开头段落:
在Python中,保存print输出可以通过多种方式实现,如使用文件重定向、使用sys.stdout
、以及利用日志模块等。其中,最常用的方法之一是通过文件重定向,将标准输出重定向到文件中,以便将所有print输出保存到文件。例如,使用with open('output.txt', 'w') as f:
语句可以将print输出写入output.txt
文件。这样做的好处是简单易行,并且不需要额外的模块支持。接下来,我将详细介绍这些方法及其具体实现步骤。
正文:
一、文件重定向
在Python中,文件重定向是一种简单而有效的方式来保存print输出。通过将标准输出重定向到文件,可以直接将print函数的输出写入到指定的文件中。
- 基本实现
要实现文件重定向,可以使用Python的内置函数open()来打开一个文件,并将其设置为标准输出流。以下是一个简单的例子:
with open('output.txt', 'w') as f:
print('Hello, World!', file=f)
在这个例子中,print()
函数中的file
参数指定了输出目标为文件对象f
,这使得输出被写入到output.txt
文件中。
- 重定向整个程序的输出
如果想要重定向整个程序的输出,可以使用sys.stdout
来实现。sys.stdout
是Python的标准输出流,通过将其重定向到一个文件对象,可以捕获整个程序中的所有print输出:
import sys
打开文件
with open('output.txt', 'w') as f:
# 保存原始的标准输出流
original_stdout = sys.stdout
try:
# 重定向标准输出流到文件
sys.stdout = f
# 这里的print输出将被写入文件
print('This will be written to the file.')
finally:
# 恢复标准输出流
sys.stdout = original_stdout
在这个例子中,通过保存和恢复原始的sys.stdout
,保证了程序的其他部分不受影响。
二、使用日志模块
Python的日志模块提供了一个强大的日志记录功能,可以用于记录程序的运行信息,包括print输出。日志模块不仅可以将信息输出到控制台,还可以将其保存到文件中。
- 基本配置
首先,使用logging模块的基本配置功能,可以很容易地设置日志记录的格式和输出目的地:
import logging
设置日志格式和输出文件
logging.basicConfig(filename='output.log', level=logging.INFO, format='%(asctime)s - %(message)s')
记录信息
logging.info('This is an info message.')
在这个配置中,日志信息被写入output.log
文件,并包括时间戳和消息内容。
- 自定义日志记录器
对于更复杂的需求,可以创建自定义的日志记录器,以支持不同的日志级别和输出格式:
import logging
创建自定义日志记录器
logger = logging.getLogger('my_logger')
logger.setLevel(logging.DEBUG)
创建文件处理器
file_handler = logging.FileHandler('custom_output.log')
file_handler.setLevel(logging.DEBUG)
创建格式化器并添加到处理器
formatter = logging.Formatter('%(asctime)s - %(levelname)s - %(message)s')
file_handler.setFormatter(formatter)
将处理器添加到日志记录器
logger.addHandler(file_handler)
记录不同级别的信息
logger.debug('This is a debug message.')
logger.info('This is an info message.')
通过这种方式,可以灵活地控制日志的输出级别和格式,更好地满足不同场景下的需求。
三、使用上下文管理器
上下文管理器是Python中用于管理资源的一种方式,可以与文件重定向结合使用,以简化代码并提高可读性。
- 自定义上下文管理器
通过实现一个自定义上下文管理器,可以方便地进行文件重定向:
import sys
class RedirectOutput:
def __init__(self, filename):
self.filename = filename
def __enter__(self):
self.original_stdout = sys.stdout
self.file = open(self.filename, 'w')
sys.stdout = self.file
def __exit__(self, exc_type, exc_val, exc_tb):
sys.stdout = self.original_stdout
self.file.close()
使用自定义上下文管理器
with RedirectOutput('context_output.txt'):
print('This will be written to the file using a context manager.')
这个上下文管理器通过实现__enter__
和__exit__
方法来管理资源的打开和关闭,并确保在退出时恢复标准输出。
- 使用内置的contextlib模块
Python的contextlib模块提供了一些工具来简化上下文管理器的使用,例如redirect_stdout
,可以用于重定向标准输出:
import sys
from contextlib import redirect_stdout
使用redirect_stdout上下文管理器
with open('contextlib_output.txt', 'w') as f, redirect_stdout(f):
print('This will be written to the file using contextlib.')
这种方式更加简洁,并且利用了标准库中的现有工具,减少了自定义代码的复杂性。
四、捕获输出到变量
有时,在程序中需要捕获print输出到变量中而不是直接写入文件,这可以通过io.StringIO
对象来实现。
- 使用io.StringIO
io.StringIO
是一个内存中的文件对象,可以像文件一样操作,并用于捕获输出:
import io
import sys
创建StringIO对象
output = io.StringIO()
重定向标准输出到StringIO对象
with redirect_stdout(output):
print('Capture this output.')
获取捕获的内容
captured_output = output.getvalue()
print('Captured:', captured_output)
在这个例子中,输出被捕获到output
对象中,并可以通过getvalue()
方法获取。
- 实际应用
这种技术在测试环境中特别有用,可以用于捕获函数的输出并进行断言测试:
import io
import sys
from contextlib import redirect_stdout
def my_function():
print('This function prints something.')
def test_my_function():
output = io.StringIO()
with redirect_stdout(output):
my_function()
assert output.getvalue() == 'This function prints something.\n'
test_my_function()
通过这种方式,可以确保函数的输出符合预期,提供了一种有效的测试方法。
总结
综上所述,Python提供了多种方式来保存print输出,包括文件重定向、使用日志模块、上下文管理器以及捕获输出到变量中。每种方法都有其适用的场景和优缺点。通过选择合适的方法,可以有效地管理和记录程序的输出,提高代码的可读性和可维护性。
相关问答FAQs:
如何在Python中保存打印输出?
在Python中,可以使用文件操作来保存打印输出。通过重定向标准输出到文件,您可以将print
函数的结果写入文件中。例如,可以使用以下代码将输出保存到名为output.txt
的文件中:
with open('output.txt', 'w') as f:
print("Hello, World!", file=f)
这段代码会将“Hello, World!”写入output.txt
文件。
如何将多个打印输出保存到同一个文件中?
为了将多个打印输出保存到同一个文件中,可以在with
语句中多次调用print
函数。所有的输出都会追加到指定的文件中。例如:
with open('output.txt', 'w') as f:
print("First line", file=f)
print("Second line", file=f)
print("Third line", file=f)
这样会在output.txt
文件中依次写入三行内容。
有没有办法将打印输出同时显示在控制台和文件中?
可以通过自定义输出流来实现将打印输出同时显示在控制台和文件中。下面是一个简单的实现示例:
import sys
class Logger:
def __init__(self, filename):
self.terminal = sys.stdout
self.log = open(filename, 'w')
def write(self, message):
self.terminal.write(message)
self.log.write(message)
def flush(self):
pass
sys.stdout = Logger("output.txt")
print("This will be logged to both the console and the file.")
在运行这段代码后,您会在控制台和output.txt
文件中看到相同的输出。
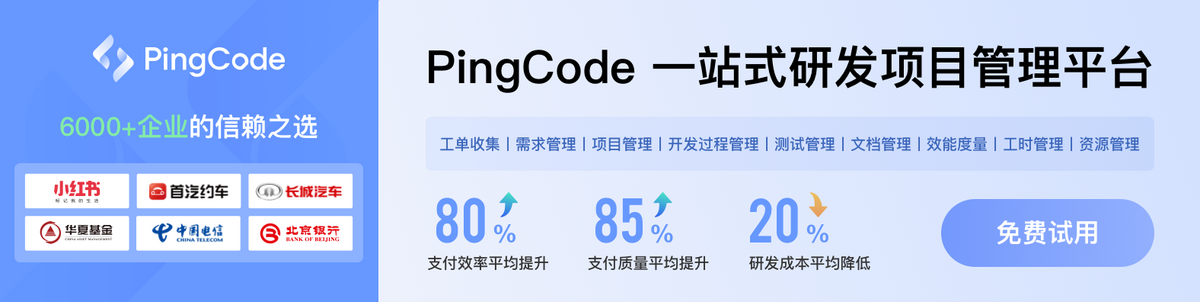