在Python 3.0中创建类非常简单,通过使用class
关键字、类定义、初始化方法等步骤,可以完成一个类的创建。类的定义主要包括三个部分:类的名称、类的属性、类的方法。 例如,使用class
关键字可以定义一个名为Person
的类,然后使用__init__
方法初始化类的属性,最后再定义一些方法来操作这些属性。以下是创建类的基本步骤:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
print(f"Hello, my name is {self.name} and I am {self.age} years old.")
类的初始化方法__init__
用于设置对象的初始状态,定义类时必不可少。在上述示例中,__init__
方法接受两个参数name
和age
,并将其赋值给实例变量self.name
和self.age
。此外,我们定义了一个名为greet
的方法,该方法用于打印出对象的问候语句。
一、类的基本概念
在深入讨论如何在Python 3.0中创建类之前,我们需要先了解一些基本概念。
1、什么是类
类是对象的蓝图或模板,它定义了对象的属性和行为。类可以看作是用户定义的数据类型,包含数据和方法。
2、什么是对象
对象是类的实例。对象由类创建,并具有类定义的属性和方法。每个对象都是独立的,具有不同的状态。
二、创建类的步骤
1、定义类
使用class
关键字定义类。类名通常使用大写字母开头的驼峰命名法。
class Person:
pass
2、初始化方法
__init__
方法是类的初始化方法,当创建类的实例时会自动调用该方法。它用于初始化对象的属性。
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
3、定义方法
方法是类中的函数,用于定义对象的行为。方法的第一个参数通常是self
,表示对象自身。
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
print(f"Hello, my name is {self.name} and I am {self.age} years old.")
三、创建类的实例
1、实例化对象
创建类的实例时,会自动调用__init__
方法,初始化对象的属性。
person1 = Person("Alice", 30)
person2 = Person("Bob", 25)
2、访问属性和方法
通过点号操作符访问对象的属性和方法。
print(person1.name) # 输出:Alice
print(person1.age) # 输出:30
person1.greet() # 输出:Hello, my name is Alice and I am 30 years old.
四、类的继承
1、定义子类
使用继承可以创建一个新类,该类继承自一个或多个基类。子类继承了基类的属性和方法,并可以添加新的属性和方法或重写基类的方法。
class Student(Person):
def __init__(self, name, age, student_id):
super().__init__(name, age)
self.student_id = student_id
def study(self):
print(f"{self.name} is studying.")
2、使用子类
子类具有基类的所有属性和方法,并可以调用基类的方法。
student1 = Student("Charlie", 20, "S12345")
print(student1.name) # 输出:Charlie
print(student1.student_id) # 输出:S12345
student1.greet() # 输出:Hello, my name is Charlie and I am 20 years old.
student1.study() # 输出:Charlie is studying.
五、类的高级特性
1、类属性和实例属性
类属性是类的属性,而实例属性是对象的属性。类属性在所有实例之间共享,而实例属性是特定于每个实例的。
class Person:
species = "Homo sapiens" # 类属性
def __init__(self, name, age):
self.name = name # 实例属性
self.age = age # 实例属性
person1 = Person("Alice", 30)
person2 = Person("Bob", 25)
print(person1.species) # 输出:Homo sapiens
print(person2.species) # 输出:Homo sapiens
2、类方法和静态方法
类方法使用@classmethod
装饰器定义,第一个参数是cls
,表示类本身。静态方法使用@staticmethod
装饰器定义,不需要传递self
或cls
参数。
class Person:
species = "Homo sapiens"
def __init__(self, name, age):
self.name = name
self.age = age
@classmethod
def set_species(cls, species):
cls.species = species
@staticmethod
def is_adult(age):
return age >= 18
person1 = Person("Alice", 30)
Person.set_species("Homo sapiens sapiens")
print(person1.species) # 输出:Homo sapiens sapiens
print(Person.is_adult(20)) # 输出:True
print(Person.is_adult(15)) # 输出:False
六、特殊方法和运算符重载
1、特殊方法
特殊方法是以双下划线开头和结尾的方法,例如__init__
、__str__
等。它们使类的实例与内置类型的行为一致。
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __str__(self):
return f"Person(name={self.name}, age={self.age})"
person1 = Person("Alice", 30)
print(person1) # 输出:Person(name=Alice, age=30)
2、运算符重载
通过定义特殊方法,可以重载运算符,使其适用于类的实例。
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __add__(self, other):
return Vector(self.x + other.x, self.y + other.y)
def __str__(self):
return f"Vector({self.x}, {self.y})"
v1 = Vector(2, 3)
v2 = Vector(1, 1)
v3 = v1 + v2
print(v3) # 输出:Vector(3, 4)
七、类的封装、继承、多态
1、封装
封装是面向对象编程的重要特性,它通过将数据和方法封装在类中,提供数据隐藏和保护。
class Person:
def __init__(self, name, age):
self.__name = name # 私有属性
self.__age = age # 私有属性
def get_name(self):
return self.__name
def set_name(self, name):
self.__name = name
person1 = Person("Alice", 30)
print(person1.get_name()) # 输出:Alice
person1.set_name("Bob")
print(person1.get_name()) # 输出:Bob
2、继承
继承允许一个类继承另一个类的属性和方法,从而实现代码复用。
class Student(Person):
def __init__(self, name, age, student_id):
super().__init__(name, age)
self.student_id = student_id
3、多态
多态允许不同类的对象以相同的方式使用,即使它们的实现不同。
class Person:
def greet(self):
print("Hello!")
class Student(Person):
def greet(self):
print("Hi, I'm a student!")
def greet_person(person):
person.greet()
person1 = Person()
student1 = Student()
greet_person(person1) # 输出:Hello!
greet_person(student1) # 输出:Hi, I'm a student!
八、类的装饰器和元类
1、类装饰器
类装饰器是用于修饰类的函数,它接受一个类并返回一个类。
def add_repr(cls):
cls.__repr__ = lambda self: f"{self.__class__.__name__}(name={self.name}, age={self.age})"
return cls
@add_repr
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
person1 = Person("Alice", 30)
print(person1) # 输出:Person(name=Alice, age=30)
2、元类
元类是用于创建类的类。通过定义元类,可以控制类的创建和行为。
class Meta(type):
def __new__(cls, name, bases, dct):
dct['species'] = 'Homo sapiens'
return super().__new__(cls, name, bases, dct)
class Person(metaclass=Meta):
def __init__(self, name, age):
self.name = name
self.age = age
person1 = Person("Alice", 30)
print(person1.species) # 输出:Homo sapiens
九、类和对象的内存管理
1、垃圾回收
Python使用引用计数和垃圾回收机制管理内存。当对象的引用计数为零时,垃圾回收器会自动回收内存。
import gc
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
person1 = Person("Alice", 30)
del person1
gc.collect() # 强制进行垃圾回收
2、弱引用
弱引用允许对象被垃圾回收,即使存在对该对象的引用。使用weakref
模块可以创建弱引用。
import weakref
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
person1 = Person("Alice", 30)
weak_person = weakref.ref(person1)
print(weak_person()) # 输出:<__main__.Person object at 0x...>
del person1
print(weak_person()) # 输出:None
十、类的模块化和包
1、模块化
将类定义放在单独的模块中,可以提高代码的可读性和可维护性。通过import
语句可以导入模块中的类。
# person.py
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
main.py
from person import Person
person1 = Person("Alice", 30)
print(person1.name) # 输出:Alice
2、包
包是包含多个模块的目录。通过在包目录中创建__init__.py
文件,可以将多个模块组织在一起。
# package/__init__.py
from .person import Person
package/person.py
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
main.py
from package import Person
person1 = Person("Alice", 30)
print(person1.name) # 输出:Alice
十一、类的单例模式
1、单例模式
单例模式确保一个类只有一个实例,并提供一个全局访问点。通过重写__new__
方法可以实现单例模式。
class Singleton:
_instance = None
def __new__(cls, *args, kwargs):
if not cls._instance:
cls._instance = super().__new__(cls, *args, kwargs)
return cls._instance
singleton1 = Singleton()
singleton2 = Singleton()
print(singleton1 is singleton2) # 输出:True
十二、类的工厂模式
1、工厂模式
工厂模式通过创建工厂类或工厂方法来创建对象,而不是直接实例化类。这样可以提高代码的灵活性和可维护性。
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
class PersonFactory:
@staticmethod
def create_person(name, age):
return Person(name, age)
person1 = PersonFactory.create_person("Alice", 30)
print(person1.name) # 输出:Alice
十三、类的抽象基类
1、抽象基类
抽象基类是不能被实例化的类,它定义了子类必须实现的方法。通过abc
模块可以创建抽象基类。
from abc import ABC, abstractmethod
class Animal(ABC):
@abstractmethod
def make_sound(self):
pass
class Dog(Animal):
def make_sound(self):
print("Woof!")
dog = Dog()
dog.make_sound() # 输出:Woof!
十四、类的接口
1、接口
接口是定义一组方法的类,子类必须实现这些方法。Python没有内置的接口机制,但可以通过抽象基类实现接口。
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def area(self):
pass
@abstractmethod
def perimeter(self):
pass
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
def perimeter(self):
return 2 * (self.width + self.height)
rectangle = Rectangle(3, 4)
print(rectangle.area()) # 输出:12
print(rectangle.perimeter()) # 输出:14
十五、类的组合
1、组合
组合是通过将一个类的对象作为另一个类的属性来实现的。组合允许类之间的关系更加灵活。
class Engine:
def start(self):
print("Engine started")
class Car:
def __init__(self):
self.engine = Engine()
def drive(self):
self.engine.start()
print("Car is driving")
car = Car()
car.drive()
输出:
Engine started
Car is driving
十六、类的装饰器模式
1、装饰器模式
装饰器模式通过在不修改原始类的情况下,动态地添加功能。装饰器模式使用组合技术,将装饰器类的对象包装在被装饰类的对象周围。
class Component:
def operation(self):
pass
class ConcreteComponent(Component):
def operation(self):
print("ConcreteComponent operation")
class Decorator(Component):
def __init__(self, component):
self._component = component
def operation(self):
self._component.operation()
class ConcreteDecorator(Decorator):
def operation(self):
super().operation()
print("ConcreteDecorator additional operation")
component = ConcreteComponent()
decorator = ConcreteDecorator(component)
decorator.operation()
输出:
ConcreteComponent operation
ConcreteDecorator additional operation
通过以上步骤和示例,我们可以全面理解如何在Python 3.0中创建类,并掌握类的各种高级特性和设计模式。这些知识将帮助我们编写更为高效、灵活和可维护的代码。
相关问答FAQs:
如何在Python 3.0中定义一个类?
在Python 3.0中,定义一个类使用class
关键字,后跟类名和冒号。类体应缩进,可以包含属性和方法。例如,创建一个名为Dog
的类,可以如下定义:
class Dog:
def __init__(self, name, age):
self.name = name
self.age = age
def bark(self):
return "Woof!"
在类中如何定义和使用方法?
类中的方法是定义在类体内的函数,通常用于操作类的属性。通过self
参数可以访问实例属性。使用方法时,通过实例调用,例如:
my_dog = Dog("Buddy", 3)
print(my_dog.bark()) # 输出:Woof!
如何创建类的实例并访问其属性?
类的实例是通过调用类名并传递必要参数创建的。实例的属性可以通过点操作符访问。例如:
my_dog = Dog("Buddy", 3)
print(my_dog.name) # 输出:Buddy
print(my_dog.age) # 输出:3
这样,你就可以在Python 3.0中创建类、定义方法、实例化对象并访问其属性。
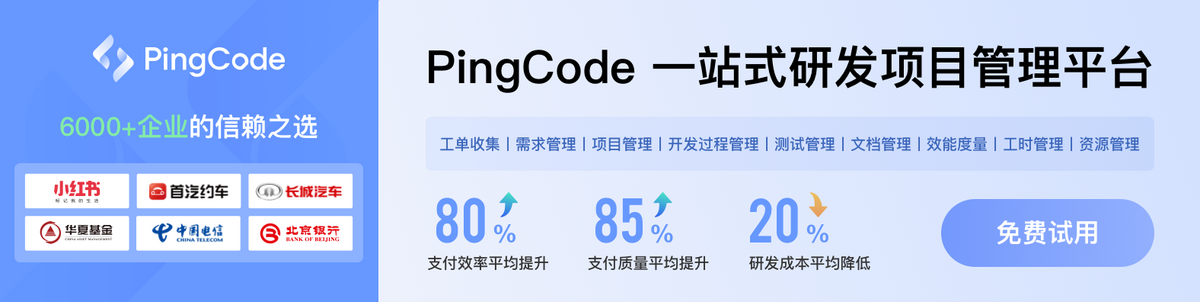