Python选择性运行的方法包括使用条件语句、函数、模块导入、命令行参数、装饰器、上下文管理器。其中,条件语句是最常见且直接的方法,可以根据特定条件控制代码的执行。
条件语句(if-else):通过条件语句,可以根据具体条件决定是否运行某段代码。例如:
flag = True
if flag:
print("This code runs only if flag is True")
这种方法非常灵活,可以根据各种条件控制代码执行。接下来,我们将详细介绍如何使用Python的这些方法实现选择性运行代码。
一、条件语句
条件语句基础
条件语句是实现选择性运行的最基本方法。Python中使用if
、elif
、else
语句来实现条件判断。
x = 10
if x > 5:
print("x is greater than 5")
elif x == 5:
print("x is equal to 5")
else:
print("x is less than 5")
多重条件判断
在实际应用中,可能需要根据多个条件判断是否运行代码。可以使用逻辑运算符and
、or
来组合多个条件。
x = 10
y = 20
if x > 5 and y < 25:
print("Both conditions are true")
elif x > 5 or y < 25:
print("At least one condition is true")
else:
print("Both conditions are false")
二、函数
函数的定义和调用
函数是Python中组织代码的基本单元,可以通过定义和调用函数实现选择性运行代码。
def my_function():
print("This is my function")
condition = True
if condition:
my_function()
带参数的函数
函数可以带参数,根据不同参数执行不同的代码。
def greet(name):
print(f"Hello, {name}")
if True:
greet("Alice")
else:
greet("Bob")
返回值的函数
函数可以有返回值,根据返回值决定是否执行其他代码。
def check_value(x):
return x > 10
if check_value(15):
print("Value is greater than 10")
else:
print("Value is less than or equal to 10")
三、模块导入
按需导入模块
Python允许按需导入模块,这样可以根据条件决定是否导入某个模块并运行其中的代码。
import sys
if 'numpy' in sys.modules:
import numpy as np
print("Numpy is imported")
else:
print("Numpy is not imported")
条件导入模块
可以根据条件导入不同的模块。
use_numpy = True
if use_numpy:
import numpy as np
print("Numpy is imported")
else:
import math
print("Math is imported")
四、命令行参数
使用sys.argv
Python脚本可以接收命令行参数,根据参数决定是否运行某段代码。
import sys
if len(sys.argv) > 1 and sys.argv[1] == 'run':
print("Running the script with 'run' argument")
else:
print("Script not run")
使用argparse
模块
argparse
模块是Python标准库中的命令行参数解析模块,功能更强大。
import argparse
parser = argparse.ArgumentParser(description="A script that runs conditionally")
parser.add_argument('--run', action='store_true', help="Run the script")
args = parser.parse_args()
if args.run:
print("Running the script")
else:
print("Script not run")
五、装饰器
基础装饰器
装饰器可以在函数执行前后添加额外行为,实现选择性运行代码。
def my_decorator(func):
def wrapper():
print("Something before the function")
func()
print("Something after the function")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
带参数的装饰器
装饰器可以带参数,允许更灵活的控制。
def conditional_decorator(condition):
def decorator(func):
def wrapper(*args, kwargs):
if condition:
return func(*args, kwargs)
else:
print("Condition not met, function not run")
return wrapper
return decorator
@conditional_decorator(condition=True)
def say_hello():
print("Hello!")
say_hello()
六、上下文管理器
基本上下文管理器
上下文管理器可以在代码块前后执行特定操作,实现选择性运行。
class MyContext:
def __enter__(self):
print("Entering the context")
return self
def __exit__(self, exc_type, exc_value, traceback):
print("Exiting the context")
with MyContext():
print("Inside the context")
条件上下文管理器
上下文管理器可以根据条件决定是否执行。
class ConditionalContext:
def __init__(self, condition):
self.condition = condition
def __enter__(self):
if self.condition:
print("Condition met, entering context")
return self
else:
return None
def __exit__(self, exc_type, exc_value, traceback):
if self.condition:
print("Condition met, exiting context")
condition = True
with ConditionalContext(condition) as context:
if context:
print("Inside the context")
else:
print("Context not entered")
七、结论
综上所述,Python提供了多种方法来实现选择性运行代码,包括条件语句、函数、模块导入、命令行参数、装饰器和上下文管理器。这些方法各有优劣,可以根据具体需求选择合适的方法。在日常开发中,灵活运用这些方法,可以大大提高代码的可读性和可维护性。条件语句、函数、模块导入、命令行参数、装饰器、上下文管理器是实现选择性运行的主要手段,掌握这些方法将有助于编写高效、灵活的Python代码。
相关问答FAQs:
如何在Python中实现条件性代码执行?
在Python中,可以通过使用条件语句(如if、elif和else)来实现代码的选择性运行。通过这些语句,可以根据特定条件的真假来执行不同的代码块。例如,使用if
语句来检查变量的值,如果满足条件则执行相应的代码,这样可以灵活地控制程序的执行流。
有什么方法可以提高Python代码的选择性运行效率?
为了提高选择性运行的效率,可以利用函数和类来封装代码逻辑。这样,在需要执行特定功能时,只调用相应的函数或类的方法,避免不必要的代码执行。此外,使用装饰器和上下文管理器也可以帮助简化代码并提高执行效率,使得条件判断和执行变得更加清晰和高效。
如何使用命令行参数来实现Python脚本的选择性执行?
通过argparse
模块,可以方便地从命令行获取参数并根据这些参数来决定代码的执行逻辑。定义不同的命令行参数后,可以在脚本中检查这些参数的值,从而选择性地运行特定的功能或代码块。这种方式特别适用于需要根据用户输入或配置文件来调整程序行为的场景。
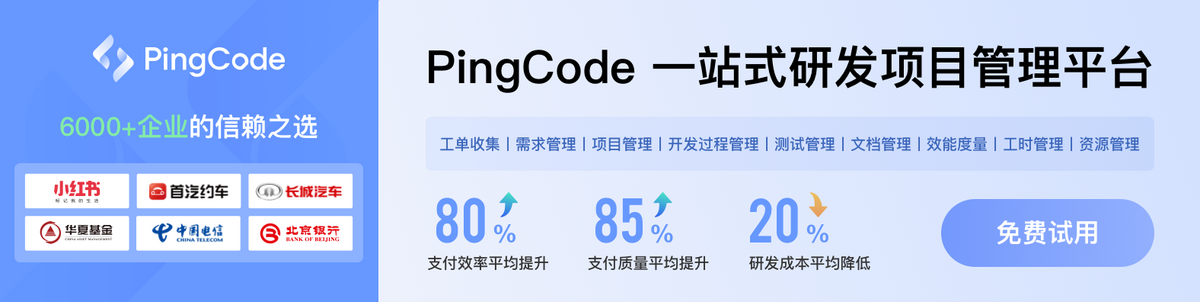