在Python中关闭线程可以通过设置标志位、使用threading.Event
对象、以及利用concurrent.futures
模块来实现。设置标志位是通过在线程运行过程中检查某个变量的状态,threading.Event
对象则是通过更为灵活的线程间通信机制,concurrent.futures
模块则提供了一个更高级的接口来管理线程的生命周期。下面将详细介绍如何实现这些方法。
一、使用标志位关闭线程
使用标志位是关闭线程的一个简单且常见的方法。通过在主线程中设置一个全局变量作为标志位,线程在运行过程中不断检查这个标志位的状态,决定是否退出。
1.1、定义标志位
首先,我们需要定义一个全局变量作为标志位。这个变量可以是一个简单的布尔值,表示线程是否应该继续运行。
import threading
import time
定义一个全局变量作为标志位
exit_flag = False
1.2、线程函数中检查标志位
在线程函数中,我们需要定期检查这个标志位。如果标志位被设置为True
,则线程应该执行清理操作并退出。
def thread_function():
while not exit_flag:
print("Thread is running")
time.sleep(1)
print("Thread is exiting")
1.3、主线程中设置标志位
在主线程中,我们可以根据需要随时设置标志位为True
,从而通知线程退出。
def main():
global exit_flag
thread = threading.Thread(target=thread_function)
thread.start()
# 主线程等待一段时间
time.sleep(5)
# 设置标志位,通知线程退出
exit_flag = True
# 等待线程结束
thread.join()
print("Main thread is exiting")
main()
二、使用threading.Event
对象关闭线程
threading.Event
对象提供了一种更高级的线程间通信机制,可以用于通知线程停止。
2.1、创建threading.Event
对象
我们需要创建一个threading.Event
对象,该对象可以在线程间共享。
import threading
import time
创建一个Event对象
exit_event = threading.Event()
2.2、线程函数中检查Event
对象
在线程函数中,我们可以使用wait()
方法定期检查Event
对象的状态。如果Event
对象被设置,则wait()
方法会返回True
,此时线程应该退出。
def thread_function():
while not exit_event.is_set():
print("Thread is running")
time.sleep(1)
print("Thread is exiting")
2.3、主线程中设置Event
对象
在主线程中,我们可以使用set()
方法设置Event
对象,从而通知线程退出。
def main():
thread = threading.Thread(target=thread_function)
thread.start()
# 主线程等待一段时间
time.sleep(5)
# 设置Event对象,通知线程退出
exit_event.set()
# 等待线程结束
thread.join()
print("Main thread is exiting")
main()
三、使用concurrent.futures
模块关闭线程
concurrent.futures
模块提供了一个更高级的接口来管理线程和进程,使用ThreadPoolExecutor
可以方便地管理线程的生命周期。
3.1、创建ThreadPoolExecutor
我们可以使用ThreadPoolExecutor
创建一个线程池,并提交任务给线程池执行。
from concurrent.futures import ThreadPoolExecutor
import time
def thread_function():
while True:
print("Thread is running")
time.sleep(1)
3.2、提交任务并管理线程
我们可以使用ThreadPoolExecutor
的submit()
方法提交任务,并通过shutdown()
方法关闭线程池。
def main():
with ThreadPoolExecutor(max_workers=1) as executor:
future = executor.submit(thread_function)
# 主线程等待一段时间
time.sleep(5)
# 关闭线程池,等待线程结束
executor.shutdown(wait=True)
print("Main thread is exiting")
main()
通过以上几种方法,我们可以灵活地控制线程的启动和关闭。选择合适的方法取决于应用场景和具体需求。在多线程编程中,合理地管理线程的生命周期是保证程序稳定性和性能的重要因素。
相关问答FAQs:
如何安全地终止一个运行中的线程?
在Python中,安全地终止一个线程通常可以通过设置一个标志来实现。在线程的执行过程中,可以定期检查这个标志,如果标志被设置为True,线程就可以优雅地退出。使用threading.Event
对象来管理这种标志是一个好的选择。这样可以确保线程在适当的时候停止,而不是强行终止。
使用join()
方法有什么作用?join()
方法用于等待线程完成。调用该方法的线程会暂停,直到被调用的线程完成。如果希望在主线程中等待子线程执行完毕,可以使用join()
,这对于确保所有线程都正确执行并释放资源是非常重要的。
在Python中有没有强制关闭线程的方法?
Python并不支持强制关闭线程的直接方法。这是因为强制中止一个线程可能导致数据不一致或资源泄漏。尽管可以使用一些不推荐的方法(如使用异常),但最佳实践是通过设置退出标志来实现线程的安全退出。这样可以确保线程在完成当前任务后平稳地终止。
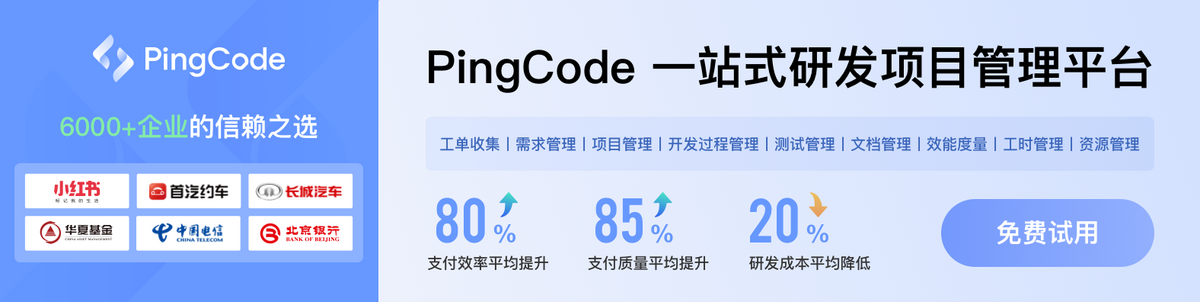