要在Python中为闹钟添加颜色,可以使用诸如tkinter
库来创建图形用户界面(GUI)并添加颜色、使用pygame
库进行更高级的图形处理、或使用控制台颜色库如colorama
为命令行界面添加颜色。在本文中,我们将深入探讨这三种方法,并结合实例代码和详细说明,帮助您在Python项目中为闹钟添加颜色。
一、使用 tkinter
库创建有颜色的闹钟
tkinter
是Python的标准GUI库,可以用来创建图形化的应用程序。它简单易用,适合初学者。
1、创建基本的 tkinter
窗口
首先,我们需要安装tkinter
库(大多数Python安装包已经包含了这个库)。接下来我们来创建一个基本的窗口。
import tkinter as tk
def create_window():
window = tk.Tk()
window.title("Alarm Clock")
window.geometry("300x200")
window.configure(bg="lightblue")
window.mainloop()
create_window()
2、添加标签和按钮并设置颜色
在基本窗口的基础上,我们可以添加标签、输入框和按钮,并设置它们的颜色。
import tkinter as tk
def create_window():
window = tk.Tk()
window.title("Alarm Clock")
window.geometry("300x200")
window.configure(bg="lightblue")
label = tk.Label(window, text="Set Alarm Time", bg="lightblue", fg="darkblue", font=("Helvetica", 16))
label.pack(pady=10)
time_entry = tk.Entry(window, font=("Helvetica", 16))
time_entry.pack(pady=10)
set_button = tk.Button(window, text="Set Alarm", bg="darkblue", fg="white", font=("Helvetica", 16), command=lambda: set_alarm(time_entry.get()))
set_button.pack(pady=10)
window.mainloop()
def set_alarm(time):
print(f"Alarm set for {time}")
create_window()
3、实现闹钟功能
我们可以进一步实现闹钟功能,让它在设定的时间响铃,并且在界面上显示当前时间。
import tkinter as tk
from datetime import datetime
import time
import threading
def create_window():
window = tk.Tk()
window.title("Alarm Clock")
window.geometry("300x200")
window.configure(bg="lightblue")
label = tk.Label(window, text="Set Alarm Time (HH:MM)", bg="lightblue", fg="darkblue", font=("Helvetica", 16))
label.pack(pady=10)
time_entry = tk.Entry(window, font=("Helvetica", 16))
time_entry.pack(pady=10)
set_button = tk.Button(window, text="Set Alarm", bg="darkblue", fg="white", font=("Helvetica", 16), command=lambda: set_alarm(time_entry.get()))
set_button.pack(pady=10)
current_time_label = tk.Label(window, text="", bg="lightblue", fg="darkblue", font=("Helvetica", 16))
current_time_label.pack(pady=10)
def update_time():
while True:
current_time = datetime.now().strftime("%H:%M:%S")
current_time_label.config(text=current_time)
time.sleep(1)
threading.Thread(target=update_time).start()
window.mainloop()
def set_alarm(alarm_time):
alarm_hour, alarm_minute = map(int, alarm_time.split(":"))
while True:
now = datetime.now()
if now.hour == alarm_hour and now.minute == alarm_minute:
print("Alarm ringing!")
break
time.sleep(1)
create_window()
在以上代码中,我们添加了一个标签来显示当前时间,并使用多线程来不断更新这个时间标签。
二、使用 pygame
库创建有颜色的闹钟
pygame
是一个跨平台的Python模块,专门为编写视频游戏设计。它包含计算机图形和声音库,可以用来创建更高级的闹钟应用。
1、安装并初始化 pygame
首先,我们需要安装pygame
库:
pip install pygame
接下来,我们初始化pygame
并创建一个基本窗口。
import pygame
import time
import threading
pygame.init()
def create_window():
screen = pygame.display.set_mode((300, 200))
pygame.display.set_caption("Alarm Clock")
screen.fill((173, 216, 230)) # 设置背景颜色为lightblue
font = pygame.font.Font(None, 36)
text = font.render('Set Alarm Time (HH:MM)', True, (0, 0, 139)) # darkblue
screen.blit(text, (20, 20))
pygame.display.flip()
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
pygame.quit()
create_window()
2、添加输入和按钮并设置颜色
我们可以进一步添加文本输入和按钮,并设置它们的颜色。
import pygame
import time
import threading
pygame.init()
def create_window():
screen = pygame.display.set_mode((300, 200))
pygame.display.set_caption("Alarm Clock")
screen.fill((173, 216, 230)) # 设置背景颜色为lightblue
font = pygame.font.Font(None, 36)
text = font.render('Set Alarm Time (HH:MM)', True, (0, 0, 139)) # darkblue
screen.blit(text, (20, 20))
input_box = pygame.Rect(20, 70, 140, 32)
color_inactive = pygame.Color('lightskyblue3')
color_active = pygame.Color('dodgerblue2')
color = color_inactive
active = False
text = ''
done = False
while not done:
for event in pygame.event.get():
if event.type == pygame.QUIT:
done = True
if event.type == pygame.MOUSEBUTTONDOWN:
if input_box.collidepoint(event.pos):
active = not active
else:
active = False
color = color_active if active else color_inactive
if event.type == pygame.KEYDOWN:
if active:
if event.key == pygame.K_RETURN:
print(text)
text = ''
elif event.key == pygame.K_BACKSPACE:
text = text[:-1]
else:
text += event.unicode
screen.fill((173, 216, 230)) # lightblue
screen.blit(font.render('Set Alarm Time (HH:MM)', True, (0, 0, 139)), (20, 20))
txt_surface = font.render(text, True, color)
width = max(200, txt_surface.get_width()+10)
input_box.w = width
screen.blit(txt_surface, (input_box.x+5, input_box.y+5))
pygame.draw.rect(screen, color, input_box, 2)
pygame.display.flip()
pygame.quit()
create_window()
3、实现闹钟功能
最后,我们实现闹钟功能,让它在设定的时间响铃。
import pygame
import time
import threading
from datetime import datetime
pygame.init()
def create_window():
screen = pygame.display.set_mode((300, 200))
pygame.display.set_caption("Alarm Clock")
screen.fill((173, 216, 230)) # lightblue
font = pygame.font.Font(None, 36)
text = font.render('Set Alarm Time (HH:MM)', True, (0, 0, 139)) # darkblue
screen.blit(text, (20, 20))
input_box = pygame.Rect(20, 70, 140, 32)
color_inactive = pygame.Color('lightskyblue3')
color_active = pygame.Color('dodgerblue2')
color = color_inactive
active = False
text = ''
done = False
while not done:
for event in pygame.event.get():
if event.type == pygame.QUIT:
done = True
if event.type == pygame.MOUSEBUTTONDOWN:
if input_box.collidepoint(event.pos):
active = not active
else:
active = False
color = color_active if active else color_inactive
if event.type == pygame.KEYDOWN:
if active:
if event.key == pygame.K_RETURN:
threading.Thread(target=set_alarm, args=(text,)).start()
text = ''
elif event.key == pygame.K_BACKSPACE:
text = text[:-1]
else:
text += event.unicode
screen.fill((173, 216, 230)) # lightblue
screen.blit(font.render('Set Alarm Time (HH:MM)', True, (0, 0, 139)), (20, 20))
txt_surface = font.render(text, True, color)
width = max(200, txt_surface.get_width()+10)
input_box.w = width
screen.blit(txt_surface, (input_box.x+5, input_box.y+5))
pygame.draw.rect(screen, color, input_box, 2)
pygame.display.flip()
pygame.quit()
def set_alarm(alarm_time):
alarm_hour, alarm_minute = map(int, alarm_time.split(":"))
while True:
now = datetime.now()
if now.hour == alarm_hour and now.minute == alarm_minute:
print("Alarm ringing!")
break
time.sleep(1)
create_window()
在以上代码中,我们实现了一个简单的闹钟功能,它会在设定的时间响铃。
三、使用 colorama
库在控制台添加颜色
如果您只是想在控制台程序中添加颜色,可以使用colorama
库。colorama
可以让您在控制台输出彩色文本。
1、安装并初始化 colorama
首先,安装colorama
库:
pip install colorama
接下来,我们初始化colorama
并创建一个简单的闹钟程序。
import time
from datetime import datetime
from colorama import init, Fore, Back, Style
init()
def set_alarm(alarm_time):
alarm_hour, alarm_minute = map(int, alarm_time.split(":"))
while True:
now = datetime.now()
if now.hour == alarm_hour and now.minute == alarm_minute:
print(Fore.RED + "Alarm ringing!" + Style.RESET_ALL)
break
time.sleep(1)
def main():
print(Fore.BLUE + "Set Alarm Time (HH:MM)" + Style.RESET_ALL)
alarm_time = input(Fore.GREEN + "Enter time: " + Style.RESET_ALL)
set_alarm(alarm_time)
main()
2、添加更多颜色效果
我们可以进一步添加更多颜色效果,使控制台输出更加丰富多彩。
import time
from datetime import datetime
from colorama import init, Fore, Back, Style
init()
def set_alarm(alarm_time):
alarm_hour, alarm_minute = map(int, alarm_time.split(":"))
while True:
now = datetime.now()
if now.hour == alarm_hour and now.minute == alarm_minute:
print(Fore.RED + Back.YELLOW + "Alarm ringing!" + Style.RESET_ALL)
break
time.sleep(1)
def main():
print(Fore.BLUE + "Set Alarm Time (HH:MM)" + Style.RESET_ALL)
alarm_time = input(Fore.GREEN + "Enter time: " + Style.RESET_ALL)
set_alarm(alarm_time)
main()
在以上代码中,我们使用colorama
库为控制台输出添加了不同的颜色和背景颜色效果。
总结
本文介绍了三种在Python中为闹钟添加颜色的方法:使用tkinter
库创建图形用户界面、使用pygame
库进行更高级的图形处理、使用colorama
库为控制台输出添加颜色。每种方法都有其独特的优势和适用场景,您可以根据实际需求选择合适的方法。在实际开发中,灵活运用这些库,可以大大增强Python应用程序的用户体验。
相关问答FAQs:
如何在Python写的闹钟中添加不同的颜色?
在Python中,您可以使用colorama
库或tkinter
库来为闹钟添加颜色效果。colorama
库可以在终端中实现文本颜色,而tkinter
则允许您创建图形用户界面(GUI),并为按钮和背景设置颜色。安装colorama
只需使用pip install colorama
。如果选择tkinter
,则无需额外安装,因为它通常是Python的标准库之一。
使用Python闹钟时,如何实现彩色提醒?
您可以在设置闹钟时,使用不同的颜色来表示不同的提醒。例如,当闹钟响起时,可以通过改变文本颜色或背景颜色来引起注意。使用colorama
库时,可以通过调用相关的颜色代码来设置文本颜色;如果使用tkinter
,则可以更改按钮或标签的背景颜色。
我如何能让我的Python闹钟在特定时间以颜色闪烁?
要实现颜色闪烁效果,可以使用tkinter
库中的after()
方法来定期改变颜色。例如,您可以在闹钟时间到达时,设置一个循环,每隔一定时间改变背景颜色。这样,用户就能更明显地感知到闹钟正在响起,并且可以通过视觉效果引起他们的注意。
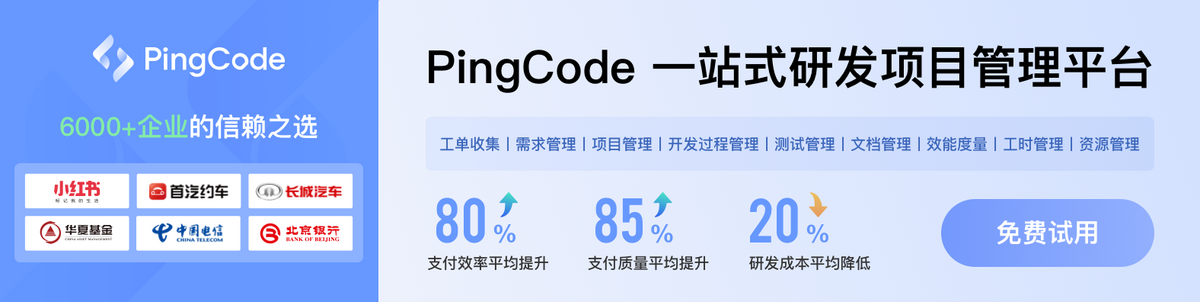