用python禁止软件安装的方法包括:监控系统进程、修改系统注册表、使用第三方库与工具、创建白名单和黑名单。 其中,监控系统进程 是一种较为直接和常见的方法,通过监控系统中正在运行的进程,及时发现并阻止安装程序的运行,从而达到禁止软件安装的目的。
监控系统进程的方法可以通过调用Python的系统管理相关库来实现,比如psutil
库。psutil
库可以帮助我们方便地获取系统的进程信息,从而进行实时监控。当发现有安装程序运行时,立即终止该进程。下面我们将详细介绍如何使用psutil
库来实现这个功能。
一、监控系统进程
1、安装psutil库
psutil
是一个跨平台库,能够轻松获取系统进程和系统利用率(CPU、内存、磁盘、网络、传感器)的信息。首先需要安装该库,可以使用pip命令进行安装:
pip install psutil
2、编写监控脚本
编写一个简单的脚本来监控系统进程,并根据进程名称或其他特征来判断是否为安装程序。一旦发现安装程序,则终止该进程。以下是一个示例脚本:
import psutil
import time
安装程序的关键字列表,可以根据实际情况调整
installation_keywords = ["setup", "install", "installer", "msiexec"]
def is_installation_process(process_name):
"""判断进程是否为安装程序"""
for keyword in installation_keywords:
if keyword in process_name.lower():
return True
return False
def monitor_processes():
"""监控系统进程并终止安装程序"""
while True:
# 获取所有进程
for process in psutil.process_iter(['pid', 'name']):
try:
process_name = process.info['name']
if is_installation_process(process_name):
print(f"Found installation process: {process_name}, terminating it...")
psutil.Process(process.info['pid']).terminate()
except (psutil.NoSuchProcess, psutil.AccessDenied, psutil.ZombieProcess):
pass
# 每隔5秒钟检查一次
time.sleep(5)
if __name__ == "__main__":
monitor_processes()
上述脚本首先定义了一些安装程序的关键字,然后通过遍历系统中的所有进程,检查进程名称是否包含这些关键字。如果发现符合条件的安装程序,则立即终止该进程。
二、修改系统注册表
在Windows系统中,可以通过修改注册表来禁止软件安装。具体方法包括禁用Windows Installer服务、限制用户权限等。可以使用Python的winreg
库来操作注册表。
1、禁用Windows Installer服务
import winreg
def disable_windows_installer():
"""禁用Windows Installer服务"""
try:
key = winreg.OpenKey(winreg.HKEY_LOCAL_MACHINE, r"SYSTEM\CurrentControlSet\Services\MSIServer", 0, winreg.KEY_SET_VALUE)
winreg.SetValueEx(key, "Start", 0, winreg.REG_DWORD, 4)
winreg.CloseKey(key)
print("Windows Installer service has been disabled.")
except Exception as e:
print(f"Failed to disable Windows Installer service: {e}")
if __name__ == "__main__":
disable_windows_installer()
上述脚本通过修改注册表中的MSIServer
服务的启动类型,将其设置为4(禁用)。这样可以有效地阻止使用Windows Installer进行的软件安装。
2、限制用户权限
可以通过修改注册表来限制普通用户对安装程序的执行权限,从而禁止软件安装。具体方法是修改注册表中的Policies
项。
import winreg
def restrict_user_permissions():
"""限制普通用户对安装程序的执行权限"""
try:
key = winreg.OpenKey(winreg.HKEY_LOCAL_MACHINE, r"SOFTWARE\Policies\Microsoft\Windows\Installer", 0, winreg.KEY_SET_VALUE)
winreg.SetValueEx(key, "DisableUserInstalls", 0, winreg.REG_DWORD, 1)
winreg.CloseKey(key)
print("User permissions to install software have been restricted.")
except Exception as e:
print(f"Failed to restrict user permissions: {e}")
if __name__ == "__main__":
restrict_user_permissions()
上述脚本通过修改注册表中的DisableUserInstalls
项,将其设置为1(禁用)。这样普通用户将无法运行安装程序。
三、使用第三方库与工具
除了psutil
和winreg
,还有一些第三方库和工具可以帮助实现禁止软件安装的功能。例如,可以使用pywin32
库来调用Windows API,进行更复杂的系统管理操作。
1、安装pywin32库
可以使用pip命令安装pywin32
库:
pip install pywin32
2、使用pywin32库调用Windows API
以下是一个示例脚本,使用pywin32
库来禁用Windows Installer服务:
import win32serviceutil
def disable_windows_installer_service():
"""禁用Windows Installer服务"""
try:
win32serviceutil.ChangeServiceConfig(
'MSIServer',
startType=win32serviceutil.SERVICE_DISABLED
)
print("Windows Installer service has been disabled.")
except Exception as e:
print(f"Failed to disable Windows Installer service: {e}")
if __name__ == "__main__":
disable_windows_installer_service()
四、创建白名单和黑名单
可以通过创建白名单和黑名单来控制哪些程序可以安装,哪些程序不可以安装。具体方法是维护一个包含允许安装的程序和禁止安装的程序的列表,根据列表进行控制。
1、定义白名单和黑名单
whitelist = ["allowed_installer.exe"]
blacklist = ["forbidden_installer.exe"]
2、编写监控脚本
import psutil
import time
def is_in_blacklist(process_name):
"""判断进程是否在黑名单中"""
for forbidden in blacklist:
if forbidden in process_name:
return True
return False
def is_in_whitelist(process_name):
"""判断进程是否在白名单中"""
for allowed in whitelist:
if allowed in process_name:
return True
return False
def monitor_processes():
"""监控系统进程,根据白名单和黑名单进行控制"""
while True:
# 获取所有进程
for process in psutil.process_iter(['pid', 'name']):
try:
process_name = process.info['name']
if is_in_blacklist(process_name) and not is_in_whitelist(process_name):
print(f"Found forbidden installation process: {process_name}, terminating it...")
psutil.Process(process.info['pid']).terminate()
except (psutil.NoSuchProcess, psutil.AccessDenied, psutil.ZombieProcess):
pass
# 每隔5秒钟检查一次
time.sleep(5)
if __name__ == "__main__":
monitor_processes()
上述脚本通过判断进程名称是否在黑名单中,并且不在白名单中,一旦发现符合条件的安装程序,则立即终止该进程。
总结
通过监控系统进程、修改系统注册表、使用第三方库与工具、创建白名单和黑名单等方法,可以有效地禁止软件安装。每种方法都有其优缺点,可以根据实际需求选择合适的方法。同时,需要注意的是,禁止软件安装可能会对系统正常使用造成一定影响,因此在实施这些方法时需要谨慎。
相关问答FAQs:
1. 如何使用Python编写脚本来限制软件安装?
要使用Python编写脚本以限制软件安装,您可以利用系统权限和监控工具。通过检测常见的安装程序,如.exe
或.msi
文件,并在其运行时终止进程或给出警告信息,从而阻止软件的安装。此外,结合操作系统的用户权限设置,您可以确保只有特定用户有权进行软件安装。
2. 在Windows系统中,使用Python如何监控和阻止安装程序的执行?
在Windows系统中,可以使用Python的psutil
库监控当前运行的进程。通过监听安装程序的进程名称,您可以编写代码在检测到特定进程时,自动结束该进程。结合Windows的事件日志,您还可以记录这些活动,以便进行审计和分析。
3. Python是否可以与组策略结合使用,以防止软件安装?
虽然Python本身无法直接修改组策略,但可以通过调用Windows管理工具(如gpedit.msc
)和相应的命令行工具,利用Python脚本执行相关的组策略设置。这样可以为用户创建更严格的安装限制,从而有效地控制软件的安装行为。
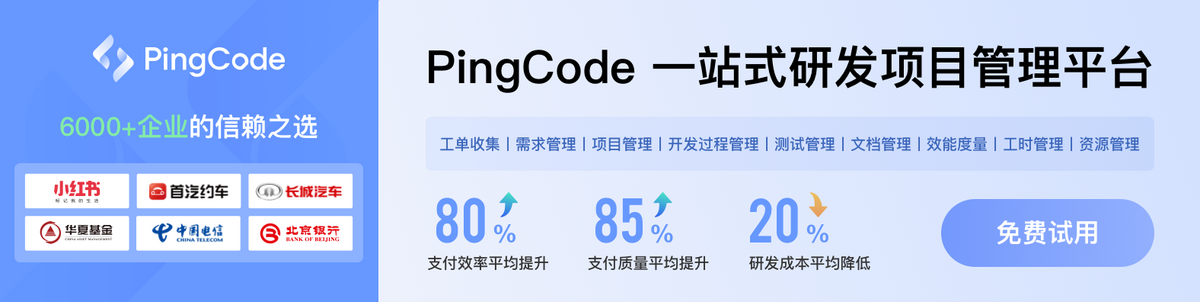