要在Python中链接外部文件,可以使用多种方式,包括读取文件、写入文件、使用外部库等方法。下面将重点介绍如何读取文件这一点。
读取文件
在Python中读取文件通常使用内置的open()
函数。这个函数可以打开一个文件,并返回一个文件对象,然后可以使用这个文件对象来读取文件的内容。以下是详细步骤和示例:
# 打开文件
file = open('example.txt', 'r') # 'r'表示以读模式打开
读取文件内容
content = file.read()
打印文件内容
print(content)
关闭文件
file.close()
在上面的示例中,open('example.txt', 'r')
表示以读模式打开名为example.txt
的文件,file.read()
读取整个文件的内容,最后用file.close()
关闭文件以释放资源。
一、文件模式
文件模式决定了你对文件的操作类型,常见的模式有:
- 'r': 读模式 (默认模式)
- 'w': 写模式 (会覆盖文件内容)
- 'a': 追加模式 (在文件末尾添加内容)
- 'b': 二进制模式 (与其他模式结合使用,如 'rb', 'wb')
二、读取文件内容
除了read()
方法,Python还提供了其他几种方法来读取文件内容:
1. read(size)
方法
read(size)
方法读取指定大小的内容,如果不指定大小,则读取整个文件。例如:
file = open('example.txt', 'r')
content = file.read(100) # 读取前100个字符
print(content)
file.close()
2. readline()
方法
readline()
方法一次读取文件的一行内容。例如:
file = open('example.txt', 'r')
line = file.readline() # 读取第一行
print(line)
file.close()
3. readlines()
方法
readlines()
方法一次读取文件的所有行,并返回一个包含每行内容的列表。例如:
file = open('example.txt', 'r')
lines = file.readlines() # 读取所有行
for line in lines:
print(line)
file.close()
三、写入文件
要将数据写入文件,可以使用write()
或writelines()
方法。需要注意的是,在写模式下打开文件时,如果文件不存在会创建一个新文件,如果文件存在会覆盖其内容。
1. write()
方法
write()
方法将字符串写入文件。例如:
file = open('example.txt', 'w')
file.write('Hello, World!')
file.close()
2. writelines()
方法
writelines()
方法将一个字符串列表写入文件。例如:
lines = ['Hello, World!\n', 'Python is great!\n']
file = open('example.txt', 'w')
file.writelines(lines)
file.close()
四、使用上下文管理器
使用with
语句可以更加简洁地管理文件的打开和关闭,确保文件在使用完后自动关闭。例如:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
五、处理CSV文件
CSV文件是一种常见的数据格式,Python的csv
模块提供了方便的方法来读取和写入CSV文件。
1. 读取CSV文件
import csv
with open('example.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
2. 写入CSV文件
import csv
data = [['Name', 'Age'], ['Alice', 30], ['Bob', 25]]
with open('example.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerows(data)
六、处理JSON文件
JSON文件是一种轻量级的数据交换格式,Python的json
模块提供了方便的方法来读取和写入JSON文件。
1. 读取JSON文件
import json
with open('example.json', 'r') as file:
data = json.load(file)
print(data)
2. 写入JSON文件
import json
data = {'Name': 'Alice', 'Age': 30}
with open('example.json', 'w') as file:
json.dump(data, file)
七、处理Excel文件
Excel文件在数据处理和分析中也非常常见,可以使用pandas
库来方便地读取和写入Excel文件。
1. 读取Excel文件
import pandas as pd
df = pd.read_excel('example.xlsx')
print(df)
2. 写入Excel文件
import pandas as pd
data = {'Name': ['Alice', 'Bob'], 'Age': [30, 25]}
df = pd.DataFrame(data)
df.to_excel('example.xlsx', index=False)
八、处理文本文件
文本文件是最常见的文件类型之一,可以使用多种方式来处理它们。
1. 逐行读取
with open('example.txt', 'r') as file:
for line in file:
print(line.strip())
2. 逐行写入
lines = ['Hello, World!', 'Python is great!']
with open('example.txt', 'w') as file:
for line in lines:
file.write(line + '\n')
九、文件路径和目录操作
Python的os
模块提供了丰富的文件和目录操作功能。
1. 获取当前工作目录
import os
current_directory = os.getcwd()
print(current_directory)
2. 改变工作目录
import os
os.chdir('/path/to/directory')
3. 列出目录内容
import os
directory_contents = os.listdir('/path/to/directory')
print(directory_contents)
4. 创建新目录
import os
os.mkdir('/path/to/new_directory')
5. 删除文件
import os
os.remove('example.txt')
十、文件异常处理
在文件操作过程中,可能会遇到各种异常情况,如文件不存在、权限不足等。可以使用try-except
语句来处理这些异常。
try:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
except FileNotFoundError:
print('File not found')
except PermissionError:
print('Permission denied')
十一、处理二进制文件
有时需要处理二进制文件,如图像、音频等。可以使用'b'模式来处理这些文件。
1. 读取二进制文件
with open('example.png', 'rb') as file:
data = file.read()
print(data)
2. 写入二进制文件
with open('example_copy.png', 'wb') as file:
file.write(data)
十二、文件对象方法
文件对象提供了多种方法来操作文件,如tell()
、seek()
等。
1. tell()
方法
tell()
方法返回文件对象的当前位置。
with open('example.txt', 'r') as file:
content = file.read(10)
position = file.tell()
print(position)
2. seek()
方法
seek()
方法移动文件对象的位置。
with open('example.txt', 'r') as file:
file.seek(5)
content = file.read()
print(content)
十三、处理大文件
处理大文件时,可以使用逐行读取或分块读取的方法,以减少内存使用。
1. 逐行读取
with open('large_file.txt', 'r') as file:
for line in file:
process(line)
2. 分块读取
def read_in_chunks(file_object, chunk_size=1024):
while True:
data = file_object.read(chunk_size)
if not data:
break
yield data
with open('large_file.txt', 'r') as file:
for chunk in read_in_chunks(file):
process(chunk)
十四、总结
通过以上内容,我们详细介绍了Python中链接外部文件的各种方法和技巧。包括读取文件、写入文件、处理CSV文件、处理JSON文件、处理Excel文件、文件路径和目录操作、文件异常处理、处理二进制文件、文件对象方法、处理大文件等方面。掌握这些方法,可以使你在处理文件时更加得心应手,提高工作效率。Python内置的文件操作功能非常强大,再结合外部库,可以满足几乎所有的文件处理需求。
相关问答FAQs:
如何在Python中读取外部文件?
在Python中,可以使用内置的open()
函数来读取外部文件。通过指定文件的路径和模式(如只读模式'r'),可以成功打开文件。读取内容可以使用read()
, readline()
, 或 readlines()
方法,这些方法将文件内容以字符串或列表的形式返回。例如,使用with open('filename.txt', 'r') as file:
可以保证文件在使用后自动关闭。
Python支持哪些文件格式的读取?
Python支持多种文件格式的读取,包括文本文件(如.txt, .csv)、二进制文件(如.jpg, .png)以及JSON、XML等结构化数据格式。对于每种文件类型,通常会有相应的库或模块来处理。例如,pandas
库可用于读取CSV文件,而json
模块则用于处理JSON格式的数据。
如何在Python中写入外部文件?
在Python中,可以使用open()
函数结合写入模式'w'或'a'来创建或修改外部文件。使用'w'模式会覆盖原有内容,而'a'模式则会在文件末尾添加新内容。写入内容可以使用write()
或writelines()
方法。例如,使用with open('output.txt', 'w') as file:
来写入文本,确保文件在操作结束后被关闭。
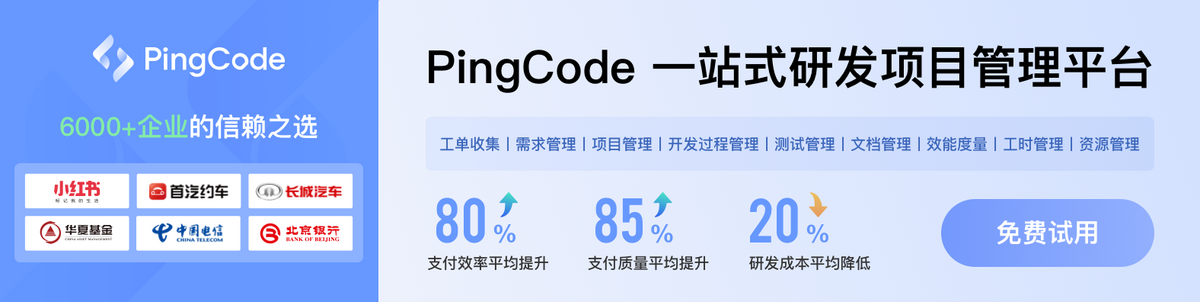