Python保留计算过程的方法有:使用变量、使用日志系统、使用数据结构、使用装饰器、使用上下文管理器。以下详细描述其中的一个方法——使用日志系统。
使用日志系统可以帮助我们在计算过程中记录每一步的计算结果、输入和输出等详细信息。Python 标准库中的 logging
模块是一个非常强大且灵活的日志记录工具。通过配置 logging
模块,我们可以将日志输出到控制台、文件甚至远程服务器。记录的日志信息可以包括时间戳、日志级别、消息内容等。这样一来,即使程序运行很久以后,我们仍然可以通过日志文件查看计算过程中的详细信息,帮助我们进行调试和优化。
一、使用变量保留计算过程
使用变量是最直接和常见的方法。通过在计算过程中引入中间变量,可以保留每一步的计算结果,方便后续使用或调试。
# 示例:计算一个表达式的过程
a = 10
b = 5
c = a + b
d = c * 2
e = d / 3
print("a =", a)
print("b =", b)
print("c =", c)
print("d =", d)
print("e =", e)
在上述示例中,我们通过引入中间变量 c
, d
和 e
,保留了每一步的计算结果,并通过 print
函数输出这些结果。在实际开发中,可以将这些中间变量保存到一个数据结构中,如列表或字典,以便更加系统地管理和使用。
二、使用日志系统保留计算过程
日志系统是一种非常灵活和强大的保留计算过程的方法。通过配置日志系统,可以将计算过程中的详细信息记录下来,并输出到控制台、文件或远程服务器。
import logging
配置日志系统
logging.basicConfig(level=logging.DEBUG, format='%(asctime)s - %(levelname)s - %(message)s', filename='calculation.log', filemode='w')
def calculate(a, b):
logging.info(f'Starting calculation with a={a}, b={b}')
c = a + b
logging.debug(f'Calculated c = a + b = {c}')
d = c * 2
logging.debug(f'Calculated d = c * 2 = {d}')
e = d / 3
logging.debug(f'Calculated e = d / 3 = {e}')
logging.info(f'Finished calculation with result e={e}')
return e
result = calculate(10, 5)
print(f'Result: {result}')
在上述示例中,我们通过 logging
模块配置了日志系统,并在计算过程中记录了每一步的计算结果。这样一来,即使程序运行很久以后,我们仍然可以通过日志文件查看计算过程中的详细信息,帮助我们进行调试和优化。
三、使用数据结构保留计算过程
在复杂的计算过程中,可以使用数据结构(如列表、字典或类)来系统地管理和保留计算过程中的中间结果。
# 示例:使用字典保留计算过程
calculation_steps = {}
a = 10
b = 5
calculation_steps['initial'] = {'a': a, 'b': b}
c = a + b
calculation_steps['step1'] = {'c': c}
d = c * 2
calculation_steps['step2'] = {'d': d}
e = d / 3
calculation_steps['step3'] = {'e': e}
for step, values in calculation_steps.items():
print(f'{step}: {values}')
在上述示例中,我们通过字典 calculation_steps
保留了计算过程中的每一步结果。这样一来,我们可以更加系统地管理和使用这些中间结果。
四、使用装饰器保留计算过程
装饰器是一种非常强大的工具,可以在不修改原有函数代码的情况下,增强函数的功能。通过使用装饰器,我们可以方便地记录函数的输入、输出和中间计算结果。
import logging
配置日志系统
logging.basicConfig(level=logging.DEBUG, format='%(asctime)s - %(levelname)s - %(message)s', filename='calculation.log', filemode='w')
def log_calculation(func):
def wrapper(*args, kwargs):
logging.info(f'Starting calculation with args={args}, kwargs={kwargs}')
result = func(*args, kwargs)
logging.info(f'Finished calculation with result={result}')
return result
return wrapper
@log_calculation
def calculate(a, b):
c = a + b
logging.debug(f'Calculated c = a + b = {c}')
d = c * 2
logging.debug(f'Calculated d = c * 2 = {d}')
e = d / 3
logging.debug(f'Calculated e = d / 3 = {e}')
return e
result = calculate(10, 5)
print(f'Result: {result}')
在上述示例中,我们定义了一个装饰器 log_calculation
,用于记录函数的输入、输出和中间计算结果。通过使用该装饰器,我们可以方便地增强 calculate
函数的功能,而无需修改原有代码。
五、使用上下文管理器保留计算过程
上下文管理器是一种非常强大的工具,可以在代码块的进入和退出时执行特定的操作。通过使用上下文管理器,我们可以方便地记录计算过程中的详细信息。
import logging
配置日志系统
logging.basicConfig(level=logging.DEBUG, format='%(asctime)s - %(levelname)s - %(message)s', filename='calculation.log', filemode='w')
class CalculationContext:
def __init__(self, a, b):
self.a = a
self.b = b
def __enter__(self):
logging.info(f'Starting calculation with a={self.a}, b={self.b}')
return self
def __exit__(self, exc_type, exc_value, traceback):
if exc_type is not None:
logging.error(f'Error occurred: {exc_value}')
else:
logging.info('Finished calculation')
return False
def calculate(a, b):
with CalculationContext(a, b):
c = a + b
logging.debug(f'Calculated c = a + b = {c}')
d = c * 2
logging.debug(f'Calculated d = c * 2 = {d}')
e = d / 3
logging.debug(f'Calculated e = d / 3 = {e}')
return e
result = calculate(10, 5)
print(f'Result: {result}')
在上述示例中,我们定义了一个上下文管理器 CalculationContext
,用于记录计算过程中的详细信息。通过使用该上下文管理器,我们可以方便地记录计算过程中的详细信息,并在出现异常时记录错误信息。
六、使用装饰器保留计算过程
装饰器是Python中一种非常强大的功能,它允许我们在不改变原有函数代码的情况下,增加额外的功能。我们可以利用装饰器来记录函数的输入、输出和中间计算结果,从而实现保留计算过程的目的。
import logging
配置日志系统
logging.basicConfig(level=logging.DEBUG, format='%(asctime)s - %(levelname)s - %(message)s', filename='calculation.log', filemode='w')
def log_calculation(func):
def wrapper(*args, kwargs):
logging.info(f'Starting calculation with args={args}, kwargs={kwargs}')
result = func(*args, kwargs)
logging.info(f'Finished calculation with result={result}')
return result
return wrapper
@log_calculation
def calculate(a, b):
c = a + b
logging.debug(f'Calculated c = a + b = {c}')
d = c * 2
logging.debug(f'Calculated d = c * 2 = {d}')
e = d / 3
logging.debug(f'Calculated e = d / 3 = {e}')
return e
result = calculate(10, 5)
print(f'Result: {result}')
在上述示例中,我们定义了一个装饰器 log_calculation
,用于记录函数的输入、输出和中间计算结果。通过使用该装饰器,我们可以方便地增强 calculate
函数的功能,而无需修改原有代码。
七、使用上下文管理器保留计算过程
上下文管理器是一种非常强大的工具,可以在代码块的进入和退出时执行特定的操作。通过使用上下文管理器,我们可以方便地记录计算过程中的详细信息。
import logging
配置日志系统
logging.basicConfig(level=logging.DEBUG, format='%(asctime)s - %(levelname)s - %(message)s', filename='calculation.log', filemode='w')
class CalculationContext:
def __init__(self, a, b):
self.a = a
self.b = b
def __enter__(self):
logging.info(f'Starting calculation with a={self.a}, b={self.b}')
return self
def __exit__(self, exc_type, exc_value, traceback):
if exc_type is not None:
logging.error(f'Error occurred: {exc_value}')
else:
logging.info('Finished calculation')
return False
def calculate(a, b):
with CalculationContext(a, b):
c = a + b
logging.debug(f'Calculated c = a + b = {c}')
d = c * 2
logging.debug(f'Calculated d = c * 2 = {d}')
e = d / 3
logging.debug(f'Calculated e = d / 3 = {e}')
return e
result = calculate(10, 5)
print(f'Result: {result}')
在上述示例中,我们定义了一个上下文管理器 CalculationContext
,用于记录计算过程中的详细信息。通过使用该上下文管理器,我们可以方便地记录计算过程中的详细信息,并在出现异常时记录错误信息。
八、使用自定义类保留计算过程
在复杂的计算过程中,我们可以通过定义自定义类来系统地管理和保留计算过程中的中间结果。这种方法可以使我们的代码更加结构化和易于维护。
class Calculation:
def __init__(self, a, b):
self.a = a
self.b = b
self.steps = []
def add_step(self, description, value):
self.steps.append({'description': description, 'value': value})
def execute(self):
self.add_step('Initial values', {'a': self.a, 'b': self.b})
c = self.a + self.b
self.add_step('a + b', c)
d = c * 2
self.add_step('c * 2', d)
e = d / 3
self.add_step('d / 3', e)
return e
def print_steps(self):
for step in self.steps:
print(f"{step['description']}: {step['value']}")
calculation = Calculation(10, 5)
result = calculation.execute()
calculation.print_steps()
print(f'Result: {result}')
在上述示例中,我们定义了一个 Calculation
类,用于系统地管理和保留计算过程中的中间结果。通过这种方法,我们可以使代码更加结构化和易于维护。
九、使用第三方库保留计算过程
在实际开发中,我们还可以利用一些第三方库来保留计算过程。例如,Pandas 是一个非常强大的数据分析库,可以方便地记录和管理计算过程中的中间结果。
import pandas as pd
初始化数据框
df = pd.DataFrame(columns=['Step', 'Value'])
计算过程
a = 10
b = 5
df = df.append({'Step': 'Initial values', 'Value': {'a': a, 'b': b}}, ignore_index=True)
c = a + b
df = df.append({'Step': 'a + b', 'Value': c}, ignore_index=True)
d = c * 2
df = df.append({'Step': 'c * 2', 'Value': d}, ignore_index=True)
e = d / 3
df = df.append({'Step': 'd / 3', 'Value': e}, ignore_index=True)
打印计算步骤
print(df)
print(f'Result: {e}')
在上述示例中,我们利用 Pandas 库记录和管理计算过程中的中间结果。通过这种方法,我们可以更加方便地进行数据分析和可视化。
十、使用嵌套函数保留计算过程
嵌套函数也是一种保留计算过程的有效方法。通过定义嵌套函数,我们可以在内部函数中记录中间计算结果,并将这些结果传递给外部函数。
def calculate(a, b):
steps = []
def add_step(description, value):
steps.append({'description': description, 'value': value})
add_step('Initial values', {'a': a, 'b': b})
c = a + b
add_step('a + b', c)
d = c * 2
add_step('c * 2', d)
e = d / 3
add_step('d / 3', e)
return e, steps
result, steps = calculate(10, 5)
打印计算步骤
for step in steps:
print(f"{step['description']}: {step['value']}")
print(f'Result: {result}')
在上述示例中,我们通过定义嵌套函数 add_step
来记录中间计算结果,并将这些结果传递给外部函数 calculate
。通过这种方法,我们可以方便地保留计算过程中的详细信息。
十一、使用生成器保留计算过程
生成器是一种非常强大的工具,可以在计算过程中逐步生成中间结果。通过使用生成器,我们可以方便地记录和保留计算过程中的中间结果。
def calculate(a, b):
yield {'description': 'Initial values', 'value': {'a': a, 'b': b}}
c = a + b
yield {'description': 'a + b', 'value': c}
d = c * 2
yield {'description': 'c * 2', 'value': d}
e = d / 3
yield {'description': 'd / 3', 'value': e}
return e
steps = list(calculate(10, 5))
打印计算步骤
for step in steps:
print(f"{step['description']}: {step['value']}")
print(f'Result: {steps[-1]['value']}")
在上述示例中,我们通过生成器 calculate
逐步生成中间结果,并将这些结果保留在列表 steps
中。通过这种方法,我们可以方便地记录和保留计算过程中的详细信息。
十二、使用闭包保留计算过程
闭包是一种非常强大的工具,可以在函数内部保留计算过程中的中间结果。通过使用闭包,我们可以方便地记录和保留计算过程中的详细信息。
def calculate(a, b):
steps = []
def add_step(description, value):
steps.append({'description': description, 'value': value})
def execute():
add_step('Initial values', {'a': a, 'b': b})
c = a + b
add_step('a + b', c)
d = c * 2
add_step('c * 2', d)
e = d / 3
add_step('d / 3', e)
return e
return execute, steps
execute, steps = calculate(10, 5)
result = execute()
打印计算步骤
for step in steps:
print(f"{step['description']}: {step['value']}")
print(f'Result: {result}')
在上述示例中,我们通过闭包 add_step
和 execute
来记录和保留计算过程中的中间结果。通过这种方法,我们可以方便地记录和保留计算过程中的详细信息。
十三、使用元编程保留计算过程
元编程是一种非常强大的编程技巧,可以在运行时动态修改程序的行为。通过使用元编程,我们可以在不修改原有代码的情况下,记录和保留计算过程中的详细信息。
import logging
配置日志系统
logging.basicConfig(level=logging.DEBUG, format='%(asctime)s - %(levelname)s - %(message)s', filename='calculation.log', filemode='w')
class MetaCalculation(type):
def __new__(cls, name, bases, attrs):
for key, value in attrs.items():
if callable(value):
def log_func(func):
def wrapper(*args, kwargs):
logging.info(f'Starting {key} with args={args}, kwargs={kwargs}')
result = func(*args,
相关问答FAQs:
如何在Python中记录计算过程?
在Python中,可以使用日志记录模块(如logging
)来保存计算过程。通过配置日志级别和格式,可以有效地捕获程序的运行信息,包括输入参数、中间结果和最终输出。例如,通过设置日志级别为DEBUG,程序将在控制台和指定文件中输出详细的计算步骤,便于后续的审查和调试。
有没有推荐的工具或库可以帮助保留Python计算过程?
除了使用内置的logging
模块,其他一些工具和库也可以有效帮助记录计算过程。pandas
库提供了数据框的功能,可以在数据处理时保存中间结果到文件。Jupyter Notebook
非常适合逐步执行代码并即时查看计算结果,用户可以直接在Notebook中查看每一步的输出和图表,这样便于跟踪计算过程。
如何从计算结果中恢复计算过程?
在Python中,如果需要从最终结果反推计算过程,可以考虑使用pickle
模块保存中间数据。通过序列化对象,用户可以在需要时加载它们,分析中间步骤。此外,保持良好的代码注释和文档也是恢复计算过程的重要方法,能够帮助其他开发者理解每一步的逻辑和目的。
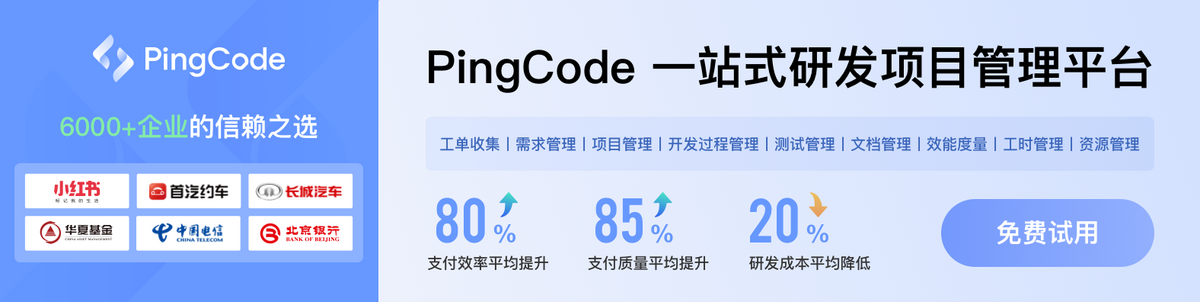