Python中的装饰器是一种设计模式,用于修改函数或方法的行为、代码复用、日志记录、权限校验。装饰器通过在不改变原函数代码的情况下,动态地增加功能,使代码更易于维护和扩展。装饰器本质上是一个高阶函数,它接收一个函数作为参数,并返回一个新的函数。以下将详细介绍Python装饰器的概念、应用场景和实现方式。
一、装饰器的基本概念和工作原理
装饰器是Python中一个强大的工具,可以在不改变原有函数源代码的情况下,动态地为函数添加功能。装饰器的工作原理是接收一个函数作为参数,并返回一个新的函数。新的函数通常会在调用原始函数之前或之后加入一些额外的操作。
装饰器的使用非常简单,只需在函数定义的前一行加上一个“@”符号,后跟装饰器函数的名称。装饰器的基本形式如下:
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
在这个例子中,say_hello
函数被my_decorator
装饰器修饰。当调用say_hello
时,实际上调用的是wrapper
函数,其中包含了对say_hello
的调用,以及额外的操作。
二、装饰器的应用场景
1、日志记录
装饰器可用于在函数调用时记录日志信息。通过在装饰器中加入日志代码,可以在每次函数调用时自动记录相关信息,而无需在每个函数中手动添加日志代码。
def log_decorator(func):
def wrapper(*args, kwargs):
print(f"Calling function {func.__name__} with arguments {args} and {kwargs}")
result = func(*args, kwargs)
print(f"Function {func.__name__} returned {result}")
return result
return wrapper
@log_decorator
def add(a, b):
return a + b
add(5, 3)
2、权限校验
在某些情况下,需要在调用函数之前检查用户的权限。装饰器可以在调用函数之前进行权限校验,确保用户有权执行该操作。
def permission_required(permission):
def decorator(func):
def wrapper(*args, kwargs):
if not has_permission(permission):
raise PermissionError("You do not have permission to execute this function.")
return func(*args, kwargs)
return wrapper
return decorator
@permission_required("admin")
def delete_user(user_id):
print(f"User {user_id} has been deleted.")
3、缓存
装饰器还可以用于实现缓存功能,以提高函数的执行效率。通过缓存函数的返回值,当相同的输入再次传递给函数时,可以直接返回缓存的结果,而不必再次计算。
def cache(func):
cached_results = {}
def wrapper(*args):
if args in cached_results:
return cached_results[args]
result = func(*args)
cached_results[args] = result
return result
return wrapper
@cache
def fibonacci(n):
if n in (0, 1):
return n
return fibonacci(n-1) + fibonacci(n-2)
print(fibonacci(30))
三、装饰器的实现方式
1、函数装饰器
函数装饰器是最常见的装饰器类型。它是一个接收函数作为参数的函数,并返回一个新的函数。函数装饰器可以使用在任何函数上,通过“@”符号调用。
2、类装饰器
类装饰器是一种更为高级的装饰器形式。它是一个类,其__call__
方法被实现为装饰器逻辑。类装饰器通常用于需要在装饰器中保存状态或数据的场景。
class CountCalls:
def __init__(self, func):
self.func = func
self.num_calls = 0
def __call__(self, *args, kwargs):
self.num_calls += 1
print(f"Call {self.num_calls} of {self.func.__name__!r}")
return self.func(*args, kwargs)
@CountCalls
def say_hello():
print("Hello!")
say_hello()
say_hello()
3、装饰器链
在Python中,可以对一个函数应用多个装饰器,这被称为装饰器链。在这种情况下,装饰器将从下到上依次应用于函数。
def decorate1(func):
def wrapper(*args, kwargs):
print("Decorate1 before function call")
result = func(*args, kwargs)
print("Decorate1 after function call")
return result
return wrapper
def decorate2(func):
def wrapper(*args, kwargs):
print("Decorate2 before function call")
result = func(*args, kwargs)
print("Decorate2 after function call")
return result
return wrapper
@decorate1
@decorate2
def greet(name):
print(f"Hello, {name}!")
greet("World")
四、装饰器的注意事项
1、装饰器的顺序
当使用多个装饰器时,装饰器的应用顺序从内到外,即最内层的装饰器先被应用。例如,在上面的装饰器链例子中,decorate2
会先被应用,然后是decorate1
。
2、保留原函数的元数据
使用装饰器时,原始函数的元数据(如函数名、文档字符串等)会被替换为装饰器返回的新函数的元数据。为了保留原始函数的元数据,可以使用functools.wraps
装饰器。
import functools
def my_decorator(func):
@functools.wraps(func)
def wrapper(*args, kwargs):
print("Something is happening before the function is called.")
result = func(*args, kwargs)
print("Something is happening after the function is called.")
return result
return wrapper
3、带参数的装饰器
有时装饰器需要接收参数来影响其行为。这可以通过创建一个返回装饰器的函数来实现。
def repeat(num_times):
def decorator(func):
@functools.wraps(func)
def wrapper(*args, kwargs):
for _ in range(num_times):
result = func(*args, kwargs)
return result
return wrapper
return decorator
@repeat(num_times=3)
def greet(name):
print(f"Hello, {name}!")
greet("Alice")
总之,装饰器是Python中一个非常强大的工具,可以用于增强函数的功能,提高代码的复用性和可维护性。在使用装饰器时,了解其工作原理和应用场景,可以帮助开发者更加高效地编写Python代码。
相关问答FAQs:
如何在Python中使用装饰器?
装饰器是Python中一种非常强大的工具,可以在不修改函数代码的情况下,增强或改变函数的行为。使用装饰器时,通常会定义一个接受函数作为参数的外部函数,并在内部定义一个新的函数来包装原始函数。然后,通过@符号将装饰器应用于目标函数。例如:
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
装饰器可以用于哪些场景?
装饰器可以在多种场景中使用,例如:记录日志、性能测试、权限验证、缓存结果、监控函数执行时间等。通过装饰器,可以在不改变原有函数逻辑的情况下,添加额外的功能。例如,可以创建一个装饰器来记录函数执行的时间,以便进行性能分析。
如何创建带参数的装饰器?
要创建带参数的装饰器,可以再嵌套一层函数。外层函数接收装饰器参数,内层函数接收被装饰的函数。以下是一个示例,展示如何实现一个带参数的装饰器:
def repeat(num_times):
def decorator_repeat(func):
def wrapper(*args, **kwargs):
for _ in range(num_times):
func(*args, **kwargs)
return wrapper
return decorator_repeat
@repeat(3)
def greet(name):
print(f"Hello, {name}!")
greet("Alice")
在这个例子中,greet
函数会被调用三次。通过这种方式,装饰器的灵活性得到了极大的增强。
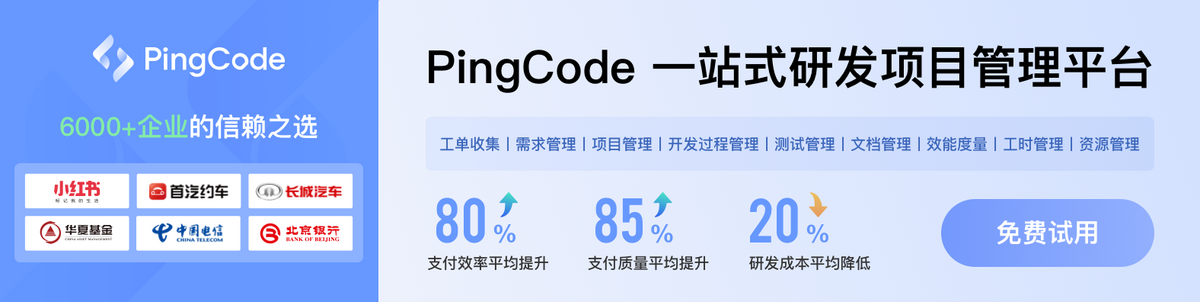