要在Python中使用jQuery,可以借助于Web框架和工具、将jQuery与HTML模板结合、使用Flask或Django等框架集成前后端。在Python环境中直接使用jQuery是不可能的,因为jQuery是一个专门用于操作浏览器中的HTML文档的JavaScript库,而Python是一种后端编程语言。然而,可以通过一些方法在Python项目中有效地利用jQuery的强大功能。首先,你可以使用Web框架如Flask或Django来构建Web应用程序,在这些框架中,你可以将jQuery脚本嵌入到HTML模板中,从而使jQuery与Python后端通信。其次,使用Ajax技术,jQuery可以与Python后端进行异步数据交换,实现动态更新网页内容的功能。
一、使用WEB框架(如Flask、Django)来集成jQuery
在Python Web开发中,Flask和Django是两种流行的框架。它们都支持将jQuery集成到Web应用中。通过这些框架,开发者可以在Python后端处理业务逻辑和数据,然后使用jQuery在前端进行动态交互。
- Flask与jQuery集成
Flask是一个轻量级的Web框架,非常适合小型项目和原型开发。要在Flask项目中使用jQuery,你首先需要创建一个Flask应用,并在HTML模板中引入jQuery库。以下是一个简单的示例:
# app.py
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def home():
return render_template('index.html')
if __name__ == '__main__':
app.run(debug=True)
在项目的templates
文件夹中,创建一个index.html
文件,并在其中引入jQuery:
<!-- templates/index.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Flask with jQuery</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<h1>Hello, Flask with jQuery!</h1>
<button id="myButton">Click Me</button>
<script>
$(document).ready(function(){
$('#myButton').click(function(){
alert('Button clicked!');
});
});
</script>
</body>
</html>
在这个示例中,Flask用于渲染HTML模板,而jQuery用于处理按钮的点击事件。
- Django与jQuery集成
Django是一个功能更全面的Web框架,适用于较大规模的项目。Django的模板系统同样支持集成jQuery。以下是一个Django项目的简单示例:
首先,创建一个Django项目和应用:
django-admin startproject myproject
cd myproject
django-admin startapp myapp
在myapp
目录下的views.py
文件中定义视图:
# myapp/views.py
from django.shortcuts import render
def home(request):
return render(request, 'index.html')
在myproject/urls.py
中配置URL:
# myproject/urls.py
from django.contrib import admin
from django.urls import path
from myapp import views
urlpatterns = [
path('admin/', admin.site.urls),
path('', views.home, name='home'),
]
创建templates
目录,并在其中创建index.html
文件:
<!-- templates/index.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Django with jQuery</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<h1>Hello, Django with jQuery!</h1>
<button id="myButton">Click Me</button>
<script>
$(document).ready(function(){
$('#myButton').click(function(){
alert('Button clicked!');
});
});
</script>
</body>
</html>
在这个Django示例中,jQuery同样用于处理前端事件。
二、使用AJAX进行数据交换
Ajax(异步JavaScript和XML)是一种在不重新加载整个网页的情况下与服务器交换数据的技术。通过Ajax,jQuery可以与Python后端进行数据交互。
- 在Flask中使用Ajax
在Flask应用中,你可以使用Ajax从客户端发送请求到服务器端,服务器端处理请求后返回数据给客户端。以下是一个简单的示例:
在app.py
中添加一个路由来处理Ajax请求:
# app.py
from flask import Flask, render_template, jsonify
app = Flask(__name__)
@app.route('/')
def home():
return render_template('index.html')
@app.route('/get_data')
def get_data():
return jsonify({'message': 'Hello from Flask!'})
if __name__ == '__main__':
app.run(debug=True)
在index.html
中使用jQuery的Ajax功能:
<!-- templates/index.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Flask Ajax Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<h1>Flask Ajax Example</h1>
<button id="myButton">Get Data</button>
<p id="response"></p>
<script>
$(document).ready(function(){
$('#myButton').click(function(){
$.ajax({
url: '/get_data',
method: 'GET',
success: function(response) {
$('#response').text(response.message);
}
});
});
});
</script>
</body>
</html>
在这个示例中,当用户点击按钮时,jQuery会发送Ajax请求到Flask服务器,并将返回的消息显示在页面上。
- 在Django中使用Ajax
在Django项目中,也可以使用Ajax实现类似的功能:
在myapp/views.py
中定义一个处理Ajax请求的视图:
# myapp/views.py
from django.http import JsonResponse
from django.shortcuts import render
def home(request):
return render(request, 'index.html')
def get_data(request):
return JsonResponse({'message': 'Hello from Django!'})
在myproject/urls.py
中添加URL配置:
# myproject/urls.py
from django.contrib import admin
from django.urls import path
from myapp import views
urlpatterns = [
path('admin/', admin.site.urls),
path('', views.home, name='home'),
path('get_data/', views.get_data, name='get_data'),
]
在index.html
中使用Ajax与Django后端通信:
<!-- templates/index.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Django Ajax Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<h1>Django Ajax Example</h1>
<button id="myButton">Get Data</button>
<p id="response"></p>
<script>
$(document).ready(function(){
$('#myButton').click(function(){
$.ajax({
url: '/get_data/',
method: 'GET',
success: function(response) {
$('#response').text(response.message);
}
});
});
});
</script>
</body>
</html>
在这个Django示例中,Ajax请求被发送到Django服务器,服务器返回的数据被显示在网页上。
三、在前后端之间传递数据
在Web开发中,前后端之间的数据传递是一个重要的环节。通过jQuery和Ajax,可以在不刷新页面的情况下,动态地与服务器进行数据交换。
- 发送和接收JSON数据
JSON(JavaScript Object Notation)是一种轻量级的数据交换格式,易于阅读和编写,同时也易于机器解析和生成。JSON是前后端数据交换的常用格式。
在Flask或Django中,可以使用JSON数据在前后端之间传递信息。例如,在Flask中,你可以返回一个JSON响应:
# app.py (Flask)
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/get_json')
def get_json():
data = {'name': 'John', 'age': 30}
return jsonify(data)
if __name__ == '__main__':
app.run(debug=True)
然后在前端使用jQuery的Ajax请求:
<!-- index.html -->
<script>
$(document).ready(function(){
$('#myButton').click(function(){
$.ajax({
url: '/get_json',
method: 'GET',
success: function(response) {
console.log(response);
}
});
});
});
</script>
在Django中,你可以以类似的方式返回JSON数据:
# myapp/views.py (Django)
from django.http import JsonResponse
def get_json(request):
data = {'name': 'John', 'age': 30}
return JsonResponse(data)
配置URL并在前端发送Ajax请求:
<!-- index.html -->
<script>
$(document).ready(function(){
$('#myButton').click(function(){
$.ajax({
url: '/get_json/',
method: 'GET',
success: function(response) {
console.log(response);
}
});
});
});
</script>
- 处理表单提交
在Web应用中,表单提交是获取用户输入的重要方式。通过jQuery和Ajax,可以在不刷新页面的情况下提交表单数据。
在Flask中,你可以处理表单提交并返回响应:
# app.py (Flask)
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route('/submit_form', methods=['POST'])
def submit_form():
name = request.form.get('name')
return jsonify({'message': f'Hello, {name}!'})
if __name__ == '__main__':
app.run(debug=True)
在前端,使用jQuery Ajax提交表单:
<!-- index.html -->
<form id="myForm">
<input type="text" name="name" placeholder="Enter your name">
<button type="submit">Submit</button>
</form>
<p id="response"></p>
<script>
$(document).ready(function(){
$('#myForm').submit(function(event){
event.preventDefault();
$.ajax({
url: '/submit_form',
method: 'POST',
data: $(this).serialize(),
success: function(response) {
$('#response').text(response.message);
}
});
});
});
</script>
在Django中,处理表单提交与Flask类似:
# myapp/views.py (Django)
from django.http import JsonResponse
from django.views.decorators.csrf import csrf_exempt
@csrf_exempt
def submit_form(request):
if request.method == 'POST':
name = request.POST.get('name')
return JsonResponse({'message': f'Hello, {name}!'})
配置URL并在前端提交表单:
<!-- index.html -->
<form id="myForm">
<input type="text" name="name" placeholder="Enter your name">
<button type="submit">Submit</button>
</form>
<p id="response"></p>
<script>
$(document).ready(function(){
$('#myForm').submit(function(event){
event.preventDefault();
$.ajax({
url: '/submit_form/',
method: 'POST',
data: $(this).serialize(),
success: function(response) {
$('#response').text(response.message);
}
});
});
});
</script>
通过这些示例,我们可以看到如何在Python Web框架中有效地使用jQuery和Ajax进行动态交互和数据交换。在开发过程中,请确保正确配置CSRF(跨站请求伪造)保护,以保障应用程序的安全。
相关问答FAQs:
如何在Python项目中集成jQuery?
在Python项目中使用jQuery,通常需要通过Web框架(如Flask或Django)来构建前端和后端交互。可以将jQuery库通过CDN链接或直接下载到项目中,然后在HTML模板中引用它。确保在加载jQuery之前,HTML文档的DOM元素已经渲染完毕,以避免脚本错误。
使用jQuery可以实现哪些Python项目中的前端功能?
jQuery可以为Python项目的前端提供丰富的交互功能。例如,可以通过jQuery简化AJAX请求,动态加载数据而不刷新页面;也可以创建动态表单验证、动画效果和用户界面交互,提升用户体验。这些功能能够使Web应用更加生动和易于使用。
在Python后端如何处理jQuery发送的AJAX请求?
对于使用jQuery发送的AJAX请求,Python后端可以通过相应的路由来处理。一般来说,使用Flask或Django等框架可以轻松设置路由,并解析请求中的数据。返回的数据可以是JSON格式,方便jQuery在前端进行进一步处理和展示。确保在后端设置正确的响应头,以支持跨域请求(CORS),如果需要的话。
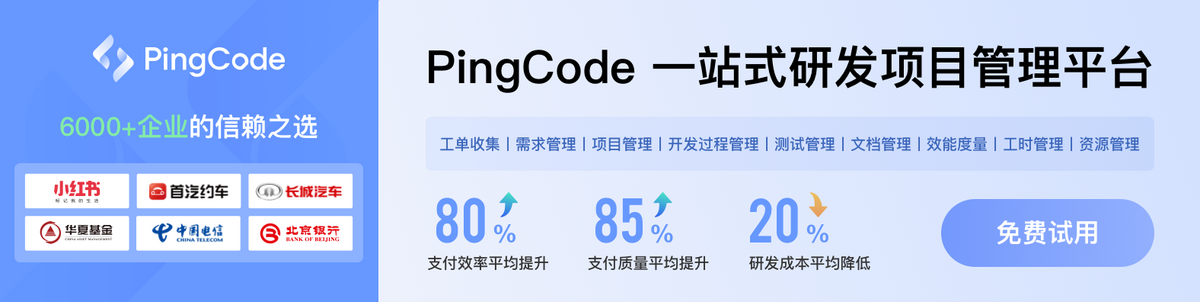