要建立一个Boot客户管理系统项目,首先需要明确项目的核心功能和技术架构。核心步骤包括:定义项目需求、选择合适的技术栈、设计数据库架构、实现基本功能模块、进行测试和部署。 其中,选择合适的技术栈非常重要,本文将详细阐述如何选择和使用Spring Boot来实现一个客户管理系统。
一、定义项目需求
在开始构建客户管理系统之前,首先需要明确系统需要实现的核心功能。通常客户管理系统需要具备以下功能:
- 客户信息管理:包括客户的新增、删除、修改、查询等基本操作。
- 客户状态跟踪:记录客户的状态变化,例如潜在客户、正式客户、流失客户等。
- 客户关系管理:跟踪客户的互动记录,例如电话记录、邮件记录等。
- 报表和分析:生成客户数据的统计报表,帮助企业进行数据分析和决策。
明确这些需求后,可以进一步细化每个功能的具体实现。
二、选择合适的技术栈
要构建一个现代化的客户管理系统,选择合适的技术栈是至关重要的一步。Spring Boot 是一个非常流行的Java框架,适合构建企业级应用。以下是推荐的技术栈:
- Spring Boot:用于快速构建Java应用,简化配置。
- Spring Data JPA:用于数据持久化,简化数据库操作。
- Thymeleaf:用于前端页面渲染。
- Spring Security:用于权限管理和认证。
- H2/MySQL:作为数据库,H2用于开发测试环境,MySQL用于生产环境。
- Maven/Gradle:用于项目构建和管理依赖。
选择这些技术栈可以大大简化开发流程,提高开发效率。
三、设计数据库架构
在设计数据库架构时,需要根据业务需求设计合理的数据库表结构。以下是一个简单的客户管理系统的数据库表设计示例:
CREATE TABLE Customer (
id BIGINT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(255) NOT NULL,
email VARCHAR(255) NOT NULL,
phone VARCHAR(20),
status VARCHAR(50),
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
CREATE TABLE Interaction (
id BIGINT AUTO_INCREMENT PRIMARY KEY,
customer_id BIGINT,
interaction_type VARCHAR(50),
interaction_date TIMESTAMP,
notes TEXT,
FOREIGN KEY (customer_id) REFERENCES Customer(id)
);
这个设计包括了客户基本信息表 Customer
和客户互动记录表 Interaction
,并通过外键关联。
四、实现基本功能模块
在明确需求和设计好数据库架构后,可以开始实现基本功能模块。以下是基于Spring Boot的一些示例代码。
- 项目结构
首先,创建一个新的Spring Boot项目,项目结构如下:
src
├── main
│ ├── java
│ │ └── com.example.crm
│ │ ├── CrmApplication.java
│ │ ├── controller
│ │ ├── model
│ │ ├── repository
│ │ ├── service
│ │ └── util
│ └── resources
│ ├── application.properties
│ ├── static
│ └── templates
└── test
- 定义实体类
在 model
包下定义实体类:
package com.example.crm.model;
import javax.persistence.*;
import java.time.LocalDateTime;
@Entity
public class Customer {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String email;
private String phone;
private String status;
private LocalDateTime createdAt;
// Getters and Setters
}
@Entity
public class Interaction {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@ManyToOne
@JoinColumn(name = "customer_id")
private Customer customer;
private String interactionType;
private LocalDateTime interactionDate;
private String notes;
// Getters and Setters
}
- 定义仓库接口
在 repository
包下定义仓库接口:
package com.example.crm.repository;
import com.example.crm.model.Customer;
import org.springframework.data.jpa.repository.JpaRepository;
public interface CustomerRepository extends JpaRepository<Customer, Long> {
}
package com.example.crm.repository;
import com.example.crm.model.Interaction;
import org.springframework.data.jpa.repository.JpaRepository;
public interface InteractionRepository extends JpaRepository<Interaction, Long> {
}
- 实现服务层
在 service
包下实现服务层:
package com.example.crm.service;
import com.example.crm.model.Customer;
import com.example.crm.repository.CustomerRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class CustomerService {
@Autowired
private CustomerRepository customerRepository;
public List<Customer> getAllCustomers() {
return customerRepository.findAll();
}
public Customer getCustomerById(Long id) {
return customerRepository.findById(id).orElse(null);
}
public Customer createCustomer(Customer customer) {
return customerRepository.save(customer);
}
public Customer updateCustomer(Long id, Customer customerDetails) {
Customer customer = customerRepository.findById(id).orElse(null);
if (customer != null) {
customer.setName(customerDetails.getName());
customer.setEmail(customerDetails.getEmail());
customer.setPhone(customerDetails.getPhone());
customer.setStatus(customerDetails.getStatus());
return customerRepository.save(customer);
}
return null;
}
public void deleteCustomer(Long id) {
customerRepository.deleteById(id);
}
}
- 实现控制层
在 controller
包下实现控制层:
package com.example.crm.controller;
import com.example.crm.model.Customer;
import com.example.crm.service.CustomerService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.*;
@Controller
@RequestMapping("/customers")
public class CustomerController {
@Autowired
private CustomerService customerService;
@GetMapping
public String getAllCustomers(Model model) {
model.addAttribute("customers", customerService.getAllCustomers());
return "customerList";
}
@GetMapping("/new")
public String showCreateCustomerForm(Model model) {
model.addAttribute("customer", new Customer());
return "customerForm";
}
@PostMapping
public String createCustomer(@ModelAttribute Customer customer) {
customerService.createCustomer(customer);
return "redirect:/customers";
}
@GetMapping("/{id}/edit")
public String showEditCustomerForm(@PathVariable Long id, Model model) {
model.addAttribute("customer", customerService.getCustomerById(id));
return "customerForm";
}
@PostMapping("/{id}")
public String updateCustomer(@PathVariable Long id, @ModelAttribute Customer customer) {
customerService.updateCustomer(id, customer);
return "redirect:/customers";
}
@GetMapping("/{id}/delete")
public String deleteCustomer(@PathVariable Long id) {
customerService.deleteCustomer(id);
return "redirect:/customers";
}
}
- 配置应用程序
在 application.properties
文件中配置数据库连接和其他设置:
spring.datasource.url=jdbc:h2:mem:testdb
spring.datasource.driverClassName=org.h2.Driver
spring.datasource.username=sa
spring.datasource.password=password
spring.jpa.database-platform=org.hibernate.dialect.H2Dialect
spring.h2.console.enabled=true
- 创建前端页面
在 templates
目录下创建前端页面:
customerList.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Customer List</title>
</head>
<body>
<h1>Customer List</h1>
<table>
<tr>
<th>ID</th>
<th>Name</th>
<th>Email</th>
<th>Phone</th>
<th>Status</th>
<th>Actions</th>
</tr>
<tr th:each="customer : ${customers}">
<td th:text="${customer.id}">1</td>
<td th:text="${customer.name}">John Doe</td>
<td th:text="${customer.email}">john@example.com</td>
<td th:text="${customer.phone}">123-456-7890</td>
<td th:text="${customer.status}">Active</td>
<td>
<a th:href="@{/customers/{id}/edit(id=${customer.id})}">Edit</a>
<a th:href="@{/customers/{id}/delete(id=${customer.id})}">Delete</a>
</td>
</tr>
</table>
<a href="/customers/new">Add New Customer</a>
</body>
</html>
customerForm.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Customer Form</title>
</head>
<body>
<h1>Customer Form</h1>
<form th:action="@{/customers}" th:object="${customer}" method="post">
<input type="hidden" th:field="*{id}"/>
<label>Name: <input type="text" th:field="*{name}"/></label><br/>
<label>Email: <input type="text" th:field="*{email}"/></label><br/>
<label>Phone: <input type="text" th:field="*{phone}"/></label><br/>
<label>Status: <input type="text" th:field="*{status}"/></label><br/>
<button type="submit">Save</button>
</form>
<a href="/customers">Back to List</a>
</body>
</html>
五、进行测试和部署
- 进行测试
确保所有功能模块都经过充分的测试,包括单元测试和集成测试。可以使用JUnit和Spring Boot Test进行测试。
package com.example.crm;
import com.example.crm.model.Customer;
import com.example.crm.service.CustomerService;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import static org.junit.jupiter.api.Assertions.assertEquals;
import static org.junit.jupiter.api.Assertions.assertNotNull;
@SpringBootTest
class CrmApplicationTests {
@Autowired
private CustomerService customerService;
@Test
void testCreateCustomer() {
Customer customer = new Customer();
customer.setName("John Doe");
customer.setEmail("john@example.com");
customer.setPhone("123-456-7890");
customer.setStatus("Active");
Customer savedCustomer = customerService.createCustomer(customer);
assertNotNull(savedCustomer);
assertEquals("John Doe", savedCustomer.getName());
}
}
- 部署
在完成测试后,可以将项目打包并部署到服务器上。Spring Boot提供了简便的方式打包应用:
mvn clean package
生成的JAR文件可以直接在服务器上运行:
java -jar target/crm-0.0.1-SNAPSHOT.jar
六、总结
通过以上步骤,我们详细阐述了如何构建一个基于Spring Boot的客户管理系统项目。关键步骤包括定义项目需求、选择技术栈、设计数据库架构、实现基本功能模块、进行测试和部署。希望这些内容对你构建客户管理系统有所帮助。
相关问答FAQs:
如何选择合适的项目管理工具来配合boot客户管理系统?
在选择项目管理工具时,考虑工具的易用性、与boot客户管理系统的兼容性、团队成员的熟悉程度以及功能需求。一个理想的工具应能够支持任务分配、进度跟踪和团队协作,同时与客户管理系统的数据无缝集成,以提升整体工作效率。
在boot客户管理系统中,如何有效地跟踪项目进展?
有效跟踪项目进展的关键在于设定清晰的里程碑和任务分配。确保每个任务都有明确的负责人和截止日期,并定期更新状态。利用系统内置的报告功能,定期检查进度,并根据需要调整计划,以确保项目按时完成。
如何在boot客户管理系统中管理客户与项目之间的关系?
在管理客户与项目的关系时,可以通过创建项目档案与客户信息的关联来实现。记录客户需求、反馈和项目进度,确保所有团队成员都能访问这些信息。定期与客户沟通,更新项目状态,并根据客户的反馈及时调整项目方向,以提高客户满意度。
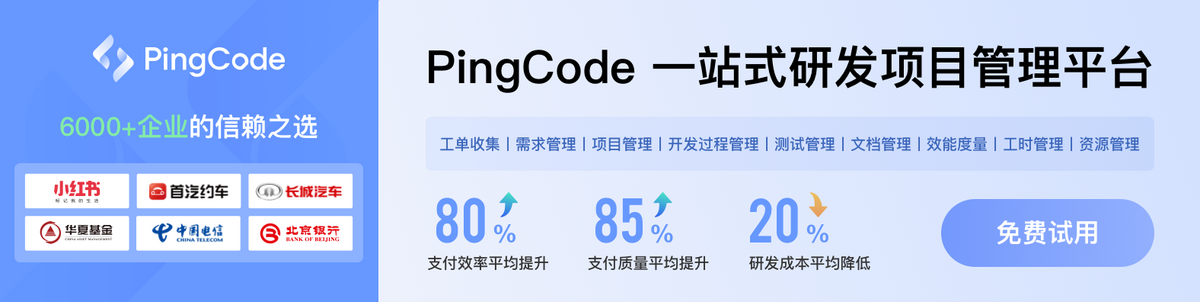