在Python中,创建和读取文本文件可以通过内置的文件操作函数来实现,主要包括打开文件、写入内容、读取内容等操作。具体的方法是使用open()
函数来打开文件,使用write()
函数来写入内容,使用read()
函数来读取内容,使用close()
函数来关闭文件。 下面将详细介绍这些方法,并通过实例说明如何在Python中创建和读取文本文件。
一、创建文本文件
1、使用open()
函数创建文件
要在Python中创建一个文本文件,可以使用open()
函数。open()
函数有两个主要参数:文件名和模式。常见的模式有:
'w'
:写入模式。如果文件不存在,将创建一个新文件;如果文件存在,将覆盖原有内容。'a'
:追加模式。如果文件不存在,将创建一个新文件;如果文件存在,将在文件末尾追加内容。
# 创建并写入文件
file = open('example.txt', 'w')
file.write('Hello, World!')
file.close()
2、使用with
语句创建文件
使用with
语句可以更简洁地处理文件操作,并且在块结束时会自动关闭文件。
# 使用with语句创建并写入文件
with open('example.txt', 'w') as file:
file.write('Hello, World!')
二、读取文本文件
1、使用open()
函数读取文件
要读取文件中的内容,可以使用open()
函数并指定读取模式 'r'
。
读取整个文件内容
# 读取整个文件内容
file = open('example.txt', 'r')
content = file.read()
print(content)
file.close()
读取文件中的每一行
# 读取文件中的每一行
file = open('example.txt', 'r')
for line in file:
print(line, end='')
file.close()
2、使用with
语句读取文件
同样,可以使用with
语句更简洁地读取文件。
读取整个文件内容
# 使用with语句读取整个文件内容
with open('example.txt', 'r') as file:
content = file.read()
print(content)
读取文件中的每一行
# 使用with语句读取文件中的每一行
with open('example.txt', 'r') as file:
for line in file:
print(line, end='')
三、文件读写模式详解
1、写入模式 'w'
写入模式 'w'
会创建一个新文件或覆盖现有文件的内容。如果文件不存在,将创建一个新文件。
with open('example.txt', 'w') as file:
file.write('This is a test file.')
2、追加模式 'a'
追加模式 'a'
会在文件末尾追加内容。如果文件不存在,将创建一个新文件。
with open('example.txt', 'a') as file:
file.write('\nThis is an appended line.')
3、读取模式 'r'
读取模式 'r'
用于读取文件内容。如果文件不存在,将引发 FileNotFoundError
。
with open('example.txt', 'r') as file:
content = file.read()
print(content)
4、读取和写入模式 'r+'
读取和写入模式 'r+'
允许同时读取和写入文件。如果文件不存在,将引发 FileNotFoundError
。
with open('example.txt', 'r+') as file:
content = file.read()
print('Before Writing:')
print(content)
file.write('\nThis is a new line written in r+ mode.')
file.seek(0)
content = file.read()
print('After Writing:')
print(content)
5、二进制模式 'b'
二进制模式用于读取和写入非文本文件,如图像文件。可以在模式字符串后添加 'b'
来指定二进制模式。
# 写入二进制文件
with open('example.bin', 'wb') as file:
file.write(b'\x00\x01\x02\x03')
读取二进制文件
with open('example.bin', 'rb') as file:
content = file.read()
print(content)
四、处理大文件
1、逐行读取
对于大文件,建议逐行读取以避免占用过多内存。
with open('large_file.txt', 'r') as file:
for line in file:
process(line) # 假设有一个处理函数 process
2、逐块读取
可以通过指定 read()
函数的大小参数来逐块读取文件内容。
with open('large_file.txt', 'r') as file:
while True:
chunk = file.read(1024) # 每次读取 1024 字节
if not chunk:
break
process(chunk) # 假设有一个处理函数 process
五、文件路径和目录
1、相对路径与绝对路径
文件路径可以是相对路径或绝对路径。相对路径是相对于当前工作目录的路径,而绝对路径是文件在文件系统中的完整路径。
# 使用相对路径
with open('example.txt', 'r') as file:
content = file.read()
print(content)
使用绝对路径
with open('/path/to/your/file/example.txt', 'r') as file:
content = file.read()
print(content)
2、检查文件是否存在
在操作文件之前,可以使用 os.path
模块来检查文件是否存在。
import os
if os.path.exists('example.txt'):
with open('example.txt', 'r') as file:
content = file.read()
print(content)
else:
print('File does not exist.')
3、创建目录
可以使用 os.makedirs()
函数来创建目录。
import os
if not os.path.exists('new_directory'):
os.makedirs('new_directory')
with open('new_directory/example.txt', 'w') as file:
file.write('This file is in a new directory.')
六、异常处理
1、捕获文件操作异常
在进行文件操作时,可以使用 try-except
语句来捕获可能的异常。
try:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
except FileNotFoundError:
print('File not found.')
except IOError:
print('An I/O error occurred.')
2、确保文件关闭
在发生异常时,确保文件被正确关闭可以使用 finally
语句。
file = None
try:
file = open('example.txt', 'r')
content = file.read()
print(content)
except FileNotFoundError:
print('File not found.')
except IOError:
print('An I/O error occurred.')
finally:
if file:
file.close()
七、文件编码
1、指定文件编码
在处理包含非ASCII字符的文件时,可以指定文件编码。
# 写入文件时指定编码
with open('example.txt', 'w', encoding='utf-8') as file:
file.write('你好,世界!')
读取文件时指定编码
with open('example.txt', 'r', encoding='utf-8') as file:
content = file.read()
print(content)
2、处理不同编码的文件
如果文件使用不同的编码,可以使用 codecs
模块来读取和写入文件。
import codecs
读取GBK编码的文件
with codecs.open('example_gbk.txt', 'r', encoding='gbk') as file:
content = file.read()
print(content)
写入UTF-8编码的文件
with codecs.open('example_utf8.txt', 'w', encoding='utf-8') as file:
file.write('你好,世界!')
八、文件操作的最佳实践
1、使用with
语句
with
语句确保文件在使用结束后自动关闭,避免资源泄露。
with open('example.txt', 'r') as file:
content = file.read()
print(content)
2、检查文件是否存在
在进行文件操作之前,检查文件是否存在可以避免潜在的错误。
import os
if os.path.exists('example.txt'):
with open('example.txt', 'r') as file:
content = file.read()
print(content)
else:
print('File does not exist.')
3、处理文件操作异常
使用 try-except
语句捕获文件操作中的异常,确保程序的健壮性。
try:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
except FileNotFoundError:
print('File not found.')
except IOError:
print('An I/O error occurred.')
4、使用合适的文件模式
根据需要选择合适的文件模式,例如写入模式 'w'
、追加模式 'a'
、读取模式 'r'
、二进制模式 'b'
等。
# 追加模式
with open('example.txt', 'a') as file:
file.write('\nThis is an appended line.')
5、指定文件编码
在处理包含非ASCII字符的文件时,指定文件编码可以避免字符编码问题。
with open('example.txt', 'r', encoding='utf-8') as file:
content = file.read()
print(content)
通过以上方法和最佳实践,您可以在Python中高效地创建和读取文本文件。掌握这些技术将有助于您在实际项目中更好地处理文件操作任务。
相关问答FAQs:
如何使用Python创建一个新的文本文件?
在Python中,可以使用内置的open()
函数创建新的文本文件。通过指定文件的名称和模式为'w'
(写入模式),如果文件不存在,将会被创建。以下是一个简单的示例:
with open('example.txt', 'w') as file:
file.write('这是一个新创建的文本文件。')
这段代码会在当前目录下创建一个名为example.txt
的文件,并写入指定的内容。
如何读取Python中的文本文件内容?
要读取文本文件,可以使用open()
函数并将模式设置为'r'
(读取模式)。使用read()
方法可以一次性读取整个文件的内容,或者使用readlines()
方法按行读取。示例如下:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
这段代码会打开example.txt
文件并打印出其内容。
如何在Python中处理文件读取错误?
在处理文件时,可能会遇到一些错误,比如文件不存在或没有读取权限。可以使用try
和except
语句来捕获这些异常,从而避免程序崩溃。示例如下:
try:
with open('example.txt', 'r') as file:
content = file.read()
except FileNotFoundError:
print("文件未找到,请检查文件名和路径。")
except PermissionError:
print("没有权限读取该文件。")
这种方式不仅可以提高代码的健壮性,还能为用户提供清晰的错误提示。
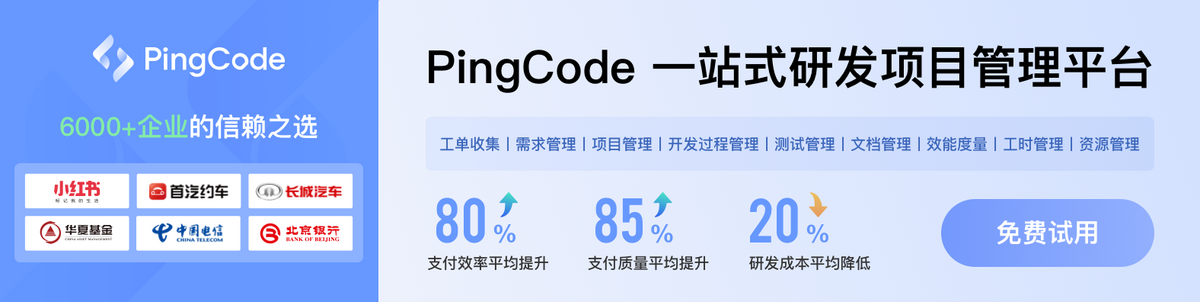