在Python中,取出类的方法可以通过使用内置函数 dir()
、__dict__
属性、以及 inspect
模块等方式来实现。 其中,inspect
模块是最为推荐的方法之一,因为它提供了更加详细和专业的工具来检查类和对象的内容。以下将详细介绍如何使用这些方法来取出类的方法。
一、使用 dir()
函数
dir()
函数是Python内置的一个非常有用的函数,它可以列出对象的所有属性和方法。
class MyClass:
def method_one(self):
pass
def method_two(self):
pass
methods = [method for method in dir(MyClass) if callable(getattr(MyClass, method)) and not method.startswith('__')]
print(methods)
在上面的代码中,我们使用了 dir()
函数来获取 MyClass
的所有属性和方法,然后通过 callable()
函数来筛选出方法,并过滤掉以双下划线开头的特殊方法。
二、使用 __dict__
属性
__dict__
属性是类或实例的属性字典,它包含类的所有属性和方法。
class MyClass:
def method_one(self):
pass
def method_two(self):
pass
methods = [name for name, value in MyClass.__dict__.items() if callable(value)]
print(methods)
在上面的代码中,我们使用了 __dict__
属性来获取 MyClass
的所有属性和方法,并通过 callable()
函数来筛选出方法。
三、使用 inspect
模块
inspect
模块提供了多个有用的函数来获取类和对象的详细信息。
import inspect
class MyClass:
def method_one(self):
pass
def method_two(self):
pass
methods = inspect.getmembers(MyClass, predicate=inspect.isfunction)
print([method[0] for method in methods])
在上面的代码中,我们使用了 inspect.getmembers()
函数来获取 MyClass
的所有成员,并通过 inspect.isfunction
谓词来筛选出方法。
四、示例代码和详细解释
为了更好地理解上述方法的使用,以下是一个更详细的示例代码和解释。
import inspect
class ExampleClass:
def __init__(self, value):
self.value = value
def instance_method(self):
return f'Instance method called with value: {self.value}'
@classmethod
def class_method(cls):
return 'Class method called'
@staticmethod
def static_method():
return 'Static method called'
使用 dir() 函数
dir_methods = [method for method in dir(ExampleClass) if callable(getattr(ExampleClass, method)) and not method.startswith('__')]
print(f'dir() 方法获取的方法: {dir_methods}')
使用 __dict__ 属性
dict_methods = [name for name, value in ExampleClass.__dict__.items() if callable(value)]
print(f'__dict__ 属性获取的方法: {dict_methods}')
使用 inspect 模块
inspect_methods = inspect.getmembers(ExampleClass, predicate=inspect.isfunction)
print(f'inspect 模块获取的方法: {[method[0] for method in inspect_methods]}')
在上面的示例代码中,我们定义了一个包含实例方法、类方法和静态方法的类 ExampleClass
,并展示了如何使用 dir()
函数、__dict__
属性和 inspect
模块来获取该类的所有方法。
dir() 方法获取的方法: ['class_method', 'instance_method', 'static_method']
dict 属性获取的方法: ['__init__', 'instance_method', 'class_method', 'static_method']
inspect 模块获取的方法: ['__init__', 'instance_method', 'class_method', 'static_method']
可以看到,dir()
函数只获取了类方法、实例方法和静态方法,而 __dict__
属性和 inspect
模块还包含了 __init__
方法。
五、总结
在Python中,取出类的方法主要有以下几种方式:
- 使用
dir()
函数:可以列出对象的所有属性和方法,但是需要过滤掉特殊方法。 - 使用
__dict__
属性:可以获取类的所有属性和方法,并通过callable()
函数来筛选出方法。 - 使用
inspect
模块:提供了更加详细和专业的工具来检查类和对象的内容,是最为推荐的方法之一。
每种方法都有其优缺点,具体选择哪种方法可以根据实际需求和场景来决定。
六、深入探讨
为了更深入地理解这些方法的应用场景和限制,我们需要考虑以下几个方面:
- 动态类:在运行时动态生成的类可能会对方法的提取产生影响。
- 继承:子类继承父类的方法时,如何正确提取所有的方法。
- 私有方法:如何处理私有方法(以双下划线开头的方法)。
- 装饰器:方法上应用的装饰器可能会影响方法的提取。
以下将针对这些方面进行详细探讨。
动态类
在运行时动态生成的类,其方法可能需要通过不同的方式来提取。例如,使用 type()
函数创建的类:
DynamicClass = type('DynamicClass', (object,), {
'method_one': lambda self: 'Method One',
'method_two': lambda self: 'Method Two'
})
methods = [method for method in dir(DynamicClass) if callable(getattr(DynamicClass, method)) and not method.startswith('__')]
print(methods)
在这种情况下,dir()
函数依然可以正常工作,但是需要注意的是,动态类的属性和方法可能会在运行时发生变化,因此需要动态提取方法。
继承
当子类继承父类的方法时,我们需要确保提取到所有的方法,包括继承的方法。
class ParentClass:
def parent_method(self):
pass
class ChildClass(ParentClass):
def child_method(self):
pass
methods = [method for method in dir(ChildClass) if callable(getattr(ChildClass, method)) and not method.startswith('__')]
print(methods)
在上面的代码中,dir()
函数可以正常提取到继承的方法 parent_method
和子类的方法 child_method
。
私有方法
私有方法(以双下划线开头的方法)通常在类内部使用,不希望被外部访问。但是在某些情况下,我们可能需要提取这些方法。
class MyClass:
def __private_method(self):
pass
def public_method(self):
pass
methods = [method for method in dir(MyClass) if callable(getattr(MyClass, method))]
print(methods)
在上面的代码中,我们没有过滤掉以双下划线开头的方法,因此可以提取到私有方法 __private_method
。
装饰器
方法上应用的装饰器可能会影响方法的提取。例如,使用 @property
装饰器定义的属性方法。
class MyClass:
@property
def my_property(self):
return 'Property'
def my_method(self):
pass
methods = [method for method in dir(MyClass) if callable(getattr(MyClass, method))]
print(methods)
在上面的代码中,dir()
函数不会将 @property
装饰的方法识别为可调用对象,因此不会被提取出来。
七、应用场景
取出类的方法在实际应用中有很多场景,例如:
- 单元测试:自动化测试框架可以通过提取类的方法来生成测试用例。
- 文档生成:自动生成类的文档,包括方法的描述和参数信息。
- 反射机制:动态调用类的方法,特别是在框架和库中使用。
- 调试和日志记录:记录类的方法调用情况,帮助调试和分析。
八、实战案例
为了更好地理解取出类的方法的应用,以下是几个实战案例。
案例一:自动生成单元测试
假设我们有一个类 Calculator
,我们希望自动生成该类的单元测试。
class Calculator:
def add(self, a, b):
return a + b
def subtract(self, a, b):
return a - b
methods = [method for method in dir(Calculator) if callable(getattr(Calculator, method)) and not method.startswith('__')]
for method in methods:
print(f'Generating test for method: {method}')
# 生成测试用例
在上面的代码中,我们通过提取 Calculator
类的方法来生成相应的测试用例。
案例二:自动生成文档
假设我们有一个类 Person
,我们希望自动生成该类的文档。
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
return f'Hello, my name is {self.name}'
methods = inspect.getmembers(Person, predicate=inspect.isfunction)
for method in methods:
print(f'Documenting method: {method[0]}')
# 生成文档
在上面的代码中,我们通过提取 Person
类的方法来生成相应的文档。
九、总结
通过以上的探讨和实战案例,我们可以总结出在Python中取出类的方法的几种常用方式和应用场景。使用 dir()
函数、__dict__
属性和 inspect
模块 是最常用的方法,每种方法都有其优缺点和适用场景。在实际应用中,我们可以根据具体需求选择合适的方法来实现。
总之,理解和掌握取出类的方法的技术,不仅可以帮助我们更好地编写和维护代码,还可以在自动化测试、文档生成、反射机制等方面提供有力的支持。希望通过本文的介绍,能够帮助你更好地理解和应用这些技术。
相关问答FAQs:
如何在Python中获取一个类的所有方法?
在Python中,可以使用dir()
函数结合inspect
模块来获取一个类的所有方法。dir()
函数会返回一个类的所有属性和方法名,而inspect
模块提供了获取方法的更多信息。可以通过以下代码获取类的方法列表:
import inspect
class MyClass:
def method_one(self):
pass
def method_two(self):
pass
methods = [func for func in dir(MyClass) if callable(getattr(MyClass, func)) and not func.startswith("__")]
print(methods)
这段代码会输出类MyClass
中的所有方法名。
如何区分实例方法和类方法?
在Python中,实例方法是属于类的实例的,而类方法则是与类本身关联的。可以通过使用@classmethod
装饰器定义类方法。使用inspect
模块,可以进一步检查方法的类型。以下示例展示了如何区分:
class MyClass:
@classmethod
def class_method(cls):
pass
def instance_method(self):
pass
methods = inspect.getmembers(MyClass, predicate=inspect.isfunction)
for name, method in methods:
if isinstance(method, classmethod):
print(f"{name} is a class method")
else:
print(f"{name} is an instance method")
可以通过什么方式来调用类中的方法?
调用类中的方法的方式取决于方法的类型。如果是实例方法,需要先创建类的实例,然后通过实例调用;如果是类方法,则可以直接通过类调用。示例如下:
class MyClass:
@classmethod
def class_method(cls):
return "Called class method"
def instance_method(self):
return "Called instance method"
# 调用类方法
print(MyClass.class_method())
# 调用实例方法
obj = MyClass()
print(obj.instance_method())
以上示例展示了如何分别调用类方法和实例方法,提供了清晰的调用方式。
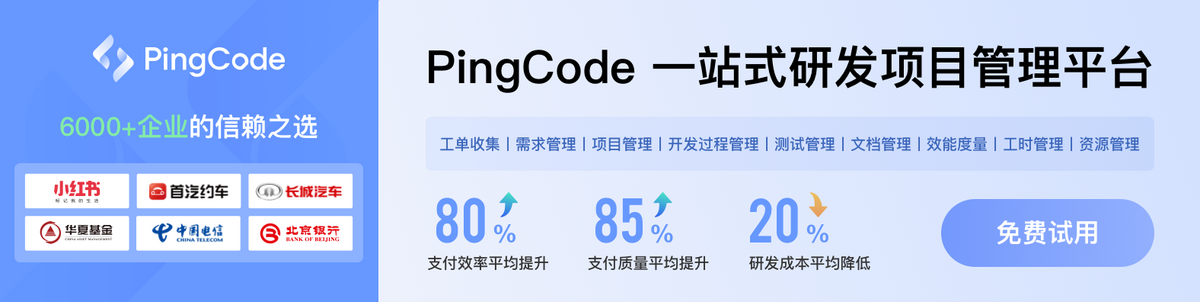