在Python中,添加回车换行主要有以下几种方法:使用\n
换行符、使用三引号字符串、使用print()
函数中的end
参数。其中,最常用的是使用\n
换行符。\n
是一个特殊字符,用于表示换行。例如:
print("Hello\nWorld")
这段代码将输出:
Hello
World
接下来我们将详细讨论每种方法的具体用法及其应用场景。
一、使用\n
换行符
基本用法
在Python字符串中,\n
表示换行符。当你在字符串中加入\n
时,Python会在输出时将其转换为一个换行操作。
text = "This is the first line.\nThis is the second line."
print(text)
输出结果为:
This is the first line.
This is the second line.
应用场景
- 多行文本输出:当你需要在输出中显示多行文本时,
\n
非常有用。 - 生成带有格式的输出:在生成报告、日志文件或其他带有格式的文本时,
\n
可以帮助你创建清晰的结构。
二、使用三引号字符串
基本用法
Python 支持使用三引号(单引号或双引号)来定义多行字符串。在这种情况下,你可以直接在字符串中换行,而不需要显式地使用\n
。
text = """This is the first line.
This is the second line."""
print(text)
输出结果为:
This is the first line.
This is the second line.
应用场景
- 定义多行字符串:当你需要定义一个包含多行文本的字符串时,三引号字符串非常方便。
- 保持文本格式:当你希望保持文本的原始格式(例如在文档字符串中),使用三引号字符串可以帮助你做到这一点。
三、使用print()
函数中的end
参数
基本用法
print()
函数的end
参数用于指定输出结束时的字符。默认情况下,end
参数的值是\n
,这意味着每次调用print()
函数时,输出都会以换行结束。但你可以将其修改为其他值。
print("This is the first line.", end="\n")
print("This is the second line.")
输出结果为:
This is the first line.
This is the second line.
你还可以将end
参数设置为其他字符或字符串,例如空格或逗号。
print("This is the first line.", end=" ")
print("This is the second line.")
输出结果为:
This is the first line. This is the second line.
应用场景
- 控制输出格式:当你需要在输出中添加特定的字符或字符串时,修改
end
参数可以帮助你做到这一点。 - 防止自动换行:当你不希望在每次
print()
调用后自动换行时,可以将end
参数设置为空字符串(""
)。
四、使用join()
方法
基本用法
join()
方法用于将一个可迭代对象(例如列表或元组)中的元素连接成一个字符串。你可以使用\n
作为分隔符来实现多行输出。
lines = ["This is the first line.", "This is the second line."]
text = "\n".join(lines)
print(text)
输出结果为:
This is the first line.
This is the second line.
应用场景
- 连接多行文本:当你有一个包含多行文本的列表或其他可迭代对象时,
join()
方法可以帮助你将它们连接成一个字符串。 - 动态生成多行字符串:当你需要根据某些条件动态生成多行字符串时,
join()
方法非常有用。
五、使用字符串格式化
基本用法
Python 提供了多种字符串格式化方法,例如f-string
、str.format()
和百分号格式化。你可以使用这些方法来创建带有换行符的字符串。
line1 = "This is the first line."
line2 = "This is the second line."
text = f"{line1}\n{line2}"
print(text)
输出结果为:
This is the first line.
This is the second line.
应用场景
- 动态生成带有格式的字符串:当你需要根据变量的值生成带有换行符的字符串时,字符串格式化方法非常有用。
- 提高代码可读性:使用字符串格式化方法可以使代码更加清晰和易读。
六、使用os.linesep
基本用法
os.linesep
是一个字符串,表示当前操作系统的换行符。在大多数操作系统中,os.linesep
的值是\n
,但在某些操作系统中可能是其他值(例如在 Windows 中,os.linesep
的值是\r\n
)。
import os
text = f"This is the first line.{os.linesep}This is the second line."
print(text)
输出结果为:
This is the first line.
This is the second line.
应用场景
- 跨平台开发:当你编写需要在多个操作系统上运行的代码时,使用
os.linesep
可以确保你的代码在不同操作系统上都能正确处理换行符。 - 提高代码的可移植性:使用
os.linesep
可以使你的代码更加通用和可移植。
七、读取和写入文件时添加换行符
基本用法
在读取和写入文件时,你可以使用换行符来控制文件内容的格式。例如:
with open("example.txt", "w") as file:
file.write("This is the first line.\n")
file.write("This is the second line.\n")
然后,读取文件内容时:
with open("example.txt", "r") as file:
content = file.read()
print(content)
输出结果为:
This is the first line.
This is the second line.
应用场景
- 生成多行文本文件:当你需要创建包含多行文本的文件时,可以在写入文件时使用换行符。
- 读取带有格式的文件:当你读取包含多行文本的文件时,可以使用换行符来解析文件内容。
八、使用正则表达式处理换行符
基本用法
正则表达式可以用于匹配和处理包含换行符的字符串。例如:
import re
text = "This is the first line.\nThis is the second line."
pattern = re.compile(r"\n")
result = pattern.split(text)
print(result)
输出结果为:
['This is the first line.', 'This is the second line.']
应用场景
- 解析多行文本:当你需要解析包含多行文本的字符串时,正则表达式可以帮助你匹配和处理换行符。
- 文本处理和转换:在文本处理和转换任务中,正则表达式可以用于匹配和替换换行符。
九、总结
在Python中,添加回车换行的方法有很多种,每种方法都有其独特的应用场景和优势。无论是使用\n
换行符、三引号字符串、print()
函数的end
参数,还是使用join()
方法、字符串格式化、os.linesep
、文件操作和正则表达式,都可以根据具体需求选择合适的方法。通过掌握这些方法,你可以更加灵活地处理和生成多行文本,提高代码的可读性和可维护性。
相关问答FAQs:
如何在Python中使用换行符?
在Python中,换行符通常使用 \n
表示。你可以在字符串中直接插入 \n
,这样在打印该字符串时,输出会自动换行。例如:
print("Hello\nWorld")
输出将会是:
Hello
World
在多行字符串中如何添加换行?
使用三重引号('''
或 """
)可以轻松创建多行字符串。在这种情况下,换行会自动被包含在输出中。例如:
multiline_string = """这是一行
这是另一行"""
print(multiline_string)
输出将会是:
这是一行
这是另一行
如何在文件中写入换行符?
在Python中,写入文件时可以使用换行符 \n
来控制内容的换行。例如:
with open('example.txt', 'w') as file:
file.write("第一行\n第二行\n第三行")
这段代码会在 example.txt
文件中写入三行内容,每行之间用换行符分隔。
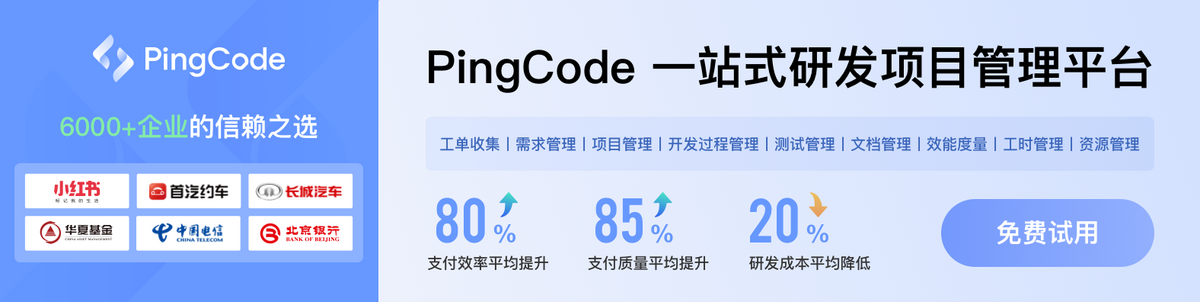