用Python写电影测评可以使用多种技术和工具,如数据抓取、自然语言处理、情感分析等,数据抓取、自然语言处理、情感分析、数据可视化是关键步骤。本文将详细介绍这些步骤中的每一个,并提供一些代码示例和专业见解,以帮助你更好地理解和实现电影测评。
一、数据抓取
数据抓取是电影测评的第一步。你需要从互联网上抓取电影的评论数据,通常这包括从IMDb、Rotten Tomatoes等网站抓取评论。为了实现这一目标,可以使用Python的requests
和BeautifulSoup
库。
1、使用Requests库抓取网页内容
首先,你需要安装requests
库。可以通过以下命令安装:
pip install requests
然后,使用requests
库抓取网页内容。以下是一个简单的示例:
import requests
url = 'https://www.imdb.com/title/tt0111161/reviews?ref_=tt_ql_3'
response = requests.get(url)
if response.status_code == 200:
print("Successfully fetched the webpage content!")
else:
print("Failed to fetch the webpage content.")
2、使用BeautifulSoup解析网页内容
接下来,你可以使用BeautifulSoup
解析网页内容。以下是一个示例:
from bs4 import BeautifulSoup
soup = BeautifulSoup(response.content, 'html.parser')
reviews = soup.find_all('div', class_='text show-more__control')
for review in reviews:
print(review.get_text())
二、自然语言处理
抓取到评论数据后,需要对数据进行预处理和分析。自然语言处理(NLP)技术可以帮助你清洗和分析文本数据。常用的NLP库有nltk
和spaCy
。
1、文本预处理
文本预处理是NLP的第一步,包括去除标点符号、转换为小写、去除停用词等。以下是一个示例:
import nltk
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
import string
nltk.download('punkt')
nltk.download('stopwords')
def preprocess_text(text):
# 转换为小写
text = text.lower()
# 去除标点符号
text = text.translate(str.maketrans('', '', string.punctuation))
# 分词
words = word_tokenize(text)
# 去除停用词
words = [word for word in words if word not in stopwords.words('english')]
return words
sample_review = "This movie is fantastic! The plot was gripping and the characters were well-developed."
processed_review = preprocess_text(sample_review)
print(processed_review)
2、情感分析
情感分析是电影测评的核心步骤之一。可以使用TextBlob
或VADER
等库进行情感分析。以下是使用TextBlob
进行情感分析的示例:
from textblob import TextBlob
def analyze_sentiment(text):
blob = TextBlob(text)
sentiment = blob.sentiment
return sentiment
sample_review = "This movie is fantastic! The plot was gripping and the characters were well-developed."
sentiment = analyze_sentiment(sample_review)
print(sentiment)
三、数据可视化
数据可视化可以帮助你更直观地展示电影测评的结果。常用的数据可视化库有matplotlib
和seaborn
。
1、使用Matplotlib进行数据可视化
以下是一个简单的示例,展示如何使用matplotlib
绘制情感分析结果的柱状图:
import matplotlib.pyplot as plt
reviews = ["This movie is fantastic!", "The plot was boring.", "Great cinematography but the story was lacking."]
sentiments = [analyze_sentiment(review).polarity for review in reviews]
plt.bar(range(len(reviews)), sentiments)
plt.xlabel('Review Index')
plt.ylabel('Sentiment Polarity')
plt.title('Sentiment Analysis of Movie Reviews')
plt.show()
2、使用Seaborn进行数据可视化
seaborn
是基于matplotlib
的高级数据可视化库,提供了更美观的图形。以下是一个示例:
import seaborn as sns
sns.barplot(x=list(range(len(reviews))), y=sentiments)
plt.xlabel('Review Index')
plt.ylabel('Sentiment Polarity')
plt.title('Sentiment Analysis of Movie Reviews')
plt.show()
四、综合示例
在这一部分,我们将综合使用上述技术,展示一个完整的电影测评示例。
1、抓取IMDb电影评论
首先,我们抓取IMDb某部电影的评论:
import requests
from bs4 import BeautifulSoup
url = 'https://www.imdb.com/title/tt0111161/reviews?ref_=tt_ql_3'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
reviews = soup.find_all('div', class_='text show-more__control')
2、预处理评论数据
接下来,我们对评论数据进行预处理:
import nltk
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
import string
nltk.download('punkt')
nltk.download('stopwords')
def preprocess_text(text):
text = text.lower()
text = text.translate(str.maketrans('', '', string.punctuation))
words = word_tokenize(text)
words = [word for word in words if word not in stopwords.words('english')]
return words
processed_reviews = [preprocess_text(review.get_text()) for review in reviews]
3、进行情感分析
然后,我们对预处理后的评论数据进行情感分析:
from textblob import TextBlob
def analyze_sentiment(text):
blob = TextBlob(text)
sentiment = blob.sentiment
return sentiment
sentiments = [analyze_sentiment(' '.join(review)).polarity for review in processed_reviews]
4、数据可视化
最后,我们使用seaborn
库对情感分析结果进行可视化:
import seaborn as sns
import matplotlib.pyplot as plt
sns.barplot(x=list(range(len(processed_reviews))), y=sentiments)
plt.xlabel('Review Index')
plt.ylabel('Sentiment Polarity')
plt.title('Sentiment Analysis of IMDb Movie Reviews')
plt.show()
五、更多高级技术
除了上述基本技术,还有一些高级技术可以用来提升电影测评的质量和效果。
1、使用机器学习进行情感分析
可以使用机器学习算法进行更高级的情感分析。以下是一个使用scikit-learn
进行情感分析的示例:
from sklearn.feature_extraction.text import CountVectorizer
from sklearn.model_selection import train_test_split
from sklearn.naive_bayes import MultinomialNB
from sklearn.metrics import accuracy_score
准备数据
reviews = ["This movie is fantastic!", "The plot was boring.", "Great cinematography but the story was lacking."]
labels = [1, 0, 0] # 1表示正面评论,0表示负面评论
特征提取
vectorizer = CountVectorizer()
X = vectorizer.fit_transform(reviews)
划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, labels, test_size=0.2, random_state=42)
训练模型
model = MultinomialNB()
model.fit(X_train, y_train)
预测
y_pred = model.predict(X_test)
评估模型
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy * 100:.2f}%")
2、使用深度学习进行情感分析
深度学习特别适合处理大规模的文本数据,可以使用TensorFlow
或PyTorch
等框架进行情感分析。以下是一个简单的示例,使用TensorFlow
进行情感分析:
import tensorflow as tf
from tensorflow.keras.preprocessing.text import Tokenizer
from tensorflow.keras.preprocessing.sequence import pad_sequences
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Embedding, LSTM, Dense
准备数据
reviews = ["This movie is fantastic!", "The plot was boring.", "Great cinematography but the story was lacking."]
labels = [1, 0, 0]
文本预处理
tokenizer = Tokenizer(num_words=10000)
tokenizer.fit_on_texts(reviews)
sequences = tokenizer.texts_to_sequences(reviews)
padded_sequences = pad_sequences(sequences, maxlen=100)
构建模型
model = Sequential([
Embedding(input_dim=10000, output_dim=128, input_length=100),
LSTM(64),
Dense(1, activation='sigmoid')
])
编译模型
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
训练模型
model.fit(padded_sequences, labels, epochs=10, batch_size=2)
预测
predictions = model.predict(padded_sequences)
print(predictions)
六、总结
用Python写电影测评涉及多个步骤,包括数据抓取、自然语言处理、情感分析和数据可视化。数据抓取、自然语言处理、情感分析、数据可视化是关键步骤。通过综合使用这些技术,可以实现一个完整的电影测评系统。高级技术如机器学习和深度学习可以进一步提升测评的质量和效果。希望本文提供的示例和见解能帮助你更好地实现电影测评。
相关问答FAQs:
如何开始用Python编写电影测评程序?
要开始编写电影测评程序,可以从选择一个合适的开发环境开始,例如Jupyter Notebook或任何Python IDE。接下来,您可以使用Python的标准库或第三方库(如BeautifulSoup和Requests)来抓取电影信息。如果您希望让用户提交评价,可以考虑使用Flask或Django等框架来创建一个简单的Web应用程序。
有哪些Python库适合处理电影数据?
处理电影数据时,有几个非常有用的Python库。Pandas可以帮助您轻松管理和分析数据,而NumPy则提供了强大的数学功能。此外,BeautifulSoup和Scrapy是用于网络抓取的好工具,允许您从网站提取电影信息和用户评论。对于数据可视化,Matplotlib和Seaborn是非常不错的选择。
如何提高电影测评的准确性和可信度?
为了提高电影测评的准确性和可信度,可以考虑引入多种评分机制,例如让用户根据多个维度(如剧情、表演、导演等)进行评分。此外,收集用户评论并进行情感分析可以帮助了解整体观众的反应。使用机器学习模型对用户的评分进行预测和分析,也可以提升测评的全面性和深度。
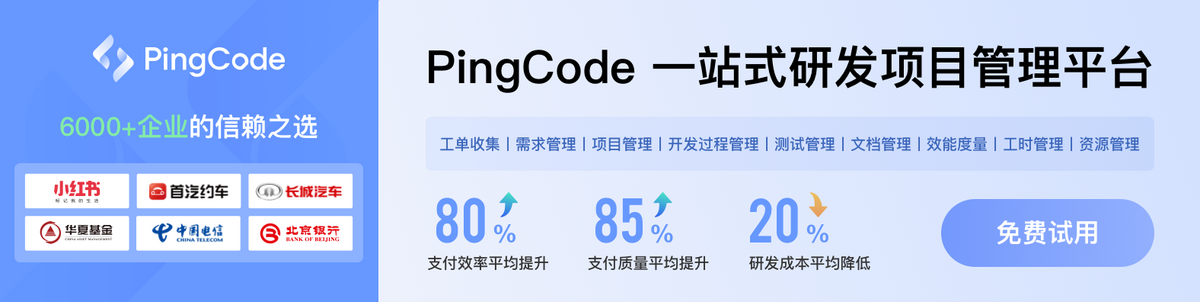