JavaScript 中的 sort
方法是用于对数组的元素进行排序的功能。数组的排序是根据字符串Unicode码点进行的。这表示,首先,所有被排序的元素会先被转换为字符串,接着根据字符串的字符编码的数值大小进行排序。默认情况下,sort
函数会以字典顺序对数字进行排序,这可能会导致直觉上的“错误”结果。例如,数字数组 [10, 21, 2] 使用默认的 sort
方法排序后将会得到 [10, 2, 21],显然这并不是数值大小的排序。为了让 sort
方法按照数值大小排序,可以向 sort
方法传递一个比较函数。
详细地说,比较函数应当接收两个参数(我们通常命名为 a 和 b),然后根据返回值来决定这两个参数在排序后的顺序。如果比较函数返回一个小于0的值,那么 a
会被排在 b
之前;返回0时,a
和 b
的顺序不变;返回一个大于0的值,则 b
会被排在 a
之前。这样,通过自定义比较函数,可以实现数值排序、按长度排序甚至是完全自定义的排序逻辑。
一、SORT方法的基本用法
JavaScript 的 sort
方法是定义在数组的原型上,这意味着只有数组类型的对象可以直接使用此方法。排序的基本方法非常简单:只需调用数组对象的 sort
方法即可。如果不提供任何参数,sort
方法将会根据字符编码的顺序对数组元素进行排序。
由于 sort
方法默认会将所有元素转换为字符串然后比较,这种行为在排序数字时往往不符合预期。下面是一个例子:
let numbers = [3, 1, 4, 1, 5, 9];
numbers.sort();
console.log(numbers); // 输出: [1, 1, 3, 4, 5, 9]
在这个例子中,尽管结果看起来是正确的,但这是因为数字转换为字符串后的排序恰好和它们的数值大小排序相符。对于一些特定的数字数组,这种巧合可能不会发生。
二、自定义比较函数
为了实现按照数值大小对数字进行排序,我们需要向 sort
方法提供一个比较函数。比较函数的设计应该根据元素之间比较的逻辑来编写。
比如,按照数值大小排序的比较函数可以这样写:
function numberCompare(a, b) {
return a - b;
}
使用这个比较函数进行排序如下:
let numbers = [10, 21, 2];
numbers.sort(numberCompare);
console.log(numbers); // 输出: [2, 10, 21]
在这个例子中,由于比较函数正确地返回了两个数之间的差值,sort
方法能够按照数值大小进行正确排序。
三、SORT方法排序的稳定性
排序的稳定性是指排序算法在排序具有相等关键字的元素时,能否保持这些元素原有的相对顺序。从 ES2019 开始,JavaScript 规范要求 sort
方法必须是稳定的。这意味着,例如,在一个对象数组中,如果两个对象的某个属性相同,它们原有的顺序将会被 sort
方法保留。
稳定性对于一些特定的排序任务来说是非常重要的。例如,若一个列表已经根据一个属性排序,接着需要根据另一个属性进行次级排序,稳定的排序算法能够保证第一次排序的结果不会因第二次排序而被打乱。
四、复杂数据结构的排序
对于复杂的数据结构,如对象或数组的数组,我们通常需要根据对象的某个属性或数组的某个元素来排序。这时,比较函数的编写方式需要更为灵活和精确。
此时,比较函数可能需要更复杂的逻辑来比较两个对象:
let people = [
{name: "John", age: 30},
{name: "Jane", age: 25},
{name: "Gary", age: 28},
];
people.sort(function(a, b) {
return a.age - b.age;
});
console.log(people);
在这个例子中,比较函数根据 age
属性进行排序,从而实现了根据年龄对对象数组进行排序的目的。
相关问答FAQs:
FAQs about sorting in JavaScript
1. How does the JavaScript sort() method work?
The sort() method in JavaScript is used to sort elements in an array in ascending order by default. It reorders the elements of the array in place, meaning it modifies the original array. The sorting order is determined by converting the elements into strings and comparing their Unicode values. However, this default sorting behavior may not always produce the desired results when sorting numbers or non-ASCII characters. In such cases, a custom sorting function needs to be provided.
2. How can I sort an array of numbers in descending order using JavaScript?
To sort an array of numbers in descending order, you can use the sort() method with a custom sorting function. The custom sorting function should compare two numbers and return a negative value if the first number should be sorted before the second, a positive value if the first number should be sorted after the second, or zero if the two numbers are equal. By subtracting the second number from the first, you can achieve the descending order. Here's an example:
let numbers = [5, 2, 8, 1, 9];
numbers.sort((a, b) => b - a);
console.log(numbers); // Output: [9, 8, 5, 2, 1]
3. Can the JavaScript sort() method handle sorting of complex objects?
Yes, the sort() method in JavaScript can handle sorting of complex objects. To sort an array of objects based on a specific property, you can provide a custom sorting function that compares the desired property of two objects. For example, let's say you have an array of objects representing books, and you want to sort them based on their release year in ascending order:
let books = [
{ title: "Book A", releaseYear: 2000 },
{ title: "Book B", releaseYear: 1995 },
{ title: "Book C", releaseYear: 2010 }
];
books.sort((a, b) => a.releaseYear - b.releaseYear);
console.log(books);
// Output: [{ title: "Book B", releaseYear: 1995 }, { title: "Book A", releaseYear: 2000 }, { title: "Book C", releaseYear: 2010 }]
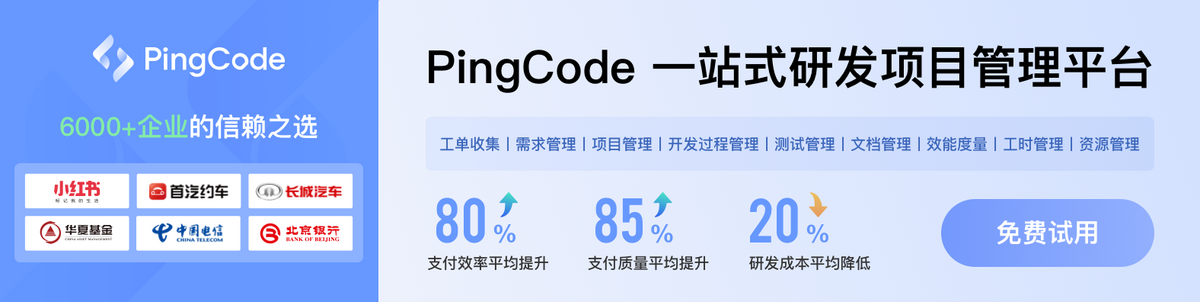