Python中的嵌套可以通过嵌套的函数、嵌套的循环以及嵌套的数据结构来实现。嵌套函数用于在一个函数内定义另一个函数、嵌套循环可以在一个循环内使用另一个循环来处理多层次的数据结构、嵌套的数据结构如列表、字典、元组等可以在一个数据结构中包含另一个数据结构。 下面将详细介绍其中的嵌套循环。
嵌套循环在处理多维数据结构时非常有用。例如,在处理二维列表时,外层循环可以遍历每一行,内层循环可以遍历每一列。通过这种方式,可以有效地处理复杂的数据结构。嵌套循环的使用可以极大地简化代码,提高程序的可读性和效率。
一、嵌套函数
嵌套函数是指在一个函数内部定义另一个函数。嵌套函数在需要封装逻辑、避免代码重复或隐藏实现细节时非常有用。
1. 嵌套函数的基本用法
嵌套函数可以访问其外部函数的局部变量。这使得嵌套函数成为实现闭包的关键。
def outer_function(text):
def inner_function():
print(text)
return inner_function
my_function = outer_function("Hello, World!")
my_function() # 输出: Hello, World!
在这个例子中,inner_function
是一个嵌套函数,它可以访问外部函数 outer_function
的参数 text
。这种特性使得嵌套函数非常适合用来创建工厂函数或装饰器。
2. 闭包与嵌套函数
闭包是指函数能够记住定义它的环境中的变量,即使在其环境已不复存在的情况下。闭包常用于装饰器中。
def make_multiplier_of(n):
def multiplier(x):
return x * n
return multiplier
times3 = make_multiplier_of(3)
print(times3(10)) # 输出: 30
times5 = make_multiplier_of(5)
print(times5(10)) # 输出: 50
二、嵌套循环
嵌套循环是指在一个循环体内使用另一个循环。它们在处理多维数据结构时特别有用。
1. 二维列表的嵌套循环
在处理二维列表(或矩阵)时,嵌套循环是最常用的工具。
matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
for row in matrix:
for element in row:
print(element, end=' ')
print()
2. 多层嵌套循环的应用
在某些复杂的算法中,可能需要使用多层嵌套循环。一个经典的例子是解决数独问题。
def is_valid_move(board, row, col, num):
for x in range(9):
if board[row][x] == num or board[x][col] == num:
return False
startRow = row - row % 3
startCol = col - col % 3
for i in range(3):
for j in range(3):
if board[i + startRow][j + startCol] == num:
return False
return True
三、嵌套的数据结构
Python支持多种嵌套的数据结构,比如列表中的列表,字典中的字典等等。
1. 嵌套列表
嵌套列表是指在一个列表中包含其他列表。这种结构常用于表示二维或多维数据。
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
for sublist in nested_list:
for item in sublist:
print(item, end=' ')
print()
2. 嵌套字典
嵌套字典是指在一个字典中包含其他字典。这种结构常用于表示层次化的数据。
nested_dict = {
'key1': {'subkey1': 1, 'subkey2': 2},
'key2': {'subkey3': 3, 'subkey4': 4}
}
for key, subdict in nested_dict.items():
print(f"{key}:")
for subkey, value in subdict.items():
print(f" {subkey}: {value}")
四、嵌套生成器
Python的生成器表达式也可以嵌套使用,用于生成复杂的生成器。
1. 基本嵌套生成器
嵌套生成器可以用于生成二维列表的所有元素。
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
flattened = (x for sublist in nested_list for x in sublist)
for item in flattened:
print(item, end=' ')
2. 复杂的嵌套生成器
在处理需要过滤或转换的数据时,嵌套生成器可以非常强大。
nested_list = [[1, -2, 3], [-4, 5, -6], [7, -8, 9]]
positive_flattened = (x for sublist in nested_list for x in sublist if x > 0)
for item in positive_flattened:
print(item, end=' ')
五、嵌套类
在面向对象编程中,可以在一个类中定义另一个类。
1. 基本嵌套类
嵌套类可以用于实现复杂的对象结构。
class OuterClass:
class InnerClass:
def __init__(self, value):
self.value = value
def display(self):
print(f"Value: {self.value}")
outer = OuterClass()
inner = outer.InnerClass(10)
inner.display()
2. 嵌套类的应用
嵌套类常用于实现复杂的数据结构或设计模式。
class Tree:
class Node:
def __init__(self, value):
self.value = value
self.children = []
def add_child(self, child_node):
self.children.append(child_node)
def __init__(self, root_value):
self.root = self.Node(root_value)
def add_node(self, parent_value, child_value):
# 递归查找并添加节点
def _add(node):
if node.value == parent_value:
node.add_child(self.Node(child_value))
return True
for child in node.children:
if _add(child):
return True
return False
_add(self.root)
tree = Tree(1)
tree.add_node(1, 2)
tree.add_node(1, 3)
tree.add_node(2, 4)
六、嵌套装饰器
装饰器是Python的一种特殊函数,可以在不修改原有函数代码的前提下,动态地增加功能。嵌套装饰器是指多个装饰器叠加使用。
1. 基本嵌套装饰器
装饰器可以通过嵌套实现更加复杂的功能组合。
def decorator_one(func):
def wrapper():
print("Decorator One")
func()
return wrapper
def decorator_two(func):
def wrapper():
print("Decorator Two")
func()
return wrapper
@decorator_one
@decorator_two
def greet():
print("Hello, World!")
greet()
2. 嵌套装饰器的应用
嵌套装饰器在需要多个功能叠加时非常有用,比如同时实现日志记录和访问控制。
def logged(func):
def wrapper(*args, kwargs):
print(f"Logging: {func.__name__}")
return func(*args, kwargs)
return wrapper
def authenticated(func):
def wrapper(user, *args, kwargs):
if user == "admin":
return func(user, *args, kwargs)
else:
print("Authentication failed!")
return None
return wrapper
@logged
@authenticated
def view_dashboard(user):
print(f"Welcome {user}, you have access to the dashboard.")
view_dashboard("admin")
view_dashboard("guest")
通过以上内容,我们可以看到Python中的嵌套应用非常广泛且强大,不仅仅限于简单的循环或函数,而是在多层次、多维度的数据处理、逻辑实现中扮演着重要角色。无论是编写简单的脚本还是复杂的系统,理解和掌握这些嵌套技术都将极大地提高代码的效率和可维护性。
相关问答FAQs:
Python嵌套结构的主要应用场景是什么?
在Python中,嵌套结构主要用于提高代码的组织性和可读性。常见的应用场景包括嵌套函数、嵌套循环以及嵌套数据结构(如列表、字典等)。通过这些嵌套结构,可以实现更复杂的数据处理和逻辑控制,比如在一个循环内定义另一个循环来处理多维数据。
如何在Python中嵌套函数,并且如何调用它们?
在Python中,可以在一个函数内部定义另一个函数,这被称为嵌套函数。定义嵌套函数时,外层函数可以访问内层函数的变量。调用时,需要先调用外层函数,内层函数才会被执行。例如:
def outer_function():
def inner_function():
return "Hello from inner function!"
return inner_function()
print(outer_function()) # 输出: Hello from inner function!
使用嵌套数据结构时,有哪些常见的错误需要避免?
在使用嵌套数据结构(如列表和字典)时,容易出现一些常见错误,包括索引错误、键错误和类型错误。确保在访问嵌套结构的元素时,使用正确的索引或键。此外,注意数据结构的深度,过于复杂的嵌套可能导致代码难以维护和理解。建议在设计时保持结构的简单性与清晰性。
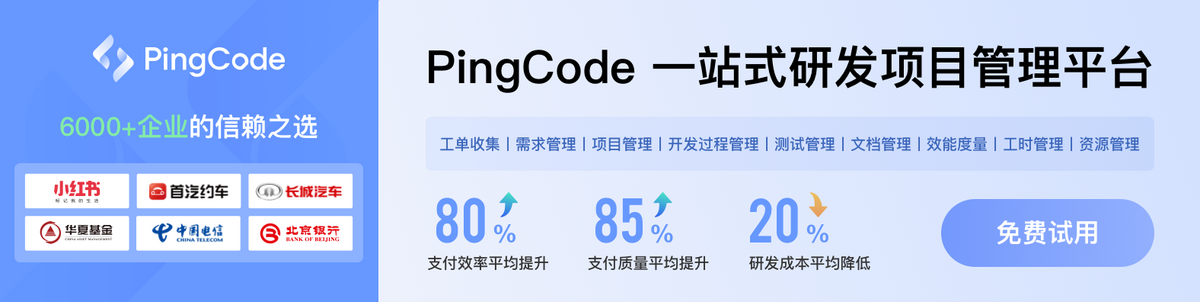