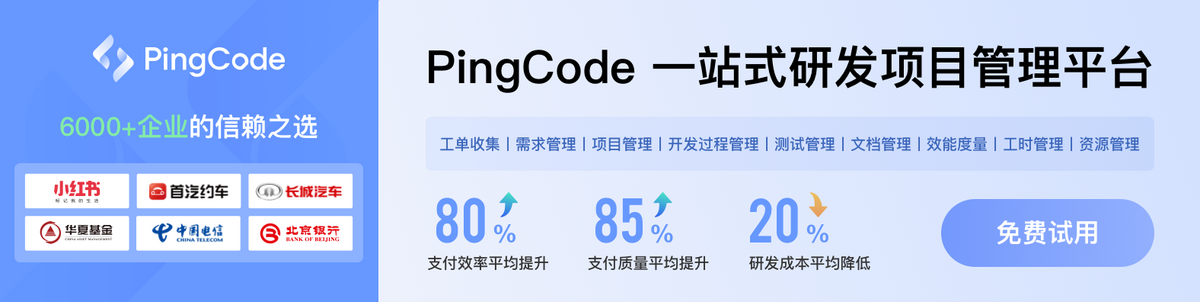