在Python中,出现“x00”的情况通常与处理字符串和字节数据相关。使用字符串表示、使用字节表示、使用格式化字符串、使用转义字符都可能导致“x00”的出现。接下来,我们将详细描述使用格式化字符串这一点,并深入探讨其他方法的实现。
使用格式化字符串可以让我们在字符串中插入特定的字符或字节值。例如,我们可以使用格式化字符串来插入十六进制表示的字符。以下是一个示例代码:
hex_value = '\x00'
formatted_string = f'The hex value is: {hex_value}'
print(formatted_string)
在这个示例中,\x00
表示十六进制值0,formatted_string
中插入了这个值并打印出来。
接下来,我们将详细介绍其他方法,并探讨在各种场景中如何使用这些方法。
一、字符串表示
字符串表示是处理文本数据的基本方法。在Python中,字符串可以通过单引号、双引号和三引号来表示。以下是一些常见的字符串操作:
# 单引号和双引号
single_quote_str = 'This is a string with single quotes.'
double_quote_str = "This is a string with double quotes."
三引号(用于多行字符串)
multi_line_str = '''This is a multi-line string.
It spans multiple lines.
'''
插入特殊字符
special_char_str = 'This is a string with a newline character.\nAnd this is a new line.'
print(single_quote_str)
print(double_quote_str)
print(multi_line_str)
print(special_char_str)
字符串转义字符
在字符串中,我们可以使用转义字符来表示一些特殊字符,例如换行符(\n
)、制表符(\t
)以及十六进制字符(\x00
)。以下是一些常见的转义字符:
# 换行符
newline_str = 'This is a string with a newline character.\nThis is a new line.'
制表符
tab_str = 'This is a string with a tab character.\tThis is after the tab.'
十六进制字符
hex_char_str = 'This is a string with a hex character: \x41' # \x41 表示字符 'A'
print(newline_str)
print(tab_str)
print(hex_char_str)
二、字节表示
在处理二进制数据时,我们通常使用字节表示。Python中的字节类型是bytes
,表示不可变的字节序列。以下是一些常见的字节操作:
# 创建字节对象
byte_obj = b'This is a byte object.'
字节对象中的十六进制表示
hex_byte_obj = b'\x00\x41\x42' # 包含字节值 0x00, 0x41 ('A'), 0x42 ('B')
转换为字符串(假设是ASCII编码)
decoded_str = byte_obj.decode('ascii')
print(byte_obj)
print(hex_byte_obj)
print(decoded_str)
字节与字符串的转换
在处理网络数据或文件时,我们经常需要在字节和字符串之间进行转换。以下是一些常见的转换方法:
# 字符串到字节
str_data = 'Hello, World!'
byte_data = str_data.encode('utf-8')
字节到字符串
decoded_str = byte_data.decode('utf-8')
print(byte_data)
print(decoded_str)
三、格式化字符串
格式化字符串是插入变量和特定字符到字符串中的一种方式。在Python中,我们可以使用%
运算符、str.format()
方法和f-string来进行格式化。以下是一些示例:
使用%
运算符
name = 'John'
age = 30
formatted_str = 'My name is %s and I am %d years old.' % (name, age)
print(formatted_str)
使用str.format()
方法
name = 'John'
age = 30
formatted_str = 'My name is {} and I am {} years old.'.format(name, age)
print(formatted_str)
使用f-string
name = 'John'
age = 30
formatted_str = f'My name is {name} and I am {age} years old.'
print(formatted_str)
四、转义字符
转义字符用于在字符串中插入一些无法直接输入的字符,例如换行符、制表符和引号。在Python中,常见的转义字符包括:
\n
:换行符\t
:制表符\
:反斜杠\'
:单引号\"
:双引号\xhh
:十六进制字符
以下是一些示例代码:
# 换行符
newline_str = 'This is a string with a newline character.\nThis is a new line.'
制表符
tab_str = 'This is a string with a tab character.\tThis is after the tab.'
单引号和双引号
single_quote_str = 'This is a string with a single quote: \''
double_quote_str = "This is a string with a double quote: \""
十六进制字符
hex_char_str = 'This is a string with a hex character: \x41' # \x41 表示字符 'A'
print(newline_str)
print(tab_str)
print(single_quote_str)
print(double_quote_str)
print(hex_char_str)
五、字节操作与文件处理
在处理文件时,我们经常需要读取和写入字节数据。Python提供了多种文件操作方法,例如open()
函数。以下是一些示例:
读取字节数据
# 打开文件以字节模式读取
with open('example.bin', 'rb') as file:
byte_data = file.read()
print(byte_data)
写入字节数据
# 打开文件以字节模式写入
with open('output.bin', 'wb') as file:
byte_data = b'\x00\x41\x42'
file.write(byte_data)
读取文本数据
# 打开文件以文本模式读取
with open('example.txt', 'r') as file:
text_data = file.read()
print(text_data)
写入文本数据
# 打开文件以文本模式写入
with open('output.txt', 'w') as file:
text_data = 'Hello, World!'
file.write(text_data)
六、二进制数据处理
在处理二进制数据时,我们通常需要进行位操作、字节操作和解析数据结构。Python提供了丰富的库和函数来处理二进制数据,例如struct
模块。以下是一些示例:
位操作
# 按位与
a = 0b1100
b = 0b1010
result = a & b # 结果是 0b1000
按位或
result = a | b # 结果是 0b1110
按位异或
result = a ^ b # 结果是 0b0110
按位取反
result = ~a # 结果是 -0b1101
左移位
result = a << 2 # 结果是 0b110000
右移位
result = a >> 2 # 结果是 0b0011
print(result)
使用struct
模块解析数据结构
import struct
定义二进制数据
byte_data = b'\x00\x01\x00\x02'
解析数据
unpacked_data = struct.unpack('>HH', byte_data) # '>HH' 表示大端字节序的两个无符号短整型
print(unpacked_data) # 输出: (1, 2)
构建二进制数据
import struct
构建数据
packed_data = struct.pack('>HH', 1, 2) # '>HH' 表示大端字节序的两个无符号短整型
print(packed_data) # 输出: b'\x00\x01\x00\x02'
七、处理网络数据
在网络编程中,我们经常需要处理字节数据。Python的socket
模块提供了丰富的网络操作功能。以下是一些示例:
发送和接收数据
import socket
创建客户端套接字
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
client_socket.connect(('localhost', 8080))
发送数据
client_socket.send(b'Hello, Server!')
接收数据
response = client_socket.recv(1024)
print(response)
关闭套接字
client_socket.close()
处理网络协议
在处理网络协议时,我们需要解析和构建数据包。以下是一个处理简单自定义协议的示例:
import struct
定义协议格式
HEADER_FORMAT = '>I' # 大端字节序的无符号整型
def send_message(socket, message):
# 构建数据包
header = struct.pack(HEADER_FORMAT, len(message))
packet = header + message.encode('utf-8')
socket.send(packet)
def receive_message(socket):
# 读取头部
header_data = socket.recv(struct.calcsize(HEADER_FORMAT))
if not header_data:
return None
message_length = struct.unpack(HEADER_FORMAT, header_data)[0]
# 读取消息
message_data = socket.recv(message_length)
return message_data.decode('utf-8')
使用示例
with socket.socket(socket.AF_INET, socket.SOCK_STREAM) as client_socket:
client_socket.connect(('localhost', 8080))
send_message(client_socket, 'Hello, Server!')
response = receive_message(client_socket)
print(response)
八、Unicode与编码
在处理文本数据时,我们需要了解Unicode和编码。Unicode是一种字符编码标准,能够表示几乎所有的书写系统。Python中的字符串默认使用Unicode。以下是一些常见的编码操作:
编码与解码
# 编码字符串为字节
unicode_str = 'Hello, 世界!'
utf8_bytes = unicode_str.encode('utf-8')
解码字节为字符串
decoded_str = utf8_bytes.decode('utf-8')
print(utf8_bytes)
print(decoded_str)
处理不同编码
在处理不同编码的文本数据时,我们需要指定正确的编码格式。以下是一些示例:
# 编码为GBK
gbk_bytes = unicode_str.encode('gbk')
解码为Unicode
decoded_str_gbk = gbk_bytes.decode('gbk')
print(gbk_bytes)
print(decoded_str_gbk)
九、正则表达式
正则表达式是一种强大的工具,用于匹配和操作字符串。Python的re
模块提供了丰富的正则表达式功能。以下是一些常见的操作:
匹配与查找
import re
匹配模式
pattern = r'\d+' # 匹配一个或多个数字
搜索字符串
match = re.search(pattern, 'The price is 100 dollars.')
if match:
print(f'Matched: {match.group()}')
替换与分割
# 替换匹配的子串
replaced_str = re.sub(pattern, 'XXX', 'The price is 100 dollars.')
print(replaced_str)
分割字符串
split_str = re.split(r'\s+', 'This is a test string.')
print(split_str)
十、异常处理
在编写Python代码时,处理异常是保证程序稳定性的重要手段。以下是一些常见的异常处理方法:
捕获异常
try:
result = 10 / 0
except ZeroDivisionError as e:
print(f'Error: {e}')
自定义异常
class CustomError(Exception):
pass
try:
raise CustomError('This is a custom error.')
except CustomError as e:
print(f'Error: {e}')
十一、数据结构
Python提供了丰富的数据结构,例如列表、元组、集合和字典。以下是一些常见的数据结构操作:
列表
# 创建列表
numbers = [1, 2, 3, 4, 5]
访问元素
print(numbers[0])
添加元素
numbers.append(6)
删除元素
numbers.remove(3)
print(numbers)
元组
# 创建元组
coordinates = (10.0, 20.0)
访问元素
print(coordinates[0])
元组是不可变的,无法修改元素
集合
# 创建集合
unique_numbers = {1, 2, 3, 4, 5}
添加元素
unique_numbers.add(6)
删除元素
unique_numbers.remove(3)
print(unique_numbers)
字典
# 创建字典
person = {'name': 'John', 'age': 30}
访问元素
print(person['name'])
修改元素
person['age'] = 31
添加元素
person['city'] = 'New York'
print(person)
十二、面向对象编程
Python支持面向对象编程(OOP),允许我们定义类和对象。以下是一些常见的OOP操作:
定义类与对象
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
print(f'Hello, my name is {self.name} and I am {self.age} years old.')
创建对象
john = Person('John', 30)
john.greet()
继承与多态
class Employee(Person):
def __init__(self, name, age, employee_id):
super().__init__(name, age)
self.employee_id = employee_id
def work(self):
print(f'Employee {self.name} is working.')
创建对象
jane = Employee('Jane', 25, 'E123')
jane.greet()
jane.work()
十三、装饰器
装饰器是一种特殊的函数,用于修改或扩展另一个函数的行为。以下是一些常见的装饰器操作:
定义装饰器
def my_decorator(func):
def wrapper():
print('Something is happening before the function is called.')
func()
print('Something is happening after the function is called.')
return wrapper
@my_decorator
def say_hello():
print('Hello!')
say_hello()
装饰器与参数
def my_decorator(func):
def wrapper(*args, kwargs):
print('Something is happening before the function is called.')
result = func(*args, kwargs)
print('Something is happening after the function is called.')
return result
return wrapper
@my_decorator
def greet(name):
print(f'Hello, {name}!')
greet('John')
十四、生成器
生成器是一种特殊的函数,用于生成迭代器。生成器使用yield
关键字来生成值。以下是一些常见的生成器操作:
定义生成器
def my_generator():
yield 1
yield 2
yield 3
使用生成器
for value in my_generator():
print(value)
生成器表达式
# 使用生成器表达式
gen_expr = (x * x for x in range(5))
for value in gen_expr:
print(value)
十五、模块与包
Python的模块和包用于组织代码和复用功能。以下是一些常见的模块与包操作:
导入模块
import math
使用模块
print(math.sqrt(16))
创建模块
# 创建一个名为 my_module.py 的模块
def greet(name):
return f'Hello, {name}!'
导入自定义模块
import my_module
print(my_module.greet('John'))
创建包
# 创建一个名为 my_package 的包
包含 __init__.py 文件和其他模块
__init__.py 文件可以为空或包含初始化代码
导入包
import my_package.my_module
print(my_package.my_module.greet('John'))
十六、并发编程
Python支持多种并发编程方式,例如多线程、多进程和异步编程。以下是一些常见的并发编程操作:
多线程
相关问答FAQs:
什么是 x00 在 Python 中的含义?
在 Python 中,x00 通常表示一个十六进制数,其中 x 表示十六进制的开始,而 00 则是该数字的具体值。十六进制数常用于表示字节或颜色值。例如,x00 可以表示一个字节的值为零,常用于处理二进制数据或进行位操作。
如何在 Python 中使用 x00 表示字节?
要在 Python 中使用 x00 表示字节,可以使用字节串(bytes)或字节数组(bytearray)。例如,可以创建一个包含 x00 的字节串,方法如下:my_bytes = b'\x00'
。这样就可以在后续的操作中使用这个字节。
在 Python 中如何将 x00 转换为整数?
可以使用内置的 int
函数将 x00 转换为整数。在 Python 中,十六进制数的转换可以通过在字符串前加上 0x
来实现,例如:int('0x00', 16)
将返回 0。这种方式适用于任意的十六进制数转换。
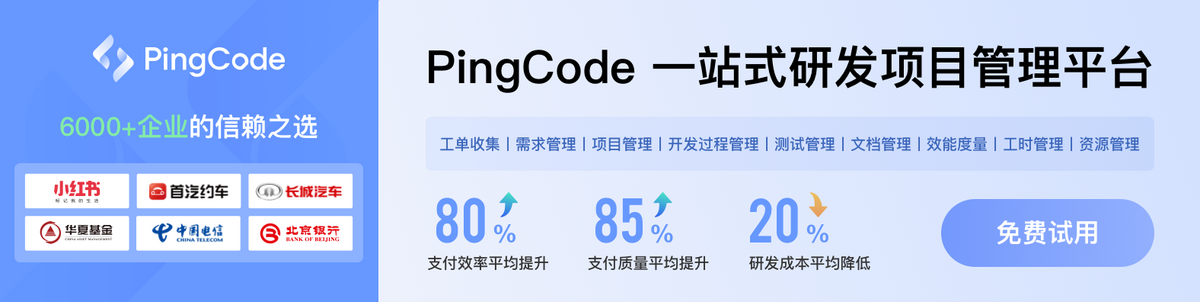