在Python中杀掉线程的方法包括:使用标志位、利用守护线程、使用线程池、以及使用第三方库(如threading2
)等。在这些方法中,使用标志位是一种较为常见且安全的方式。具体来说,通过设置一个全局变量或共享变量,在线程运行过程中定期检查该变量的值,如果值发生改变则终止线程的执行。
例如,假设我们有一个线程在执行某个耗时任务,我们可以在任务开始之前设置一个标志位,在任务执行过程中不断检查该标志位的值,如果标志位被设置为True,则终止任务的执行。
import threading
import time
定义一个标志位
stop_thread = False
def thread_task():
global stop_thread
while not stop_thread:
print("Thread is running...")
time.sleep(1)
print("Thread has been stopped.")
创建线程
thread = threading.Thread(target=thread_task)
启动线程
thread.start()
主线程等待5秒钟
time.sleep(5)
设置标志位为True,终止线程
stop_thread = True
等待线程结束
thread.join()
print("Main thread is finished.")
在这个示例中,我们通过设置stop_thread
标志位来控制线程的执行。当主线程等待5秒钟后,将stop_thread
设置为True,子线程检测到标志位的变化后退出循环,终止任务的执行。
接下来,我们将详细介绍Python中杀掉线程的其他方法和场景应用。
一、使用标志位
标志位是一种简单而有效的线程控制方法。通过在主线程中设置一个标志位,在线程执行过程中不断检查该标志位的值,如果标志位被设置为终止状态,则线程可以安全地退出。
1.1、实现步骤
- 定义一个全局变量或共享变量作为标志位。
- 在线程任务函数中定期检查标志位的值。
- 主线程设置标志位以终止线程。
1.2、示例代码
import threading
import time
定义一个标志位
stop_thread = False
def thread_task():
global stop_thread
while not stop_thread:
print("Thread is running...")
time.sleep(1)
print("Thread has been stopped.")
创建线程
thread = threading.Thread(target=thread_task)
启动线程
thread.start()
主线程等待5秒钟
time.sleep(5)
设置标志位为True,终止线程
stop_thread = True
等待线程结束
thread.join()
print("Main thread is finished.")
在这个示例中,我们通过设置stop_thread
标志位来控制线程的执行。当主线程等待5秒钟后,将stop_thread
设置为True,子线程检测到标志位的变化后退出循环,终止任务的执行。
二、使用守护线程
守护线程是一种特殊的线程,它的生命周期依赖于主线程。当主线程结束时,守护线程会自动退出。我们可以通过设置线程为守护线程来控制线程的执行。
2.1、实现步骤
- 创建线程时设置为守护线程。
- 主线程结束时,守护线程自动退出。
2.2、示例代码
import threading
import time
def thread_task():
while True:
print("Daemon thread is running...")
time.sleep(1)
创建守护线程
daemon_thread = threading.Thread(target=thread_task)
daemon_thread.setDaemon(True)
启动守护线程
daemon_thread.start()
主线程等待5秒钟
time.sleep(5)
主线程结束,守护线程自动退出
print("Main thread is finished.")
在这个示例中,我们创建了一个守护线程,并设置其为守护线程。当主线程等待5秒钟后结束,守护线程也会自动退出。
三、使用线程池
线程池是一种管理线程的高级方式,通过线程池可以方便地管理和控制线程的生命周期。在Python中,可以使用concurrent.futures
模块提供的ThreadPoolExecutor
类来创建和管理线程池。
3.1、实现步骤
- 创建一个线程池。
- 向线程池提交任务。
- 通过
shutdown
方法终止线程池中的所有线程。
3.2、示例代码
import concurrent.futures
import time
def thread_task():
while True:
print("Thread pool task is running...")
time.sleep(1)
创建线程池
with concurrent.futures.ThreadPoolExecutor(max_workers=2) as executor:
# 向线程池提交任务
future = executor.submit(thread_task)
# 主线程等待5秒钟
time.sleep(5)
# 通过shutdown方法终止线程池中的所有线程
executor.shutdown(wait=False)
print("Main thread is finished.")
在这个示例中,我们创建了一个线程池,并向线程池提交了一个任务。主线程等待5秒钟后,通过shutdown
方法终止线程池中的所有线程。
四、使用第三方库
Python中还有一些第三方库可以帮助我们更好地管理线程的生命周期。例如,threading2
库提供了一些高级功能,可以方便地终止线程。
4.1、安装threading2
库
pip install threading2
4.2、示例代码
import threading2
import time
def thread_task():
while True:
print("threading2 task is running...")
time.sleep(1)
创建线程
thread = threading2.Thread(target=thread_task)
启动线程
thread.start()
主线程等待5秒钟
time.sleep(5)
终止线程
thread.terminate()
print("Main thread is finished.")
在这个示例中,我们使用threading2
库提供的Thread
类创建了一个线程,并在主线程等待5秒钟后终止该线程。
五、使用信号量控制线程
信号量是一种用于限制资源访问的机制,通过信号量可以控制线程的执行。在Python中,可以使用threading
模块提供的Semaphore
类来实现信号量控制。
5.1、实现步骤
- 创建一个信号量对象。
- 在线程任务函数中使用信号量控制线程的执行。
- 主线程通过释放信号量来终止线程。
5.2、示例代码
import threading
import time
创建一个信号量对象
sem = threading.Semaphore(0)
def thread_task():
while True:
print("Semaphore thread is running...")
time.sleep(1)
sem.acquire()
if sem._value == -1:
break
print("Semaphore thread has been stopped.")
创建线程
thread = threading.Thread(target=thread_task)
启动线程
thread.start()
主线程等待5秒钟
time.sleep(5)
释放信号量,终止线程
sem.release()
print("Main thread is finished.")
在这个示例中,我们创建了一个信号量对象,并在线程任务函数中使用信号量控制线程的执行。当主线程等待5秒钟后,释放信号量以终止线程。
六、使用条件变量控制线程
条件变量是一种用于线程间通信的机制,通过条件变量可以实现线程的同步和控制。在Python中,可以使用threading
模块提供的Condition
类来实现条件变量控制。
6.1、实现步骤
- 创建一个条件变量对象。
- 在线程任务函数中使用条件变量控制线程的执行。
- 主线程通过通知条件变量来终止线程。
6.2、示例代码
import threading
import time
创建一个条件变量对象
condition = threading.Condition()
def thread_task():
with condition:
while True:
print("Condition thread is running...")
time.sleep(1)
condition.wait()
if condition._is_owned():
break
print("Condition thread has been stopped.")
创建线程
thread = threading.Thread(target=thread_task)
启动线程
thread.start()
主线程等待5秒钟
time.sleep(5)
通知条件变量,终止线程
with condition:
condition.notify_all()
print("Main thread is finished.")
在这个示例中,我们创建了一个条件变量对象,并在线程任务函数中使用条件变量控制线程的执行。当主线程等待5秒钟后,通知条件变量以终止线程。
七、使用事件对象控制线程
事件对象是一种用于线程间同步的机制,通过事件对象可以实现线程的控制。在Python中,可以使用threading
模块提供的Event
类来实现事件对象控制。
7.1、实现步骤
- 创建一个事件对象。
- 在线程任务函数中使用事件对象控制线程的执行。
- 主线程通过设置事件对象来终止线程。
7.2、示例代码
import threading
import time
创建一个事件对象
event = threading.Event()
def thread_task():
while not event.is_set():
print("Event thread is running...")
time.sleep(1)
print("Event thread has been stopped.")
创建线程
thread = threading.Thread(target=thread_task)
启动线程
thread.start()
主线程等待5秒钟
time.sleep(5)
设置事件对象,终止线程
event.set()
print("Main thread is finished.")
在这个示例中,我们创建了一个事件对象,并在线程任务函数中使用事件对象控制线程的执行。当主线程等待5秒钟后,设置事件对象以终止线程。
八、使用定时器控制线程
定时器是一种用于在指定时间后执行某个函数的机制,通过定时器可以实现线程的控制。在Python中,可以使用threading
模块提供的Timer
类来实现定时器控制。
8.1、实现步骤
- 创建一个定时器对象。
- 在定时器到期后执行指定的函数以终止线程。
8.2、示例代码
import threading
import time
def thread_task():
while True:
print("Timer thread is running...")
time.sleep(1)
def stop_thread():
global stop_thread_flag
stop_thread_flag = True
print("Timer thread has been stopped.")
定义一个标志位
stop_thread_flag = False
创建线程
thread = threading.Thread(target=thread_task)
启动线程
thread.start()
创建定时器,5秒后终止线程
timer = threading.Timer(5, stop_thread)
timer.start()
等待线程结束
while not stop_thread_flag:
time.sleep(1)
print("Main thread is finished.")
在这个示例中,我们创建了一个定时器,并在定时器到期后执行指定的函数以终止线程。主线程等待定时器到期后,终止线程的执行。
总结
通过上述几种方法,我们可以在Python中灵活地控制和终止线程的执行。根据具体的应用场景和需求,可以选择合适的方法来实现线程的管理和控制。其中,使用标志位是一种较为常见且安全的方式,可以避免强制终止线程带来的潜在风险。此外,合理地使用守护线程、线程池、信号量、条件变量、事件对象和定时器等机制,可以更好地实现线程的管理和控制。
相关问答FAQs:
如何安全地停止一个正在运行的线程?
在Python中,安全停止线程通常是通过设置一个标志位来实现的。在线程中,可以定期检查这个标志,如果标志被设置,线程就可以安全地退出。使用threading.Event
对象是一种常见的方法,可以让线程在需要时优雅地结束。
Python中有没有内置的方法可以强制终止线程?
Python标准库并没有提供直接强制终止线程的方法。这是因为强制结束线程可能导致资源泄漏或锁未释放等问题。因此,使用标志位和threading.Event
来请求线程结束是更为推荐的方式。
如何管理多个线程的生命周期?
管理多个线程的生命周期可以使用Thread.join()
方法。通过调用此方法,主线程可以等待特定线程的完成,确保所有线程在程序退出前完成其工作。此外,使用线程池(如concurrent.futures.ThreadPoolExecutor
)可以更轻松地管理多个线程的启动和结束,从而提高代码的可读性和效率。
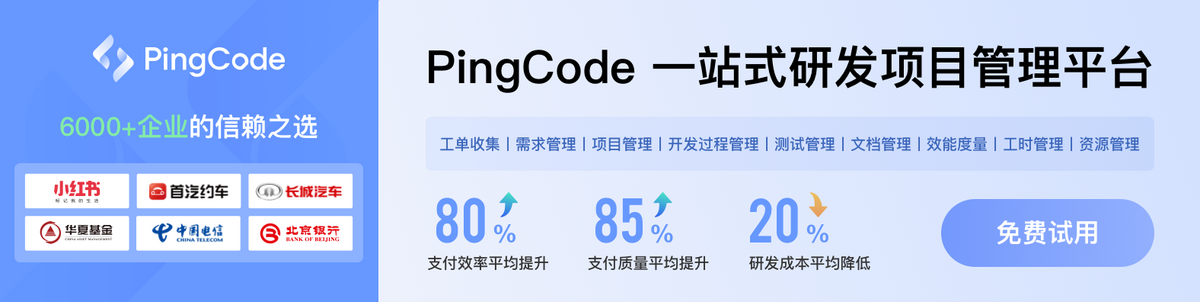