在Python中去除标点符号可以通过多种方法实现、最常用的方法包括使用字符串操作、正则表达式和Python内置的库。下面将详细描述一种常用的方法,即使用str.translate
和string.punctuation
来去除标点符号。
str.translate
方法结合string.punctuation
模块是去除标点符号的有效方法。string.punctuation
包含了所有常见的标点符号,str.maketrans
可以创建一个映射表,然后str.translate
使用该映射表去掉字符串中的标点符号。这种方法简单直接,适合处理绝大多数文本数据。
接下来,我们将深入探讨几种不同的方法来去除Python中的标点符号,包括使用正则表达式、字符串操作以及其他工具和库。
一、使用字符串操作去除标点符号
使用Python的字符串操作方法去除标点符号是一种简单而有效的方法,特别是在处理较小的数据集时。
1. 使用str.translate
和string.punctuation
str.translate
方法结合string.punctuation
模块是去除标点符号的一种常用方法。
import string
def remove_punctuation(text):
translator = str.maketrans('', '', string.punctuation)
return text.translate(translator)
text = "Hello, world! This is a test."
clean_text = remove_punctuation(text)
print(clean_text) # 输出: Hello world This is a test
这种方法利用str.maketrans
创建一个映射表,将所有标点符号映射为None
,然后使用str.translate
去除这些标点符号。
2. 使用列表解析去除标点
列表解析结合string.punctuation
可以快速去除标点符号。
import string
def remove_punctuation(text):
return ''.join([char for char in text if char not in string.punctuation])
text = "Hello, world! This is a test."
clean_text = remove_punctuation(text)
print(clean_text) # 输出: Hello world This is a test
这种方法通过迭代字符串中的每个字符,并将非标点符号字符加入新的字符串中。
二、使用正则表达式去除标点符号
正则表达式是一种强大且灵活的文本处理工具,适合于复杂的模式匹配和替换。
1. 使用re.sub
去除标点
re.sub
函数可以用来替换字符串中的特定模式,这里用于去除标点符号。
import re
def remove_punctuation(text):
return re.sub(r'[^\w\s]', '', text)
text = "Hello, world! This is a test."
clean_text = remove_punctuation(text)
print(clean_text) # 输出: Hello world This is a test
在这个例子中,[^\w\s]
是一个正则表达式,匹配所有非字母数字字符和空白字符,然后将其替换为空字符串。
2. 自定义正则模式
可以根据需要自定义正则表达式模式,以去除特定的标点符号或字符。
import re
def remove_custom_punctuation(text, punctuation):
pattern = f"[{re.escape(punctuation)}]"
return re.sub(pattern, '', text)
text = "Hello, world! This is a test."
custom_punctuation = ",!"
clean_text = remove_custom_punctuation(text, custom_punctuation)
print(clean_text) # 输出: Hello world This is a test.
通过re.escape
函数可以确保在正则表达式中正确处理特殊字符。
三、使用外部库去除标点符号
Python的生态系统中有许多库可以帮助简化文本处理任务。
1. 使用nltk
库去除标点
nltk
是Python中强大的自然语言处理库,提供了许多文本处理功能。
import nltk
from nltk.tokenize import word_tokenize
nltk.download('punkt')
def remove_punctuation(text):
words = word_tokenize(text)
words = [word for word in words if word.isalnum()]
return ' '.join(words)
text = "Hello, world! This is a test."
clean_text = remove_punctuation(text)
print(clean_text) # 输出: Hello world This is a test
nltk
库的word_tokenize
函数用于将文本拆分为单词,然后过滤掉非字母数字的单词。
2. 使用spaCy
库去除标点
spaCy
是另一个流行的自然语言处理库,适用于复杂的文本处理任务。
import spacy
nlp = spacy.load("en_core_web_sm")
def remove_punctuation(text):
doc = nlp(text)
return ' '.join([token.text for token in doc if not token.is_punct])
text = "Hello, world! This is a test."
clean_text = remove_punctuation(text)
print(clean_text) # 输出: Hello world This is a test
spaCy
的nlp
对象处理文本,并通过is_punct
属性过滤掉标点符号。
四、处理不同语言和字符集
去除标点符号不仅限于英文文本,在处理其他语言时,可能需要考虑特定语言的字符集和标点符号。
1. 处理非英文标点符号
在处理多语言文本时,可能需要扩展标点符号的范围。
import re
def remove_punctuation_multilang(text):
return re.sub(r'[^\w\s]', '', text, flags=re.UNICODE)
text = "Bonjour, le monde! 这是一个测试。"
clean_text = remove_punctuation_multilang(text)
print(clean_text) # 输出: Bonjour le monde 这是一个测试
通过使用re.UNICODE
标志,可以确保正则表达式处理Unicode字符。
2. 使用自定义标点符号列表
可以根据特定语言或应用场景自定义标点符号列表。
def remove_custom_punctuation(text, punctuation):
return ''.join([char for char in text if char not in punctuation])
text = "Bonjour, le monde! 这是一个测试。"
custom_punctuation = ",!。"
clean_text = remove_custom_punctuation(text, custom_punctuation)
print(clean_text) # 输出: Bonjour le monde 这是一个测试
通过自定义标点符号列表,可以灵活地处理不同语言和应用场景。
五、总结
在Python中去除标点符号有多种方法可供选择,包括使用字符串操作、正则表达式和外部库。选择合适的方法取决于具体的应用场景和数据集的规模。对于简单的文本处理任务,字符串操作和正则表达式通常是足够的;而对于复杂的自然语言处理任务,nltk
和spaCy
等库可以提供更强大的功能和灵活性。在处理多语言文本时,需要特别注意字符集和语言特定的标点符号。
相关问答FAQs:
如何在Python中删除字符串中的所有标点符号?
在Python中,可以使用内置的字符串方法以及正则表达式来去除标点符号。使用str.translate()
方法结合str.maketrans()
可以有效地移除标点符号。此外,使用re
模块的正则表达式也能实现类似的效果。以下是一个示例代码:
import string
text = "Hello, world! This is a test."
clean_text = text.translate(str.maketrans('', '', string.punctuation))
print(clean_text) # 输出: Hello world This is a test
如何使用正则表达式去除Python字符串中的标点?
使用re
模块的re.sub()
函数可以轻松地去除标点符号。例如,可以通过以下代码实现:
import re
text = "Hello, world! This is a test."
clean_text = re.sub(r'[^\w\s]', '', text)
print(clean_text) # 输出: Hello world This is a test
这种方法允许您根据需要自定义要保留的字符。
在处理中文文本时,如何去除标点符号?
处理中文文本时,可以使用re
模块来删除标点符号。由于中文标点符号与英文不同,您可以定义一个包含中文标点的正则表达式来进行匹配和替换。以下是一个示例:
import re
text = "你好,世界!这是一个测试。"
clean_text = re.sub(r'[,。!?;:“”‘’]', '', text)
print(clean_text) # 输出: 你好世界这是一个测试
这种方式可以有效地去除中文中的常见标点符号。
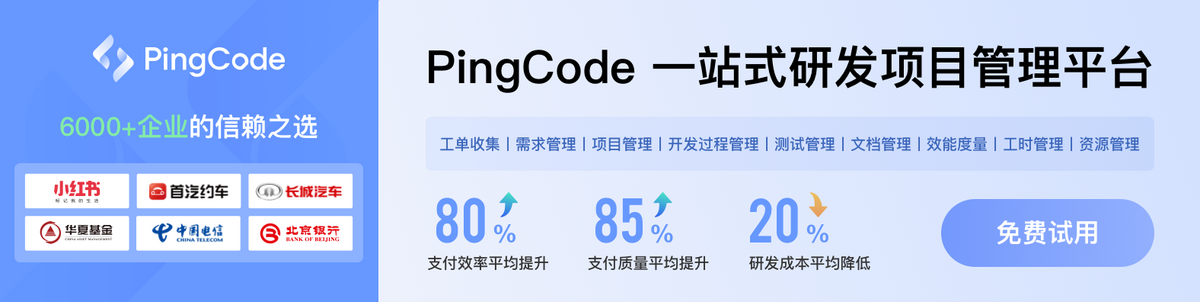