Python中可以通过使用os
模块的system
函数调用系统命令、使用subprocess
模块执行ping命令、使用scapy
库进行网络包发送。这三种方法各有优势,os.system
方法简单直接,但不适合获取和解析输出结果;subprocess
模块提供了更灵活的进程管理和输出捕获功能;scapy
库适合需要更底层网络操作的场合。接下来,我将详细介绍这三种方法。
一、使用OS模块
Python的os
模块提供了与操作系统交互的功能。通过os.system
方法,我们可以直接调用系统的ping命令。这种方法简单快捷,但无法获取ping命令的返回结果进行进一步处理。
- 使用
os.system
进行ping
使用os.system
可以快速调用系统命令来执行ping操作。其优点是简单直接,但缺点是无法获取和处理命令的输出。
import os
def ping_ip(ip_address):
response = os.system(f"ping -c 1 {ip_address}")
if response == 0:
print(f"{ip_address} is reachable.")
else:
print(f"{ip_address} is not reachable.")
ping_ip("8.8.8.8")
在这个例子中,我们通过os.system
来执行ping命令,并通过返回值判断目标IP是否可达。返回值为0表示目标IP可达,否则不可达。
二、使用Subprocess模块
subprocess
模块是Python中用于执行外部命令的强大工具。通过subprocess
,我们可以更灵活地管理子进程,并且能够捕获和处理命令的输出。
- 使用
subprocess.run
执行ping并获取输出
subprocess.run
方法可以执行外部命令,并返回一个CompletedProcess
实例,包含命令执行的结果信息。
import subprocess
def ping_ip(ip_address):
try:
result = subprocess.run(["ping", "-c", "1", ip_address], capture_output=True, text=True, check=True)
print(f"{ip_address} is reachable.")
print("Output:\n", result.stdout)
except subprocess.CalledProcessError:
print(f"{ip_address} is not reachable.")
ping_ip("8.8.8.8")
在这个例子中,我们通过subprocess.run
来执行ping命令,并使用capture_output=True
来捕获命令的输出,text=True
将输出转换为字符串格式。check=True
会在命令返回非零状态码时抛出CalledProcessError
异常。
- 处理命令输出
通过解析ping命令的输出,我们可以提取更详细的信息,例如响应时间、丢包率等。
import subprocess
import re
def ping_ip(ip_address):
try:
result = subprocess.run(["ping", "-c", "1", ip_address], capture_output=True, text=True, check=True)
print(f"{ip_address} is reachable.")
# 解析输出提取响应时间
match = re.search(r'time=(\d+\.?\d*) ms', result.stdout)
if match:
print(f"Response time: {match.group(1)} ms")
else:
print("Could not parse response time.")
except subprocess.CalledProcessError:
print(f"{ip_address} is not reachable.")
ping_ip("8.8.8.8")
在这个例子中,我们使用正则表达式从ping命令的输出中提取响应时间。通过解析输出,我们可以获取更详细的网络信息。
三、使用Scapy库
scapy
是Python中一个强大的网络包处理库,适合需要更底层网络操作的场合。通过scapy
,我们可以构造、发送和接收网络包。
- 使用
scapy
进行ICMP包发送
通过scapy
,我们可以手动构造ICMP请求包,并发送到目标IP,接收ICMP响应包。
from scapy.all import *
def ping_ip(ip_address):
icmp_request = IP(dst=ip_address)/ICMP()
response = sr1(icmp_request, timeout=2, verbose=0)
if response:
print(f"{ip_address} is reachable.")
print(f"Response time: {response.time - icmp_request.sent_time} seconds")
else:
print(f"{ip_address} is not reachable.")
ping_ip("8.8.8.8")
在这个例子中,我们使用scapy
构造了一个ICMP请求包,并发送到目标IP。如果接收到响应包,则目标IP可达,并计算响应时间。
- 捕获并处理ICMP响应
通过捕获和处理ICMP响应包,我们可以获取更详细的网络信息,例如TTL值、响应时间等。
from scapy.all import *
def ping_ip(ip_address):
icmp_request = IP(dst=ip_address)/ICMP()
response = sr1(icmp_request, timeout=2, verbose=0)
if response:
print(f"{ip_address} is reachable.")
print(f"Response time: {response.time - icmp_request.sent_time} seconds")
print(f"TTL: {response.ttl}")
else:
print(f"{ip_address} is not reachable.")
ping_ip("8.8.8.8")
在这个例子中,我们从ICMP响应包中提取了TTL值和响应时间,通过这些信息可以对目标IP的网络状况进行进一步分析。
总结
在Python中,通过os
模块、subprocess
模块和scapy
库,我们可以实现对IP地址的ping操作。os.system
方法简单直接,但功能有限;subprocess
模块提供了更灵活的进程管理和输出捕获功能;scapy
库适合需要更底层网络操作的场合。根据具体需求选择合适的方法,可以有效地实现网络连通性检查和分析。
相关问答FAQs:
如何使用Python实现IP地址的Ping操作?
使用Python进行IP地址的Ping操作,最常用的方法是利用subprocess
模块。通过该模块,可以在Python中执行系统命令,包括ping
命令。具体步骤包括导入模块、构建Ping命令以及处理返回结果。可以参考以下代码示例:
import subprocess
def ping_ip(ip):
command = ["ping", "-c", "4", ip] # 在Windows上使用["ping", "-n", "4", ip]
result = subprocess.run(command, stdout=subprocess.PIPE, text=True)
return result.stdout
ip_address = "8.8.8.8"
print(ping_ip(ip_address))
在Python中是否有现成的库可以进行Ping操作?
是的,Python中有一些第三方库可以简化Ping操作,比如ping3
和scapy
。这些库提供了更为友好的API,使得Ping操作变得更加简单。例如,使用ping3
库的代码如下:
from ping3 import ping
response_time = ping('8.8.8.8')
print(f'Response time: {response_time} seconds')
在Ping操作中,如何处理异常情况?
在进行Ping操作时,可能会遇到网络不通或目标主机不可达等情况。为了提高程序的健壮性,可以使用异常处理机制来捕获这些错误。示例如下:
import subprocess
def ping_ip(ip):
try:
command = ["ping", "-c", "4", ip] # Windows使用["ping", "-n", "4", ip]
result = subprocess.run(command, stdout=subprocess.PIPE, text=True)
if result.returncode == 0:
return result.stdout
else:
return f"Ping to {ip} failed."
except Exception as e:
return f"An error occurred: {e}"
ip_address = "8.8.8.8"
print(ping_ip(ip_address))
这些方法不仅能帮助用户快速实现Ping功能,还能在实际应用中应对多种情况。
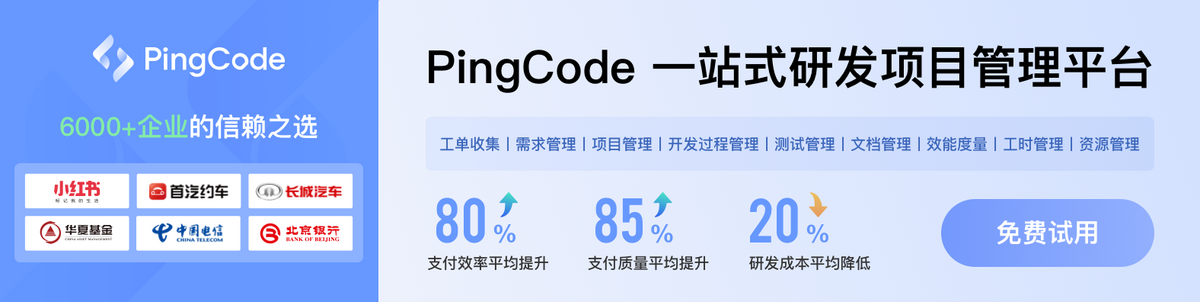