在Python中检测字符串是否符合特定条件,可以使用内置方法、正则表达式(regex)和自定义函数等方式。其中,使用in
操作符检查子字符串、使用字符串方法如startswith()
和endswith()
、通过正则表达式进行模式匹配是常见的手段。 例如,in
操作符可以用来检查一个字符串是否包含特定的子字符串,而startswith()
和endswith()
方法可以帮助判断字符串是否以特定字符或字符串开始或结束。这些方法在数据验证、格式检查和文本分析等方面都有广泛应用。
一、使用in
操作符检测子字符串
使用in
操作符是检测字符串中是否包含特定子字符串的最简单方法之一。它不仅简洁,而且高效,适用于大多数需要快速检查子字符串的场景。
-
基本用法
in
操作符用于检查一个字符串是否被包含在另一个字符串中。它返回一个布尔值,True
表示存在,False
表示不存在。例如:string = "Hello, World!"
if "World" in string:
print("Found!")
else:
print("Not found.")
在上面的例子中,
"World"
是string
的一部分,因此输出为"Found!"
。 -
忽略大小写
在某些情况下,您可能希望进行不区分大小写的子字符串检查。在这种情况下,可以将两个字符串都转换为小写或大写,然后再使用
in
操作符。string = "Hello, World!"
substring = "world"
if substring.lower() in string.lower():
print("Found!")
else:
print("Not found.")
这样,即使子字符串与原字符串的大小写不匹配,检查也会成功。
二、使用字符串方法startswith()
和endswith()
这些方法专门用于检测字符串是否以特定字符或子字符串开始或结束,适用于验证字符串格式。
-
startswith()
方法startswith()
方法用于检查字符串是否以指定的前缀开头。它返回True
或False
。string = "Hello, World!"
if string.startswith("Hello"):
print("String starts with 'Hello'.")
else:
print("String does not start with 'Hello'.")
-
endswith()
方法endswith()
方法用于检查字符串是否以指定的后缀结尾。string = "Hello, World!"
if string.endswith("World!"):
print("String ends with 'World!'.")
else:
print("String does not end with 'World!'.")
这些方法非常适合用于文件名验证、路径检查等需要识别特定前缀或后缀的情境。
三、使用正则表达式进行复杂模式匹配
正则表达式是一种强大的工具,用于进行复杂的字符串模式匹配。Python的re
模块提供了正则表达式的支持。
-
基本匹配
使用
re.search()
函数可以在字符串中搜索正则表达式模式。它返回一个匹配对象,如果没有找到匹配则返回None
。import re
string = "Hello, World!"
pattern = r"World"
if re.search(pattern, string):
print("Pattern found!")
else:
print("Pattern not found.")
-
忽略大小写
在正则表达式中,可以通过
re.IGNORECASE
标志来忽略大小写。if re.search(pattern, string, re.IGNORECASE):
print("Pattern found (case-insensitive)!")
-
复杂模式
正则表达式可以用于查找复杂的模式,例如电话号码、电子邮件地址等。
email = "example@example.com"
pattern = r"[^@]+@[^@]+\.[^@]+"
if re.match(pattern, email):
print("Valid email address.")
else:
print("Invalid email address.")
正则表达式在日志分析、文本处理和数据提取等方面有着广泛的应用。
四、自定义函数进行特定检测
在某些情况下,内置方法和正则表达式可能无法满足所有需求。这时,可以编写自定义函数来实现特定的字符串检测逻辑。
-
检测回文
例如,编写一个函数来检测字符串是否为回文(即无论正反方向读都相同)。
def is_palindrome(s):
s = s.lower().replace(" ", "")
return s == s[::-1]
string = "A man a plan a canal Panama"
if is_palindrome(string):
print("The string is a palindrome.")
else:
print("The string is not a palindrome.")
-
检测特定格式
可以编写函数来检测字符串是否符合特定格式,例如日期格式、IP地址格式等。
def is_valid_date(date_string):
from datetime import datetime
try:
datetime.strptime(date_string, "%Y-%m-%d")
return True
except ValueError:
return False
date_string = "2023-11-01"
if is_valid_date(date_string):
print("Valid date format.")
else:
print("Invalid date format.")
自定义函数提供了灵活性,可以根据具体需求进行个性化的字符串检测。
五、结合多种方法进行高级字符串检测
在实际应用中,可能需要结合多种方法来实现复杂的字符串检测任务。例如,在处理用户输入、数据清洗或文本分析时,往往需要灵活运用多种技术。
-
组合使用内置方法和正则表达式
在处理文本时,可以组合使用内置字符串方法和正则表达式,以便在不同的条件下进行检测。
def check_string(s):
if s.startswith("Error") and re.search(r"\d{3}", s):
print("String indicates an error with a code.")
else:
print("String does not match the criteria.")
log_string = "Error 404: Not Found"
check_string(log_string)
-
结合数据结构和字符串检测
在某些应用中,可能需要结合数据结构(如列表、字典)和字符串检测来实现更复杂的逻辑。
def find_keywords(text, keywords):
found_keywords = [keyword for keyword in keywords if keyword in text]
return found_keywords
text = "Python is a versatile programming language."
keywords = ["Python", "Java", "programming"]
matched_keywords = find_keywords(text, keywords)
print("Matched keywords:", matched_keywords)
这种方法适用于需要在大文本中查找多个关键字的情境。
六、性能优化与注意事项
在进行字符串检测时,效率和性能往往是需要考虑的重要因素。对于大规模数据处理或实时系统,优化字符串检测的性能是关键。
-
避免重复计算
在某些情况下,可以通过缓存或提前计算来避免重复计算。例如,在需要多次检测相同的子字符串时,可以先将其结果存储起来。
def find_substrings(text, substrings):
results = {}
for substring in substrings:
results[substring] = substring in text
return results
text = "This is a sample text for substring detection."
substrings = ["sample", "text", "Python"]
results = find_substrings(text, substrings)
print(results)
-
选择合适的方法
根据具体需求选择合适的方法。例如,对于简单的子字符串检测,
in
操作符通常比正则表达式更高效;对于复杂模式匹配,则可以使用正则表达式。import time
def measure_time(func, *args):
start = time.time()
func(*args)
end = time.time()
return end - start
text = "a" * 1000000 + "b"
print("Using 'in':", measure_time(lambda: "b" in text))
print("Using regex:", measure_time(lambda: re.search(r"b", text)))
通过合理选择和组合不同的字符串检测方法,可以有效提升程序的性能和可靠性。
总结而言,Python提供了丰富的工具和方法来进行字符串检测。通过内置方法、正则表达式和自定义函数,可以灵活实现从简单到复杂的字符串检测任务。在实际应用中,结合多种技术和优化手段,可以提高检测的准确性和效率。无论是在数据处理、文本分析还是用户输入验证中,字符串检测都是一个至关重要的环节。
相关问答FAQs:
如何在Python中检测字符串的类型?
在Python中,可以使用内置的isinstance()
函数来检测一个变量是否为字符串类型。示例如下:
my_var = "Hello, World!"
if isinstance(my_var, str):
print("这是一个字符串。")
else:
print("这不是一个字符串。")
这种方法适用于确定一个变量的类型,非常直观且易于理解。
Python中如何检查字符串是否为空?
要检查字符串是否为空,可以直接使用条件语句。空字符串在布尔上下文中被视为False
。示例代码如下:
my_str = ""
if not my_str:
print("字符串为空。")
else:
print("字符串不为空。")
这种方法简单明了,适合在多个场合下使用。
在Python中如何判断字符串是否包含特定子串?
可以使用in
运算符来检测一个字符串是否包含另一个字符串。例如:
main_str = "Python编程非常有趣"
sub_str = "编程"
if sub_str in main_str:
print("主字符串包含子字符串。")
else:
print("主字符串不包含子字符串。")
这种方式不仅简洁,而且易于理解,适合用于字符串的查找操作。
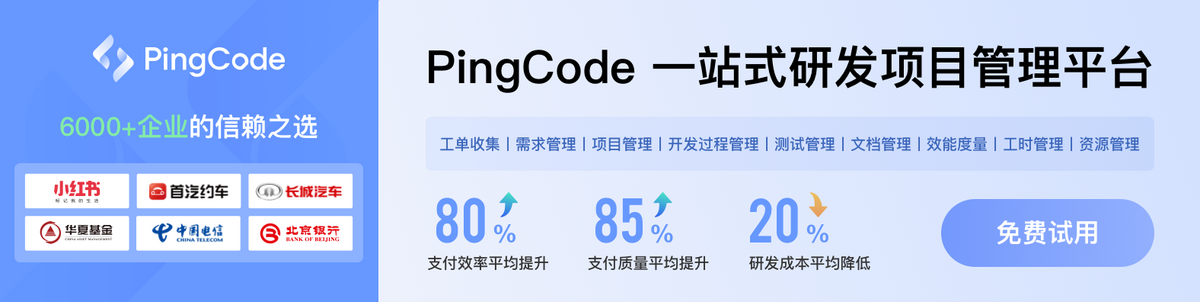