使用Python进行点名可以通过创建一个学生名单、随机选择学生进行点名、记录点名结果等方式实现。常用的方法包括:使用列表存储学生名单、使用随机模块进行抽取、使用文件读取和写入进行记录。下面我们详细介绍如何实现这些功能。
一、创建学生名单
首先,需要创建一个学生名单,通常可以使用Python中的列表来存储学生的名字。这样可以方便地对学生进行操作,比如增删改查。
students = ["Alice", "Bob", "Charlie", "David", "Eva"]
二、随机选择学生进行点名
为了随机选择一名学生进行点名,可以使用Python的random
模块。这个模块提供了随机选择的功能,可以用来从学生名单中随机抽取一个名字。
import random
def random_call(students):
return random.choice(students)
chosen_student = random_call(students)
print(f"The student chosen for roll call is: {chosen_student}")
三、记录点名结果
在实际应用中,可能需要记录每次点名的结果,比如保存到一个文件中。可以使用Python的文件操作来实现这一功能。
def record_roll_call(student):
with open("roll_call_record.txt", "a") as file:
file.write(f"{student}\n")
record_roll_call(chosen_student)
四、改进点名系统
在实现了基本的点名功能之后,可以对系统进行一些改进,比如防止重复点名、支持多次点名、统计点名次数等。
- 防止重复点名
可以在每次点名后,将点名的学生从名单中移除,确保下次不会重复点到同一个学生。
def random_call_no_repeat(students):
if students:
chosen_student = random.choice(students)
students.remove(chosen_student)
return chosen_student
else:
return None
chosen_student = random_call_no_repeat(students)
if chosen_student:
print(f"The student chosen for roll call is: {chosen_student}")
record_roll_call(chosen_student)
else:
print("All students have been called.")
- 支持多次点名
如果要进行多次点名,可以在点名后重置学生名单,或者在每次点名前检查名单是否需要重置。
original_students = ["Alice", "Bob", "Charlie", "David", "Eva"]
def reset_students():
return original_students.copy()
students = reset_students()
for _ in range(3): # Example: Call 3 times
chosen_student = random_call_no_repeat(students)
if chosen_student:
print(f"The student chosen for roll call is: {chosen_student}")
record_roll_call(chosen_student)
else:
print("All students have been called. Resetting list.")
students = reset_students()
- 统计点名次数
可以增加一个功能,统计每个学生被点名的次数,以便了解点名的均匀性。
from collections import defaultdict
call_count = defaultdict(int)
def random_call_with_count(students):
if students:
chosen_student = random.choice(students)
call_count[chosen_student] += 1
return chosen_student
else:
return None
students = reset_students()
for _ in range(10): # Example: Call 10 times
chosen_student = random_call_with_count(students)
if chosen_student:
print(f"The student chosen for roll call is: {chosen_student}")
record_roll_call(chosen_student)
else:
print("All students have been called. Resetting list.")
students = reset_students()
print("Roll call count:", dict(call_count))
通过以上步骤,可以使用Python实现一个简单的点名系统,能够随机选择学生、记录点名结果,并支持多次点名和统计功能。在实际应用中,根据需求可以进一步拓展和优化系统,比如增加图形用户界面、结合数据库进行管理等。
相关问答FAQs:
如何用Python制作一个简单的点名系统?
要制作一个简单的点名系统,可以使用Python的列表来存储学生姓名,结合输入功能来实现点名的过程。可以通过随机选择学生姓名或按顺序遍历列表来实现。例如,使用random.choice()
函数可以随机选取一个姓名,确保每次点名的结果不一样。
点名系统中可以加入哪些功能以提高使用体验?
可以考虑增加学生缺席记录、自动生成点名报告以及支持多班级管理等功能。比如,记录每位学生的出勤情况可以帮助老师更好地了解学生的出席状态。此外,使用CSV文件保存点名记录,方便后续的数据分析和查询。
如何确保点名系统的可靠性和准确性?
确保点名系统的可靠性可以通过数据验证和错误处理来实现。在输入学生姓名时,可以设置条件,确保不出现重复姓名或拼写错误。同时,定期备份点名数据,以防数据丢失或损坏,保障系统的稳定性和可靠性。
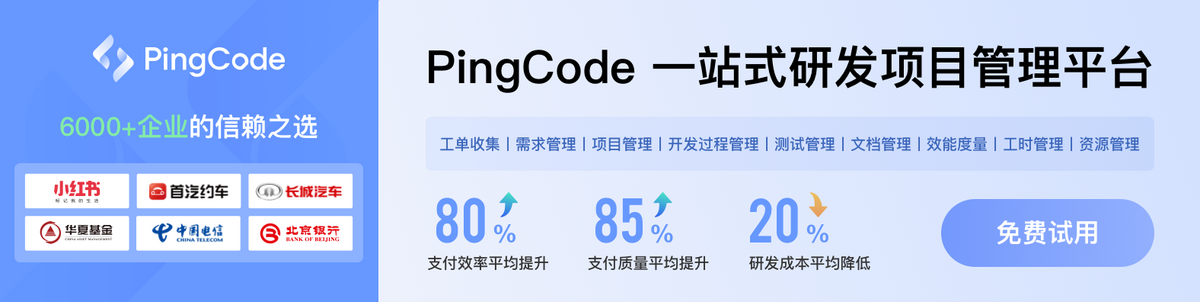