在Python中,文件复制可以通过多种方式实现,包括使用内置模块shutil
、os
模块以及手动读取和写入文件等方法。推荐使用shutil
模块,因为它提供了简单且高效的接口来进行文件复制操作。 shutil.copy()
是一个常用的函数,它能复制文件的内容和权限,但不包括元数据。shutil.copy2()
则可以复制文件的内容、权限以及元数据。os
模块可以用于更低级的文件操作,但通常需要手动处理文件流。如果需要复制整个目录,shutil.copytree()
是一个合适的选择。
以下将详细介绍不同的文件复制方法:
一、使用SHUTIL模块复制文件
shutil
模块是Python标准库的一部分,专门用于高阶文件操作,包括文件复制。
1.1、使用shutil.copy()
shutil.copy(src, dst)
是最简单的文件复制方法。它复制文件内容和权限,但不复制元数据。
import shutil
def copy_file(src, dst):
try:
shutil.copy(src, dst)
print(f"File {src} copied to {dst} successfully.")
except FileNotFoundError:
print("The source file does not exist.")
except PermissionError:
print("Permission denied.")
except Exception as e:
print(f"Error occurred: {e}")
解释:shutil.copy()
函数需要两个参数:源文件路径src
和目标路径dst
。如果目标路径是一个目录,它会将文件复制到该目录中。
1.2、使用shutil.copy2()
shutil.copy2(src, dst)
与shutil.copy()
类似,但它还复制文件的元数据,如修改时间和访问权限。
import shutil
def copy_file_with_metadata(src, dst):
try:
shutil.copy2(src, dst)
print(f"File {src} copied to {dst} with metadata successfully.")
except FileNotFoundError:
print("The source file does not exist.")
except PermissionError:
print("Permission denied.")
except Exception as e:
print(f"Error occurred: {e}")
解释:shutil.copy2()
适用于需要保留文件属性的场景。
1.3、使用shutil.copyfile()
shutil.copyfile(src, dst)
仅复制文件内容,不复制权限和元数据。目标必须是完整文件路径。
import shutil
def copy_file_strict(src, dst):
try:
shutil.copyfile(src, dst)
print(f"File {src} copied to {dst} successfully.")
except FileNotFoundError:
print("The source file does not exist.")
except PermissionError:
print("Permission denied.")
except Exception as e:
print(f"Error occurred: {e}")
解释:适用于只需复制文件内容的情况,且目标路径必须是一个文件,而不是目录。
二、使用OS模块进行文件复制
os
模块提供了更底层的文件操作功能,但需要手动处理文件流。
2.1、手动读取和写入文件
可以使用os
模块手动打开、读取和写入文件来实现复制。
import os
def copy_file_manual(src, dst):
try:
with open(src, 'rb') as fsrc:
with open(dst, 'wb') as fdst:
while True:
buf = fsrc.read(1024 * 1024)
if not buf:
break
fdst.write(buf)
print(f"File {src} copied to {dst} successfully.")
except FileNotFoundError:
print("The source file does not exist.")
except PermissionError:
print("Permission denied.")
except Exception as e:
print(f"Error occurred: {e}")
解释:这种方法提供了对复制过程的完全控制,可以处理大文件和网络流等复杂场景。
2.2、使用os.system调用操作系统命令
还可以通过os.system()
调用操作系统的cp
命令进行复制。
import os
def copy_file_os_command(src, dst):
try:
command = f"cp {src} {dst}"
os.system(command)
print(f"File {src} copied to {dst} using OS command successfully.")
except Exception as e:
print(f"Error occurred: {e}")
解释:这种方法依赖于操作系统的命令,跨平台兼容性较差,不推荐在需要移植的程序中使用。
三、复制整个目录
在许多情况下,需要复制的不仅是单个文件,而是整个目录。
3.1、使用shutil.copytree()
shutil.copytree(src, dst)
可以递归地复制整个目录树。
import shutil
def copy_directory(src, dst):
try:
shutil.copytree(src, dst)
print(f"Directory {src} copied to {dst} successfully.")
except FileExistsError:
print("The destination directory already exists.")
except FileNotFoundError:
print("The source directory does not exist.")
except PermissionError:
print("Permission denied.")
except Exception as e:
print(f"Error occurred: {e}")
解释:shutil.copytree()
自动处理子目录和文件,非常适合需要完整复制目录结构的场景。
3.2、复制目录中的特定文件类型
可以结合os
和shutil
模块复制目录中某种特定类型的文件。
import os
import shutil
def copy_specific_file_type(src, dst, file_extension):
try:
for root, dirs, files in os.walk(src):
for file in files:
if file.endswith(file_extension):
full_file_path = os.path.join(root, file)
shutil.copy(full_file_path, dst)
print(f"Files with extension {file_extension} from {src} copied to {dst} successfully.")
except FileNotFoundError:
print("The source directory does not exist.")
except PermissionError:
print("Permission denied.")
except Exception as e:
print(f"Error occurred: {e}")
解释:这种方法通过遍历源目录,找到匹配的文件类型并复制到目标目录。
四、错误处理和性能优化
在实际使用中,文件复制操作可能会遇到各种错误,需要进行适当的错误处理和性能优化。
4.1、错误处理
使用try
和except
块捕捉和处理常见错误,如FileNotFoundError
和PermissionError
。
解释:确保程序不会因未处理的异常而崩溃,并向用户提供有用的错误信息。
4.2、性能优化
对于大文件,使用较大的缓冲区(例如1MB)可以提高复制速度。
def copy_large_file(src, dst):
try:
with open(src, 'rb') as fsrc, open(dst, 'wb') as fdst:
while True:
buf = fsrc.read(1024 * 1024) # 1MB buffer
if not buf:
break
fdst.write(buf)
print(f"Large file {src} copied to {dst} successfully.")
except Exception as e:
print(f"Error occurred: {e}")
解释:使用较大的缓冲区能减少I/O操作的次数,从而提升复制性能。
4.3、多线程复制
对于需要同时复制多个文件的情况,可以使用多线程来提高效率。
import threading
def copy_file_thread(src, dst):
shutil.copy(src, dst)
print(f"File {src} copied to {dst} in a thread.")
def copy_files_multithread(file_list, dst):
threads = []
for src in file_list:
thread = threading.Thread(target=copy_file_thread, args=(src, dst))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
print("All files copied in multiple threads.")
Example usage
file_list = ['file1.txt', 'file2.txt', 'file3.txt']
copy_files_multithread(file_list, '/path/to/destination/')
解释:多线程可以在I/O操作上提升性能,但需要小心处理线程安全问题。
这些方法提供了多种方式来应对不同的文件复制需求,选择合适的方法可以大大提高程序的效率和可靠性。
相关问答FAQs:
如何使用Python复制文件?
使用Python复制文件可以通过标准库中的shutil
模块实现。shutil.copy(src, dst)
函数可以将源文件src
复制到目标路径dst
。如果目标路径是一个目录,源文件将被复制到该目录中,文件名保持不变。
在Python中复制文件时需要注意哪些事项?
在复制文件时,确保源文件路径正确,并且目标路径有写入权限。如果目标文件已经存在,shutil.copy
将覆盖它。此外,如果需要保留文件的权限和元数据,可以使用shutil.copy2(src, dst)
,它会复制文件的所有信息。
Python是否支持批量复制文件?
是的,Python可以通过遍历文件夹中的文件来实现批量复制。可以使用os
模块结合shutil
模块,遍历指定目录下的所有文件,并将它们复制到目标目录。这样可以一次性处理多个文件,提高效率。
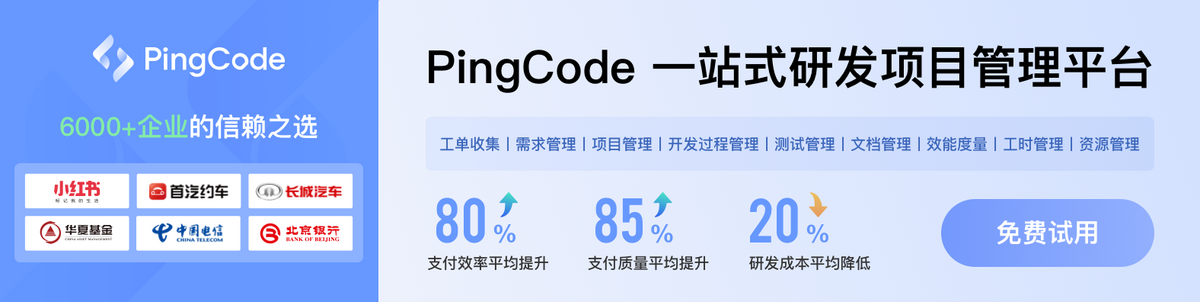