Python替换脸的方法包括使用OpenCV进行人脸检测、通过dlib进行人脸特征点检测、使用图像处理库如PIL进行图像合成。在这些方法中,OpenCV是一个强大的计算机视觉库,能够快速检测人脸并进行简单的图像处理。通过dlib库,可以精确定位人脸的特征点,从而实现更高精度的替换效果。最后,使用PIL等图像处理库进行图像的合成与处理,可以实现最终的脸部替换效果。下面将详细介绍如何运用这些工具来实现脸部替换。
一、使用OpenCV进行人脸检测
OpenCV是一个开源的计算机视觉库,提供了多种图像处理功能。通过OpenCV,我们可以实现对图像中人脸的检测,这是脸部替换的第一步。
-
安装OpenCV
要使用OpenCV,首先需要安装该库。在Python环境中,可以通过pip命令安装:
pip install opencv-python
-
加载图像并进行人脸检测
使用OpenCV的
CascadeClassifier
进行人脸检测是最常用的方法之一。可以加载预先训练好的分类器,例如haarcascade_frontalface_default.xml
,并检测图像中的人脸。import cv2
加载预训练的人脸检测模型
face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + 'haarcascade_frontalface_default.xml')
读取图像
image = cv2.imread('input.jpg')
转换为灰度图
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
检测人脸
faces = face_cascade.detectMultiScale(gray, 1.1, 4)
绘制检测到的人脸
for (x, y, w, h) in faces:
cv2.rectangle(image, (x, y), (x + w, y + h), (255, 0, 0), 2)
显示结果
cv2.imshow('Detected Faces', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
-
提取人脸区域
在检测到人脸后,可以提取图像中人脸的区域,以便进行进一步处理。
# 提取人脸区域
for (x, y, w, h) in faces:
face_region = image[y:y+h, x:x+w]
# 可以在这里对face_region进行处理,如替换
二、使用dlib进行人脸特征点检测
dlib是一个广泛使用的机器学习库,具有强大的人脸识别和特征点检测能力。通过检测人脸特征点,可以实现更精确的脸部替换。
-
安装dlib
dlib库可以通过pip安装,但需要注意的是,它对编译环境有一定的要求。
pip install dlib
-
加载图像并检测特征点
使用dlib的
shape_predictor
来检测人脸特征点。import dlib
import cv2
加载dlib的预训练模型
predictor_path = 'shape_predictor_68_face_landmarks.dat'
face_detector = dlib.get_frontal_face_detector()
shape_predictor = dlib.shape_predictor(predictor_path)
读取图像
image = cv2.imread('input.jpg')
转换为灰度图
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
检测人脸
faces = face_detector(gray)
检测特征点
for face in faces:
landmarks = shape_predictor(gray, face)
for n in range(0, 68):
x = landmarks.part(n).x
y = landmarks.part(n).y
cv2.circle(image, (x, y), 2, (0, 255, 0), -1)
显示结果
cv2.imshow('Landmarks', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
-
使用特征点进行脸部替换
通过检测到的人脸特征点,可以将一张脸的特征与另一张脸的特征进行匹配和替换。
# 假设已检测到两张人脸的特征点:source_landmarks 和 target_landmarks
计算仿射变换矩阵
points1 = [source_landmarks.part(i) for i in range(68)]
points2 = [target_landmarks.part(i) for i in range(68)]
points1 = np.array([(point.x, point.y) for point in points1], dtype=np.float32)
points2 = np.array([(point.x, point.y) for point in points2], dtype=np.float32)
获取仿射变换矩阵
M = cv2.getAffineTransform(points1[:3], points2[:3])
应用仿射变换
warped_image = cv2.warpAffine(source_face, M, (target_face.shape[1], target_face.shape[0]))
替换目标脸区域
target_face[y:y+h, x:x+w] = warped_image
三、使用PIL进行图像合成
PIL(Python Imaging Library)是一个强大的图像处理库,可以用于图像的合成和处理。
-
安装PIL
PIL的现代替代库是Pillow,可以通过pip安装:
pip install pillow
-
加载图像并进行处理
使用PIL加载图像并进行基本处理,如调整大小、旋转等。
from PIL import Image
打开图像
image = Image.open('input.jpg')
调整大小
resized_image = image.resize((100, 100))
旋转图像
rotated_image = resized_image.rotate(45)
显示图像
rotated_image.show()
-
合成图像
使用PIL进行图像的合成,如将一张脸合成到另一张图像上。
from PIL import Image
打开两张图像
base_image = Image.open('base.jpg')
face_image = Image.open('face.jpg')
合成图像
base_image.paste(face_image, (50, 50), face_image)
显示结果
base_image.show()
四、综合应用实现脸部替换
通过综合运用OpenCV、dlib和PIL,可以实现更加复杂和精确的脸部替换。以下是一个简单的综合应用示例:
- 检测源图像和目标图像中的人脸
- 使用dlib检测特征点并计算仿射变换矩阵
- 使用OpenCV对源图像进行仿射变换
- 使用PIL合成结果图像
import cv2
import dlib
import numpy as np
from PIL import Image
加载dlib的预训练模型
predictor_path = 'shape_predictor_68_face_landmarks.dat'
face_detector = dlib.get_frontal_face_detector()
shape_predictor = dlib.shape_predictor(predictor_path)
读取源图像和目标图像
source_image = cv2.imread('source.jpg')
target_image = cv2.imread('target.jpg')
转换为灰度图
source_gray = cv2.cvtColor(source_image, cv2.COLOR_BGR2GRAY)
target_gray = cv2.cvtColor(target_image, cv2.COLOR_BGR2GRAY)
检测人脸
source_faces = face_detector(source_gray)
target_faces = face_detector(target_gray)
假设只处理检测到的第一张人脸
source_landmarks = shape_predictor(source_gray, source_faces[0])
target_landmarks = shape_predictor(target_gray, target_faces[0])
计算仿射变换矩阵
source_points = np.array([(source_landmarks.part(i).x, source_landmarks.part(i).y) for i in range(68)], dtype=np.float32)
target_points = np.array([(target_landmarks.part(i).x, target_landmarks.part(i).y) for i in range(68)], dtype=np.float32)
M = cv2.getAffineTransform(source_points[:3], target_points[:3])
应用仿射变换
warped_source = cv2.warpAffine(source_image, M, (target_image.shape[1], target_image.shape[0]))
转换为PIL图像进行合成
warped_source_pil = Image.fromarray(cv2.cvtColor(warped_source, cv2.COLOR_BGR2RGB))
target_image_pil = Image.fromarray(cv2.cvtColor(target_image, cv2.COLOR_BGR2RGB))
合成图像
target_image_pil.paste(warped_source_pil, (0, 0), warped_source_pil)
显示结果
target_image_pil.show()
通过上述步骤,您可以实现简单的脸部替换。这些方法可以根据具体需求进行调整和优化。使用OpenCV、dlib和PIL等工具,您可以在Python中实现强大的图像处理和脸部替换功能。
相关问答FAQs:
如何在Python中实现人脸替换的基本步骤?
在Python中实现人脸替换通常需要使用图像处理库,如OpenCV和Dlib。首先,您需要检测人脸并提取特征。接着,可以使用图像转换技术将一个人的脸替换到另一个人的脸上。常见的步骤包括读取图像、检测人脸、提取面部特征、进行图像变换和最后合成新图像。具体实现可以参考一些开源项目或教程。
使用Python进行人脸替换需要哪些库和工具?
实现人脸替换的常用库包括OpenCV、Dlib、NumPy和Pillow。OpenCV主要用于图像处理和人脸检测,Dlib提供了高效的人脸特征点检测,NumPy用于数组操作,Pillow则是处理图像文件的好帮手。您可以通过pip install
命令安装这些库,以便开始项目。
人脸替换在Python中有哪些应用场景?
人脸替换技术在多个领域都有广泛应用,包括娱乐行业(如电影特效和视频制作)、社交媒体(如滤镜和特效)、以及安全监控和身份验证等。通过这种技术,可以创造出有趣的图像和视频效果,增强用户的互动体验,甚至用于数据增强以提高机器学习模型的表现。
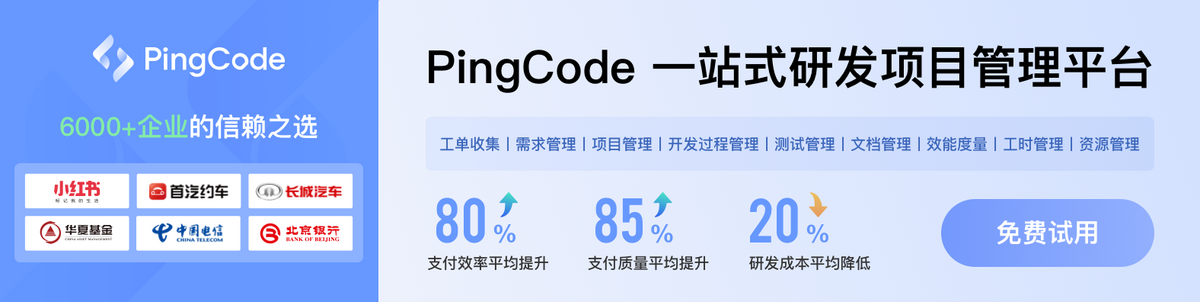