将Python变成脚本的方法有多种:将Python代码保存为.py文件、使用shebang声明、赋予执行权限。 其中,将Python代码保存为.py文件是最基础和必要的一步。通过这种方式,我们可以直接在终端或命令行中运行Python脚本。
一、将Python代码保存为.py文件
-
创建并保存.py文件
- 打开你喜欢的文本编辑器,如VS Code、Sublime Text或Notepad++。
- 编写你的Python代码。
- 保存文件,确保文件扩展名为.py。例如,保存为
script.py
。
-
运行.py文件
- 打开终端或命令行。
- 导航到文件所在目录。
- 运行
python script.py
或python3 script.py
。
二、使用shebang声明
-
添加shebang行
在你的Python脚本的第一行添加shebang声明:
#!/usr/bin/env python3
-
赋予执行权限
- 在终端中导航到文件所在目录。
- 使用
chmod
命令赋予执行权限:
chmod +x script.py
-
直接运行脚本
- 在终端中运行脚本:
./script.py
三、赋予执行权限
-
在终端中赋予执行权限
- 在终端中导航到文件所在目录。
- 使用
chmod
命令赋予执行权限:
chmod +x script.py
-
运行脚本
- 在终端中直接运行脚本:
./script.py
四、脚本部署和管理
-
使用虚拟环境
- 创建和激活虚拟环境:
python3 -m venv myenv
source myenv/bin/activate
- 在虚拟环境中运行你的脚本。
-
使用环境变量
- 设置环境变量以确保脚本运行所需的环境:
export MY_VARIABLE=value
-
调度任务
- 使用cron或其他调度工具来定时运行Python脚本。例如,使用cron:
crontab -e
添加一行:
0 * * * * /path/to/your/script.py
五、脚本日志记录与调试
-
日志记录
- 使用Python的
logging
模块来记录脚本的运行情况:
import logging
logging.basicConfig(filename='script.log', level=logging.INFO)
logging.info('This is an info message')
- 使用Python的
-
调试
- 使用
pdb
模块来调试Python脚本:
import pdb
pdb.set_trace()
- 通过在脚本中插入
pdb.set_trace()
来设置断点。
- 使用
六、脚本优化和性能提升
-
代码优化
- 避免使用不必要的循环和条件判断。
- 使用高效的数据结构,如字典和集合。
-
性能分析
- 使用
cProfile
模块来分析脚本性能:
python -m cProfile script.py
- 使用
-
多线程和多进程
- 使用
threading
模块来实现多线程:
import threading
def worker():
print("Worker thread")
thread = threading.Thread(target=worker)
thread.start()
- 使用
multiprocessing
模块来实现多进程:
import multiprocessing
def worker():
print("Worker process")
process = multiprocessing.Process(target=worker)
process.start()
- 使用
七、脚本打包与分发
-
使用
pyinstaller
打包- 安装
pyinstaller
:
pip install pyinstaller
- 打包脚本:
pyinstaller --onefile script.py
- 安装
-
使用
setuptools
分发- 创建
setup.py
文件:
from setuptools import setup
setup(
name='script',
version='0.1',
py_modules=['script'],
entry_points={
'console_scripts': [
'script=script:main',
],
},
)
- 安装脚本:
pip install .
- 创建
八、脚本安全与维护
-
安全性
- 避免在脚本中硬编码敏感信息,如密码和API密钥。
- 使用环境变量来管理敏感信息:
import os
api_key = os.getenv('API_KEY')
-
维护
- 定期更新和维护脚本,确保与最新的库和依赖项兼容。
- 编写单元测试来确保脚本的稳定性和正确性:
import unittest
class TestScript(unittest.TestCase):
def test_example(self):
self.assertEqual(1, 1)
if __name__ == '__main__':
unittest.main()
九、脚本国际化与本地化
-
国际化
- 使用
gettext
模块来实现脚本的国际化:
import gettext
gettext.bindtextdomain('myapp', 'locale')
gettext.textdomain('myapp')
_ = gettext.gettext
print(_('Hello, world!'))
- 使用
-
本地化
- 创建
locale
目录结构:
mkdir -p locale/en/LC_MESSAGES
mkdir -p locale/es/LC_MESSAGES
- 创建和编译翻译文件:
msginit --locale=en --input=myapp.pot --output=locale/en/LC_MESSAGES/myapp.po
msginit --locale=es --input=myapp.pot --output=locale/es/LC_MESSAGES/myapp.po
msgfmt locale/en/LC_MESSAGES/myapp.po -o locale/en/LC_MESSAGES/myapp.mo
msgfmt locale/es/LC_MESSAGES/myapp.po -o locale/es/LC_MESSAGES/myapp.mo
- 创建
十、脚本兼容性与跨平台运行
-
兼容性
- 确保脚本兼容Python 2和Python 3:
from __future__ import print_function
-
跨平台运行
- 避免使用特定于操作系统的路径和命令。
- 使用
os
模块来处理路径和文件操作:
import os
path = os.path.join('directory', 'file.txt')
十一、脚本使用文档与帮助信息
-
使用文档
- 编写脚本使用文档,详细说明脚本的功能、参数和使用方法。
- 使用Markdown格式编写文档,以便在GitHub等平台上展示。
-
帮助信息
- 在脚本中添加命令行参数解析和帮助信息:
import argparse
parser = argparse.ArgumentParser(description='Script description')
parser.add_argument('--example', type=str, help='Example argument')
args = parser.parse_args()
print(args.example)
十二、脚本版本控制与协作
-
版本控制
- 使用Git来管理脚本的版本控制。
- 定期提交代码变更,并写明详细的提交信息:
git init
git add script.py
git commit -m "Initial commit"
-
协作开发
- 使用GitHub等平台来协作开发和管理脚本。
- 创建和管理Pull Request,以便代码审查和合并:
git branch feature_branch
git checkout feature_branch
git push origin feature_branch
十三、脚本测试与持续集成
-
单元测试
- 编写单元测试来确保脚本的稳定性和正确性:
import unittest
class TestScript(unittest.TestCase):
def test_example(self):
self.assertEqual(1, 1)
if __name__ == '__main__':
unittest.main()
-
持续集成
- 使用CI工具,如Travis CI或GitHub Actions,来自动化脚本的测试和部署。
- 创建CI配置文件:
# .travis.yml
language: python
python:
- "3.8"
install:
- pip install -r requirements.txt
script:
- python -m unittest discover
十四、脚本的扩展与模块化
-
模块化
- 将脚本拆分为多个模块,以提高代码的可读性和可维护性。
- 创建并导入模块:
# module.py
def example_function():
return "Hello, world!"
script.py
from module import example_function
print(example_function())
-
扩展
- 使用第三方库和工具来扩展脚本的功能。
- 安装并导入第三方库:
pip install requests
import requests
response = requests.get('https://api.example.com')
print(response.json())
十五、脚本的容器化与部署
-
容器化
- 使用Docker来容器化你的Python脚本。
- 创建Dockerfile:
FROM python:3.8-slim
COPY script.py /app/script.py
WORKDIR /app
RUN pip install -r requirements.txt
CMD ["python", "script.py"]
-
部署
- 使用Docker Compose来管理多容器应用:
version: '3.8'
services:
app:
build: .
volumes:
- .:/app
command: python script.py
- 使用Kubernetes来部署和管理容器化的Python脚本。
十六、脚本的优化与性能提升
-
代码优化
- 避免使用不必要的循环和条件判断。
- 使用高效的数据结构,如字典和集合。
-
性能分析
- 使用
cProfile
模块来分析脚本性能:
python -m cProfile script.py
- 使用
-
多线程和多进程
- 使用
threading
模块来实现多线程:
import threading
def worker():
print("Worker thread")
thread = threading.Thread(target=worker)
thread.start()
- 使用
multiprocessing
模块来实现多进程:
import multiprocessing
def worker():
print("Worker process")
process = multiprocessing.Process(target=worker)
process.start()
- 使用
通过以上各个方面的详细介绍,希望能帮助你更好地理解和掌握将Python变成脚本的方法和技巧。无论是从基本的脚本创建与运行,还是到高级的优化与部署,都涵盖了Python脚本开发中的方方面面。希望这些内容对你的Python脚本开发工作有所帮助。
相关问答FAQs:
如何将Python脚本转换为可执行文件?
将Python脚本转换为可执行文件可以使用工具如PyInstaller或cx_Freeze。这些工具可以将Python代码打包成独立的可执行文件,用户无需安装Python环境。只需在命令行中运行相关命令即可完成打包,生成的文件可以在目标操作系统上直接运行。
Python脚本可以在哪些操作系统上运行?
Python脚本具有跨平台的特性,可以在Windows、macOS和Linux等多个操作系统上运行。只需确保相应系统上安装了Python解释器即可。此外,使用PyInstaller等工具打包后,生成的可执行文件也能在所选择的操作系统上运行。
如何在Python脚本中处理命令行参数?
可以使用argparse
模块来处理命令行参数。通过定义参数和选项,能够让用户在运行脚本时输入自定义的参数,从而改变程序的行为。这种方式使得脚本更加灵活和用户友好,能够满足不同用户的需求。
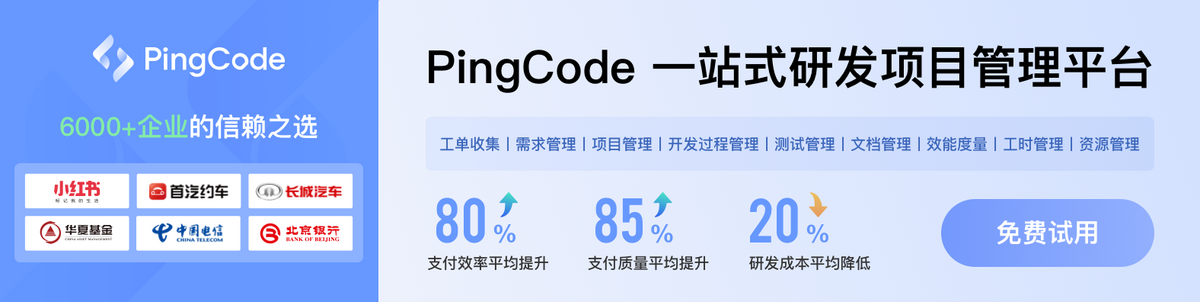