Python设计华丽界面的方式有使用高级GUI库、结合CSS样式和HTML进行设计、利用图形库进行自定义绘图。其中,使用高级GUI库是最常见和有效的方法。Python有许多用于创建图形用户界面(GUI)的库,其中一些提供了丰富的功能和高度的定制选项,使开发者可以创建功能强大且视觉吸引力的应用程序界面。以下是几种常用的高级GUI库:
- PyQt5/PySide2:这是Python中非常流行的GUI库,基于Qt框架,提供了丰富的控件和强大的功能。
- Tkinter:这是Python内置的GUI库,简单易学,适合创建基本的桌面应用程序。
- Kivy:适用于跨平台应用程序开发,特别是移动应用程序,具有高度的定制能力。
- WxPython:这是一个跨平台的GUI库,基于wxWidgets,功能强大且灵活。
下面,我们将详细介绍其中的PyQt5,并展示如何使用它来设计一个华丽的界面。
一、PyQt5的安装和基础
PyQt5是一个功能强大的Python库,它将Qt库(一个跨平台的C++图形用户界面工具包)绑定到Python上,使得Python程序员可以使用Qt的全部功能来创建图形用户界面应用程序。
1、安装PyQt5
要安装PyQt5,可以使用pip命令:
pip install PyQt5
安装完成后,你就可以开始编写基于PyQt5的GUI应用程序了。
2、创建一个简单的窗口
创建一个简单的窗口是学习PyQt5的第一步。以下是一个基本的示例代码:
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow
def main():
app = QApplication(sys.argv)
window = QMainWindow()
window.setWindowTitle('My First PyQt5 App')
window.setGeometry(100, 100, 600, 400)
window.show()
sys.exit(app.exec_())
if __name__ == '__main__':
main()
这段代码创建了一个基本的窗口,设置了窗口标题和大小,并显示了窗口。
二、设计界面的布局
在PyQt5中,可以使用多种布局管理器来组织控件,使界面更加整洁和美观。
1、水平布局和垂直布局
水平布局(QHBoxLayout)和垂直布局(QVBoxLayout)是最常用的布局管理器,它们分别将控件水平和垂直排列。
from PyQt5.QtWidgets import QApplication, QMainWindow, QWidget, QHBoxLayout, QPushButton
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle('Horizontal Layout Example')
self.setGeometry(100, 100, 300, 200)
widget = QWidget()
hbox = QHBoxLayout()
btn1 = QPushButton('Button 1')
btn2 = QPushButton('Button 2')
btn3 = QPushButton('Button 3')
hbox.addWidget(btn1)
hbox.addWidget(btn2)
hbox.addWidget(btn3)
widget.setLayout(hbox)
self.setCentralWidget(widget)
def main():
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
if __name__ == '__main__':
main()
2、网格布局
网格布局(QGridLayout)允许控件以网格形式排列,提供了更灵活的布局方式。
from PyQt5.QtWidgets import QApplication, QMainWindow, QWidget, QGridLayout, QPushButton
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle('Grid Layout Example')
self.setGeometry(100, 100, 400, 300)
widget = QWidget()
grid = QGridLayout()
btn1 = QPushButton('Button 1')
btn2 = QPushButton('Button 2')
btn3 = QPushButton('Button 3')
btn4 = QPushButton('Button 4')
grid.addWidget(btn1, 0, 0)
grid.addWidget(btn2, 0, 1)
grid.addWidget(btn3, 1, 0)
grid.addWidget(btn4, 1, 1)
widget.setLayout(grid)
self.setCentralWidget(widget)
def main():
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
if __name__ == '__main__':
main()
三、使用样式表美化界面
PyQt5支持使用Qt样式表(类似于CSS)来美化控件的外观,使界面更加华丽。
1、基本样式表
以下示例展示了如何使用样式表为按钮添加样式:
from PyQt5.QtWidgets import QApplication, QMainWindow, QWidget, QVBoxLayout, QPushButton
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle('QSS Example')
self.setGeometry(100, 100, 300, 200)
widget = QWidget()
vbox = QVBoxLayout()
btn1 = QPushButton('Button 1')
btn2 = QPushButton('Button 2')
btn1.setStyleSheet('background-color: green; color: white; font-size: 16px;')
btn2.setStyleSheet('background-color: blue; color: yellow; font-size: 16px;')
vbox.addWidget(btn1)
vbox.addWidget(btn2)
widget.setLayout(vbox)
self.setCentralWidget(widget)
def main():
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
if __name__ == '__main__':
main()
2、复杂样式表
你可以创建更复杂的样式表来定义控件的不同状态和更多属性:
btn1.setStyleSheet('''
QPushButton {
background-color: green;
color: white;
font-size: 16px;
border-radius: 10px;
}
QPushButton:hover {
background-color: darkgreen;
}
QPushButton:pressed {
background-color: lightgreen;
}
''')
四、使用图形和动画
使用图形和动画可以使界面更加动感和吸引人。
1、绘制图形
PyQt5提供了QPainter类来绘制各种图形,如线条、矩形、圆形等。
from PyQt5.QtWidgets import QApplication, QMainWindow, QWidget
from PyQt5.QtGui import QPainter, QPen
from PyQt5.QtCore import Qt
class DrawingWidget(QWidget):
def paintEvent(self, event):
painter = QPainter(self)
painter.setPen(QPen(Qt.black, 5, Qt.SolidLine))
painter.drawLine(50, 50, 200, 200)
painter.drawRect(100, 100, 150, 100)
painter.drawEllipse(200, 150, 100, 100)
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle('Drawing Example')
self.setGeometry(100, 100, 400, 300)
self.drawing_widget = DrawingWidget()
self.setCentralWidget(self.drawing_widget)
def main():
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
if __name__ == '__main__':
main()
2、添加动画
使用QPropertyAnimation类可以为控件添加动画效果。
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton
from PyQt5.QtCore import QPropertyAnimation, QRect
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle('Animation Example')
self.setGeometry(100, 100, 400, 300)
self.button = QPushButton('Animated Button', self)
self.button.setGeometry(50, 50, 200, 50)
self.animation = QPropertyAnimation(self.button, b'geometry')
self.animation.setDuration(2000)
self.animation.setStartValue(QRect(50, 50, 200, 50))
self.animation.setEndValue(QRect(150, 150, 200, 50))
self.animation.start()
def main():
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
if __name__ == '__main__':
main()
五、结合CSS和HTML进行设计
除了使用样式表,PyQt5还可以结合HTML和CSS来创建更复杂和美观的界面。
1、使用QWebEngineView显示网页
QWebEngineView是一个基于Chromium的组件,可以在PyQt5应用程序中显示网页。
from PyQt5.QtWidgets import QApplication, QMainWindow
from PyQt5.QtWebEngineWidgets import QWebEngineView
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle('WebEngine Example')
self.setGeometry(100, 100, 800, 600)
self.browser = QWebEngineView()
self.browser.setUrl('https://www.example.com')
self.setCentralWidget(self.browser)
def main():
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
if __name__ == '__main__':
main()
2、内嵌HTML和CSS代码
你也可以在应用程序中嵌入HTML和CSS代码来创建自定义的界面。
from PyQt5.QtWidgets import QApplication, QMainWindow, QVBoxLayout, QWidget
from PyQt5.QtWebEngineWidgets import QWebEngineView
from PyQt5.QtCore import QUrl
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle('Embedded HTML Example')
self.setGeometry(100, 100, 800, 600)
widget = QWidget()
vbox = QVBoxLayout()
self.browser = QWebEngineView()
html_content = '''
<html>
<head>
<style>
body { background-color: #f0f0f0; font-family: Arial, sans-serif; }
h1 { color: #333; }
button { background-color: #4CAF50; color: white; padding: 10px 20px; border: none; border-radius: 5px; cursor: pointer; }
button:hover { background-color: #45a049; }
</style>
</head>
<body>
<h1>Welcome to PyQt5</h1>
<button onclick="alert('Button Clicked!')">Click Me</button>
</body>
</html>
'''
self.browser.setHtml(html_content)
vbox.addWidget(self.browser)
widget.setLayout(vbox)
self.setCentralWidget(widget)
def main():
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
if __name__ == '__main__':
main()
六、使用图形库进行自定义绘图
如果需要更高的自定义能力,可以使用图形库如Pygame或matplotlib进行自定义绘图。
1、使用Pygame绘图
Pygame是一个专为游戏开发设计的库,但也可以用于创建高度自定义的图形界面。
import pygame
import sys
def main():
pygame.init()
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption('Pygame Example')
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
screen.fill((255, 255, 255))
pygame.draw.rect(screen, (0, 128, 0), (100, 100, 200, 100))
pygame.draw.circle(screen, (0, 0, 255), (400, 300), 50)
pygame.display.flip()
pygame.quit()
sys.exit()
if __name__ == '__main__':
main()
2、使用matplotlib绘图
matplotlib是一个功能强大的绘图库,常用于数据可视化,但也可以用于创建自定义图形界面。
import matplotlib.pyplot as plt
from matplotlib.backends.backend_qt5agg import FigureCanvasQTAgg as FigureCanvas
from PyQt5.QtWidgets import QApplication, QMainWindow, QVBoxLayout, QWidget
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle('Matplotlib Example')
self.setGeometry(100, 100, 800, 600)
widget = QWidget()
vbox = QVBoxLayout()
fig, ax = plt.subplots()
ax.plot([0, 1, 2, 3], [0, 1, 4, 9])
ax.set_title('Simple Plot')
canvas = FigureCanvas(fig)
vbox.addWidget(canvas)
widget.setLayout(vbox)
self.setCentralWidget(widget)
def main():
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
if __name__ == '__main__':
main()
七、综合示例
以下是一个综合示例,展示如何结合多种技术创建一个功能丰富且界面华丽的应用程序。
from PyQt5.QtWidgets import QApplication, QMainWindow, QVBoxLayout, QWidget, QPushButton
from PyQt5.QtWebEngineWidgets import QWebEngineView
from PyQt5.QtCore import QPropertyAnimation, QRect
import matplotlib.pyplot as plt
from matplotlib.backends.backend_qt5agg import FigureCanvasQTAgg as FigureCanvas
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle('Comprehensive Example')
self.setGeometry(100, 100, 1200, 800)
widget = QWidget()
vbox = QVBoxLayout()
# Web browser
self.browser = QWebEngineView()
html_content = '''
<html>
<head>
<style>
body { background-color: #f0f0f0; font-family: Arial, sans-serif; }
h1 { color: #333; }
button { background-color: #4CAF50; color: white; padding: 10px 20px; border: none; border-radius: 5px; cursor: pointer; }
button:hover { background-color: #45a049; }
</style>
</head>
<body>
<h1>Welcome to PyQt5</h1>
<button onclick="alert('Button Clicked!')">Click Me</button>
</body>
</html>
'''
self.browser.setHtml(html_content)
# Animated button
self.button = QPushButton('Animated Button')
self.button.setStyleSheet('background-color: orange; color: white; font-size: 16px; border-radius: 10px;')
self.button.setGeometry(50, 50, 200, 50)
self.animation = QPropertyAnimation(self.button, b'geometry')
self.animation.setDuration(2000)
self.animation.setStartValue(QRect(50, 50, 200, 50))
self.animation.setEndValue(QRect(300, 50, 200, 50))
self.animation.start()
# Matplotlib plot
fig, ax = plt.subplots()
ax.plot([0, 1, 2, 3], [0, 1, 4, 9])
ax.set_title('Simple Plot')
canvas = FigureCanvas(fig)
vbox.addWidget(self.browser)
vbox.addWidget(self.button)
vbox.addWidget(canvas)
widget.setLayout(vbox)
self.setCentralWidget(widget)
def main():
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
if __name__ == '__main__':
main()
总结起来,设计一个华丽的界面需要结合多种技术和工具,包括使用高级GUI库(如PyQt5)、样式表、图形和动画、以及图形库进行自定义绘图。通过灵活使用这些工具,可以创建出功能强大且视觉吸引力的应用程序界面。
相关问答FAQs:
如何选择适合Python的GUI库来设计华丽的界面?
在Python中,有多种GUI库可供选择,如Tkinter、PyQt、Kivy和wxPython。选择合适的库取决于你的需求和项目的复杂性。Tkinter适合简单的应用,PyQt提供了丰富的界面组件和更好的美观性,而Kivy则适合跨平台移动应用开发。了解每个库的特点,有助于你设计出华丽的界面。
设计华丽界面时有哪些配色和字体选择建议?
在设计界面时,配色和字体的选择至关重要。建议使用互补色或类似色以增强视觉吸引力,同时确保文字与背景之间有足够的对比度。对于字体,选择简洁易读的样式,并确保在各个设备上都能良好显示。可以参考一些在线配色工具和字体组合网站,以获得灵感。
如何使用Python实现动态效果和交互功能以提升界面美观性?
动态效果和交互功能可以显著提升用户体验。使用Python中的动画库或GUI库自带的动画功能,可以为按钮、窗口等元素添加动画效果。结合事件处理机制,能够让用户在界面上进行交互时获得即时反馈,从而使界面显得更加生动和华丽。
在设计华丽界面时,如何确保用户体验的流畅性?
确保用户体验的流畅性可以从多个方面入手。尽量减少界面中的加载时间,优化图像和资源的使用。使用合适的布局管理器,以确保组件在不同屏幕尺寸下的良好适应。同时,提供清晰的导航和反馈机制,让用户能够轻松理解和操作界面。
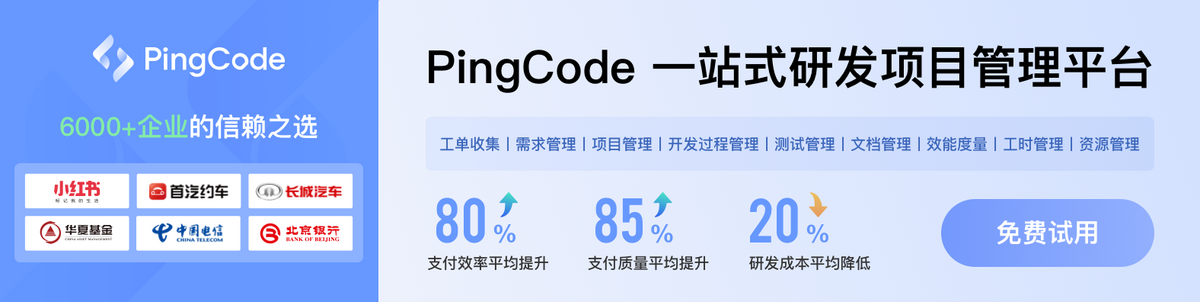