Python下载匹配文件的常用方法包括使用正则表达式、os库、glob库等工具,可以帮助我们高效地从文件夹或网络中找到并下载特定格式或名称的文件。在实际应用中,正则表达式灵活且强大,os库适合本地文件管理,而glob库则提供了一种简便的文件模式匹配方法。下面将详细介绍如何使用这些工具来实现Python的下载匹配功能。
一、使用正则表达式进行文件匹配
正则表达式(Regular Expression)是一种强大的字符串处理工具,可以用来匹配复杂的字符串模式。在Python中,正则表达式由re模块提供支持。
-
基本用法
使用正则表达式进行匹配时,首先需要编译一个正则表达式对象,然后使用该对象的match或search方法来查找匹配项。
import re
pattern = re.compile(r'\d{4}_report\.pdf')
file_list = ['2020_report.pdf', '2021_report.pdf', 'summary.txt']
for file in file_list:
if pattern.match(file):
print(f"Matched file: {file}")
这个示例中,我们使用正则表达式匹配文件名中包含的年份和特定的文件类型(.pdf)。
-
高级匹配
除了简单的匹配,正则表达式还支持更复杂的模式,例如匹配文件名中的特定字符串或数字范围。
pattern = re.compile(r'(202[0-2])_report\.pdf')
这个模式匹配2020到2022年之间的报告文件。
二、使用os库进行文件管理
os库是Python中用于操作系统功能的标准库,可以用来遍历目录结构,查找并管理文件。
-
遍历目录
使用os.walk可以遍历目录树,获取所有文件名。
import os
for root, dirs, files in os.walk('/path/to/search'):
for file in files:
if file.endswith('.pdf'):
print(f"Found PDF file: {file}")
这种方法适合用来查找特定类型的文件,比如PDF或图像文件。
-
文件路径操作
os.path模块提供了丰富的文件路径操作功能,例如获取文件名、扩展名、绝对路径等。
import os
file_path = '/path/to/file/document.txt'
print(os.path.basename(file_path)) # 输出文件名
print(os.path.splitext(file_path)) # 输出文件名和扩展名
三、使用glob库进行文件模式匹配
glob模块提供了文件模式匹配功能,支持使用通配符查找文件。
-
基本用法
glob.glob函数接受一个模式字符串,返回匹配的文件路径列表。
import glob
pdf_files = glob.glob('/path/to/search/*.pdf')
for file in pdf_files:
print(f"Matched PDF file: {file}")
这种方法非常适合查找特定扩展名的文件。
-
递归查找
glob模块还支持递归查找,通过在模式中使用来匹配所有子目录。
pdf_files = glob.glob('/path/to/search//*.pdf', recursive=True)
这种方式可以实现对整个目录树的搜索。
四、结合网络请求下载文件
在某些情况下,我们需要从网络上下载匹配的文件,这可以通过requests库与正则表达式或其他匹配工具结合实现。
-
使用requests下载文件
requests库是Python中用于发送HTTP请求的第三方库,可以用来下载文件。
import requests
url = 'https://example.com/files/2021_report.pdf'
response = requests.get(url)
with open('2021_report.pdf', 'wb') as f:
f.write(response.content)
这个示例展示了如何从网络上下载一个PDF文件。
-
结合正则表达式匹配URL
有时,我们需要从网页中提取并下载特定的文件链接,这可以通过正则表达式实现。
import requests
import re
url = 'https://example.com/files'
response = requests.get(url)
matches = re.findall(r'https://example\.com/files/\d{4}_report\.pdf', response.text)
for match in matches:
file_response = requests.get(match)
file_name = match.split('/')[-1]
with open(file_name, 'wb') as f:
f.write(file_response.content)
这个示例展示了如何从网页中提取符合特定格式的PDF文件链接并下载。
五、综合应用与优化
在实际应用中,下载匹配文件可能需要综合使用上述多种方法,并考虑性能和效率。
-
批量下载与多线程
当需要下载大量文件时,可以使用多线程或异步IO来提高下载效率。
import threading
def download_file(url):
response = requests.get(url)
file_name = url.split('/')[-1]
with open(file_name, 'wb') as f:
f.write(response.content)
urls = ['https://example.com/files/2020_report.pdf', 'https://example.com/files/2021_report.pdf']
threads = []
for url in urls:
thread = threading.Thread(target=download_file, args=(url,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
这种方法可以显著提高下载速度。
-
错误处理与重试机制
在网络下载过程中,可能会遇到连接超时或失败的情况。可以使用重试机制来提高下载的成功率。
import requests
from requests.exceptions import RequestException
def download_with_retry(url, retries=3):
for _ in range(retries):
try:
response = requests.get(url)
file_name = url.split('/')[-1]
with open(file_name, 'wb') as f:
f.write(response.content)
break
except RequestException as e:
print(f"Error downloading {url}: {e}")
continue
这种方法可以在下载失败时进行多次重试。
通过以上方法,Python能够高效地实现匹配文件的下载,无论是本地文件还是网络资源。这些工具和技术的结合可以在实际应用中提供强大的文件管理和下载解决方案。
相关问答FAQs:
如何在Python中下载特定类型的文件?
在Python中,可以使用requests
库来下载特定类型的文件。首先,确保安装了requests库。然后,使用requests.get()
方法获取文件的URL,并将其内容写入本地文件。例如,下载一个PDF文件的代码如下:
import requests
url = 'http://example.com/file.pdf'
response = requests.get(url)
with open('downloaded_file.pdf', 'wb') as file:
file.write(response.content)
这种方法适用于任何类型的文件,只需更改URL和文件名即可。
Python中如何处理下载进度?
可以通过获取响应的内容长度来实现下载进度的显示。使用iter_content()
方法逐块下载文件并计算已下载的数据量,以下是一个简单的示例:
import requests
from tqdm import tqdm
url = 'http://example.com/largefile.zip'
response = requests.get(url, stream=True)
total_size = int(response.headers.get('content-length', 0))
with open('largefile.zip', 'wb') as file, tqdm(
desc='Downloading',
total=total_size,
unit='B',
unit_scale=True,
unit_divisor=1024,
) as bar:
for data in response.iter_content(chunk_size=1024):
file.write(data)
bar.update(len(data))
使用tqdm
库可以方便地显示进度条。
如何在Python中处理下载错误?
在下载文件的过程中,可能会遇到网络问题或文件不存在的情况。可以使用try-except
结构来处理这些错误。例如:
import requests
url = 'http://example.com/file.zip'
try:
response = requests.get(url)
response.raise_for_status() # 检查请求是否成功
with open('file.zip', 'wb') as file:
file.write(response.content)
except requests.exceptions.RequestException as e:
print(f"下载失败: {e}")
这种方式可以确保在发生错误时,程序不会崩溃,并能输出有用的错误信息。
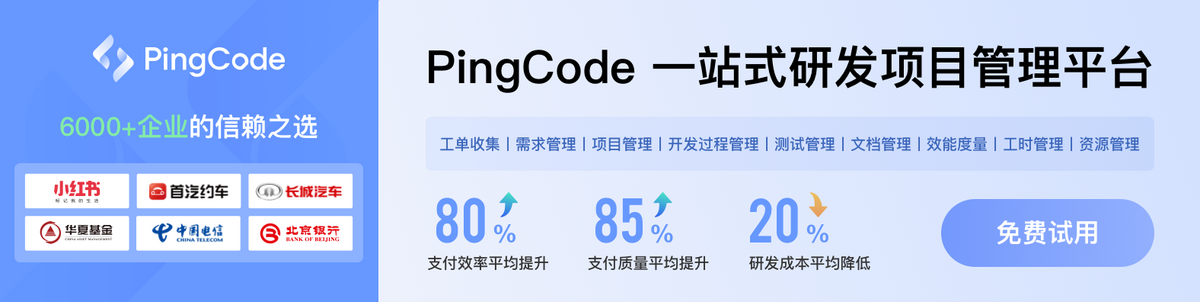