在Python中直接调用方法名主要有两种方式:通过对象调用方法、通过类调用静态方法。通过对象调用方法是最常见的调用方式,它需要先创建一个对象实例,然后通过该实例调用方法。通过类调用静态方法则是在不创建对象实例的情况下直接调用类中的静态方法。
一、通过对象调用方法
通过对象调用方法是最常见和直接的调用方式。首先需要定义一个类,然后创建该类的对象实例,通过实例对象调用方法。
1. 定义类和方法
class MyClass:
def __init__(self, value):
self.value = value
def display_value(self):
print("The value is:", self.value)
在上面的代码中,我们定义了一个名为MyClass
的类,类中有一个初始化方法__init__
和一个普通方法display_value
。
2. 创建对象并调用方法
obj = MyClass(10)
obj.display_value()
在上面的代码中,我们首先创建了一个MyClass
的对象实例obj
,然后通过obj
调用display_value
方法。
二、通过类调用静态方法
静态方法是类中的一种特殊方法,它不需要实例化类就可以直接调用。静态方法通常使用@staticmethod
装饰器来定义。
1. 定义静态方法
class MyClass:
@staticmethod
def static_method():
print("This is a static method.")
在上面的代码中,我们定义了一个静态方法static_method
,使用@staticmethod
装饰器来声明它为静态方法。
2. 直接调用静态方法
MyClass.static_method()
在上面的代码中,我们直接通过类名MyClass
调用静态方法static_method
,不需要创建对象实例。
三、通过内置函数getattr
调用方法
getattr
是Python中的一个内置函数,可以用于动态获取对象的属性或方法。
1. 使用getattr
调用对象方法
class MyClass:
def __init__(self, value):
self.value = value
def display_value(self):
print("The value is:", self.value)
obj = MyClass(10)
method = getattr(obj, 'display_value')
method()
在上面的代码中,我们使用getattr
函数动态获取对象obj
的display_value
方法,并调用该方法。
2. 使用getattr
调用类方法
class MyClass:
@staticmethod
def static_method():
print("This is a static method.")
method = getattr(MyClass, 'static_method')
method()
在上面的代码中,我们使用getattr
函数动态获取类MyClass
的static_method
方法,并调用该方法。
四、通过反射机制调用方法
反射机制是在运行时动态获取类的属性和方法的一种机制。Python中可以使用getattr
、setattr
等函数实现反射机制。
1. 使用反射机制调用对象方法
class MyClass:
def __init__(self, value):
self.value = value
def display_value(self):
print("The value is:", self.value)
obj = MyClass(10)
method_name = 'display_value'
if hasattr(obj, method_name):
method = getattr(obj, method_name)
method()
在上面的代码中,我们首先检查对象obj
是否具有名为display_value
的方法,如果有,则使用getattr
获取该方法并调用它。
2. 使用反射机制调用类方法
class MyClass:
@staticmethod
def static_method():
print("This is a static method.")
method_name = 'static_method'
if hasattr(MyClass, method_name):
method = getattr(MyClass, method_name)
method()
在上面的代码中,我们首先检查类MyClass
是否具有名为static_method
的方法,如果有,则使用getattr
获取该方法并调用它。
五、通过函数指针调用方法
在Python中,方法也可以作为函数指针进行传递和调用。
1. 将对象方法作为函数指针传递
class MyClass:
def __init__(self, value):
self.value = value
def display_value(self):
print("The value is:", self.value)
def call_method(method):
method()
obj = MyClass(10)
call_method(obj.display_value)
在上面的代码中,我们将对象obj
的display_value
方法作为参数传递给call_method
函数,并在call_method
函数中调用该方法。
2. 将类方法作为函数指针传递
class MyClass:
@staticmethod
def static_method():
print("This is a static method.")
def call_method(method):
method()
call_method(MyClass.static_method)
在上面的代码中,我们将类MyClass
的static_method
方法作为参数传递给call_method
函数,并在call_method
函数中调用该方法。
六、通过装饰器调用方法
装饰器是一种用于修改或扩展函数功能的高级特性,可以在不修改原函数的情况下增强其功能。
1. 定义一个简单的装饰器
def my_decorator(func):
def wrapper(*args, kwargs):
print("Before calling the method.")
result = func(*args, kwargs)
print("After calling the method.")
return result
return wrapper
在上面的代码中,我们定义了一个名为my_decorator
的装饰器,它在调用原函数前后打印一些信息。
2. 使用装饰器装饰对象方法
class MyClass:
def __init__(self, value):
self.value = value
@my_decorator
def display_value(self):
print("The value is:", self.value)
obj = MyClass(10)
obj.display_value()
在上面的代码中,我们使用my_decorator
装饰了对象方法display_value
,调用该方法时会先执行装饰器中的逻辑。
3. 使用装饰器装饰类方法
class MyClass:
@staticmethod
@my_decorator
def static_method():
print("This is a static method.")
MyClass.static_method()
在上面的代码中,我们使用my_decorator
装饰了类方法static_method
,调用该方法时会先执行装饰器中的逻辑。
七、通过元类调用方法
元类是用于创建类的类,可以在类创建时动态修改类的行为。
1. 定义一个简单的元类
class MyMeta(type):
def __new__(cls, name, bases, dct):
dct['greet'] = lambda self: print("Hello from", name)
return super().__new__(cls, name, bases, dct)
在上面的代码中,我们定义了一个名为MyMeta
的元类,它在类创建时添加了一个名为greet
的方法。
2. 使用元类创建类并调用方法
class MyClass(metaclass=MyMeta):
pass
obj = MyClass()
obj.greet()
在上面的代码中,我们使用MyMeta
元类创建了一个名为MyClass
的类,并通过对象实例obj
调用了元类添加的greet
方法。
八、通过闭包调用方法
闭包是指在一个函数内部定义另一个函数,内部函数可以访问外部函数的变量。
1. 定义一个简单的闭包
def outer_function(value):
def inner_function():
print("The value is:", value)
return inner_function
在上面的代码中,我们定义了一个闭包outer_function
,它返回了一个内部函数inner_function
。
2. 调用闭包方法
closure = outer_function(10)
closure()
在上面的代码中,我们调用outer_function
并传入一个参数,获得一个闭包函数closure
,然后调用该闭包函数。
九、通过多态调用方法
多态是面向对象编程中的一个重要概念,它允许不同类的对象通过相同的接口调用方法。
1. 定义基类和子类
class Animal:
def speak(self):
raise NotImplementedError("Subclasses must implement this method")
class Dog(Animal):
def speak(self):
print("Woof!")
class Cat(Animal):
def speak(self):
print("Meow!")
在上面的代码中,我们定义了一个基类Animal
和两个子类Dog
和Cat
,子类实现了基类的speak
方法。
2. 使用多态调用方法
animals = [Dog(), Cat()]
for animal in animals:
animal.speak()
在上面的代码中,我们创建了一个包含不同动物对象的列表animals
,通过遍历列表调用每个动物对象的speak
方法,展示了多态的特性。
十、通过回调函数调用方法
回调函数是一种通过参数传递的函数,可以在某个时间点或事件发生时调用。
1. 定义一个简单的回调函数
def my_callback():
print("Callback function called.")
def perform_action(callback):
print("Performing action...")
callback()
在上面的代码中,我们定义了一个回调函数my_callback
和一个调用回调函数的函数perform_action
。
2. 传递回调函数并调用
perform_action(my_callback)
在上面的代码中,我们将回调函数my_callback
作为参数传递给perform_action
函数,并在perform_action
函数中调用该回调函数。
通过以上多种方式,我们可以在Python中灵活地调用方法名,根据具体需求选择适合的方式。无论是通过对象调用方法、类调用静态方法,还是通过内置函数、反射机制、装饰器、元类等高级特性,都能满足不同场景下的需求。希望本文能帮助您更好地理解和运用Python中的方法调用。
相关问答FAQs:
如何在Python中动态调用方法名?
在Python中,可以使用内置的getattr()
函数来动态调用对象的方法。首先,您需要一个对象和一个字符串形式的方法名。使用getattr()
可以获取方法,然后直接调用它。例如:
class MyClass:
def my_method(self):
return "Hello, World!"
obj = MyClass()
method_name = 'my_method'
result = getattr(obj, method_name)() # 调用方法
print(result) # 输出: Hello, World!
可以通过字符串调用类的方法吗?
是的,您可以通过字符串来调用类中的方法。只需确保您已经实例化了该类,并使用getattr()
函数来获取方法。确保方法名是准确的,并且该方法可以被调用。
在什么情况下需要动态调用方法名?
动态调用方法名在某些情况下非常有用,例如,当您需要基于用户输入或配置文件中的参数来选择要调用的方法时。这种灵活性使得代码更具可扩展性和可维护性。
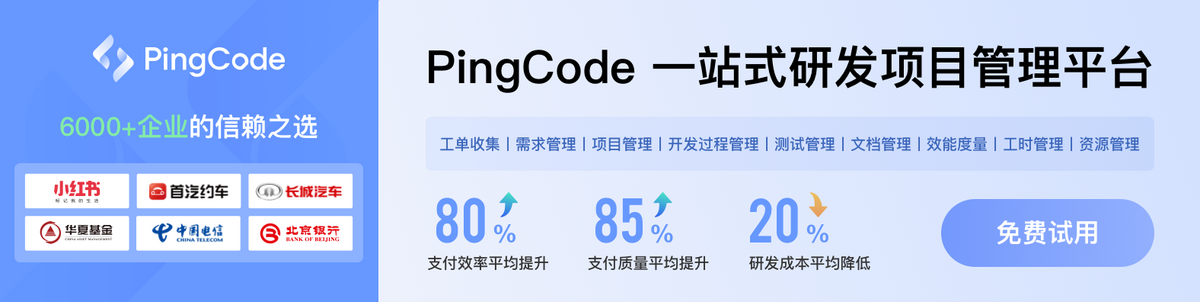