Python接口返回数据提示可以通过异常处理、日志记录、返回代码和消息、文档和注释、状态码等方式实现。其中,异常处理是特别重要的一点,它可以捕捉接口调用过程中的错误,并提供有意义的提示信息。通过使用try-except块,可以捕获特定的异常,记录错误日志,并返回用户友好的错误消息。
PYTHON接口返回数据提示的几种方式
一、异常处理
异常处理是保证程序健壮性和用户体验的关键部分。通过捕捉和处理异常,可以避免程序崩溃,并提供有意义的错误信息。
1. 捕捉和处理特定异常
在调用接口时,可能会遇到多种异常情况,如网络连接错误、请求超时、数据格式错误等。通过捕捉特定的异常,可以精确地定位和处理问题。例如:
import requests
def fetch_data_from_api(url):
try:
response = requests.get(url)
response.raise_for_status() # 检查HTTP请求是否成功
data = response.json() # 尝试解析JSON数据
return data
except requests.exceptions.RequestException as e:
print(f"请求错误: {e}")
except requests.exceptions.HTTPError as e:
print(f"HTTP错误: {e}")
except requests.exceptions.ConnectionError as e:
print(f"连接错误: {e}")
except requests.exceptions.Timeout as e:
print(f"请求超时: {e}")
except ValueError as e:
print(f"数据格式错误: {e}")
2. 使用通用异常处理
在某些情况下,可能无法预见所有可能的异常类型,可以使用一个通用的异常处理块来捕获和处理所有未预见的异常:
def fetch_data_from_api(url):
try:
response = requests.get(url)
response.raise_for_status()
data = response.json()
return data
except Exception as e:
print(f"发生了一个错误: {e}")
二、日志记录
日志记录是调试和监控接口调用过程中的重要工具。通过记录日志,可以跟踪接口调用的详细信息,帮助定位和解决问题。
1. 使用标准库logging
Python的标准库logging提供了灵活的日志记录功能,可以将日志输出到控制台、文件或其他目的地。例如:
import logging
import requests
配置日志记录
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
def fetch_data_from_api(url):
try:
response = requests.get(url)
response.raise_for_status()
data = response.json()
logging.info("成功获取数据")
return data
except requests.exceptions.RequestException as e:
logging.error(f"请求错误: {e}")
except Exception as e:
logging.error(f"发生了一个错误: {e}")
2. 自定义日志记录
可以根据需要自定义日志记录器,以便在不同的模块或组件中使用不同的日志记录设置。例如:
import logging
import requests
创建自定义日志记录器
logger = logging.getLogger('api_logger')
logger.setLevel(logging.INFO)
handler = logging.FileHandler('api_calls.log')
formatter = logging.Formatter('%(asctime)s - %(levelname)s - %(message)s')
handler.setFormatter(formatter)
logger.addHandler(handler)
def fetch_data_from_api(url):
try:
response = requests.get(url)
response.raise_for_status()
data = response.json()
logger.info("成功获取数据")
return data
except requests.exceptions.RequestException as e:
logger.error(f"请求错误: {e}")
except Exception as e:
logger.error(f"发生了一个错误: {e}")
三、返回代码和消息
在接口调用中,返回代码和消息是常见的提示方式。通过返回不同的状态码和消息,可以明确地告知调用者接口调用的结果。
1. 返回HTTP状态码和消息
在HTTP协议中,状态码和消息是标准的提示方式。例如,200表示成功,404表示资源未找到,500表示服务器内部错误等。可以在返回数据中包含状态码和消息:
import requests
def fetch_data_from_api(url):
try:
response = requests.get(url)
response.raise_for_status()
data = response.json()
return {"status_code": 200, "message": "成功", "data": data}
except requests.exceptions.RequestException as e:
return {"status_code": 400, "message": f"请求错误: {e}"}
except Exception as e:
return {"status_code": 500, "message": f"发生了一个错误: {e}"}
2. 自定义状态码和消息
在某些情况下,可能需要定义自己的状态码和消息,以便更好地符合业务需求。例如:
def fetch_data_from_api(url):
try:
response = requests.get(url)
response.raise_for_status()
data = response.json()
return {"code": 1000, "message": "成功", "data": data}
except requests.exceptions.RequestException as e:
return {"code": 1001, "message": f"请求错误: {e}"}
except Exception as e:
return {"code": 1002, "message": f"发生了一个错误: {e}"}
四、文档和注释
良好的文档和注释可以帮助开发者理解和使用接口,避免误用和错误。
1. 编写清晰的函数注释
在函数中编写清晰的注释,说明函数的用途、参数、返回值和可能的异常。例如:
def fetch_data_from_api(url):
"""
从指定的API获取数据。
参数:
url (str): API的URL。
返回:
dict: 包含状态码、消息和数据的字典。
异常:
requests.exceptions.RequestException: 如果请求失败。
Exception: 如果发生其他错误。
"""
try:
response = requests.get(url)
response.raise_for_status()
data = response.json()
return {"code": 1000, "message": "成功", "data": data}
except requests.exceptions.RequestException as e:
return {"code": 1001, "message": f"请求错误: {e}"}
except Exception as e:
return {"code": 1002, "message": f"发生了一个错误: {e}"}
2. 编写详细的文档
编写详细的文档,说明接口的使用方法、参数、返回值、示例代码等。例如,可以使用Sphinx生成API文档:
fetch_data_from_api
===================
从指定的API获取数据。
参数
----
- <strong>url</strong> (str): API的URL。
返回
----
- <strong>dict</strong>: 包含状态码、消息和数据的字典。
异常
----
- <strong>requests.exceptions.RequestException</strong>: 如果请求失败。
- <strong>Exception</strong>: 如果发生其他错误。
示例
----
.. code-block:: python
data = fetch_data_from_api("http://example.com/api")
if data["code"] == 1000:
print("成功获取数据:", data["data"])
else:
print("错误:", data["message"])
五、状态码
状态码是HTTP协议中标准的返回码,用于表示请求的结果状态。通过使用标准的状态码,可以明确地告知调用者请求的结果。
1. 常见的HTTP状态码
以下是一些常见的HTTP状态码及其含义:
- 200 OK: 请求成功。
- 201 Created: 成功创建资源。
- 204 No Content: 请求成功,但无返回内容。
- 400 Bad Request: 请求无效。
- 401 Unauthorized: 未授权。
- 403 Forbidden: 禁止访问。
- 404 Not Found: 资源未找到。
- 500 Internal Server Error: 服务器内部错误。
- 503 Service Unavailable: 服务不可用。
2. 在接口中使用状态码
在接口中返回适当的状态码,可以明确地告知调用者请求的结果。例如:
from flask import Flask, jsonify, request
app = Flask(__name__)
@app.route('/api/data', methods=['GET'])
def get_data():
try:
# 模拟数据获取
data = {"key": "value"}
return jsonify({"code": 200, "message": "成功", "data": data}), 200
except Exception as e:
return jsonify({"code": 500, "message": f"服务器内部错误: {e}"}), 500
if __name__ == '__main__':
app.run(debug=True)
六、用户友好的错误消息
提供用户友好的错误消息,可以帮助用户理解错误原因,并采取相应的措施。
1. 提供详细的错误描述
在返回错误消息时,提供详细的错误描述,可以帮助用户理解错误原因。例如:
def fetch_data_from_api(url):
try:
response = requests.get(url)
response.raise_for_status()
data = response.json()
return {"code": 200, "message": "成功", "data": data}
except requests.exceptions.ConnectionError:
return {"code": 1001, "message": "无法连接到服务器,请检查网络连接"}
except requests.exceptions.Timeout:
return {"code": 1002, "message": "请求超时,请稍后重试"}
except requests.exceptions.HTTPError as e:
return {"code": 1003, "message": f"HTTP错误: {e.response.status_code}"}
except Exception as e:
return {"code": 1004, "message": f"发生了一个错误: {e}"}
2. 提供解决建议
在错误消息中提供解决建议,可以帮助用户快速解决问题。例如:
def fetch_data_from_api(url):
try:
response = requests.get(url)
response.raise_for_status()
data = response.json()
return {"code": 200, "message": "成功", "data": data}
except requests.exceptions.ConnectionError:
return {"code": 1001, "message": "无法连接到服务器,请检查网络连接"}
except requests.exceptions.Timeout:
return {"code": 1002, "message": "请求超时,请稍后重试"}
except requests.exceptions.HTTPError as e:
return {"code": 1003, "message": f"HTTP错误: {e.response.status_code}。请检查URL是否正确"}
except Exception as e:
return {"code": 1004, "message": f"发生了一个错误: {e}。请联系技术支持"}
七、接口测试
接口测试是保证接口功能和性能的重要手段。通过自动化测试,可以及时发现和修复问题,提高接口的可靠性。
1. 使用pytest进行单元测试
pytest是一个功能强大且易于使用的测试框架,可以用于编写和执行单元测试。例如:
import pytest
from my_module import fetch_data_from_api
def test_fetch_data_from_api_success(mocker):
mocker.patch('my_module.requests.get', return_value=MockResponse(200, {"key": "value"}))
result = fetch_data_from_api("http://example.com/api")
assert result["code"] == 200
assert result["message"] == "成功"
assert result["data"] == {"key": "value"}
def test_fetch_data_from_api_connection_error(mocker):
mocker.patch('my_module.requests.get', side_effect=requests.exceptions.ConnectionError)
result = fetch_data_from_api("http://example.com/api")
assert result["code"] == 1001
assert result["message"] == "无法连接到服务器,请检查网络连接"
class MockResponse:
def __init__(self, status_code, json_data):
self.status_code = status_code
self.json_data = json_data
def raise_for_status(self):
if self.status_code != 200:
raise requests.exceptions.HTTPError(f"HTTP错误: {self.status_code}")
def json(self):
return self.json_data
2. 使用Postman进行接口测试
Postman是一个流行的API开发和测试工具,可以用于手动测试和自动化测试接口。例如,可以创建和保存请求、执行请求、查看响应、编写测试脚本等。
八、接口监控
接口监控是保证接口稳定性和性能的重要手段。通过实时监控接口的运行状况,可以及时发现和解决问题。
1. 使用Prometheus和Grafana进行监控
Prometheus是一个开源的监控和报警系统,Grafana是一个开源的可视化工具。通过将Prometheus和Grafana结合使用,可以实现接口的实时监控和可视化。例如:
# prometheus.yml
global:
scrape_interval: 15s
scrape_configs:
- job_name: 'api'
static_configs:
- targets: ['localhost:8000']
from prometheus_client import start_http_server, Counter
from flask import Flask, jsonify
app = Flask(__name__)
创建Prometheus计数器
REQUEST_COUNT = Counter('request_count', 'Total API requests')
@app.route('/api/data', methods=['GET'])
def get_data():
REQUEST_COUNT.inc() # 增加计数器
data = {"key": "value"}
return jsonify({"code": 200, "message": "成功", "data": data}), 200
if __name__ == '__main__':
start_http_server(8000) # 启动Prometheus HTTP服务器
app.run(debug=True)
2. 使用APM工具进行监控
应用性能管理(APM)工具可以提供更全面的监控和分析功能。例如,New Relic、Datadog、AppDynamics等APM工具可以监控接口的性能、错误率、响应时间等指标,帮助及时发现和解决问题。
总结
Python接口返回数据提示的方式有很多种,主要包括异常处理、日志记录、返回代码和消息、文档和注释、状态码、用户友好的错误消息、接口测试和接口监控。通过结合使用这些方法,可以有效地提升接口的健壮性和用户体验。希望本文能够帮助你更好地理解和应用这些方法,提高Python接口的开发和维护水平。
相关问答FAQs:
如何在Python接口中返回数据时提供友好的提示信息?
在Python接口中,可以使用状态码和自定义消息来提示用户。在返回数据时,可以在响应中包含一个“message”字段,提供更详细的解释。例如,若请求成功,可以返回{"status": "success", "message": "数据获取成功", "data": ...}
。这样用户能够清晰地理解接口返回的结果。
如果接口返回的数据为空,我应该如何处理?
当接口返回的数据为空时,可以设计一个统一的响应格式。例如,可以返回{"status": "success", "message": "没有找到相关数据", "data": []}
。这种方式让用户明白请求已成功,但没有找到匹配的结果,有助于提升用户体验。
在Python中如何处理错误并向用户提供反馈?
在编写Python接口时,建议使用异常处理机制来捕获可能的错误。在发生错误时,可以返回统一的错误格式,例如{"status": "error", "message": "请求参数无效", "error_code": 400}
。这种做法不仅能让用户了解具体的错误信息,还能帮助他们更好地进行调试或重新请求。
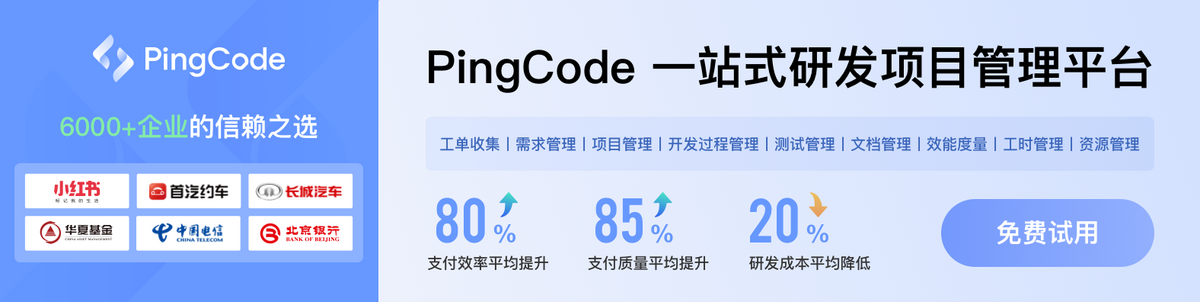