Python调用页面的方法主要包括使用请求库获取页面内容、使用Selenium进行页面交互、使用BeautifulSoup进行页面解析。其中,使用请求库是最基础的方法,可以用于获取页面的HTML内容;使用Selenium可以进行动态页面的加载和交互操作;使用BeautifulSoup可以方便地解析和提取页面中的数据。下面将详细介绍这几种方法及其应用。
一、使用请求库(Requests)获取页面内容
请求库(Requests)是Python中最常用的HTTP库之一,可以非常方便地发送HTTP请求并获取响应。
- 安装和基本用法
首先,我们需要安装Requests库。可以使用pip命令进行安装:
pip install requests
安装完成后,可以通过以下方式使用Requests库获取网页内容:
import requests
url = "http://example.com"
response = requests.get(url)
if response.status_code == 200:
print("Successfully fetched the page")
print(response.text)
else:
print("Failed to retrieve the page")
在上面的代码中,我们使用requests.get()
方法发送一个GET请求,并通过response.text
获取页面的HTML内容。
- 设置请求头和参数
有时候,我们需要模拟浏览器行为来获取网页内容,这时可以通过设置请求头来实现:
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
此外,如果页面需要传递参数,可以通过params
参数来实现:
params = {'key1': 'value1', 'key2': 'value2'}
response = requests.get(url, params=params)
二、使用Selenium进行页面交互
Selenium是一个强大的工具,可以用来自动化测试Web应用程序。它可以模拟用户在浏览器上的操作,非常适合处理动态加载的网页。
- 安装和基本用法
首先,需要安装Selenium库和对应浏览器的WebDriver。以Chrome为例:
pip install selenium
然后下载ChromeDriver并将其路径添加到系统环境变量中。
使用Selenium获取页面内容的基本步骤如下:
from selenium import webdriver
设置WebDriver
driver = webdriver.Chrome()
打开网页
driver.get("http://example.com")
获取页面内容
page_source = driver.page_source
print(page_source)
关闭浏览器
driver.quit()
- 页面交互
Selenium不仅可以获取页面内容,还可以进行交互操作,如点击按钮、填写表单等:
# 查找元素并点击
button = driver.find_element_by_id("submit-button")
button.click()
输入文本
input_box = driver.find_element_by_name("username")
input_box.send_keys("myusername")
三、使用BeautifulSoup解析页面内容
BeautifulSoup是一个用于解析HTML和XML文档的Python库,它可以方便地从网页中提取数据。
- 安装和基本用法
首先安装BeautifulSoup和lxml解析器:
pip install beautifulsoup4 lxml
然后使用BeautifulSoup解析网页内容:
from bs4 import BeautifulSoup
html_content = response.text
soup = BeautifulSoup(html_content, 'lxml')
查找所有链接
for link in soup.find_all('a'):
print(link.get('href'))
- 提取特定数据
可以通过BeautifulSoup的各种查找方法来提取特定数据:
# 提取标题
title = soup.title.string
print("Page Title:", title)
提取特定ID的元素
specific_div = soup.find(id="specific-id")
print(specific_div.text)
提取所有段落
for paragraph in soup.find_all('p'):
print(paragraph.text)
四、组合使用Requests和BeautifulSoup
Requests和BeautifulSoup的组合使用是获取和解析静态网页的经典方法。
- 获取页面并解析
首先使用Requests库获取网页内容,然后用BeautifulSoup进行解析:
import requests
from bs4 import BeautifulSoup
url = "http://example.com"
response = requests.get(url)
soup = BeautifulSoup(response.content, 'lxml')
提取页面信息
print(soup.title.string)
- 处理复杂页面
对于结构复杂的页面,可以结合CSS选择器进行数据提取:
# 使用CSS选择器提取信息
for item in soup.select('.item-class'):
print(item.text)
五、使用Scrapy框架进行爬虫开发
Scrapy是一个强大的爬虫框架,适合大规模数据抓取。
- 安装和基本用法
安装Scrapy:
pip install scrapy
创建一个Scrapy项目:
scrapy startproject myproject
定义爬虫:
import scrapy
class MySpider(scrapy.Spider):
name = 'myspider'
start_urls = ['http://example.com']
def parse(self, response):
for title in response.css('title::text'):
yield {'title': title.get()}
- 运行爬虫
在项目目录下运行爬虫:
scrapy crawl myspider
六、使用API接口获取数据
有些网站提供API接口,可以直接通过接口获取数据,而不需要解析网页。
- 了解API文档
首先需要查看目标网站是否提供API接口,并阅读相关文档以了解如何使用。
- 发送请求获取数据
使用Requests库发送请求获取数据:
api_url = "http://api.example.com/data"
response = requests.get(api_url)
if response.status_code == 200:
data = response.json()
print(data)
七、处理JavaScript渲染的页面
有些页面需要通过JavaScript渲染后才能获取完整内容,这时可以使用Selenium或Splash等工具。
- 使用Selenium
如前所述,Selenium可以加载和渲染JavaScript,并获取渲染后的页面内容。
- 使用Splash
Splash是一个JavaScript渲染服务,可以与Scrapy集成使用。
安装Splash并启动服务后,可以在Scrapy中使用:
from scrapy_splash import SplashRequest
class MySpider(scrapy.Spider):
name = 'myspider'
start_urls = ['http://example.com']
def start_requests(self):
for url in self.start_urls:
yield SplashRequest(url, self.parse, args={'wait': 1})
def parse(self, response):
yield {'title': response.css('title::text').get()}
通过以上几种方法,Python可以灵活地调用和处理网页内容,满足各种数据获取的需求。根据具体的应用场景和页面特性,可以选择适合的方法实现网页数据的抓取和处理。
相关问答FAQs:
如何在Python中实现页面的调用?
在Python中,调用网页通常可以通过使用HTTP请求库来实现。常见的库包括requests
和urllib
。使用requests
库,你可以轻松发送GET或POST请求,以获取网页内容。
使用requests库调用网页的步骤是什么?
- 首先,确保已安装
requests
库,可以通过运行pip install requests
来安装。 - 接下来,使用
requests.get(url)
发送GET请求,获取网页内容。 - 可以通过
response.text
访问返回的HTML内容,或者使用response.json()
解析JSON格式的响应。
调用网页时,如何处理异常和错误?
在调用网页时,可能会遇到网络问题或请求错误。可以使用try...except
语句来处理这些异常,确保程序的稳定性。例如,可以捕获requests.exceptions.RequestException
,并输出相应的错误信息。这样可以帮助你及时发现问题并采取措施。
是否可以在Python中解析网页内容?
可以使用BeautifulSoup
库来解析网页内容。通过安装beautifulsoup4
库并结合requests
使用,可以提取网页中的特定数据,比如标题、链接、文本等。首先获取网页内容,然后使用BeautifulSoup
解析HTML,最后使用相应的方法提取所需信息。这样就能方便地处理网页数据。
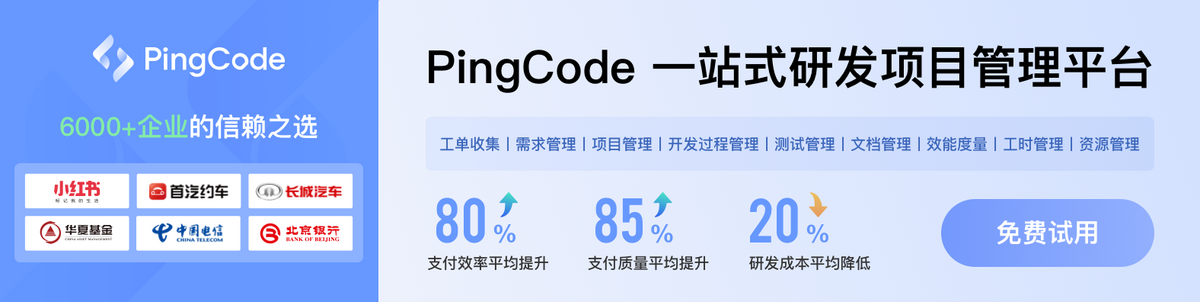