利用Python制作词云需要以下步骤:安装必要的库、准备文本数据、生成词云图像、可视化词云。 其中,安装必要的库是最基础的步骤。你需要安装wordcloud、matplotlib、Pillow等库,这些库可以帮助我们生成和展示词云图像。具体来说,你可以使用pip
命令来安装这些库,如pip install wordcloud matplotlib Pillow
。安装完成后,就可以开始准备文本数据,并使用wordcloud库生成词云图像了。
接下来,我将详细描述如何利用Python制作词云。
一、安装必要的库
在开始制作词云之前,我们需要确保安装了所需的库。常用的库包括wordcloud、matplotlib和Pillow。这些库可以帮助我们生成词云并进行可视化。
1、安装wordcloud库
wordcloud是一个流行的Python库,用于生成词云图像。你可以使用以下命令安装它:
pip install wordcloud
2、安装matplotlib库
matplotlib是一个强大的绘图库,用于在Python中创建静态、动画和交互式可视化。你可以使用以下命令安装它:
pip install matplotlib
3、安装Pillow库
Pillow是Python Imaging Library (PIL) 的一个友好的分支,用于处理图像。你可以使用以下命令安装它:
pip install Pillow
二、准备文本数据
在生成词云之前,我们需要准备好文本数据。文本数据可以是任何形式的文本,如书籍、文章、网页内容等。下面是一个简单的示例,展示如何从文本文件中读取数据并生成词云。
1、读取文本文件
首先,我们需要读取文本文件中的内容。假设你有一个名为sample.txt
的文本文件,你可以使用以下代码读取文件内容:
with open('sample.txt', 'r', encoding='utf-8') as file:
text = file.read()
2、处理文本数据
在生成词云之前,可能需要对文本数据进行一些预处理。例如,去除停用词、标点符号等。你可以使用正则表达式和Python的字符串处理函数来完成这些操作。
import re
去除标点符号
text = re.sub(r'[^\w\s]', '', text)
去除停用词
stopwords = set(['the', 'and', 'to', 'of', 'a', 'in', 'that', 'is', 'for', 'on', 'with', 'as', 'are', 'by', 'it', 'an', 'be', 'this', 'which', 'or', 'from', 'at', 'was', 'not', 'but', 'have', 'has', 'had', 'were', 'they', 'their', 'its', 'we', 'you', 'his', 'her', 'can', 'will', 'all', 'our', 'one', 'been', 'if', 'there', 'more', 'when', 'would', 'who', 'what', 'so', 'no', 'out', 'about', 'up', 'some', 'them', 'he', 'she', 'my', 'me', 'do', 'does', 'did', 'your', 'how', 'than', 'us', 'over', 'these', 'into', 'then', 'any', 'other', 'may', 'such', 'could', 'should', 'after', 'just', 'only', 'new', 'like', 'now', 'used', 'use', 'also', 'many', 'those', 'each', 'because', 'between', 'way', 'under', 'while', 'again', 'back', 'through', 'much', 'before', 'two', 'three', 'most', 'same', 'well', 'being', 'since', 'upon', 'during', 'every', 'both', 'another', 'however', 'even', 'few', 'where', 'first', 'last', 'next', 'until', 'though', 'still', 'often', 'doesnt', 'dont', 'arent', 'isnt', 'wont', 'cant', 'didnt', 'hasnt', 'havent', 'wouldnt', 'couldnt', 'shouldnt', 'cannot', 'wont', 'wasnt', 'werent', 'youre', 'youve', 'youll', 'youre', 'theyre', 'theyve', 'theyll', 'theyre', 'hes', 'shes', 'its', 'theres', 'heres', 'whos', 'whats', 'whens', 'wheres', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys', 'hows', 'im', 'ive', 'ill', 'id', 'weve', 'well', 'wed', 'lets', 'thats', 'whos', 'whats', 'wheres', 'whens', 'whys
相关问答FAQs:
如何开始使用Python制作词云?
要开始制作词云,首先需要安装相关的Python库,如wordcloud
、matplotlib
和numpy
。通过使用pip install wordcloud matplotlib numpy
命令可以轻松安装这些库。接下来,准备一个文本文件或字符串作为数据源,使用WordCloud
类生成词云图像,并通过matplotlib
库展示出来。这样,您就可以创建出具有视觉冲击力的词云。
制作词云时可以使用哪些自定义选项?
在制作词云时,可以通过设置多个参数来自定义词云的外观。例如,您可以调整词云的形状、颜色、字体和背景色等。WordCloud
类提供了参数如background_color
(背景颜色)、width
和height
(词云图像的宽和高),以及colormap
(颜色映射表)等。这些选项使您能够创建符合个人风格的独特词云。
如何处理和清理文本数据以生成更好的词云?
为了生成更具可读性和美观的词云,清理和处理文本数据非常重要。可以通过去除停用词、标点符号和特殊字符来提升词云的质量。使用nltk
或re
库可以帮助您实现这些操作。此外,考虑对词语进行词干提取或词形还原,以确保相似词汇被合并,从而使词云更具代表性。
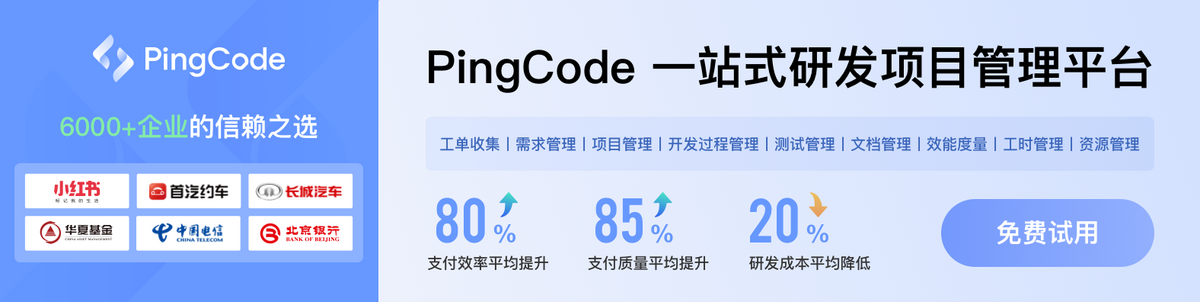