Python删除标点符号的方法有多种,包括使用正则表达式、字符串翻译表、自然语言处理库等。其中,常用的方法有使用str.translate()、re库、string库进行标点符号的删除。推荐使用str.translate()方法,因为其效率较高且易于理解。
下面将详细介绍使用str.translate()方法删除标点符号的具体步骤。
一、使用str.translate()方法
str.translate()方法通过将字符映射到另一字符来实现删除或替换。它结合str.maketrans()方法,可以高效地删除标点符号。
import string
def remove_punctuation(text):
translator = str.maketrans('', '', string.punctuation)
return text.translate(translator)
text = "Hello, world! This is a test."
clean_text = remove_punctuation(text)
print(clean_text) # 输出: Hello world This is a test
在上面的代码中,string.punctuation
包含了所有的标点符号,str.maketrans()
创建一个翻译表,将标点符号映射为空字符,从而实现删除标点符号。
优点
- 高效:str.translate()方法的性能较高,对于大文本的处理速度快。
- 简洁:代码简洁明了,易于理解和维护。
二、使用正则表达式
正则表达式是处理字符串的强大工具,使用re模块可以方便地删除标点符号。
import re
def remove_punctuation(text):
return re.sub(r'[^\w\s]', '', text)
text = "Hello, world! This is a test."
clean_text = remove_punctuation(text)
print(clean_text) # 输出: Hello world This is a test
在上面的代码中,re.sub(r'[^\w\s]', '', text)
通过正则表达式匹配所有非字母数字和空白字符的内容,并替换为空字符,从而删除标点符号。
优点
- 灵活:正则表达式可以根据需要灵活定制匹配规则。
- 功能强大:适用于各种复杂的文本处理需求。
三、使用自然语言处理库
一些自然语言处理库,如nltk,也提供了删除标点符号的功能。
import nltk
from nltk.tokenize import word_tokenize
from nltk.corpus import stopwords
nltk.download('punkt')
nltk.download('stopwords')
def remove_punctuation(text):
words = word_tokenize(text)
words = [word for word in words if word.isalnum()]
return ' '.join(words)
text = "Hello, world! This is a test."
clean_text = remove_punctuation(text)
print(clean_text) # 输出: Hello world This is a test
在上面的代码中,word_tokenize()
方法将文本分词,然后通过isalnum()
方法过滤掉非字母数字的词汇,从而删除标点符号。
优点
- 全面:适用于复杂的文本处理任务,提供了丰富的功能和工具。
- 高效:对于大量文本处理和自然语言处理任务,nltk库性能较好。
四、使用字符串替换方法
虽然不如上述方法高效,但直接使用字符串替换方法也可以实现删除标点符号。
def remove_punctuation(text):
punctuation = '''!()-[]{};:'"\,<>./?@#$%^&*_~'''
for char in text:
if char in punctuation:
text = text.replace(char, "")
return text
text = "Hello, world! This is a test."
clean_text = remove_punctuation(text)
print(clean_text) # 输出: Hello world This is a test
在上面的代码中,手动列出了所有的标点符号,并逐个替换为空字符,从而实现删除标点符号。
优点
- 简单:代码简单易懂,适合初学者使用。
- 直观:直接列出需要删除的标点符号,操作直观。
五、总结
删除标点符号的方法多种多样,选择适合的方法取决于具体需求和场景。推荐使用str.translate()方法,其高效且易于理解,适用于大部分文本处理任务。对于复杂的文本处理任务,可以考虑使用正则表达式和自然语言处理库。无论使用哪种方法,了解其优缺点和适用场景,才能更好地解决问题。
相关问答FAQs:
如何在Python中识别和删除文本中的所有标点符号?
在Python中,可以使用string
模块中的punctuation
常量来识别标点符号。通过列表推导式和join
方法,可以轻松地从字符串中删除所有标点符号。例如:
import string
text = "Hello, world! This is a test."
cleaned_text = ''.join(char for char in text if char not in string.punctuation)
print(cleaned_text) # 输出: Hello world This is a test
有没有现成的库可以帮助我删除文本中的标点符号?
是的,Python中的re
模块可以非常有效地处理正则表达式,从而删除标点符号。利用re.sub()
函数,可以通过简单的模式匹配来移除标点。示例如下:
import re
text = "Hello, world! This is a test."
cleaned_text = re.sub(r'[^\w\s]', '', text)
print(cleaned_text) # 输出: Hello world This is a test
在处理中文文本时,如何确保标点符号被正确删除?
对于中文文本,可以使用相似的方法,re
模块同样适用。由于中文标点符号与英文标点符号不同,您可以自定义正则表达式以匹配中文标点。例如:
import re
text = "你好,世界!这是一段测试。"
cleaned_text = re.sub(r'[,。!?]', '', text)
print(cleaned_text) # 输出: 你好世界这是一段测试
通过这种方式,您可以根据需要添加或删除特定的标点符号。
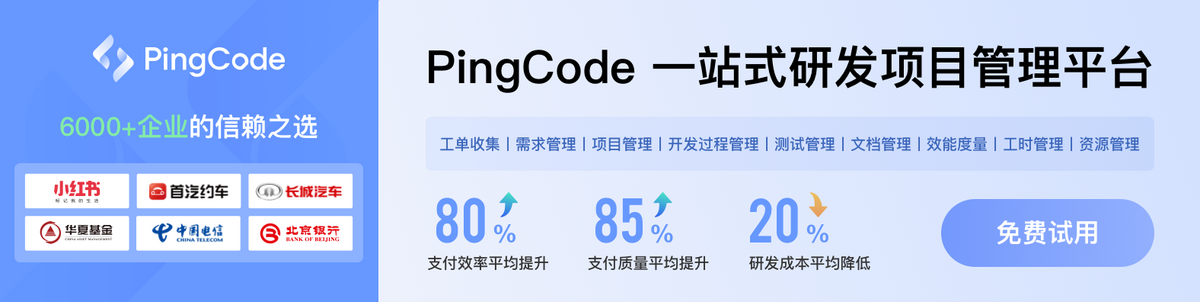