在Python中,新建文档可以通过多种方式实现,例如使用内置的open
函数、第三方库如pathlib
、os
模块等。最常用的方法包括使用open
函数、pathlib
模块、os
模块。这里我们详细介绍一下使用open
函数来新建文档的方法。
使用open
函数新建文档时,可以指定文件模式为写模式('w')或追加模式('a')。在写模式下,如果文件不存在,会自动创建一个新文件;如果文件已经存在,则会清空文件内容。而在追加模式下,如果文件存在,会在文件末尾追加内容;如果文件不存在,也会创建一个新文件。
# 使用open函数新建文档
file_path = 'example.txt'
with open(file_path, 'w') as file:
file.write("This is a new file created using open function.\n")
在上面的代码中,open
函数用来打开或创建一个文件,'w'
表示写模式。with
语句确保文件在操作完成后自动关闭。下面我们详细介绍这种方法,并探讨其他新建文档的方法。
一、使用open函数新建文档
1、基本用法
在Python中,open
函数是用来打开文件的最基本方法之一。它不仅可以读取文件,还可以创建和写入文件。open
函数的第一个参数是文件路径,第二个参数是文件模式。以下是一些常见的文件模式:
'r'
:读取模式(默认)。'w'
:写入模式。如果文件存在,会清空文件内容。'a'
:追加模式。如果文件存在,会在文件末尾追加内容。'x'
:创建模式。如果文件存在,会引发FileExistsError异常。
# 创建并写入一个新文件
file_path = 'example.txt'
with open(file_path, 'w') as file:
file.write("This is a new file created using open function.\n")
在上面的代码中,'w'
模式用于写入文件。如果文件不存在,open
函数将创建一个新文件。如果文件已经存在,open
函数会清空文件内容并重新写入新内容。
2、追加模式
有时,我们不希望清空文件内容,而是希望在文件末尾追加内容。这时可以使用追加模式('a'
)。
# 追加内容到文件末尾
file_path = 'example.txt'
with open(file_path, 'a') as file:
file.write("This line is appended to the file.\n")
在上面的代码中,'a'
模式用于追加内容。如果文件不存在,open
函数将创建一个新文件。如果文件已经存在,open
函数会在文件末尾追加新内容。
3、读写模式
有时,我们希望在同一个文件中同时进行读写操作。这时可以使用读写模式('r+'
, 'w+'
, 'a+'
)。
# 读写模式示例
file_path = 'example.txt'
with open(file_path, 'r+') as file:
content = file.read()
file.write("This line is written in read-write mode.\n")
在上面的代码中,'r+'
模式用于读写操作。需要注意的是,文件指针的位置会影响写入内容的位置。
二、使用pathlib模块新建文档
1、基本用法
pathlib
模块是Python 3.4引入的一个模块,用于处理文件和目录路径。它提供了一种面向对象的方法来处理文件系统路径。
from pathlib import Path
创建一个新的文本文件
file_path = Path('example.txt')
file_path.write_text("This is a new file created using pathlib module.\n")
在上面的代码中,Path
对象表示文件路径。write_text
方法用于写入文本到文件。如果文件不存在,write_text
方法将创建一个新文件。如果文件已经存在,write_text
方法会覆盖文件内容。
2、追加内容
pathlib
模块没有直接提供追加内容的方法,但可以通过标准的文件操作来实现。
from pathlib import Path
追加内容到文件末尾
file_path = Path('example.txt')
with file_path.open('a') as file:
file.write("This line is appended to the file using pathlib.\n")
在上面的代码中,open
方法用于以追加模式打开文件,然后使用write
方法追加内容。
3、创建目录和文件
pathlib
模块还可以用于创建目录和文件。
from pathlib import Path
创建目录和文件
directory_path = Path('example_dir')
directory_path.mkdir(exist_ok=True)
file_path = directory_path / 'example.txt'
file_path.write_text("This is a new file created in a new directory.\n")
在上面的代码中,mkdir
方法用于创建目录。exist_ok=True
参数表示如果目录已经存在,不会引发异常。然后使用/
运算符组合路径并创建文件。
三、使用os模块新建文档
1、基本用法
os
模块提供了一些与操作系统交互的函数,可以用于创建和删除文件和目录。
import os
创建一个新的文本文件
file_path = 'example.txt'
with open(file_path, 'w') as file:
file.write("This is a new file created using os module.\n")
在上面的代码中,虽然使用了os
模块,但实际创建文件仍然使用了open
函数。os
模块更常用于文件和目录的管理。
2、创建目录和文件
os
模块可以用于创建目录,然后在目录中创建文件。
import os
创建目录
directory_path = 'example_dir'
os.makedirs(directory_path, exist_ok=True)
创建文件
file_path = os.path.join(directory_path, 'example.txt')
with open(file_path, 'w') as file:
file.write("This is a new file created in a new directory using os module.\n")
在上面的代码中,makedirs
方法用于创建目录。exist_ok=True
参数表示如果目录已经存在,不会引发异常。os.path.join
方法用于组合路径。
3、检查文件是否存在
os
模块还可以用于检查文件是否存在,然后决定是否创建新文件。
import os
file_path = 'example.txt'
if not os.path.exists(file_path):
with open(file_path, 'w') as file:
file.write("This is a new file created because it did not exist.\n")
在上面的代码中,os.path.exists
方法用于检查文件是否存在。如果文件不存在,open
函数会创建一个新文件。
四、使用shutil模块新建文档
1、基本用法
shutil
模块主要用于高级的文件操作,如复制和移动文件,但也可以用于创建文件。
import shutil
创建一个新的文本文件
file_path = 'example.txt'
with open(file_path, 'w') as file:
file.write("This is a new file created using shutil module.\n")
在上面的代码中,虽然使用了shutil
模块,但实际创建文件仍然使用了open
函数。shutil
模块更常用于文件的复制和移动。
2、复制文件
shutil
模块可以用于复制文件。
import shutil
创建源文件
source_file_path = 'example.txt'
with open(source_file_path, 'w') as file:
file.write("This is the source file.\n")
复制文件
destination_file_path = 'example_copy.txt'
shutil.copy(source_file_path, destination_file_path)
在上面的代码中,copy
方法用于复制文件。第一个参数是源文件路径,第二个参数是目标文件路径。
3、移动文件
shutil
模块还可以用于移动文件。
import shutil
创建源文件
source_file_path = 'example.txt'
with open(source_file_path, 'w') as file:
file.write("This is the source file.\n")
移动文件
destination_file_path = 'example_dir/example.txt'
shutil.move(source_file_path, destination_file_path)
在上面的代码中,move
方法用于移动文件。第一个参数是源文件路径,第二个参数是目标文件路径。
五、使用tempfile模块新建临时文档
1、基本用法
tempfile
模块提供了一些用于创建临时文件和目录的函数。临时文件在关闭后会自动删除,非常适合用于临时数据存储。
import tempfile
创建一个临时文件
with tempfile.NamedTemporaryFile(delete=False) as temp_file:
temp_file.write(b"This is a temporary file created using tempfile module.\n")
temp_file_path = temp_file.name
print(f'Temporary file created at: {temp_file_path}')
在上面的代码中,NamedTemporaryFile
方法用于创建一个临时文件。delete=False
参数表示文件关闭后不会自动删除。
2、临时目录
tempfile
模块还可以用于创建临时目录。
import tempfile
import os
创建一个临时目录
with tempfile.TemporaryDirectory() as temp_dir:
print(f'Temporary directory created at: {temp_dir}')
temp_file_path = os.path.join(temp_dir, 'example.txt')
with open(temp_file_path, 'w') as temp_file:
temp_file.write("This is a file in a temporary directory.\n")
在上面的代码中,TemporaryDirectory
方法用于创建一个临时目录。目录和其中的文件在退出with
语句块后会自动删除。
六、使用第三方库新建文档
1、pandas库
pandas
库是一个强大的数据处理库,可以用于创建和操作CSV文件。
import pandas as pd
创建一个DataFrame
data = {'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35]}
df = pd.DataFrame(data)
保存DataFrame到CSV文件
file_path = 'example.csv'
df.to_csv(file_path, index=False)
在上面的代码中,to_csv
方法用于将DataFrame
保存到CSV文件。如果文件不存在,to_csv
方法将创建一个新文件。
2、openpyxl库
openpyxl
库用于创建和操作Excel文件。
from openpyxl import Workbook
创建一个工作簿
wb = Workbook()
获取活动工作表
ws = wb.active
写入数据到工作表
ws['A1'] = 'Name'
ws['B1'] = 'Age'
ws.append(['Alice', 25])
ws.append(['Bob', 30])
ws.append(['Charlie', 35])
保存工作簿到Excel文件
file_path = 'example.xlsx'
wb.save(file_path)
在上面的代码中,save
方法用于将工作簿保存到Excel文件。如果文件不存在,save
方法将创建一个新文件。
3、docx库
python-docx
库用于创建和操作Word文件。
from docx import Document
创建一个文档
doc = Document()
添加段落到文档
doc.add_paragraph("This is a new document created using python-docx module.")
保存文档到Word文件
file_path = 'example.docx'
doc.save(file_path)
在上面的代码中,save
方法用于将文档保存到Word文件。如果文件不存在,save
方法将创建一个新文件。
七、总结
在Python中,新建文档的方法多种多样,可以根据具体需求选择合适的方法。最常用的方法包括使用open
函数、pathlib
模块、os
模块。每种方法都有其优点和适用场景。通过掌握这些方法,可以更灵活地处理文件操作任务。
相关问答FAQs:
如何在Python中创建新的文本文件?
在Python中,可以使用内置的open()
函数来创建新的文本文件。通过指定文件名和模式为'w'(写入模式),可以新建一个文件。如果文件已存在,内容将被覆盖。例如:
with open('newfile.txt', 'w') as file:
file.write('这是新创建的文档。')
这种方式确保文件在使用完后会自动关闭,避免资源泄露。
Python中可以创建哪些类型的文件?
Python不仅可以创建文本文件,还可以创建多种类型的文件,包括CSV、JSON、Excel等。通过使用不同的库,比如csv
、json
、pandas
等,可以轻松生成这些格式的文件。例如,使用csv
库可以创建CSV文件:
import csv
with open('data.csv', 'w', newline='') as csvfile:
writer = csv.writer(csvfile)
writer.writerow(['姓名', '年龄'])
writer.writerow(['Alice', 30])
新建文档时如何处理文件路径问题?
在创建文件时,指定文件路径是非常重要的。如果不提供完整路径,文件将会保存在当前工作目录中。可以使用绝对路径或相对路径来指定文件位置。例如:
with open('/path/to/directory/newfile.txt', 'w') as file:
file.write('文档内容')
确保所指定的路径存在,否则会引发错误。使用os
库可以帮助检查路径是否存在或创建必要的目录。
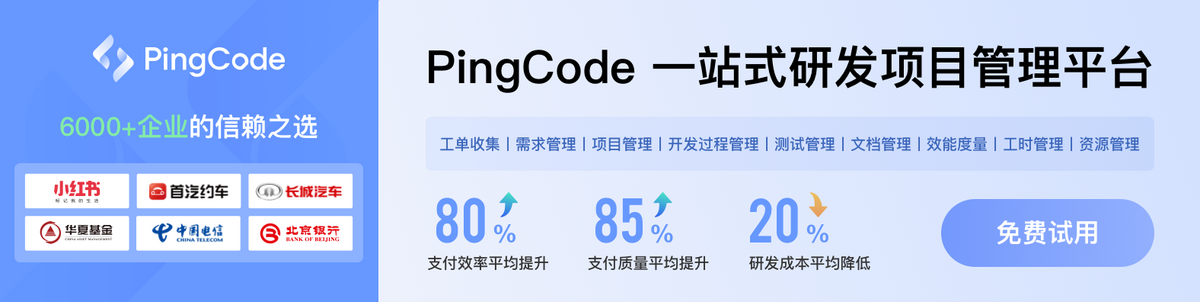