Python接口用例的运行可以通过编写接口用例、使用测试框架、发送HTTP请求、进行断言校验、生成测试报告来实现。以下详细描述如何编写和运行Python接口用例:
一、编写接口用例
编写接口用例是运行接口测试的第一步。接口用例主要包括接口的URL、请求方法、请求参数、请求头、预期结果等。在Python中,我们可以使用requests
库来发送HTTP请求,使用unittest
或pytest
作为测试框架。
1.1 使用requests
库发送HTTP请求
requests
库是Python中非常流行的HTTP请求库,能够方便地发送GET、POST、PUT、DELETE等请求。
import requests
response = requests.get("https://api.example.com/data")
print(response.status_code)
print(response.json())
在实际接口用例中,我们会根据接口的具体要求来设置请求方法、请求参数和请求头。
二、使用测试框架
Python有许多测试框架,如unittest
、pytest
等。使用测试框架可以组织和管理测试用例,并生成测试报告。
2.1 使用unittest
框架
unittest
是Python自带的测试框架,使用起来非常方便。
import unittest
import requests
class TestAPI(unittest.TestCase):
def test_get_data(self):
response = requests.get("https://api.example.com/data")
self.assertEqual(response.status_code, 200)
self.assertIn("data", response.json())
if __name__ == "__main__":
unittest.main()
2.2 使用pytest
框架
pytest
是一个更强大、更灵活的测试框架,可以替代unittest
,并且支持更多的功能,如参数化、插件等。
import requests
def test_get_data():
response = requests.get("https://api.example.com/data")
assert response.status_code == 200
assert "data" in response.json()
三、发送HTTP请求
接口测试的核心是发送HTTP请求,并对响应结果进行校验。通过requests
库,我们可以方便地发送各种类型的HTTP请求。
3.1 发送GET请求
response = requests.get("https://api.example.com/data", params={"key": "value"})
print(response.status_code)
print(response.json())
3.2 发送POST请求
response = requests.post("https://api.example.com/data", json={"key": "value"})
print(response.status_code)
print(response.json())
四、进行断言校验
断言是测试用例的重要组成部分,通过断言来验证接口的响应结果是否符合预期。
4.1 状态码断言
验证接口返回的状态码是否为预期值。
self.assertEqual(response.status_code, 200)
4.2 响应内容断言
验证接口返回的响应内容是否符合预期。
self.assertIn("data", response.json())
五、生成测试报告
测试报告可以帮助我们了解测试的执行情况和结果。在unittest
中,可以使用HTMLTestRunner
生成HTML格式的测试报告;在pytest
中,可以使用pytest-html
插件生成HTML测试报告。
5.1 使用HTMLTestRunner
生成测试报告
import unittest
from HTMLTestRunner import HTMLTestRunner
if __name__ == "__main__":
suite = unittest.TestLoader().loadTestsFromTestCase(TestAPI)
with open("report.html", "wb") as f:
runner = HTMLTestRunner(stream=f, title="API Test Report")
runner.run(suite)
5.2 使用pytest-html
生成测试报告
pip install pytest-html
pytest --html=report.html
通过以上步骤,我们可以编写和运行Python接口用例,并生成测试报告。下面将进一步详细介绍每个步骤的具体操作和注意事项。
一、编写接口用例
1.1 确定测试需求
在编写接口用例之前,需要明确测试需求,了解被测试的接口的功能、输入输出参数、预期结果等。可以通过查看接口文档、与开发人员沟通等方式获取这些信息。
1.2 设计测试用例
根据测试需求,设计具体的测试用例。测试用例应覆盖接口的正常情况、异常情况、边界情况等。可以使用Excel、Markdown等工具编写测试用例文档。
| 用例编号 | 用例描述 | 请求方法 | 请求URL | 请求参数 | 预期结果 |
| -------- | -------------- | -------- | ---------------- | -------------- | -------------- |
| TC001 | 获取数据 | GET | /data | {key: value} | 状态码200,响应包含data字段 |
| TC002 | 提交数据 | POST | /data | {key: value} | 状态码201,响应包含success字段 |
1.3 编写测试脚本
根据设计的测试用例,编写测试脚本。在Python中,可以使用requests
库发送HTTP请求,使用unittest
或pytest
编写测试脚本。
import requests
import unittest
class TestAPI(unittest.TestCase):
def test_get_data(self):
response = requests.get("https://api.example.com/data", params={"key": "value"})
self.assertEqual(response.status_code, 200)
self.assertIn("data", response.json())
def test_post_data(self):
response = requests.post("https://api.example.com/data", json={"key": "value"})
self.assertEqual(response.status_code, 201)
self.assertIn("success", response.json())
if __name__ == "__main__":
unittest.main()
二、使用测试框架
2.1 使用unittest
框架
unittest
是Python内置的测试框架,使用非常简单。可以通过创建unittest.TestCase
的子类来编写测试用例,并使用unittest.main()
来运行测试。
import unittest
class TestExample(unittest.TestCase):
def test_add(self):
self.assertEqual(1 + 1, 2)
if __name__ == "__main__":
unittest.main()
2.2 使用pytest
框架
pytest
是一个功能强大的测试框架,支持参数化、fixture、插件等功能。可以通过编写函数形式的测试用例来使用pytest
。
def test_add():
assert 1 + 1 == 2
可以使用命令行运行pytest
,并生成测试报告。
pytest --html=report.html
三、发送HTTP请求
3.1 发送GET请求
GET请求用于从服务器获取数据。可以通过设置请求URL和请求参数来发送GET请求。
response = requests.get("https://api.example.com/data", params={"key": "value"})
print(response.status_code)
print(response.json())
3.2 发送POST请求
POST请求用于向服务器提交数据。可以通过设置请求URL、请求头和请求体来发送POST请求。
response = requests.post("https://api.example.com/data", json={"key": "value"})
print(response.status_code)
print(response.json())
四、进行断言校验
断言是测试用例的重要组成部分,用于验证接口的响应结果是否符合预期。
4.1 状态码断言
状态码断言用于验证接口返回的状态码是否为预期值。
self.assertEqual(response.status_code, 200)
4.2 响应内容断言
响应内容断言用于验证接口返回的响应内容是否符合预期。
self.assertIn("data", response.json())
五、生成测试报告
测试报告可以帮助我们了解测试的执行情况和结果。在unittest
中,可以使用HTMLTestRunner
生成HTML格式的测试报告;在pytest
中,可以使用pytest-html
插件生成HTML测试报告。
5.1 使用HTMLTestRunner
生成测试报告
HTMLTestRunner
是一个可以生成HTML格式测试报告的库,可以通过pip
安装。
pip install html-testRunner
在测试脚本中使用HTMLTestRunner
生成测试报告。
import unittest
from HTMLTestRunner import HTMLTestRunner
if __name__ == "__main__":
suite = unittest.TestLoader().loadTestsFromTestCase(TestAPI)
with open("report.html", "wb") as f:
runner = HTMLTestRunner(stream=f, title="API Test Report")
runner.run(suite)
5.2 使用pytest-html
生成测试报告
pytest-html
是pytest
的一个插件,可以生成HTML格式的测试报告。可以通过pip
安装。
pip install pytest-html
使用命令行运行pytest
,并生成HTML格式的测试报告。
pytest --html=report.html
通过以上步骤,我们可以编写和运行Python接口用例,并生成测试报告。总结起来,主要包括编写接口用例、使用测试框架、发送HTTP请求、进行断言校验、生成测试报告等步骤。在实际操作中,还需要根据项目具体需求进行调整和优化。
相关问答FAQs:
如何准备运行Python接口用例的环境?
在运行Python接口用例之前,需要确保你的开发环境已经设置好。首先,安装Python及其相关库,如requests
和unittest
等,通常可以通过pip install
命令完成。此外,确保你有接口的文档,了解接口的请求类型、参数及返回格式,这些信息将帮助你构建测试用例。
在运行Python接口用例时,如何处理请求的验证和授权?
对于需要身份验证的接口,通常需要在请求中添加相应的头部信息或参数。例如,使用Bearer Token或API Key进行身份验证。在编写用例时,务必要处理这些验证信息,以确保测试的准确性和有效性。
如何查看Python接口用例的运行结果和日志信息?
在执行接口用例后,通常会有日志输出,帮助开发者了解测试的结果。可以通过设置日志级别来查看详细信息,或者使用unittest
框架的assert
方法来捕捉和输出错误信息。此外,许多测试框架允许生成报告,便于后续分析测试结果。
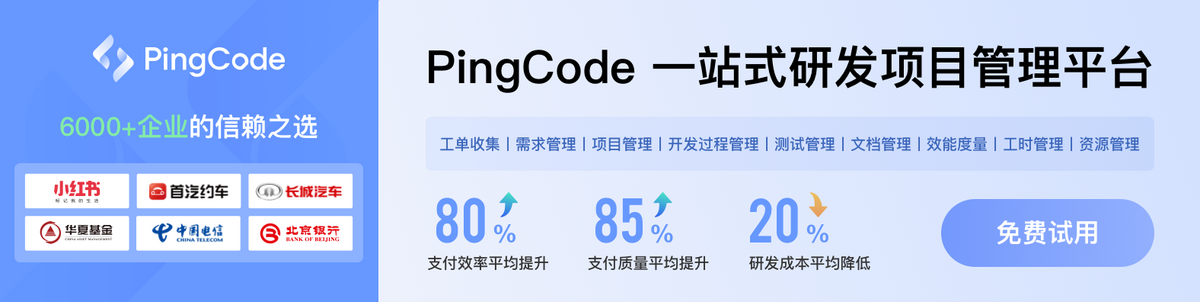