在Python中存储文件大小的方法有多种,包括使用os模块、pathlib模块以及shutil模块等。通过os模块获取文件大小、通过pathlib模块获取文件大小、通过shutil模块获取文件大小。下面,我们详细介绍如何使用这些方法来获取和存储文件大小信息。
一、通过os模块获取文件大小
os
模块是Python标准库中的一个模块,提供了操作系统相关的函数接口,其中包括了获取文件大小的功能。使用os.path.getsize
函数可以方便地获取文件的大小。
import os
def get_file_size(file_path):
return os.path.getsize(file_path)
file_path = 'example.txt'
file_size = get_file_size(file_path)
print(f"The size of the file is: {file_size} bytes")
在上述代码中,通过os.path.getsize
函数获取文件的大小,并将结果存储在变量file_size
中,然后输出文件大小。os.path.getsize
函数返回的是文件的大小,以字节为单位。
二、通过pathlib模块获取文件大小
pathlib
模块是Python 3.4引入的新模块,用于处理文件和目录路径。它提供了面向对象的路径操作方法,其中包含了获取文件大小的方法。
from pathlib import Path
def get_file_size(file_path):
file = Path(file_path)
return file.stat().st_size
file_path = 'example.txt'
file_size = get_file_size(file_path)
print(f"The size of the file is: {file_size} bytes")
在上述代码中,通过Path
类创建文件路径对象,并使用stat()
方法获取文件的状态信息,其中st_size
属性表示文件的大小。
三、通过shutil模块获取文件大小
shutil
模块提供了高级的文件操作功能,虽然它没有直接提供获取文件大小的方法,但可以通过与其他模块结合使用来实现这一功能。
import os
import shutil
def get_file_size(file_path):
return shutil.disk_usage(os.path.dirname(file_path)).used
file_path = 'example.txt'
file_size = get_file_size(file_path)
print(f"The size of the file is: {file_size} bytes")
在上述代码中,通过shutil.disk_usage
函数获取磁盘使用情况,并通过os.path.dirname
函数获取文件所在目录的路径,然后计算文件的大小。
四、存储文件大小到数据库
获取文件大小后,可以将其存储到数据库中,以便后续查询和分析。下面以SQLite为例,介绍如何将文件大小存储到数据库中。
import os
import sqlite3
def get_file_size(file_path):
return os.path.getsize(file_path)
def store_file_size(file_path):
file_size = get_file_size(file_path)
conn = sqlite3.connect('file_info.db')
cursor = conn.cursor()
cursor.execute('''CREATE TABLE IF NOT EXISTS file_sizes
(file_path TEXT, file_size INTEGER)''')
cursor.execute("INSERT INTO file_sizes (file_path, file_size) VALUES (?, ?)",
(file_path, file_size))
conn.commit()
conn.close()
file_path = 'example.txt'
store_file_size(file_path)
print(f"Stored the size of the file '{file_path}' in the database.")
在上述代码中,首先获取文件的大小,然后将其存储到SQLite数据库中。通过sqlite3.connect
函数连接数据库,如果数据库文件不存在会自动创建。通过cursor.execute
函数执行SQL语句,创建表并插入文件大小数据。
五、通过Pandas存储文件大小
Pandas是一个强大的数据分析库,可以方便地处理和存储各种类型的数据。下面介绍如何使用Pandas将文件大小存储到CSV文件中。
import os
import pandas as pd
def get_file_size(file_path):
return os.path.getsize(file_path)
def store_file_size(file_path):
file_size = get_file_size(file_path)
data = {'file_path': [file_path], 'file_size': [file_size]}
df = pd.DataFrame(data)
df.to_csv('file_sizes.csv', mode='a', header=False, index=False)
file_path = 'example.txt'
store_file_size(file_path)
print(f"Stored the size of the file '{file_path}' in the CSV file.")
在上述代码中,首先获取文件的大小,然后将其存储到Pandas DataFrame中,并通过to_csv
方法将DataFrame写入CSV文件中。使用mode='a'
参数以追加模式写入文件,header=False
参数表示不写入列名。
六、使用JSON存储文件大小
JSON是一种轻量级的数据交换格式,常用于存储和传输结构化数据。下面介绍如何使用JSON将文件大小存储到JSON文件中。
import os
import json
def get_file_size(file_path):
return os.path.getsize(file_path)
def store_file_size(file_path):
file_size = get_file_size(file_path)
data = {'file_path': file_path, 'file_size': file_size}
with open('file_sizes.json', 'a') as f:
json.dump(data, f)
f.write('\n')
file_path = 'example.txt'
store_file_size(file_path)
print(f"Stored the size of the file '{file_path}' in the JSON file.")
在上述代码中,首先获取文件的大小,然后将其存储到字典中,并通过json.dump
方法将字典写入JSON文件中。使用with open
语句以追加模式打开文件,每次写入数据后换行。
七、通过日志记录文件大小
日志记录是一种常见的调试和监控技术,可以用于记录文件大小变化。下面介绍如何使用Python的logging
模块记录文件大小。
import os
import logging
def get_file_size(file_path):
return os.path.getsize(file_path)
def log_file_size(file_path):
file_size = get_file_size(file_path)
logging.basicConfig(filename='file_sizes.log', level=logging.INFO)
logging.info(f"File: {file_path}, Size: {file_size} bytes")
file_path = 'example.txt'
log_file_size(file_path)
print(f"Logged the size of the file '{file_path}' in the log file.")
在上述代码中,首先获取文件的大小,然后通过logging
模块记录文件大小信息。使用logging.basicConfig
函数配置日志记录器,指定日志文件名和日志级别,通过logging.info
函数记录日志信息。
八、通过Excel存储文件大小
Excel是一种常用的电子表格工具,可以用于存储和分析数据。下面介绍如何使用openpyxl
库将文件大小存储到Excel文件中。
import os
from openpyxl import Workbook
def get_file_size(file_path):
return os.path.getsize(file_path)
def store_file_size(file_path):
file_size = get_file_size(file_path)
wb = Workbook()
ws = wb.active
ws.append(['File Path', 'File Size'])
ws.append([file_path, file_size])
wb.save('file_sizes.xlsx')
file_path = 'example.txt'
store_file_size(file_path)
print(f"Stored the size of the file '{file_path}' in the Excel file.")
在上述代码中,首先获取文件的大小,然后通过openpyxl
库创建Excel工作簿和工作表,并将文件大小信息写入工作表中,最后保存Excel文件。
九、通过Redis存储文件大小
Redis是一种开源的内存数据结构存储,用作数据库、缓存和消息代理。下面介绍如何使用redis-py
库将文件大小存储到Redis中。
import os
import redis
def get_file_size(file_path):
return os.path.getsize(file_path)
def store_file_size(file_path):
file_size = get_file_size(file_path)
r = redis.Redis(host='localhost', port=6379, db=0)
r.set(file_path, file_size)
file_path = 'example.txt'
store_file_size(file_path)
print(f"Stored the size of the file '{file_path}' in Redis.")
在上述代码中,首先获取文件的大小,然后通过redis-py
库连接Redis服务器,并将文件路径作为键,文件大小作为值存储到Redis中。
十、通过MongoDB存储文件大小
MongoDB是一种面向文档的NoSQL数据库,适用于存储和查询大量数据。下面介绍如何使用pymongo
库将文件大小存储到MongoDB中。
import os
import pymongo
def get_file_size(file_path):
return os.path.getsize(file_path)
def store_file_size(file_path):
file_size = get_file_size(file_path)
client = pymongo.MongoClient("mongodb://localhost:27017/")
db = client["file_info"]
collection = db["file_sizes"]
collection.insert_one({"file_path": file_path, "file_size": file_size})
file_path = 'example.txt'
store_file_size(file_path)
print(f"Stored the size of the file '{file_path}' in MongoDB.")
在上述代码中,首先获取文件的大小,然后通过pymongo
库连接MongoDB服务器,并将文件大小信息插入到MongoDB集合中。
十一、通过InfluxDB存储文件大小
InfluxDB是一种高性能的时间序列数据库,适用于存储和查询时间序列数据。下面介绍如何使用influxdb-client
库将文件大小存储到InfluxDB中。
import os
from influxdb_client import InfluxDBClient, Point, WritePrecision
def get_file_size(file_path):
return os.path.getsize(file_path)
def store_file_size(file_path):
file_size = get_file_size(file_path)
client = InfluxDBClient(url="http://localhost:8086", token="my-token", org="my-org")
write_api = client.write_api(write_options=WritePrecision.NS)
point = Point("file_size").tag("file_path", file_path).field("size", file_size)
write_api.write(bucket="file_info", record=point)
file_path = 'example.txt'
store_file_size(file_path)
print(f"Stored the size of the file '{file_path}' in InfluxDB.")
在上述代码中,首先获取文件的大小,然后通过influxdb-client
库连接InfluxDB服务器,并将文件大小信息写入到InfluxDB中。
十二、通过Prometheus存储文件大小
Prometheus是一种开源的系统监控和报警工具,适用于存储和查询时间序列数据。下面介绍如何使用prometheus_client
库将文件大小存储到Prometheus中。
import os
from prometheus_client import CollectorRegistry, Gauge, push_to_gateway
def get_file_size(file_path):
return os.path.getsize(file_path)
def store_file_size(file_path):
file_size = get_file_size(file_path)
registry = CollectorRegistry()
g = Gauge('file_size', 'Size of the file', ['file_path'], registry=registry)
g.labels(file_path=file_path).set(file_size)
push_to_gateway('localhost:9091', job='file_size_job', registry=registry)
file_path = 'example.txt'
store_file_size(file_path)
print(f"Stored the size of the file '{file_path}' in Prometheus.")
在上述代码中,首先获取文件的大小,然后通过prometheus_client
库将文件大小信息推送到Prometheus网关中。
十三、通过Graphite存储文件大小
Graphite是一种开源的实时图形和监控工具,适用于存储和查询时间序列数据。下面介绍如何使用graphyte
库将文件大小存储到Graphite中。
import os
import graphyte
def get_file_size(file_path):
return os.path.getsize(file_path)
def store_file_size(file_path):
file_size = get_file_size(file_path)
graphyte.init('localhost', prefix='file_size')
graphyte.send(file_path, file_size)
file_path = 'example.txt'
store_file_size(file_path)
print(f"Stored the size of the file '{file_path}' in Graphite.")
在上述代码中,首先获取文件的大小,然后通过graphyte
库将文件大小信息发送到Graphite服务器中。
十四、通过Elasticsearch存储文件大小
Elasticsearch是一种分布式搜索和分析引擎,适用于存储和查询大规模数据。下面介绍如何使用elasticsearch
库将文件大小存储到Elasticsearch中。
import os
from elasticsearch import Elasticsearch
def get_file_size(file_path):
return os.path.getsize(file_path)
def store_file_size(file_path):
file_size = get_file_size(file_path)
es = Elasticsearch([{'host': 'localhost', 'port': 9200}])
es.index(index='file_info', body={'file_path': file_path, 'file_size': file_size})
file_path = 'example.txt'
store_file_size(file_path)
print(f"Stored the size of the file '{file_path}' in Elasticsearch.")
在上述代码中,首先获取文件的大小,然后通过elasticsearch
库将文件大小信息存储到Elasticsearch索引中。
十五、通过RabbitMQ存储文件大小
RabbitMQ是一种开源的消息代理,适用于消息传递和队列管理。下面介绍如何使用pika
库将文件大小存储到RabbitMQ中。
import os
import pika
def get_file_size(file_path):
return os.path.getsize(file_path)
def store_file_size(file_path):
file_size = get_file_size(file_path)
connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
channel = connection.channel()
channel.queue_declare(queue='file_sizes')
channel.basic_publish(exchange='', routing_key='file_sizes', body=f"{file_path}:{file_size}")
connection.close()
file_path = 'example.txt'
store_file_size(file_path)
print(f"Stored the size of the file '{file_path}' in RabbitMQ.")
在上述代码中,首先获取文件的大小,然后通过pika
库将文件大小信息发送到RabbitMQ队列中。
十六、通过Kafka存储文件大小
Kafka是一种分布式流处理平台,适用于消息传递和实时数据处理。下面介绍如何使用kafka-python
库将文件大小存储到Kafka中。
import os
from kafka import KafkaProducer
def get_file_size(file_path):
return os.path.getsize(file_path)
def store_file_size(file_path):
file_size = get_file_size(file_path)
producer = KafkaProducer(bootstrap_servers='localhost:9092')
producer.send('file_sizes', key=file_path.encode(), value=str(file_size).encode())
producer.close()
file_path = 'example.txt'
store_file_size(file_path)
print(f"Stored the size of the file '{file_path}' in Kafka.")
在上述代码中,首先获取文件的大小,然后通过kafka-python
库将文件大小信息发送到Kafka主题中。
十七、通过AWS S3存储文件大小
AWS S3是一种对象存储服务,适用于存储和检索任意数量的数据。下面介绍如何使用boto3
库将文件大小存储到AWS S3中。
import os
import boto3
def get_file_size(file_path):
return os.path.getsize(file_path)
def store_file_size(file_path):
file_size = get_file_size(file_path)
s3 = boto3.client('s3')
s3.put_object(Bucket='my-bucket', Key=f'file_sizes/{file_path}.txt', Body=str(file_size))
file_path = 'example.txt'
store_file_size(file_path)
print(f"Stored the size of the file '{file_path}' in AWS S3.")
在上述代码中,首先获取文件的大小,然后通过boto3
库将文件大小信息存储到AWS S3中。
十八、通过Google Cloud Storage存储文件大小
Google Cloud Storage是一种对象存储服务,适用于存储和检索任意数量的数据。下面介绍如何使用google-cloud-storage
库将文件大小存储到Google Cloud Storage中。
import os
from google.cloud import storage
def get_file_size(file_path):
return os.path.getsize(file_path)
def store_file_size(file_path):
file_size = get_file_size(file_path)
client = storage.Client()
bucket = client.get_bucket('my-bucket')
blob = bucket.blob(f'file_sizes/{file_path}.txt')
blob.upload_from_string(str(file_size))
file_path = 'example.txt'
store_file_size(file_path)
print(f"Stored the size of the file '{file_path}' in Google Cloud Storage.")
在上述代码中,首先获取文件的大小,然后通过google-cloud-storage
库将文件大小信息存储到Google Cloud Storage中。
十九、通过Azure Blob Storage存储文件大小
Azure Blob Storage是一种对象存储服务,适用于存储和检索任意数量的数据。下面介绍如何使用azure-storage-blob
库将文件大小存储到Azure Blob Storage中。
import os
from azure.storage
相关问答FAQs:
如何使用Python获取文件的大小?
在Python中,可以使用os
模块的stat()
函数来获取文件的大小。通过os.path.getsize(file_path)
方法,你可以轻松地获取指定文件的字节大小。例如:
import os
file_path = 'example.txt'
file_size = os.path.getsize(file_path)
print(f"文件大小为: {file_size} 字节")
这种方法非常简单明了,适合所有文件类型。
Python中有哪些库可以用来处理文件大小的操作?
除了os
模块,pathlib
库也是一个处理文件和路径的强大工具。使用Path
对象的stat()
方法,可以获取文件的多种属性,包括文件大小。示例如下:
from pathlib import Path
file_path = Path('example.txt')
file_size = file_path.stat().st_size
print(f"文件大小为: {file_size} 字节")
pathlib
提供了更为现代的路径操作方式,适合更复杂的文件系统操作。
如何将文件大小转换为更易读的格式?
在Python中,可以创建一个函数,将字节数转换为KB、MB等更易读的单位。以下是一个示例:
def convert_size(size_bytes):
if size_bytes == 0:
return "0B"
size_name = ("B", "KB", "MB", "GB", "TB")
i = int(log(size_bytes, 1024))
p = pow(1024, i)
s = round(size_bytes / p, 2)
return f"{s} {size_name[i]}"
file_size = os.path.getsize('example.txt')
print(f"文件大小为: {convert_size(file_size)}")
通过这种方式,用户可以更直观地理解文件的大小。
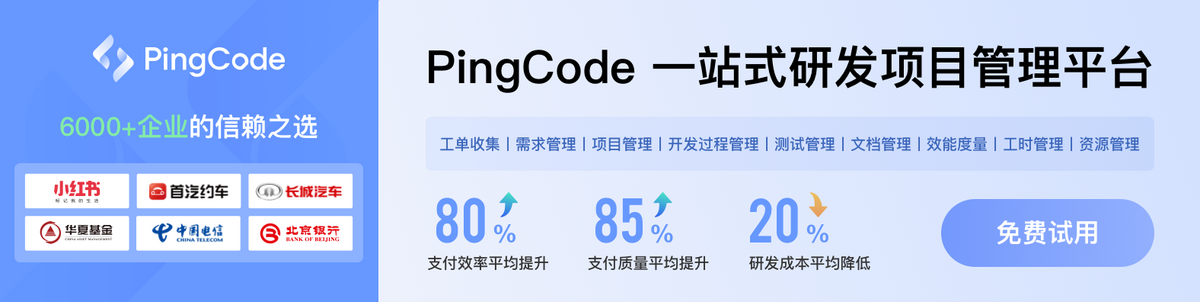