要用Python编写汇率转换程序,可以使用API获取汇率数据、设计用户友好的输入输出界面、进行错误处理等。接下来我们将详细展开如何实现这些功能。
一、使用API获取汇率数据
1、选择汇率API
为了获取最新的汇率数据,我们需要选择一个可靠的API。常用的有以下几种:
- Fixer.io:提供实时汇率和历史汇率数据。
- ExchangeRate-API:提供全球汇率和外汇市场数据。
- Open Exchange Rates:提供免费和付费选项的汇率API。
本文中,我们将使用Fixer.io作为示例。
2、安装相关库
首先,我们需要安装requests库来发送HTTP请求。可以使用以下命令安装:
pip install requests
3、编写获取汇率数据的代码
import requests
def get_exchange_rate(api_key, base_currency, target_currency):
url = f"http://data.fixer.io/api/latest?access_key={api_key}&base={base_currency}&symbols={target_currency}"
response = requests.get(url)
if response.status_code != 200:
raise Exception("Error fetching exchange rate data")
data = response.json()
if not data['success']:
raise Exception(data['error']['info'])
return data['rates'][target_currency]
示例用法
api_key = 'your_api_key'
base_currency = 'USD'
target_currency = 'EUR'
rate = get_exchange_rate(api_key, base_currency, target_currency)
print(f"Exchange rate from {base_currency} to {target_currency} is {rate}")
二、设计用户友好的输入输出界面
1、命令行输入输出
我们可以通过命令行界面让用户输入需要转换的金额和货币类型,然后输出转换后的结果。
def main():
api_key = 'your_api_key'
base_currency = input("Enter the base currency (e.g., USD): ").upper()
target_currency = input("Enter the target currency (e.g., EUR): ").upper()
amount = float(input(f"Enter the amount in {base_currency}: "))
try:
rate = get_exchange_rate(api_key, base_currency, target_currency)
converted_amount = amount * rate
print(f"{amount} {base_currency} is equal to {converted_amount} {target_currency}")
except Exception as e:
print(f"Error: {e}")
if __name__ == "__main__":
main()
2、图形用户界面(GUI)
为了提升用户体验,我们也可以使用Tkinter库来创建一个简单的GUI。
import tkinter as tk
from tkinter import messagebox
def convert_currency():
base_currency = base_currency_entry.get().upper()
target_currency = target_currency_entry.get().upper()
amount = float(amount_entry.get())
try:
rate = get_exchange_rate(api_key, base_currency, target_currency)
converted_amount = amount * rate
result_label.config(text=f"{amount} {base_currency} is equal to {converted_amount} {target_currency}")
except Exception as e:
messagebox.showerror("Error", str(e))
创建主窗口
root = tk.Tk()
root.title("Currency Converter")
添加输入框和标签
tk.Label(root, text="Base Currency:").grid(row=0, column=0)
base_currency_entry = tk.Entry(root)
base_currency_entry.grid(row=0, column=1)
tk.Label(root, text="Target Currency:").grid(row=1, column=0)
target_currency_entry = tk.Entry(root)
target_currency_entry.grid(row=1, column=1)
tk.Label(root, text="Amount:").grid(row=2, column=0)
amount_entry = tk.Entry(root)
amount_entry.grid(row=2, column=1)
添加转换按钮
convert_button = tk.Button(root, text="Convert", command=convert_currency)
convert_button.grid(row=3, column=0, columnspan=2)
添加结果标签
result_label = tk.Label(root, text="")
result_label.grid(row=4, column=0, columnspan=2)
启动主循环
root.mainloop()
三、进行错误处理
1、处理HTTP请求错误
在获取汇率数据的过程中,可能会遇到网络问题或者API服务器问题。我们需要对这些情况进行处理。
def get_exchange_rate(api_key, base_currency, target_currency):
url = f"http://data.fixer.io/api/latest?access_key={api_key}&base={base_currency}&symbols={target_currency}"
try:
response = requests.get(url)
response.raise_for_status() # 如果请求失败,抛出HTTPError
except requests.exceptions.RequestException as e:
raise Exception("Error fetching exchange rate data") from e
data = response.json()
if not data['success']:
raise Exception(data['error']['info'])
return data['rates'][target_currency]
2、处理用户输入错误
用户可能会输入无效的货币代码或者金额。我们需要对这些情况进行处理。
def main():
api_key = 'your_api_key'
base_currency = input("Enter the base currency (e.g., USD): ").upper()
target_currency = input("Enter the target currency (e.g., EUR): ").upper()
try:
amount = float(input(f"Enter the amount in {base_currency}: "))
except ValueError:
print("Invalid amount. Please enter a numeric value.")
return
try:
rate = get_exchange_rate(api_key, base_currency, target_currency)
converted_amount = amount * rate
print(f"{amount} {base_currency} is equal to {converted_amount} {target_currency}")
except Exception as e:
print(f"Error: {e}")
if __name__ == "__main__":
main()
四、扩展功能
1、增加更多货币支持
可以通过修改API请求的参数,支持更多的货币类型。
def get_exchange_rate(api_key, base_currency, target_currency):
url = f"http://data.fixer.io/api/latest?access_key={api_key}&base={base_currency}&symbols={target_currency}"
response = requests.get(url)
if response.status_code != 200:
raise Exception("Error fetching exchange rate data")
data = response.json()
if not data['success']:
raise Exception(data['error']['info'])
return data['rates'][target_currency]
支持更多货币
base_currency = 'USD'
target_currencies = ['EUR', 'GBP', 'JPY']
for target_currency in target_currencies:
rate = get_exchange_rate(api_key, base_currency, target_currency)
print(f"Exchange rate from {base_currency} to {target_currency} is {rate}")
2、添加历史汇率查询功能
有时候用户可能需要查询历史汇率数据。我们可以通过API提供的历史数据接口实现这一功能。
def get_historical_exchange_rate(api_key, base_currency, target_currency, date):
url = f"http://data.fixer.io/api/{date}?access_key={api_key}&base={base_currency}&symbols={target_currency}"
response = requests.get(url)
if response.status_code != 200:
raise Exception("Error fetching historical exchange rate data")
data = response.json()
if not data['success']:
raise Exception(data['error']['info'])
return data['rates'][target_currency]
示例用法
date = '2023-01-01'
rate = get_historical_exchange_rate(api_key, base_currency, target_currency, date)
print(f"Exchange rate from {base_currency} to {target_currency} on {date} was {rate}")
3、定期更新汇率
为了确保汇率数据的准确性,可以定期从API获取最新的汇率数据并更新到本地存储。
import time
import json
def update_exchange_rates(api_key, base_currency, target_currencies, interval=3600):
while True:
rates = {}
for target_currency in target_currencies:
rate = get_exchange_rate(api_key, base_currency, target_currency)
rates[target_currency] = rate
with open('exchange_rates.json', 'w') as f:
json.dump(rates, f)
time.sleep(interval)
示例用法
base_currency = 'USD'
target_currencies = ['EUR', 'GBP', 'JPY']
update_exchange_rates(api_key, base_currency, target_currencies)
五、总结
通过以上步骤,我们可以用Python编写一个功能齐全的汇率转换程序。使用API获取汇率数据、设计用户友好的输入输出界面、进行错误处理、增加更多货币支持、添加历史汇率查询功能、定期更新汇率等功能,使得程序更加实用和可靠。希望本文能帮助到你,更好地实现汇率转换功能。
相关问答FAQs:
如何获取实时汇率数据?
要获取实时汇率数据,您可以利用一些开放的API,例如ExchangeRate-API或Open Exchange Rates。通过这些API,您可以编写Python代码,发送HTTP请求以获取最新的汇率信息。您还可以使用requests库来简化这一过程,并将获取的JSON数据解析为Python字典,以便进行后续的汇率转换操作。
汇率转换程序需要哪些基本模块?
编写汇率转换程序时,您需要使用几个基本的Python模块。requests模块用于发送网络请求,json模块用于解析API返回的数据。此外,您可能还需要使用decimal模块来处理浮点数的精度问题,确保在货币转换时不会产生误差。
如何实现多种货币之间的转换?
在Python中实现多种货币之间的转换,可以创建一个函数,该函数接收原始货币、目标货币和金额作为参数。通过查询汇率并进行计算,您可以得出目标货币的金额。为了方便用户使用,可以将常用货币的汇率预先存储在字典中,或者动态从API获取实时数据。这样,用户只需输入金额和货币类型,即可轻松完成转换。
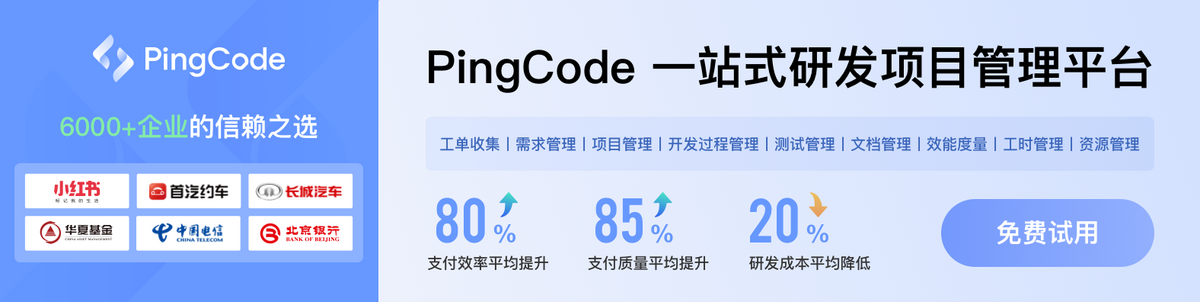