在Python中发送POST请求可以通过多种方式实现,常用的方法包括使用requests库、使用http.client模块、使用urllib模块。requests库是最为简便和常用的方式,因为它提供了一个简单的API来处理HTTP请求和响应,支持各种HTTP方法、自动处理Cookie、支持会话等功能。接下来,我将详细讲解如何使用requests库发送POST请求。
使用requests库发送POST请求时,需要安装该库,通常使用pip install requests
命令进行安装。安装完成后,可以通过requests.post()
函数发送POST请求,传递请求URL、请求头、请求体等参数,并接收服务器返回的响应数据。以下是详细的步骤和代码示例。
一、安装与基本使用
1. 安装requests库
首先,确保系统中安装了requests库。如果未安装,可以通过以下命令进行安装:
pip install requests
2. 使用requests发送POST请求
使用requests库发送POST请求非常简单。以下是基本的代码结构:
import requests
url = 'https://example.com/api'
data = {
'key1': 'value1',
'key2': 'value2'
}
response = requests.post(url, data=data)
print(response.status_code)
print(response.json())
在这段代码中,我们定义了一个请求URL和请求体数据,然后使用requests.post()
函数发送请求,并接收响应对象。可以通过response.status_code
查看响应状态码,通过response.json()
解析JSON响应。
二、发送JSON数据
1. 使用json参数
在发送POST请求时,常常需要传递JSON格式的数据。使用requests库,可以通过json
参数直接传递Python字典,requests库会自动将其转换为JSON格式。
import requests
url = 'https://example.com/api'
payload = {
'key1': 'value1',
'key2': 'value2'
}
response = requests.post(url, json=payload)
print(response.status_code)
print(response.json())
2. 手动转换为JSON格式
如果需要手动控制数据格式,可以使用json.dumps()
函数将字典转换为JSON字符串,并在请求头中设置Content-Type
为application/json
。
import requests
import json
url = 'https://example.com/api'
payload = {
'key1': 'value1',
'key2': 'value2'
}
headers = {'Content-Type': 'application/json'}
response = requests.post(url, data=json.dumps(payload), headers=headers)
print(response.status_code)
print(response.json())
三、处理请求头与认证
1. 自定义请求头
在某些情况下,可能需要自定义请求头,例如设置用户代理、认证信息等。可以通过headers
参数传递请求头。
import requests
url = 'https://example.com/api'
data = {
'key1': 'value1',
'key2': 'value2'
}
headers = {
'User-Agent': 'my-app/0.0.1',
'Custom-Header': 'CustomValue'
}
response = requests.post(url, data=data, headers=headers)
print(response.status_code)
print(response.json())
2. 处理HTTP认证
requests库还支持各种认证方式,例如Basic认证、Digest认证等。以下是使用Basic认证的示例:
import requests
from requests.auth import HTTPBasicAuth
url = 'https://example.com/api'
data = {
'key1': 'value1',
'key2': 'value2'
}
response = requests.post(url, data=data, auth=HTTPBasicAuth('username', 'password'))
print(response.status_code)
print(response.json())
四、上传文件
1. 使用files参数上传文件
requests库支持通过POST请求上传文件,可以使用files
参数传递文件对象。
import requests
url = 'https://example.com/upload'
files = {'file': open('report.csv', 'rb')}
response = requests.post(url, files=files)
print(response.status_code)
print(response.json())
2. 上传多个文件
可以同时上传多个文件,方法是将多个文件对象放入字典中。
import requests
url = 'https://example.com/upload'
files = {
'file1': open('report1.csv', 'rb'),
'file2': open('report2.csv', 'rb')
}
response = requests.post(url, files=files)
print(response.status_code)
print(response.json())
五、超时与重试机制
1. 设置请求超时
为了避免请求长时间无响应,可以设置请求超时。超时值可以是一个数字(秒)或元组(连接超时, 读取超时)。
import requests
url = 'https://example.com/api'
data = {'key': 'value'}
try:
response = requests.post(url, data=data, timeout=10)
print(response.status_code)
print(response.json())
except requests.Timeout:
print('The request timed out')
2. 实现重试机制
可以使用第三方库urllib3.util.retry
与requests.adapters.HTTPAdapter
实现重试机制。
import requests
from requests.adapters import HTTPAdapter
from urllib3.util.retry import Retry
url = 'https://example.com/api'
data = {'key': 'value'}
session = requests.Session()
retry = Retry(total=5, backoff_factor=0.1)
adapter = HTTPAdapter(max_retries=retry)
session.mount('http://', adapter)
session.mount('https://', adapter)
response = session.post(url, data=data)
print(response.status_code)
print(response.json())
六、使用Session对象
1. 保持会话
使用requests库的Session对象,可以在多个请求间共享Cookie等信息。
import requests
url = 'https://example.com/api'
data = {'key': 'value'}
session = requests.Session()
response = session.post(url, data=data)
print(response.status_code)
print(response.json())
2. 配置Session对象
可以在Session对象上配置默认的请求头、认证信息等,方便在多个请求中重用。
import requests
session = requests.Session()
session.headers.update({'User-Agent': 'my-app/0.0.1'})
url = 'https://example.com/api'
data = {'key': 'value'}
response = session.post(url, data=data)
print(response.status_code)
print(response.json())
七、处理重定向与响应
1. 禁用自动重定向
requests库默认会自动处理重定向。如果不希望自动重定向,可以将allow_redirects
参数设置为False
。
import requests
url = 'https://example.com/redirect'
data = {'key': 'value'}
response = requests.post(url, data=data, allow_redirects=False)
print(response.status_code)
print(response.headers['Location'])
2. 解析响应内容
除了使用response.json()
解析JSON响应,还可以通过response.text
获取响应文本,通过response.content
获取字节数据。
import requests
url = 'https://example.com/api'
data = {'key': 'value'}
response = requests.post(url, data=data)
print(response.status_code)
print(response.text)
通过上述各个方面的讲解,可以看出使用Python的requests库发送POST请求非常灵活,能够满足多种应用场景的需求。希望这篇文章能够帮助您更好地理解和应用Python的HTTP请求功能。
相关问答FAQs:
如何在Python中使用requests库发送POST请求?
在Python中,使用requests库发送POST请求非常简单。首先,确保你已经安装了requests库,可以通过pip install requests
进行安装。接下来,可以使用以下示例代码来发送POST请求:
import requests
url = 'https://example.com/api'
data = {'key1': 'value1', 'key2': 'value2'}
response = requests.post(url, data=data)
print(response.text)
在上面的代码中,url
是你要发送请求的地址,data
是要发送的表单数据。发送请求后,服务器的响应会保存在response
对象中,你可以通过response.text
获取响应内容。
Python发送POST请求时可以发送哪些类型的数据?
在Python中发送POST请求时,可以发送多种类型的数据,包括表单数据、JSON数据和文件等。使用requests库,可以通过设置不同的参数来实现:
- 表单数据:使用
data
参数发送。 - JSON数据:使用
json
参数发送,并确保请求头中包含Content-Type: application/json
。
示例代码如下:
import requests
import json
url = 'https://example.com/api'
# 发送JSON数据
json_data = {'key1': 'value1', 'key2': 'value2'}
response = requests.post(url, json=json_data)
print(response.text)
- 文件上传:通过
files
参数上传文件。
示例代码:
files = {'file': open('file.txt', 'rb')}
response = requests.post(url, files=files)
如何处理POST请求的响应数据?
在发送POST请求后,处理响应数据是非常重要的。使用requests库时,可以通过response
对象获取不同格式的响应数据:
- 文本内容:使用
response.text
获取响应的文本内容。 - JSON格式:如果响应内容为JSON格式,可以使用
response.json()
将其解析为Python字典。
示例:
response_json = response.json()
print(response_json['key'])
- 状态码:使用
response.status_code
查看请求的状态码,以判断请求是否成功(状态码200通常表示成功)。
通过这些方法,可以有效地获取和处理POST请求的响应数据。
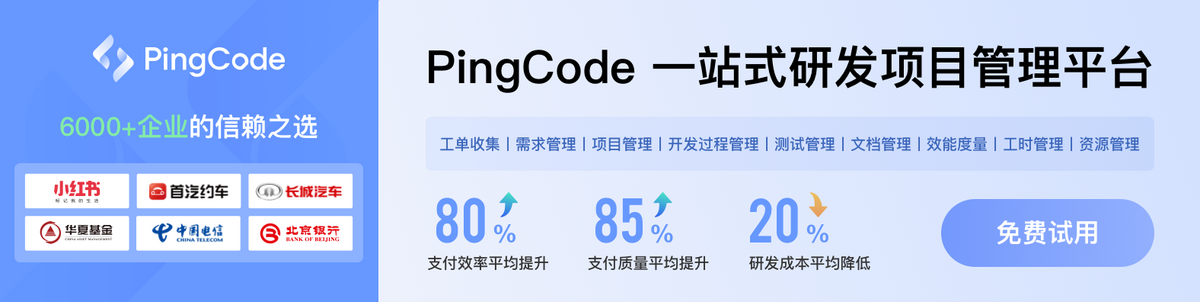