Python中没有原生的switch语句,但是可以通过多种方式实现类似的功能,比如使用字典映射、if-elif-else结构或者定义函数来模拟、提高代码的可读性和维护性。其中,使用字典映射是一种较为常用的方法,因为它简洁且易于理解。我们将详细探讨如何在Python中使用这些方法来模拟switch语句的功能。
一、字典映射实现switch功能
字典映射是一种简单而有效的方法,通过将可能的条件作为字典的键,将对应的操作作为字典的值,来模拟switch语句的功能。
- 字典映射的基本用法
在Python中,字典是一种非常强大的数据结构,可以用来存储键值对。我们可以使用字典来映射不同的条件到对应的操作函数。通过访问字典的键来执行相应的操作。
def case_one():
return "This is case one"
def case_two():
return "This is case two"
def default_case():
return "This is the default case"
def switch_case(case_value):
switch_dict = {
1: case_one,
2: case_two
}
# 使用get方法可以设置默认值
return switch_dict.get(case_value, default_case)()
print(switch_case(1)) # 输出: This is case one
print(switch_case(3)) # 输出: This is the default case
- 使用lambda表达式简化代码
如果操作比较简单,可以使用lambda表达式进一步简化代码,使得代码更加简洁。
def switch_case(case_value):
switch_dict = {
1: lambda: "This is case one",
2: lambda: "This is case two"
}
return switch_dict.get(case_value, lambda: "This is the default case")()
print(switch_case(1)) # 输出: This is case one
print(switch_case(3)) # 输出: This is the default case
二、if-elif-else结构实现switch功能
虽然字典映射是一种推荐的方式,但在某些情况下,使用if-elif-else结构可能更直观,尤其是在需要处理复杂逻辑时。
- 基本使用
if-elif-else结构是Python中最常用的条件控制结构,通过一系列的条件判断来选择执行的代码块。
def switch_case(case_value):
if case_value == 1:
return "This is case one"
elif case_value == 2:
return "This is case two"
else:
return "This is the default case"
print(switch_case(1)) # 输出: This is case one
print(switch_case(3)) # 输出: This is the default case
- 优化if-elif-else结构
当条件数量较多时,可以考虑将条件判断提取到单独的函数中,以提高代码的可读性和可维护性。
def case_one():
return "This is case one"
def case_two():
return "This is case two"
def default_case():
return "This is the default case"
def switch_case(case_value):
if case_value == 1:
return case_one()
elif case_value == 2:
return case_two()
else:
return default_case()
print(switch_case(1)) # 输出: This is case one
print(switch_case(3)) # 输出: This is the default case
三、使用类实现switch功能
在某些情况下,我们可能希望使用面向对象的方式来模拟switch语句。可以通过定义一个类来封装不同的case逻辑。
- 定义一个Switch类
定义一个类来包含所有的case逻辑,并提供一个方法来执行相应的操作。
class Switch:
def case_one(self):
return "This is case one"
def case_two(self):
return "This is case two"
def default_case(self):
return "This is the default case"
def switch_case(self, case_value):
method_name = f"case_{case_value}"
method = getattr(self, method_name, self.default_case)
return method()
switch = Switch()
print(switch.switch_case(1)) # 输出: This is case one
print(switch.switch_case(3)) # 输出: This is the default case
- 优化Switch类
可以通过将case逻辑提取到单独的方法中来进一步优化Switch类。
class Switch:
def case_one(self):
return "This is case one"
def case_two(self):
return "This is case two"
def default_case(self):
return "This is the default case"
def switch_case(self, case_value):
method_name = f"case_{case_value}"
method = getattr(self, method_name, self.default_case)
return method()
switch = Switch()
print(switch.switch_case(1)) # 输出: This is case one
print(switch.switch_case(3)) # 输出: This is the default case
四、总结
在Python中,虽然没有原生的switch语句,但可以通过字典映射、if-elif-else结构或定义类来模拟switch功能。字典映射是一种常用的方法,因为它简洁且易于理解。对于复杂的逻辑,使用if-elif-else结构可能更合适,而在需要面向对象编程时,可以考虑使用类来实现。选择哪种方法取决于具体的应用场景和代码风格偏好。
相关问答FAQs:
在Python中,如何实现类似于switch-case的功能?
Python本身并没有内置的switch-case语句,但可以使用字典来模拟这一功能。通过将函数或值与键关联,可以实现多重条件判断。例如,可以定义一个字典,其中每个键对应一个可能的输入,每个值是要执行的代码块。这种方式不仅清晰易懂,还能提高代码的可维护性。
使用if-elif-else结构和switch-case有什么区别?
if-elif-else结构是Python中处理多重条件的标准方式。相比之下,使用字典模拟的switch-case在某些情况下更加简洁和高效,尤其是在条件判断较多时。if-elif-else适合处理复杂的逻辑判断,而字典方式则更适合简单的值映射。
在Python中,如何处理switch-case中的默认情况?
在使用字典模拟switch-case时,可以通过设置一个默认值来处理未匹配的情况。可以在字典中使用get()
方法,提供一个默认返回值。这种方式可以避免KeyError,并确保在没有匹配项时仍能返回预期的输出。这种灵活性使得代码更加健壮。
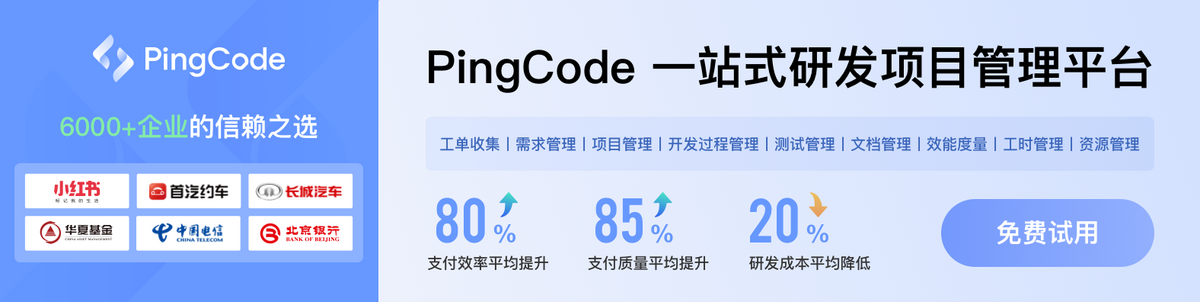