在Python中去重的方法有多种,主要包括使用集合、字典、列表推导式、pandas库等。使用集合去重是最常用的方法,因为集合本身不允许重复元素,操作简单且高效。此外,字典的键也不允许重复,可以通过字典来去重。具体选择哪种方法取决于数据类型和具体需求。下面将详细介绍这些方法及其使用场景。
一、使用集合(set)去重
集合是Python中一种内置的数据结构,其特点是不允许重复元素,因此可以利用这一特性来进行去重操作。
- 集合去重的基本用法
将列表转换为集合,集合会自动去除重复元素,然后再将其转换回列表。
list_with_duplicates = [1, 2, 2, 3, 4, 4, 5]
list_without_duplicates = list(set(list_with_duplicates))
print(list_without_duplicates)
这种方法的优点是简单快捷,适用于去重后不要求保持原列表顺序的场景。
- 保持顺序的集合去重
如果需要去重同时保持原有顺序,可以使用集合配合for循环实现:
list_with_duplicates = [1, 2, 2, 3, 4, 4, 5]
seen = set()
list_without_duplicates = []
for item in list_with_duplicates:
if item not in seen:
list_without_duplicates.append(item)
seen.add(item)
print(list_without_duplicates)
这种方法在去重的同时保留了列表的原始顺序。
二、使用字典(dict)去重
在Python 3.7及更高版本中,字典是有序的,可以利用字典的键唯一性来去重并保持顺序。
list_with_duplicates = [1, 2, 2, 3, 4, 4, 5]
list_without_duplicates = list(dict.fromkeys(list_with_duplicates))
print(list_without_duplicates)
这种方法同样能够在去重的同时保持列表的顺序,并且代码更加简洁。
三、使用列表推导式去重
列表推导式是一种优雅的Python特性,可以用于去重操作。
- 配合集合使用
list_with_duplicates = [1, 2, 2, 3, 4, 4, 5]
seen = set()
list_without_duplicates = [x for x in list_with_duplicates if not (x in seen or seen.add(x))]
print(list_without_duplicates)
这种方法通过列表推导式与集合的结合,实现了去重并保持顺序。
- 基于索引去重
通过索引和列表推导式结合,也可以实现去重。
list_with_duplicates = [1, 2, 2, 3, 4, 4, 5]
list_without_duplicates = [list_with_duplicates[i] for i in range(len(list_with_duplicates)) if list_with_duplicates[i] not in list_with_duplicates[:i]]
print(list_without_duplicates)
这种方法虽然较为复杂,但可以在某些特定场景下使用。
四、使用pandas库去重
对于大规模数据,尤其是数据框(DataFrame),可以使用pandas库的去重功能。
- pandas去重
import pandas as pd
df = pd.DataFrame({'column': [1, 2, 2, 3, 4, 4, 5]})
df_without_duplicates = df.drop_duplicates()
print(df_without_duplicates)
pandas的drop_duplicates()
方法可以直接用于数据框去重,非常高效。
- 多列去重
如果需要对多列组合进行去重,pandas也提供了便捷的方法。
df = pd.DataFrame({'col1': [1, 2, 2, 3], 'col2': [4, 4, 5, 5]})
df_without_duplicates = df.drop_duplicates(subset=['col1', 'col2'])
print(df_without_duplicates)
这种方法适用于需要对多列数据进行组合去重的情况。
五、其他高级去重方法
- 使用numpy库去重
对于数值数据,numpy库也是一个高效的选择。
import numpy as np
array_with_duplicates = np.array([1, 2, 2, 3, 4, 4, 5])
array_without_duplicates = np.unique(array_with_duplicates)
print(array_without_duplicates)
numpy的unique
方法可以高效去除数组中的重复元素。
- 结合排序进行去重
在某些情况下,可以通过先排序再去重的方式达到去重效果。
list_with_duplicates = [1, 2, 2, 3, 4, 4, 5]
list_with_duplicates.sort()
list_without_duplicates = [list_with_duplicates[i] for i in range(len(list_with_duplicates)) if i == 0 or list_with_duplicates[i] != list_with_duplicates[i - 1]]
print(list_without_duplicates)
这种方法适用于需要去重且不关心原始顺序的情况。
综上所述,Python中有多种方法可以实现去重操作,选择合适的方法可以大大提高代码的效率和可读性。根据具体需求(如是否保持原有顺序、数据规模、数据类型等)选择合适的去重策略尤为重要。无论是使用集合、字典,还是利用pandas和numpy库,都可以实现高效的去重操作。
相关问答FAQs:
如何在Python中使用集合去重?
集合是Python中一种内置的数据结构,能够自动去除重复项。您可以通过将列表转换为集合来快速实现去重。例如,使用set(your_list)
可以轻松创建一个不包含重复元素的新集合。需要注意的是,集合是无序的,如果顺序很重要,可以考虑其他方法。
Python中有哪些去重的常用方法?
除了使用集合,您还可以利用列表推导式和dict.fromkeys()
方法进行去重。列表推导式可以遍历列表并生成一个新列表,其中仅包含第一次出现的元素。dict.fromkeys()
方法则利用字典的特性,能够在保持元素顺序的同时去重。
如何在Python中处理复杂数据类型的去重?
对于包含字典或自定义对象的复杂数据结构,可以使用自定义函数进行去重。例如,通过定义一个函数来比较对象的特定属性,可以创建一个新的列表或集合来存储唯一对象。此外,pandas
库也提供了方便的方法,如drop_duplicates()
,适用于数据分析和处理。
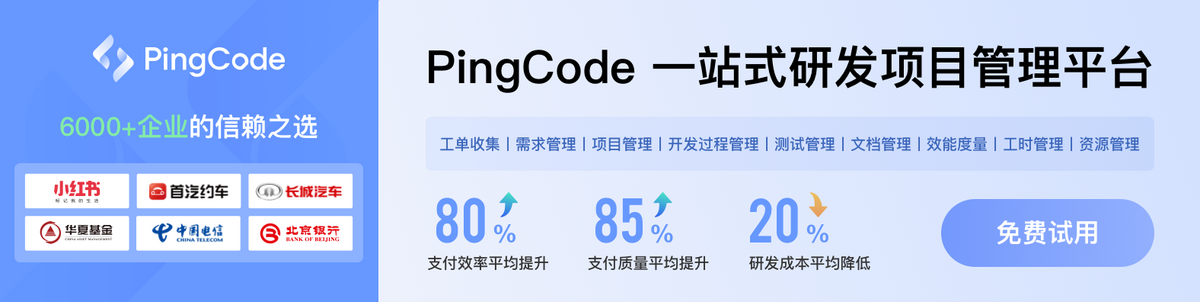