Python打开高效文件的方法包括:使用内置的open
函数、使用上下文管理器、使用缓冲区、以及使用第三方库。 其中,使用上下文管理器 是一种非常有效的方法,可以确保文件在操作完成后自动关闭,避免资源泄露。
使用上下文管理器的一个例子是with open
语句。with open
语句不仅可以简化代码,还能提高代码的可读性和安全性。例如:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
在这段代码中,with open
语句确保了文件会在离开with
代码块时自动关闭,避免了手动调用file.close()
的麻烦,从而减少了错误的可能性。
一、使用内置的open
函数
Python的open
函数是最常用的文件操作方式之一。它支持多种文件模式,如读取(r
)、写入(w
)、追加(a
)等。
file = open('example.txt', 'r')
content = file.read()
print(content)
file.close()
这种方式虽然简单直接,但需要手动关闭文件。如果忘记关闭文件,可能会导致资源泄露。因此,推荐使用上下文管理器。
二、使用上下文管理器
如前所述,使用上下文管理器可以自动管理文件的打开和关闭。以下是一些上下文管理器的例子:
1. 读取文件
with open('example.txt', 'r') as file:
content = file.read()
print(content)
2. 写入文件
with open('example.txt', 'w') as file:
file.write('Hello, World!')
3. 追加文件
with open('example.txt', 'a') as file:
file.write('Append this text.')
三、使用缓冲区
在处理大文件时,使用缓冲区可以显著提高文件操作的性能。open
函数的buffering
参数可以设置缓冲区大小。
1. 读取文件时使用缓冲区
with open('example.txt', 'r', buffering=4096) as file:
content = file.read()
print(content)
2. 写入文件时使用缓冲区
with open('example.txt', 'w', buffering=4096) as file:
file.write('Hello, World!')
四、使用第三方库
一些第三方库如pandas
和numpy
可以更加高效地处理文件操作,尤其是对于大型数据集。
1. 使用pandas
读取CSV文件
import pandas as pd
df = pd.read_csv('example.csv')
print(df.head())
2. 使用numpy
读取文本文件
import numpy as np
data = np.loadtxt('example.txt')
print(data)
五、处理大文件
处理大文件时,逐行读取可以避免内存耗尽。
1. 使用for
循环逐行读取
with open('example.txt', 'r') as file:
for line in file:
print(line.strip())
2. 使用readline
方法逐行读取
with open('example.txt', 'r') as file:
while True:
line = file.readline()
if not line:
break
print(line.strip())
六、处理二进制文件
Python的open
函数也支持二进制模式,可以用来处理图像、音频等文件。
1. 读取二进制文件
with open('example.bin', 'rb') as file:
content = file.read()
print(content)
2. 写入二进制文件
with open('example.bin', 'wb') as file:
file.write(b'Hello, World!')
七、多线程和多进程
在某些情况下,使用多线程和多进程可以提高文件操作的效率。
1. 使用多线程
import threading
def read_file(file_name):
with open(file_name, 'r') as file:
content = file.read()
print(content)
thread = threading.Thread(target=read_file, args=('example.txt',))
thread.start()
thread.join()
2. 使用多进程
import multiprocessing
def read_file(file_name):
with open(file_name, 'r') as file:
content = file.read()
print(content)
process = multiprocessing.Process(target=read_file, args=('example.txt',))
process.start()
process.join()
八、总结
通过合理使用open
函数、上下文管理器、缓冲区、第三方库、以及多线程和多进程技术,可以显著提高Python文件操作的效率和安全性。在实际应用中,根据具体需求选择合适的方法,才能更好地处理文件操作。
相关问答FAQs:
如何在Python中打开大文件而不占用过多内存?
当处理大文件时,使用Python的with open()
语句可以有效管理文件的打开和关闭。通过逐行读取文件(例如使用for line in file:
)而不是一次性加载整个文件,可以显著降低内存使用。还可以使用pandas
库中的read_csv()
方法,结合chunksize
参数,来分块读取大型CSV文件。
在Python中使用什么库可以更高效地处理文件?
针对不同类型的文件,Python提供了多种库。对于文本文件,io
模块提供了高效的输入输出操作。处理CSV文件时,pandas
库以其高效的处理能力广受欢迎。对于Excel文件,可以使用openpyxl
或xlrd
库。如果是二进制文件,struct
模块可以帮助解析数据结构。
如何在Python中读取压缩文件的内容?
Python的gzip
和zipfile
模块可以直接读取压缩文件的内容。使用这些模块,可以在不解压缩文件的情况下,直接处理数据。例如,使用gzip.open()
可以打开.gz
文件并逐行读取内容,而使用zipfile.ZipFile
可以访问压缩包内的文件并进行操作。这样可以节省存储空间和加载时间。
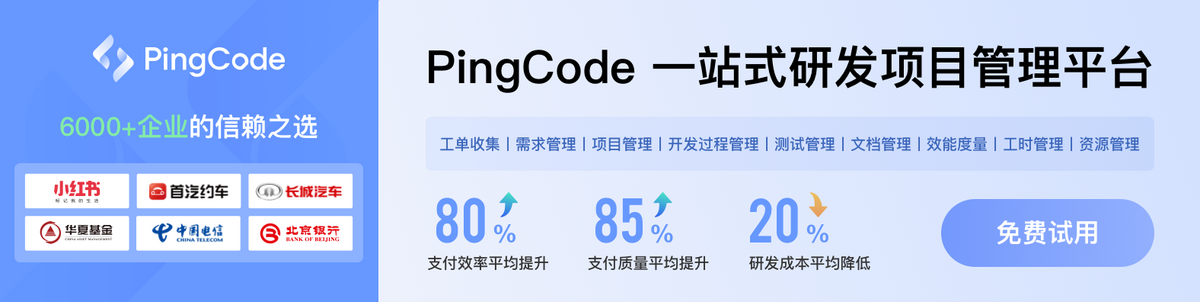