在Python中实现多线程的主要方法有:使用threading
模块、使用concurrent.futures
模块、以及使用multiprocessing
模块。threading
模块提供了对线程的基本支持、concurrent.futures
提供了更高层的接口、而multiprocessing
则适用于需要并行处理的场景。 其中,threading
模块是最常用的方法之一,因为它直接支持多线程并提供了线程同步的功能。在此,我们详细探讨如何使用threading
模块来实现多线程。
一、THREADING模块的基本用法
threading
模块是Python标准库的一部分,它为Python提供了线程支持。使用threading
模块可以轻松地创建和管理线程。
- 创建线程
在threading
模块中,创建线程通常有两种方式:通过实例化Thread
类或通过继承Thread
类并重写run
方法。
通过实例化Thread
类创建线程:
import threading
def worker():
print("Thread is running")
创建线程
thread = threading.Thread(target=worker)
启动线程
thread.start()
等待线程完成
thread.join()
通过继承Thread
类创建线程:
import threading
class MyThread(threading.Thread):
def run(self):
print("Thread is running")
创建线程
thread = MyThread()
启动线程
thread.start()
等待线程完成
thread.join()
- 线程同步
在多线程编程中,线程同步是一个重要的话题。Python提供了多种同步机制,如锁(Lock)、条件(Condition)、事件(Event)等。
使用锁进行同步:
import threading
lock = threading.Lock()
shared_resource = 0
def increment():
global shared_resource
lock.acquire()
try:
shared_resource += 1
finally:
lock.release()
创建多个线程
threads = [threading.Thread(target=increment) for _ in range(10)]
启动所有线程
for thread in threads:
thread.start()
等待所有线程完成
for thread in threads:
thread.join()
print("Shared resource value:", shared_resource)
二、CONCURRENT.FUTURES模块的高级用法
concurrent.futures
模块提供了更高层次的接口来管理线程和进程。它提供了ThreadPoolExecutor
类来管理线程池。
- 使用ThreadPoolExecutor
ThreadPoolExecutor
允许我们轻松地在多个线程中并行执行任务,并收集结果。
from concurrent.futures import ThreadPoolExecutor
def worker(n):
return n * n
创建线程池
with ThreadPoolExecutor(max_workers=5) as executor:
# 提交任务并收集结果
futures = [executor.submit(worker, i) for i in range(10)]
results = [future.result() for future in futures]
print("Results:", results)
- 管理线程池
ThreadPoolExecutor
还提供了一些高级功能,如管理线程池大小、处理异常等。
from concurrent.futures import ThreadPoolExecutor, as_completed
def worker(n):
if n == 5:
raise ValueError("Invalid value")
return n * n
创建线程池
with ThreadPoolExecutor(max_workers=5) as executor:
futures = [executor.submit(worker, i) for i in range(10)]
for future in as_completed(futures):
try:
result = future.result()
print("Result:", result)
except Exception as e:
print("Exception:", e)
三、MULTIPROCESSING模块与多线程的比较
虽然multiprocessing
模块主要用于多进程编程,但在某些情况下,它也可以用于多线程编程。特别是当需要完全绕过Python的全局解释器锁(GIL)时,multiprocessing
可能是一个更好的选择。
- 使用multiprocessing模块
multiprocessing
模块的接口与threading
模块非常相似,但它创建的是进程而不是线程。
from multiprocessing import Process
def worker():
print("Process is running")
创建进程
process = Process(target=worker)
启动进程
process.start()
等待进程完成
process.join()
- 进程池
multiprocessing
模块还提供了Pool
类,用于管理进程池。与ThreadPoolExecutor
类似,Pool
允许我们并行地运行多个进程。
from multiprocessing import Pool
def worker(n):
return n * n
创建进程池
with Pool(processes=5) as pool:
results = pool.map(worker, range(10))
print("Results:", results)
四、实际应用中的多线程
在实际应用中,多线程常用于IO密集型任务,如网络请求、文件读写等。以下是一些多线程的实际应用场景。
- 网络请求
在需要发起多个网络请求时,可以使用多线程来加快请求速度。
import threading
import requests
urls = [
"http://example.com",
"http://example.org",
"http://example.net",
]
def fetch(url):
response = requests.get(url)
print(f"Fetched {url} with status {response.status_code}")
threads = [threading.Thread(target=fetch, args=(url,)) for url in urls]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
- 文件处理
多线程可以用于同时处理多个文件,如读取、写入或数据转换。
import threading
def read_file(file_path):
with open(file_path, 'r') as file:
data = file.read()
print(f"Read {file_path}")
file_paths = ["file1.txt", "file2.txt", "file3.txt"]
threads = [threading.Thread(target=read_file, args=(file_path,)) for file_path in file_paths]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
五、线程安全与最佳实践
在多线程编程中,线程安全是一个关键问题。以下是一些确保线程安全的最佳实践。
- 使用线程安全的数据结构
Python的queue
模块提供了线程安全的队列,如Queue
、LifoQueue
、PriorityQueue
等。
import threading
import queue
q = queue.Queue()
def producer():
for i in range(5):
q.put(i)
print(f"Produced {i}")
def consumer():
while not q.empty():
item = q.get()
print(f"Consumed {item}")
prod_thread = threading.Thread(target=producer)
cons_thread = threading.Thread(target=consumer)
prod_thread.start()
cons_thread.start()
prod_thread.join()
cons_thread.join()
- 避免死锁
在使用多个锁时,确保锁的获取顺序是固定的,以避免死锁。
import threading
lock1 = threading.Lock()
lock2 = threading.Lock()
def task1():
with lock1:
with lock2:
print("Task 1")
def task2():
with lock2:
with lock1:
print("Task 2")
thread1 = threading.Thread(target=task1)
thread2 = threading.Thread(target=task2)
thread1.start()
thread2.start()
thread1.join()
thread2.join()
- 减少锁的使用
尽量减少锁的使用,避免长时间持有锁,从而提高性能。
import threading
lock = threading.Lock()
counter = 0
def increment():
global counter
for _ in range(1000):
with lock:
counter += 1
threads = [threading.Thread(target=increment) for _ in range(10)]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
print("Counter:", counter)
通过这些方法和实践,你可以在Python中实现高效的多线程程序,充分利用多核处理器的优势,提高程序的并发性能。
相关问答FAQs:
在Python中多线程的主要用途是什么?
Python中的多线程主要用于处理I/O密集型任务,如网络请求、文件操作或数据库交互。通过多线程,可以让程序在等待某个操作完成时,继续执行其他任务,从而提高程序的整体效率。在CPU密集型任务中,由于GIL(全局解释器锁)的存在,多线程的效果可能不如多进程。
如何在Python中创建和管理线程?
在Python中,可以使用threading
模块来创建和管理线程。首先,需要定义一个继承自threading.Thread
的类或使用threading.Thread
类的实例。通过重写run()
方法来实现线程要执行的任务。启动线程时,可以调用start()
方法,而通过join()
方法可以等待线程结束。这种方式可以让你更好地控制线程的生命周期。
使用多线程时需要注意哪些问题?
在使用多线程时,需特别注意线程安全问题。多个线程同时访问共享资源可能导致数据不一致或程序崩溃。可以使用锁(threading.Lock
)来保护共享资源的访问。此外,还应关注线程的创建和销毁开销,过多的线程可能导致性能下降。合理的线程数量与适当的任务划分对于提高程序性能至关重要。
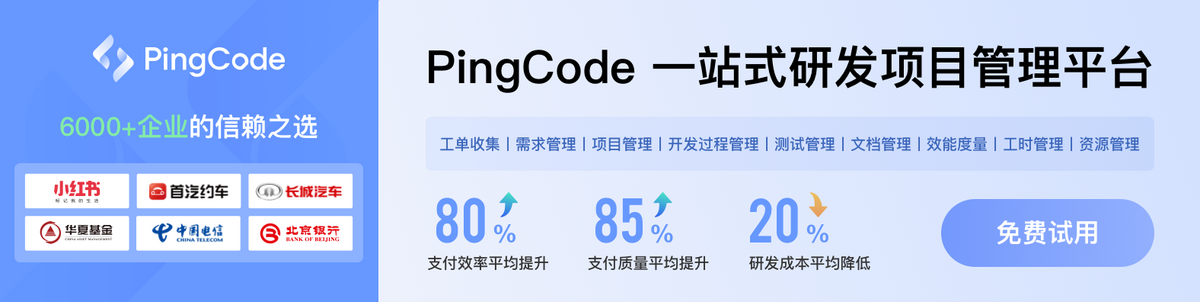