Python中可以通过多种方式使系统暂停读写操作。使用time.sleep()函数、os.system()调用系统命令、threading模块中的Event对象。其中,time.sleep() 是最简单和常用的方法,可以通过设定暂停的时间来使系统在指定时间内停止读写操作。让我们详细描述一下如何使用 time.sleep() 使系统暂停。
time.sleep() 是Python内置的time模块中的一个函数,它可以使当前线程暂停执行指定的秒数。这个函数接收一个浮点数作为参数,表示暂停的时间长度(以秒为单位)。例如,调用 time.sleep(5)
将使系统暂停5秒。这个方法适用于简单的暂停需求,不需要导入额外的模块,非常方便。
一、使用time.sleep()函数
基本使用方法
time.sleep(seconds)
函数接收一个参数 seconds,表示暂停的秒数。这个参数可以是整数,也可以是浮点数,以便表示更精确的时间。例如:
import time
print("Start")
time.sleep(2) # 暂停2秒
print("End")
在这个例子中,程序在打印“Start”后暂停2秒,然后继续执行并打印“End”。
在文件读写中的应用
我们可以使用 time.sleep()
在文件读写操作之间插入暂停。例如:
import time
with open('example.txt', 'w') as f:
f.write('First line\n')
time.sleep(2) # 暂停2秒
f.write('Second line\n')
这个例子在写入第一行后暂停2秒,再写入第二行。
结合其他操作使用
time.sleep()
也可以结合其他操作使用,如网络请求、数据处理等。例如:
import time
import requests
url = 'https://example.com'
response = requests.get(url)
print(response.status_code)
time.sleep(3) # 暂停3秒
print("Request completed")
这个例子在发送网络请求后暂停3秒,然后继续执行。
二、使用os.system()调用系统命令
基本使用方法
os.system(command)
函数可以在Python中调用系统命令。我们可以使用这个函数调用系统的暂停命令。例如:
import os
print("Start")
os.system("pause") # 暂停,等待用户输入
print("End")
在这个例子中,程序在打印“Start”后暂停,等待用户输入任意键,然后继续执行并打印“End”。
在文件读写中的应用
我们也可以使用 os.system()
在文件读写操作之间插入暂停。例如:
import os
with open('example.txt', 'w') as f:
f.write('First line\n')
os.system("pause") # 暂停,等待用户输入
f.write('Second line\n')
这个例子在写入第一行后暂停,等待用户输入任意键,再写入第二行。
结合其他操作使用
os.system()
也可以结合其他操作使用,如网络请求、数据处理等。例如:
import os
import requests
url = 'https://example.com'
response = requests.get(url)
print(response.status_code)
os.system("pause") # 暂停,等待用户输入
print("Request completed")
这个例子在发送网络请求后暂停,等待用户输入任意键,然后继续执行。
三、使用threading模块中的Event对象
基本使用方法
threading.Event
对象提供了一种更灵活的方式来控制线程的暂停和恢复。我们可以使用 Event.wait(timeout)
来暂停线程,直到事件被设置或超时。例如:
import threading
event = threading.Event()
def worker():
print("Start")
event.wait(2) # 暂停2秒
print("End")
thread = threading.Thread(target=worker)
thread.start()
在这个例子中,线程在打印“Start”后暂停2秒,然后继续执行并打印“End”。
在文件读写中的应用
我们也可以使用 Event.wait()
在文件读写操作之间插入暂停。例如:
import threading
event = threading.Event()
def write_file():
with open('example.txt', 'w') as f:
f.write('First line\n')
event.wait(2) # 暂停2秒
f.write('Second line\n')
thread = threading.Thread(target=write_file)
thread.start()
这个例子在写入第一行后暂停2秒,再写入第二行。
结合其他操作使用
Event.wait()
也可以结合其他操作使用,如网络请求、数据处理等。例如:
import threading
import requests
event = threading.Event()
def request():
url = 'https://example.com'
response = requests.get(url)
print(response.status_code)
event.wait(3) # 暂停3秒
print("Request completed")
thread = threading.Thread(target=request)
thread.start()
这个例子在发送网络请求后暂停3秒,然后继续执行。
四、使用其他Python库
除了上述三种方法外,还有一些Python库提供了更高级的暂停功能。例如,sched
模块和 asyncio
模块可以用于更复杂的调度和异步编程。
使用sched模块
sched
模块提供了一个调度程序,可以用于定时执行任务。例如:
import sched
import time
scheduler = sched.scheduler(time.time, time.sleep)
def print_event(name):
print(f"Event: {name}")
scheduler.enter(2, 1, print_event, ('first',))
scheduler.enter(4, 1, print_event, ('second',))
print("Start")
scheduler.run()
print("End")
在这个例子中,程序在启动后调度两个事件,第一个事件在2秒后执行,第二个事件在4秒后执行。
使用asyncio模块
asyncio
模块提供了用于异步编程的工具,可以用于异步地暂停和恢复。例如:
import asyncio
async def main():
print("Start")
await asyncio.sleep(2) # 异步暂停2秒
print("End")
asyncio.run(main())
在这个例子中,程序在打印“Start”后异步暂停2秒,然后继续执行并打印“End”。
五、具体应用场景
文件备份和恢复
在文件备份和恢复过程中,可能需要在操作之间插入暂停。例如:
import time
import shutil
def backup_file(src, dest):
shutil.copy(src, dest)
print("Backup completed")
time.sleep(5) # 暂停5秒
def restore_file(src, dest):
shutil.copy(src, dest)
print("Restore completed")
src_file = 'data.txt'
backup_file = 'backup.txt'
backup_file(src_file, backup_file)
restore_file(backup_file, src_file)
在这个例子中,程序在备份文件后暂停5秒,然后恢复文件。
数据处理和分析
在数据处理和分析过程中,可能需要在步骤之间插入暂停。例如:
import time
import pandas as pd
def load_data(file):
data = pd.read_csv(file)
print("Data loaded")
return data
def process_data(data):
# 数据处理逻辑
print("Data processed")
time.sleep(3) # 暂停3秒
def analyze_data(data):
# 数据分析逻辑
print("Data analyzed")
file = 'data.csv'
data = load_data(file)
process_data(data)
analyze_data(data)
在这个例子中,程序在数据处理后暂停3秒,然后进行数据分析。
网络爬虫和数据采集
在网络爬虫和数据采集过程中,可能需要在请求之间插入暂停,以避免过于频繁的请求。例如:
import time
import requests
def fetch_page(url):
response = requests.get(url)
print(f"Fetched {url} with status {response.status_code}")
return response
urls = ['https://example.com/page1', 'https://example.com/page2', 'https://example.com/page3']
for url in urls:
fetch_page(url)
time.sleep(2) # 每次请求后暂停2秒
在这个例子中,程序在每次请求后暂停2秒,然后继续请求下一个页面。
流程控制和自动化
在流程控制和自动化任务中,可能需要在步骤之间插入暂停。例如:
import time
def step_one():
print("Step one completed")
time.sleep(1) # 暂停1秒
def step_two():
print("Step two completed")
time.sleep(2) # 暂停2秒
def step_three():
print("Step three completed")
time.sleep(3) # 暂停3秒
step_one()
step_two()
step_three()
在这个例子中,程序在每个步骤后插入不同的暂停时间。
六、总结
通过上述内容,我们了解了Python中使系统暂停读写操作的多种方法,包括 使用time.sleep()函数、os.system()调用系统命令、threading模块中的Event对象、使用sched模块和asyncio模块。每种方法都有其适用的场景和优点,可以根据具体需求选择合适的方法。
time.sleep() 适用于简单的暂停需求,使用方便,不需要导入额外的模块。os.system() 可以调用系统命令,适用于需要等待用户输入的场景。threading.Event 提供了更灵活的暂停和恢复控制,适用于多线程编程。sched 和 asyncio 模块适用于更复杂的调度和异步编程需求。
在实际应用中,可以根据具体需求选择合适的方法,并结合文件备份和恢复、数据处理和分析、网络爬虫和数据采集、流程控制和自动化等场景,合理地插入暂停操作,以保证程序的稳定性和可靠性。
相关问答FAQs:
如何在Python中实现系统暂停以进行读写操作?
在Python中,可以使用内置的time
模块中的sleep()
函数来让程序暂停一段时间。这可以有效地控制读写操作的时机。例如,如果你需要在读写文件之前暂停,可以在文件操作之前调用sleep(seconds)
,其中seconds
是暂停的时间长度。
在Python中暂停的最佳实践是什么?
为了确保程序的高效性,建议在进行长时间的读写操作时,结合使用异步编程或多线程来管理系统资源。使用asyncio
库可以让你在处理文件读写时保持系统的响应能力。此外,设置合理的暂停时间可以避免过于频繁的读写操作,减少系统负担。
如何使用Python中的信号处理来控制程序的暂停?
Python的signal
模块允许程序在接收到特定信号时暂停或执行其他操作。通过定义一个信号处理函数,并在程序中注册该函数,可以实现对系统暂停的更精细控制。这对于需要响应外部事件或用户输入的情况非常有用,能够在合适的时机中断或暂停当前的读写操作。
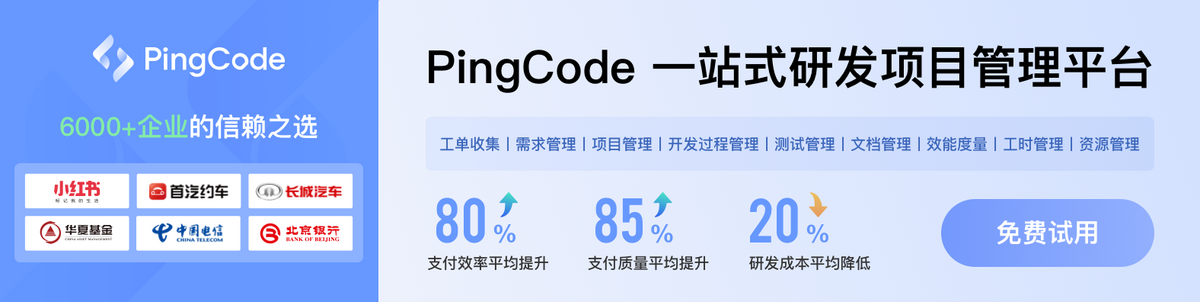