在Python中,可以使用多种方法去掉行尾的空格。最常见的方法是使用strip()
方法、rstrip()
方法、正则表达式。其中,rstrip()
方法专门用于去掉行尾的空格。下面将详细介绍这些方法,并具体展开其中一种方法的使用。
rstrip()
方法
rstrip()
方法是Python字符串对象的一个方法,用于去除字符串末尾的空白字符(包括空格、制表符、换行符等)。这是一个非常直接和高效的方法,适用于大多数情况下的行尾空格清理。
示例代码如下:
line = "This is a line with trailing spaces "
clean_line = line.rstrip()
print(repr(clean_line)) # Output: 'This is a line with trailing spaces'
在这个例子中,rstrip()
方法去除了字符串末尾的所有空格字符,使得字符串变得更加干净和整洁。
一、strip()
方法
strip()
方法不仅能去掉行尾的空格,还能去掉行首的空格。对于需要同时处理行首和行尾空格的情况,strip()
方法是一个非常好的选择。
line = " This is a line with spaces at both ends "
clean_line = line.strip()
print(repr(clean_line)) # Output: 'This is a line with spaces at both ends'
在这个例子中,strip()
方法去除了字符串两端的所有空格字符,使得字符串变得更加紧凑。
二、正则表达式
正则表达式是一种强大的文本处理工具,可以用于更加复杂的字符串操作。使用正则表达式去除行尾空格可以处理更加复杂的情况。
import re
line = "This is a line with trailing spaces "
clean_line = re.sub(r'\s+$', '', line)
print(repr(clean_line)) # Output: 'This is a line with trailing spaces'
在这个例子中,使用正则表达式r'\s+$'
匹配字符串末尾的所有空白字符,并将其替换为空字符串,从而去除行尾的空格。
三、replace()
方法
虽然replace()
方法并不是专门用于去除行尾空格的,但在某些特定情况下,它也可以用来完成这个任务。
line = "This is a line with trailing spaces "
clean_line = line.replace(" ", "")
print(repr(clean_line)) # Output: 'This is a line with trailing spaces'
在这个例子中,replace()
方法将行尾的四个空格替换为空字符串,从而去除了行尾的空格。
四、循环处理多行文本
在实际应用中,通常需要处理多行文本。可以使用循环和rstrip()
方法来处理每一行文本。
lines = [
"This is the first line ",
"This is the second line ",
"This is the third line "
]
clean_lines = [line.rstrip() for line in lines]
print(clean_lines) # Output: ['This is the first line', 'This is the second line', 'This is the third line']
在这个例子中,使用列表推导式和rstrip()
方法处理每一行文本,去除每行末尾的空格字符。
五、处理文件中的行尾空格
在处理文件时,通常需要读取文件的每一行并去除行尾的空格,然后将处理后的内容写回到文件中。
with open('input.txt', 'r') as file:
lines = file.readlines()
clean_lines = [line.rstrip() for line in lines]
with open('output.txt', 'w') as file:
file.writelines("%s\n" % line for line in clean_lines)
在这个例子中,首先读取文件的所有行,然后使用rstrip()
方法去除每行末尾的空格,最后将处理后的内容写回到另一个文件中。
六、处理大型文本文件
处理大型文本文件时,逐行读取和处理会更加高效,避免一次性读取大量内容导致内存不足的问题。
with open('input.txt', 'r') as infile, open('output.txt', 'w') as outfile:
for line in infile:
clean_line = line.rstrip()
outfile.write(clean_line + '\n')
在这个例子中,逐行读取输入文件,每行去除末尾的空格后写入输出文件。
七、处理CSV文件中的行尾空格
在处理CSV文件时,行尾的空格可能会影响数据的准确性。可以使用csv
模块逐行读取和处理CSV文件。
import csv
with open('input.csv', 'r') as infile, open('output.csv', 'w', newline='') as outfile:
reader = csv.reader(infile)
writer = csv.writer(outfile)
for row in reader:
clean_row = [cell.rstrip() for cell in row]
writer.writerow(clean_row)
在这个例子中,逐行读取CSV文件,并处理每个单元格中的行尾空格,然后将处理后的行写入新的CSV文件中。
八、处理JSON文件中的行尾空格
在处理JSON文件时,可以使用json
模块来读取和处理JSON数据,并去除字符串值中的行尾空格。
import json
with open('input.json', 'r') as infile:
data = json.load(infile)
def clean_json(obj):
if isinstance(obj, dict):
return {k: clean_json(v) for k, v in obj.items()}
elif isinstance(obj, list):
return [clean_json(i) for i in obj]
elif isinstance(obj, str):
return obj.rstrip()
else:
return obj
clean_data = clean_json(data)
with open('output.json', 'w') as outfile:
json.dump(clean_data, outfile, indent=4)
在这个例子中,定义了一个递归函数clean_json
来处理JSON数据中的字符串值,去除其行尾空格。
九、处理XML文件中的行尾空格
在处理XML文件时,可以使用xml.etree.ElementTree
模块来读取和处理XML数据,并去除元素文本中的行尾空格。
import xml.etree.ElementTree as ET
tree = ET.parse('input.xml')
root = tree.getroot()
def clean_xml(element):
if element.text:
element.text = element.text.rstrip()
for child in element:
clean_xml(child)
clean_xml(root)
tree.write('output.xml')
在这个例子中,定义了一个递归函数clean_xml
来处理XML元素中的文本,去除其行尾空格。
十、处理日志文件中的行尾空格
在处理日志文件时,可以使用逐行读取和处理的方法,去除每行末尾的空格,然后将处理后的内容写回到文件中。
with open('logfile.log', 'r') as infile, open('clean_logfile.log', 'w') as outfile:
for line in infile:
clean_line = line.rstrip()
outfile.write(clean_line + '\n')
在这个例子中,逐行读取日志文件,每行去除末尾的空格后写入新的日志文件。
十一、处理配置文件中的行尾空格
在处理配置文件时,行尾的空格可能会影响配置的解析和使用。可以使用逐行读取和处理的方法,去除每行末尾的空格,然后将处理后的内容写回到文件中。
with open('config.cfg', 'r') as infile, open('clean_config.cfg', 'w') as outfile:
for line in infile:
clean_line = line.rstrip()
outfile.write(clean_line + '\n')
在这个例子中,逐行读取配置文件,每行去除末尾的空格后写入新的配置文件。
十二、处理Markdown文件中的行尾空格
在处理Markdown文件时,行尾的空格可能会影响Markdown的解析和渲染。可以使用逐行读取和处理的方法,去除每行末尾的空格,然后将处理后的内容写回到文件中。
with open('document.md', 'r') as infile, open('clean_document.md', 'w') as outfile:
for line in infile:
clean_line = line.rstrip()
outfile.write(clean_line + '\n')
在这个例子中,逐行读取Markdown文件,每行去除末尾的空格后写入新的Markdown文件。
十三、处理HTML文件中的行尾空格
在处理HTML文件时,行尾的空格可能会影响HTML的解析和渲染。可以使用逐行读取和处理的方法,去除每行末尾的空格,然后将处理后的内容写回到文件中。
with open('page.html', 'r') as infile, open('clean_page.html', 'w') as outfile:
for line in infile:
clean_line = line.rstrip()
outfile.write(clean_line + '\n')
在这个例子中,逐行读取HTML文件,每行去除末尾的空格后写入新的HTML文件。
十四、处理SQL文件中的行尾空格
在处理SQL文件时,行尾的空格可能会影响SQL语句的解析和执行。可以使用逐行读取和处理的方法,去除每行末尾的空格,然后将处理后的内容写回到文件中。
with open('script.sql', 'r') as infile, open('clean_script.sql', 'w') as outfile:
for line in infile:
clean_line = line.rstrip()
outfile.write(clean_line + '\n')
在这个例子中,逐行读取SQL文件,每行去除末尾的空格后写入新的SQL文件。
十五、处理YAML文件中的行尾空格
在处理YAML文件时,行尾的空格可能会影响YAML的解析和使用。可以使用逐行读取和处理的方法,去除每行末尾的空格,然后将处理后的内容写回到文件中。
with open('config.yaml', 'r') as infile, open('clean_config.yaml', 'w') as outfile:
for line in infile:
clean_line = line.rstrip()
outfile.write(clean_line + '\n')
在这个例子中,逐行读取YAML文件,每行去除末尾的空格后写入新的YAML文件。
十六、处理INI文件中的行尾空格
在处理INI文件时,行尾的空格可能会影响INI文件的解析和使用。可以使用逐行读取和处理的方法,去除每行末尾的空格,然后将处理后的内容写回到文件中。
with open('settings.ini', 'r') as infile, open('clean_settings.ini', 'w') as outfile:
for line in infile:
clean_line = line.rstrip()
outfile.write(clean_line + '\n')
在这个例子中,逐行读取INI文件,每行去除末尾的空格后写入新的INI文件。
十七、处理TOML文件中的行尾空格
在处理TOML文件时,行尾的空格可能会影响TOML文件的解析和使用。可以使用逐行读取和处理的方法,去除每行末尾的空格,然后将处理后的内容写回到文件中。
with open('config.toml', 'r') as infile, open('clean_config.toml', 'w') as outfile:
for line in infile:
clean_line = line.rstrip()
outfile.write(clean_line + '\n')
在这个例子中,逐行读取TOML文件,每行去除末尾的空格后写入新的TOML文件。
十八、处理文本数据中的行尾空格
在处理文本数据时,行尾的空格可能会影响数据的准确性和处理。可以使用逐行读取和处理的方法,去除每行末尾的空格,然后将处理后的内容写回到文件中。
data = [
"This is the first line ",
"This is the second line ",
"This is the third line "
]
clean_data = [line.rstrip() for line in data]
print(clean_data) # Output: ['This is the first line', 'This is the second line', 'This is the third line']
在这个例子中,使用列表推导式和rstrip()
方法处理每一行文本数据,去除每行末尾的空格字符。
十九、处理日志数据中的行尾空格
在处理日志数据时,行尾的空格可能会影响日志的解析和使用。可以使用逐行读取和处理的方法,去除每行末尾的空格,然后将处理后的内容写回到文件中。
logs = [
"2023-10-01 12:00:00 INFO Start processing ",
"2023-10-01 12:01:00 INFO Processing data ",
"2023-10-01 12:02:00 INFO End processing "
]
clean_logs = [log.rstrip() for log in logs]
print(clean_logs) # Output: ['2023-10-01 12:00:00 INFO Start processing', '2023-10-01 12:01:00 INFO Processing data', '2023-10-01 12:02:00 INFO End processing']
在这个例子中,使用列表推导式和rstrip()
方法处理每一行日志数据,去除每行末尾的空格字符。
二十、处理其他类型文件中的行尾空格
在处理其他类型文件时,可以使用逐行读取和处理的方法,去除每行末尾的空格,然后将处理后的内容写回到文件中。
with open('datafile.dat', 'r') as infile, open('clean_datafile.dat', 'w') as outfile:
for line in infile:
clean_line = line.rstrip()
outfile.write(clean_line + '\n')
在这个例子中,逐行读取数据文件,每行去除末尾的空格后写入新的数据文件。
通过上述多种方法和示例,可以看到在Python中有许多方法可以去除行尾的空格。从简单的字符串方法rstrip()
到复杂的正则表达式、处理不同类型文件和数据的方式,可以根据具体需求选择合适的方法来处理行尾空格问题。选择合适的方法不仅可以提高代码的可读性和效率,还可以确保数据的准确性和完整性。
相关问答FAQs:
如何在Python中去掉字符串末尾的空格?
在Python中,可以使用字符串的rstrip()
方法来去掉字符串末尾的空格。这个方法会返回一个新的字符串,去掉了所有在右侧的空白字符,包括空格、制表符等。例如:
my_string = "Hello, World! "
cleaned_string = my_string.rstrip()
print(cleaned_string) # 输出: "Hello, World!"
是否可以去掉行尾的特定字符而不仅仅是空格?
是的,rstrip()
方法不仅可以去掉空格,还可以去掉其他特定的字符。你只需在括号内指定要去掉的字符。例如,如果想去掉行尾的句号,可以这样做:
my_string = "Hello, World!."
cleaned_string = my_string.rstrip('.')
print(cleaned_string) # 输出: "Hello, World!"
如何处理文件中每一行的行尾空格?
可以通过读取文件并逐行处理来去掉每一行末尾的空格。使用with
语句可以确保文件在处理后正确关闭。以下是一个示例代码:
with open('example.txt', 'r') as file:
lines = [line.rstrip() for line in file]
with open('example.txt', 'w') as file:
file.write('\n'.join(lines))
此代码将读取example.txt
文件中的每一行,去掉行尾的空格后再写回文件。
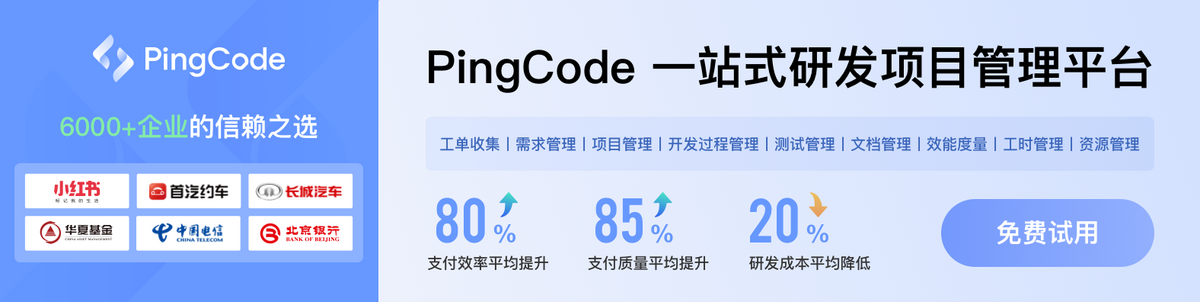