在Python中遍历索引值的方法有多种,可以使用range()
函数、enumerate()
函数、利用列表推导式等。使用enumerate()
函数是最常见和推荐的方法,因为它不仅可以遍历索引,还可以直接获取相应的元素。下面我将详细展开enumerate()
函数的使用方法。
enumerate()
函数用于遍历一个可迭代对象,同时返回元素的索引和值。它的语法为:enumerate(iterable, start=0)
,其中iterable
是任何可迭代对象,start
是索引的起始值,默认为0。使用enumerate()
函数不仅代码更加简洁,还能避免手动维护索引,从而减少出错的可能性。
举例来说,假设我们有一个列表fruits
,我们可以使用enumerate()
函数来遍历其索引和值:
fruits = ['apple', 'banana', 'cherry']
for index, value in enumerate(fruits):
print(f"Index: {index}, Value: {value}")
这段代码输出如下:
Index: 0, Value: apple
Index: 1, Value: banana
Index: 2, Value: cherry
下面我们将详细介绍各种遍历索引值的方法及其使用场景。
一、使用 enumerate()
函数
enumerate()
函数是Python中最常见的遍历索引值的方法。它不仅可以遍历索引,还可以直接获取相应的元素,非常方便。下面是一些使用enumerate()
函数的示例和说明。
1. 基本用法
在大多数情况下,我们只需要遍历列表的索引和值。使用enumerate()
函数可以非常方便地实现这一点。
fruits = ['apple', 'banana', 'cherry']
for index, value in enumerate(fruits):
print(f"Index: {index}, Value: {value}")
在这个例子中,我们使用enumerate()
函数遍历了fruits
列表的索引和值。enumerate()
函数返回一个枚举对象,枚举对象是一个可迭代对象,每次迭代都会返回一个元组,其中包含索引和值。
2. 指定起始索引
enumerate()
函数的第二个参数可以用来指定起始索引,默认从0开始。
fruits = ['apple', 'banana', 'cherry']
for index, value in enumerate(fruits, start=1):
print(f"Index: {index}, Value: {value}")
在这个例子中,我们将起始索引指定为1,因此输出的索引值从1开始。
3. 遍历其他可迭代对象
enumerate()
函数不仅可以遍历列表,还可以遍历其他可迭代对象,如元组、字符串等。
# 遍历元组
colors = ('red', 'green', 'blue')
for index, value in enumerate(colors):
print(f"Index: {index}, Value: {value}")
遍历字符串
text = "hello"
for index, char in enumerate(text):
print(f"Index: {index}, Character: {char}")
在这些例子中,我们使用enumerate()
函数遍历了元组和字符串的索引和值。
二、使用 range()
函数
除了enumerate()
函数,我们还可以使用range()
函数遍历索引值。虽然这种方法需要手动获取元素,但在某些情况下可能更为直观。
1. 基本用法
我们可以使用range()
函数生成一个索引序列,然后通过索引访问列表元素。
fruits = ['apple', 'banana', 'cherry']
for index in range(len(fruits)):
print(f"Index: {index}, Value: {fruits[index]}")
在这个例子中,我们使用range(len(fruits))
生成一个索引序列,然后通过索引访问列表元素。
2. 指定起始和步长
range()
函数还可以指定起始值、结束值和步长。
numbers = [10, 20, 30, 40, 50]
for index in range(1, len(numbers), 2):
print(f"Index: {index}, Value: {numbers[index]}")
在这个例子中,我们使用range(1, len(numbers), 2)
生成一个从1开始,步长为2的索引序列,然后通过索引访问列表元素。
3. 遍历其他可迭代对象
range()
函数也可以用于遍历其他可迭代对象的索引值。
# 遍历元组
colors = ('red', 'green', 'blue')
for index in range(len(colors)):
print(f"Index: {index}, Value: {colors[index]}")
遍历字符串
text = "hello"
for index in range(len(text)):
print(f"Index: {index}, Character: {text[index]}")
在这些例子中,我们使用range()
函数遍历了元组和字符串的索引和值。
三、使用列表推导式
列表推导式是一种简洁的语法,可以用于生成列表或其他可迭代对象。我们也可以使用列表推导式遍历索引值。
1. 基本用法
我们可以使用列表推导式生成一个索引和值的列表,然后遍历该列表。
fruits = ['apple', 'banana', 'cherry']
index_value_list = [(index, value) for index, value in enumerate(fruits)]
for index, value in index_value_list:
print(f"Index: {index}, Value: {value}")
在这个例子中,我们使用列表推导式生成了一个包含索引和值的列表,然后遍历该列表。
2. 结合条件
列表推导式还可以结合条件,用于生成满足特定条件的索引和值。
numbers = [10, 20, 30, 40, 50]
index_value_list = [(index, value) for index, value in enumerate(numbers) if value > 20]
for index, value in index_value_list:
print(f"Index: {index}, Value: {value}")
在这个例子中,我们使用列表推导式生成了一个值大于20的索引和值的列表,然后遍历该列表。
3. 遍历其他可迭代对象
列表推导式也可以用于遍历其他可迭代对象的索引值。
# 遍历元组
colors = ('red', 'green', 'blue')
index_value_list = [(index, value) for index, value in enumerate(colors)]
for index, value in index_value_list:
print(f"Index: {index}, Value: {value}")
遍历字符串
text = "hello"
index_char_list = [(index, char) for index, char in enumerate(text)]
for index, char in index_char_list:
print(f"Index: {index}, Character: {char}")
在这些例子中,我们使用列表推导式遍历了元组和字符串的索引和值。
四、使用 zip()
函数
zip()
函数可以将多个可迭代对象打包成一个元组的迭代器。在某些情况下,我们可以结合range()
函数和zip()
函数遍历索引值。
1. 基本用法
我们可以使用range()
函数生成索引序列,然后使用zip()
函数将索引和值打包在一起。
fruits = ['apple', 'banana', 'cherry']
for index, value in zip(range(len(fruits)), fruits):
print(f"Index: {index}, Value: {value}")
在这个例子中,我们使用zip(range(len(fruits)), fruits)
将索引和值打包在一起,然后遍历该迭代器。
2. 遍历多个列表
zip()
函数还可以用于遍历多个列表的索引和值。
fruits = ['apple', 'banana', 'cherry']
colors = ['red', 'yellow', 'red']
for index, (fruit, color) in enumerate(zip(fruits, colors)):
print(f"Index: {index}, Fruit: {fruit}, Color: {color}")
在这个例子中,我们使用enumerate(zip(fruits, colors))
遍历了fruits
和colors
列表的索引和值。
3. 遍历其他可迭代对象
zip()
函数也可以用于遍历其他可迭代对象的索引值。
# 遍历元组和字符串
colors = ('red', 'green', 'blue')
text = "RGB"
for index, (color, char) in enumerate(zip(colors, text)):
print(f"Index: {index}, Color: {color}, Character: {char}")
在这个例子中,我们使用enumerate(zip(colors, text))
遍历了元组和字符串的索引和值。
五、使用 itertools
模块
itertools
模块提供了许多用于操作迭代器的函数。在某些复杂的场景中,我们可以使用itertools
模块提供的函数遍历索引值。
1. 使用 itertools.count()
itertools.count()
函数生成一个无限的整数序列,我们可以结合zip()
函数遍历索引和值。
import itertools
fruits = ['apple', 'banana', 'cherry']
for index, value in zip(itertools.count(), fruits):
print(f"Index: {index}, Value: {value}")
在这个例子中,我们使用itertools.count()
生成一个无限的整数序列,然后结合zip()
函数遍历fruits
列表的索引和值。
2. 使用 itertools.islice()
itertools.islice()
函数用于对迭代器进行切片操作。我们可以结合enumerate()
函数遍历部分索引和值。
import itertools
fruits = ['apple', 'banana', 'cherry', 'date', 'elderberry']
for index, value in itertools.islice(enumerate(fruits), 1, 4):
print(f"Index: {index}, Value: {value}")
在这个例子中,我们使用itertools.islice(enumerate(fruits), 1, 4)
遍历了fruits
列表的部分索引和值(从索引1到索引3)。
3. 遍历其他可迭代对象
itertools
模块的函数也可以用于遍历其他可迭代对象的索引值。
import itertools
遍历元组
colors = ('red', 'green', 'blue', 'yellow', 'purple')
for index, value in itertools.islice(enumerate(colors), 2, 5):
print(f"Index: {index}, Value: {value}")
遍历字符串
text = "hello world"
for index, char in itertools.islice(enumerate(text), 3, 8):
print(f"Index: {index}, Character: {char}")
在这些例子中,我们使用itertools
模块的函数遍历了元组和字符串的部分索引和值。
六、总结
在Python中遍历索引值的方法有多种,可以使用range()
函数、enumerate()
函数、利用列表推导式等。其中,使用enumerate()
函数是最常见和推荐的方法,因为它不仅可以遍历索引,还可以直接获取相应的元素,代码更加简洁,减少出错的可能性。
此外,根据具体需求和场景,我们还可以选择其他方法,如使用range()
函数遍历索引值、使用列表推导式生成索引和值的列表、结合zip()
函数遍历多个列表、使用itertools
模块的函数遍历复杂场景中的索引值等。
通过掌握这些方法,我们可以灵活地在Python中遍历各种可迭代对象的索引值,提高代码的可读性和可维护性。
相关问答FAQs:
如何在Python中获取列表的索引值?
在Python中,您可以使用enumerate()
函数来遍历列表并获取每个元素的索引值。enumerate()
会返回一个包含索引和元素的元组,您可以通过循环直接访问这两个值。例如:
my_list = ['a', 'b', 'c']
for index, value in enumerate(my_list):
print(index, value)
在Python中,有哪些方法可以遍历字典的索引?
字典在Python中是无序的,但您仍然可以遍历其键、值和键值对。使用items()
方法可以同时访问键和值,使用keys()
和values()
方法则分别可以访问键和值。例如:
my_dict = {'name': 'Alice', 'age': 30}
for key, value in my_dict.items():
print(key, value)
如何通过索引值修改Python列表中的元素?
您可以通过索引直接访问并修改列表中的元素。例如,如果您想将列表中的第二个元素更改为新的值,可以这样做:
my_list = [10, 20, 30]
my_list[1] = 25 # 将第二个元素修改为25
print(my_list)
这种方法确保您能够轻松地根据索引值对列表进行更新。
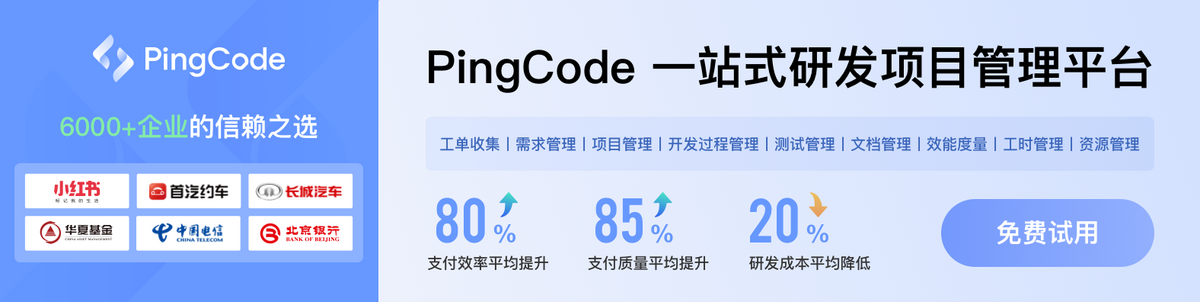